Partial fraction decomposition in MATLAB allows you to express a rational function as a sum of simpler fractions, making it easier to analyze and compute integrals.
Here is a code snippet demonstrating how to perform partial fraction decomposition in MATLAB:
syms x
num = 2*x^2 + 3*x + 1; % Numerator
den = x^3 + x^2 + x; % Denominator
partialFraction = partfrac(num/den); % Perform partial fraction decomposition
disp(partialFraction);
Understanding Partial Fraction Decomposition
Partial fraction decomposition is a critical technique in algebra that allows us to break down complex rational functions into simpler fractions. This method is especially important in control systems and signal processing, where it facilitates easier manipulation of functions for analysis and integration.
Why Use MATLAB for Partial Fractions?
MATLAB is a powerful tool for mathematical computations, providing an array of built-in functions that simplify complex calculations. By using MATLAB for partial fraction decomposition, you gain the ability to handle intricate algebraic expressions quickly and efficiently. The software’s symbolic toolbox also allows you to visualize equations, making it easier to understand the decomposition process.
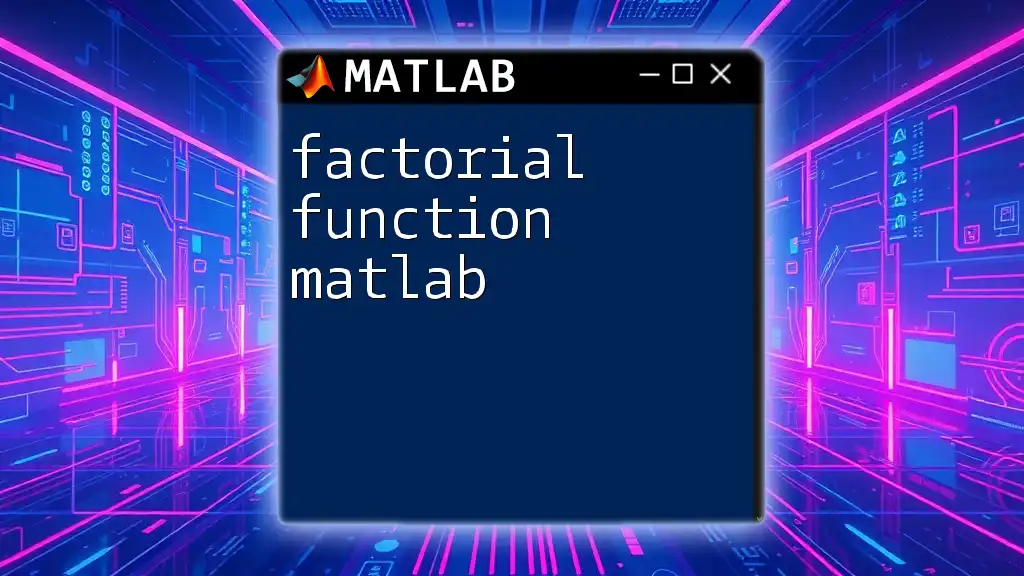
Getting Started with MATLAB
Before delving into partial fraction decomposition, it’s essential to have MATLAB set up on your machine.
Installing MATLAB
Installing MATLAB is straightforward. Make sure you download the latest version from [MathWorks](https://www.mathworks.com), as it includes enhancements and bug fixes that can improve your experience. Consider opting for the Symbolic Math Toolbox, as it provides essential features for handling algebraic expressions.
Essential MATLAB Commands
Familiarize yourself with basic MATLAB commands that will help you navigate the environment. Key commands include:
- `clc`: Clears the command window.
- `clear`: Removes all variables from the workspace.
- `close all`: Closes all figure windows.
Understanding these commands will ease your transition into more complex tasks.
