The Gaussian distribution in MATLAB can be generated using the `normpdf` function to compute the probability density function for a specified mean and standard deviation.
Here's an example of how to create and plot a Gaussian distribution in MATLAB:
mu = 0; % mean
sigma = 1; % standard deviation
x = mu-4*sigma:0.1:mu+4*sigma; % x values
y = normpdf(x, mu, sigma); % Gaussian distribution values
plot(x, y); % plot the distribution
title('Gaussian Distribution');
xlabel('X-axis');
ylabel('Probability Density');
Understanding Gaussian Distribution
Definition of Gaussian Distribution
The Gaussian distribution, also known as the normal distribution, is a fundamental concept in statistics characterized by its symmetrical, bell-shaped curve. It is defined mathematically by the formula:
\[ f(x) = \frac{1}{\sigma \sqrt{2\pi}} e^{-\frac{(x - \mu)^2}{2\sigma^2}} \]
where:
- \( \mu \) is the mean,
- \( \sigma \) is the standard deviation,
- \( e \) is the base of the natural logarithm, and
- \( x \) represents the variable.
This distribution is pivotal in various realms—statistical inference, hypothesis testing, and more—due to its unique properties.
Properties of Gaussian Distribution
One of the most remarkable attributes of the Gaussian distribution is its symmetry around the mean. The mean, median, and mode of the distribution coincide, situated at the peak of the curve. The width of the curve is determined by the standard deviation:
- The standard deviation represents the dispersion of data around the mean.
- Approximately 68% of the data falls within one standard deviation, 95% within two, and 99.7% within three, forming the well-known Empirical Rule.
Applications of Gaussian Distribution
The Gaussian distribution stretches across various applications in real-world scenarios, including natural phenomena like heights, test scores, and measurement errors. Its significance extends into fields such as finance, where it models asset returns, and machine learning, where it aids in algorithms like Gaussian Naive Bayes.
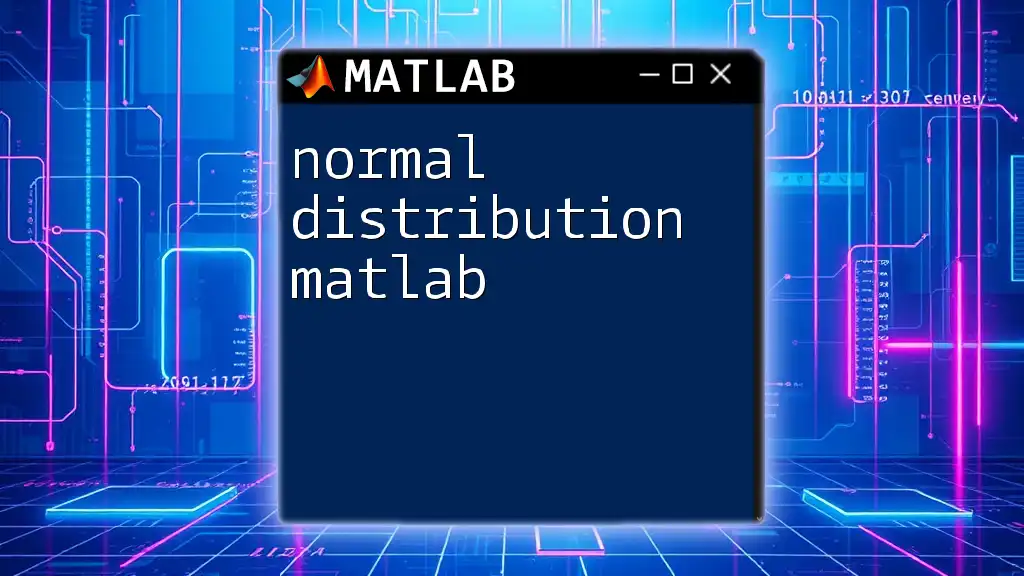
Gaussian Distribution in MATLAB
Overview of MATLAB
MATLAB is an incredible tool for numerical computing and data analysis. Its built-in functions allow users to seamlessly generate, manipulate, and analyze Gaussian distributions, making it an optimal choice for statisticians, data scientists, and engineers.
Generating Gaussian Distributed Data
Creating Random Gaussian Data
In MATLAB, you can easily create random data that follows a Gaussian distribution using the `randn` function. This function generates values from the standard normal distribution, which can be scaled and shifted to match your required mean (\( \mu \)) and standard deviation (\( \sigma \)).
Here is a code snippet to generate Gaussian distributed data:
mu = 0; % Mean
sigma = 1; % Standard deviation
numPoints = 1000;
data = mu + sigma * randn(numPoints, 1);
This code will create \( 1000 \) normally distributed data points centered around \( \mu \) with a standard deviation of \( \sigma \).