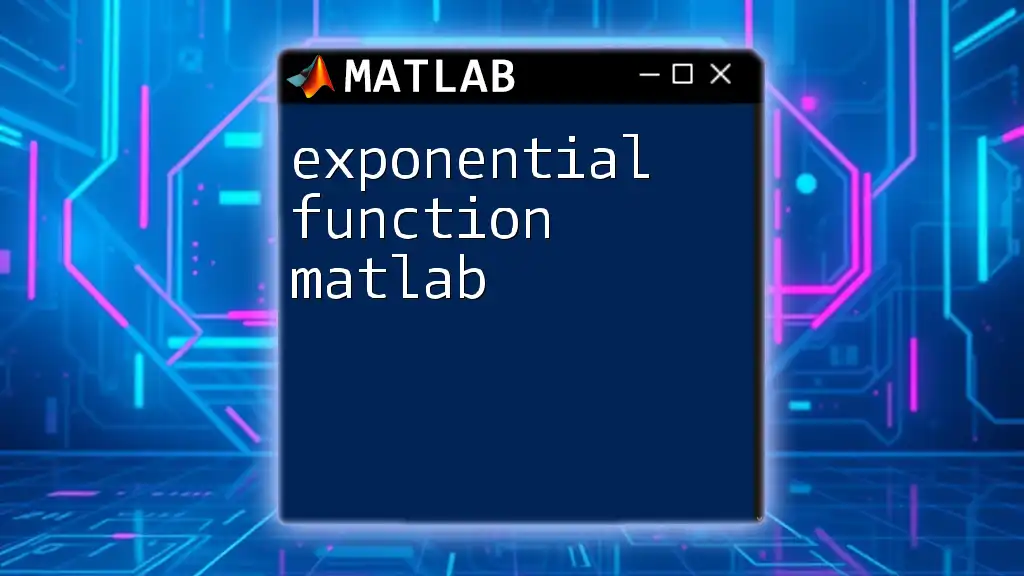
Understanding Polynomial Functions
A firm grasp of polynomial functions is necessary for effective partial fraction decomposition.
Basics of Polynomial Functions
Polynomial functions are represented in the form \( P(x) = a_n x^n + a_{n-1} x^{n-1} + ... + a_1 x + a_0 \), where \( a_i \) are coefficients and \( n \) is a non-negative integer (the degree of the polynomial). Understanding the degree, coefficients, and terms in a polynomial is essential for identifying how to break down rational functions.
Types of Polynomials in Partial Fractions
Partial fraction decomposition specifically deals with two types of fractions: proper and improper. A proper fraction has a degree in the numerator lower than that of the denominator, while an improper fraction has a numerator with a degree equal to or greater than that of the denominator. Identifying the type involved will dictate the next steps in the decomposition process.
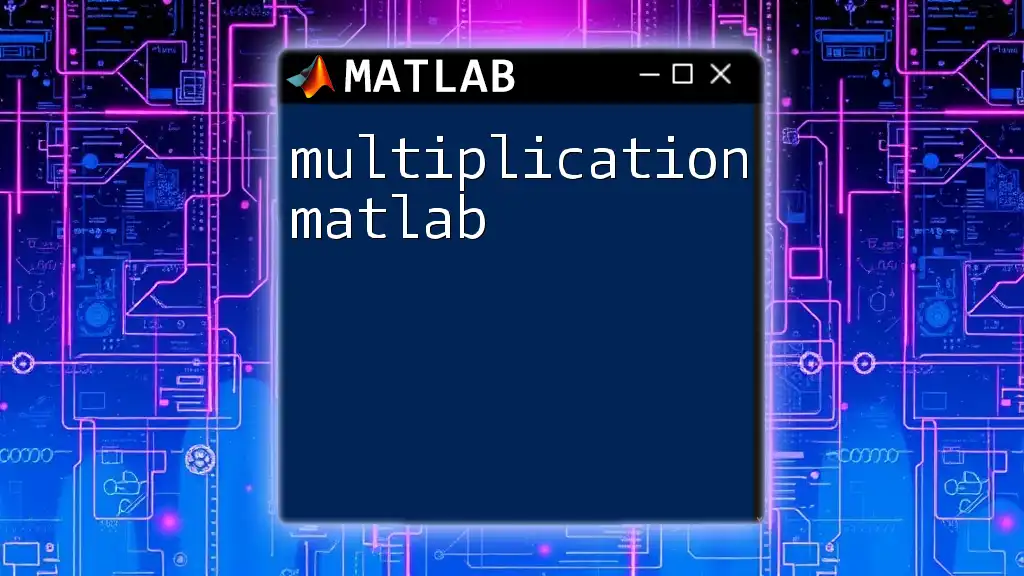
Performing Partial Fraction Decomposition in MATLAB
The primary MATLAB function for partial fraction decomposition is `residue`, which automatically calculates the residues, poles, and direct term.