Visualizing Gaussian Data
To assess your generated data visually, a histogram is an effective tool. You can combine this with the theoretical probability density function (PDF) for clarity.
Here's a code snippet that demonstrates how to create a histogram and overlay the corresponding Gaussian curve:
histogram(data, 'Normalization', 'pdf');
hold on;
x = linspace(-4, 4, 100);
y = normpdf(x, mu, sigma);
plot(x, y, 'r', 'LineWidth', 2);
title('Histogram of Gaussian Distributed Data');
hold off;
This graphical representation provides insight into how your data aligns with the expected Gaussian distribution.
Analyzing Gaussian Distribution
Fitting Data to a Gaussian Distribution
To analyze empirical data and validate its conformity to a Gaussian distribution, you can use MATLAB's `fitdist` function. This function will fit a normal distribution to your dataset, providing useful statistics about the fitted model.
The snippet below fits a model to the generated data:
pd = fitdist(data, 'Normal'); % Fit data to normal distribution
Parameters of the Fitted Distribution
After fitting your data, you might want to extract the parameters of the fitted distribution, primarily the mean and standard deviation.
You can do this with the following code:
muFitted = pd.mu;
sigmaFitted = pd.sigma;
These parameters allow you to understand how well your data aligns with the theoretical distribution.
Goodness-of-Fit Tests
To ensure that your data accurately follows a Gaussian distribution, performing goodness-of-fit tests is essential. Visual tools, such as Q-Q plots, can help you assess the fit quality.
Here’s how to create a Q-Q plot in MATLAB:
qqplot(data);
title('Q-Q Plot for Gaussian Fit');
This plot helps visualize the correspondence between your data quantiles and the theoretical quantiles of a normal distribution.
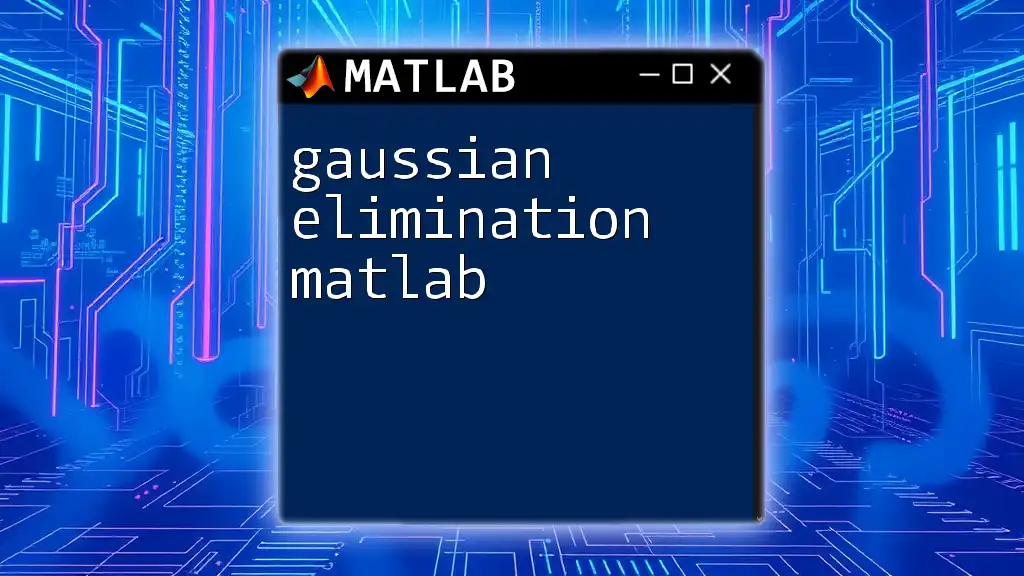
Advanced Techniques in MATLAB
Creating Custom Gaussian Functions
While MATLAB offers numerous built-in functions, defining your custom Gaussian function can enhance your flexibility and understanding. Below is an example of a user-defined function for the Gaussian probability density function:
function y = customGaussian(x, mu, sigma)
y = (1/(sigma*sqrt(2*pi))) * exp(-0.5*((x-mu)/sigma).^2);
end
This function can be called to calculate the Gaussian value at any point you specify.
Simulating Gaussian Processes
Gaussian processes are essential in various statistical modeling contexts. In MATLAB, simulating such processes can be straightforward, especially for maintaining relationships across data points.
Here’s an example that illustrates how to simulate a simple Gaussian process:
% Example of how to simulate a Gaussian process
t = linspace(0, 10, 100);
K = @(x1, x2) exp(-0.5 * (x1 - x2').^2); % Covariance function
mu = zeros(size(t));
covMatrix = K(t, t);
f = mvnrnd(mu, covMatrix);
plot(t, f);
title('Simulated Gaussian Process');
This simulation demonstrates how to generate correlated samples that follow a Gaussian distribution over time.
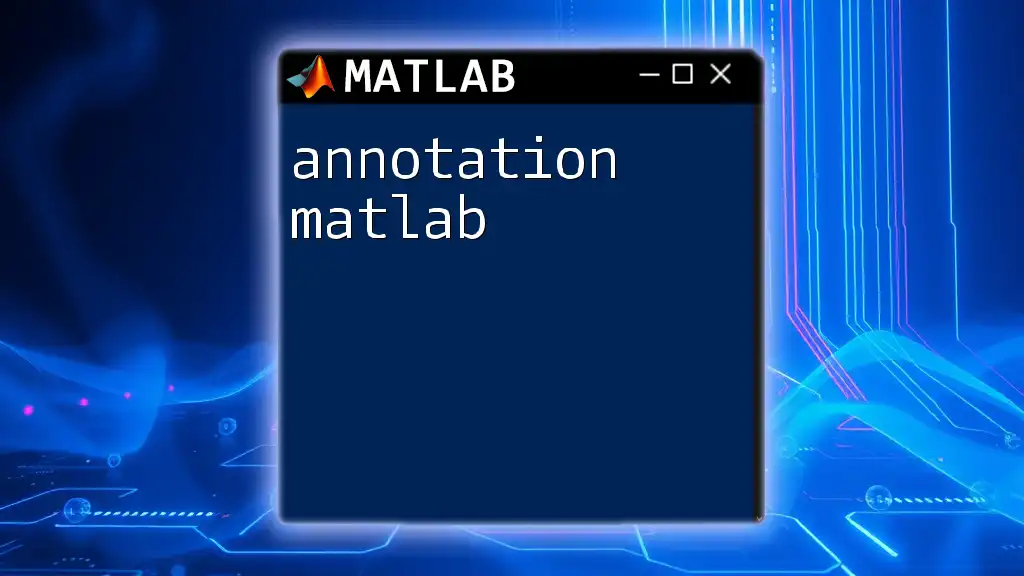
Conclusion
Throughout this guide, we explored the intricate properties and applications of the Gaussian distribution in MATLAB. From generating random data to fitting models and creating custom functions, MATLAB provides powerful tools for handling Gaussian distributions effectively.
As you practice these commands and techniques, you'll gain deeper insights into statistical data analysis. We encourage you to explore further with hands-on projects and utilize the resources available to deepen your understanding.
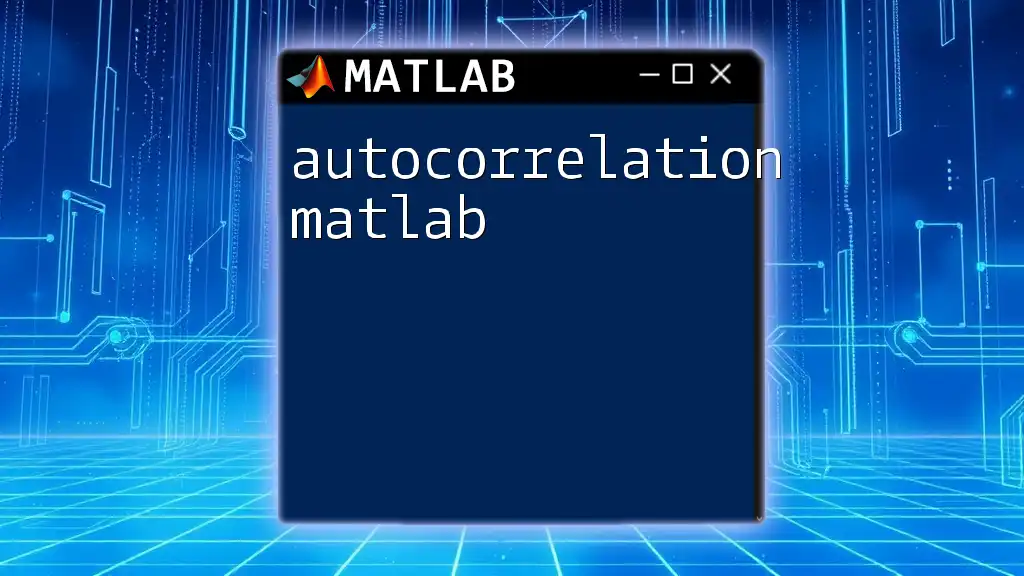
Additional Resources
For those looking to broaden their knowledge further, consider exploring MATLAB’s official documentation. There, you can find in-depth resources and tutorials to enrich your understanding of Gaussian distributions and beyond. Engaging with practical projects is another excellent way to strengthen your skills in utilizing MATLAB for statistical analysis.