The `residue` Function
The syntax of the `residue` function is as follows:
[R, P, K] = residue(b, a)
Where:
- `b`: Coefficients of the numerator.
- `a`: Coefficients of the denominator.
- `R`: Residues.
- `P`: Poles of the decomposition.
- `K`: Direct term (if applicable).
Breakdown of Parameters
Understanding these parameters is crucial:
- Numerator and Denominator: When providing `b` and `a`, ensure that you’re using the correct coefficients for the numerator and the denominator, respectively.
- Residues (R): These represent the coefficients of the partial fractions in the final output.
- Poles (P): These indicate the locations (roots) of the denominators in the partial fractions.
- Direct Term (K): This is applicable when the numerator has a degree greater than or equal to the denominator.
Step-by-Step Example
Let us work through an example to see how partial fraction decomposition operates with a rational function. Consider the function:
\[ f(x) = \frac{x}{(x^2 + 1)(x - 2)} \]
To decompose this function, follow these steps using MATLAB:
- Define the Function: Use symbolic variables to define your rational function.
syms x
f = x / ((x^2 + 1)*(x - 2));
- Obtain Numerator and Denominator:
[b, a] = numden(f);
- Apply the `residue` Function:
[R, P, K] = residue(b, a)
After running this code, MATLAB will return the residues, poles, and any direct term associated with the input function.
Interpretation of Output
The output will include the residues, which serve as the coefficients in the partial fractions, and the poles, which indicate the roots of the denominator that contribute to the decomposition.
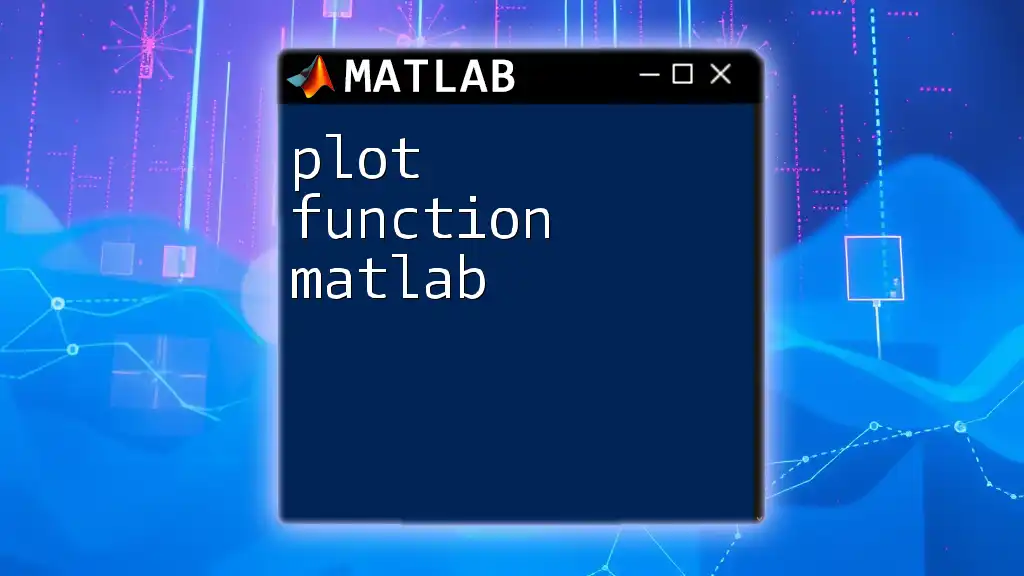
Advanced Techniques in Partial Fraction Decomposition
While the basics of partial fraction decomposition are essential, there are more advanced techniques that can prove useful.
Handling Complex Poles
Complex poles arise when the denominator has no real roots. When you encounter such situations, MATLAB can still handle these effectively. When performing decomposition, ensure that the output represents these complex terms correctly.
Example with Complex Poles
Consider the following function which includes complex terms:
f = (x^2 + 1)/(x^2 - 2);
[b, a] = numden(f);
[R, P, K] = residue(b, a)
MATLAB will yield outputs that correctly represent the residues associated with the complex poles, allowing you to work with functions that might seem daunting at first glance.
Dealing with Repeated Factors
In cases where the denominator contains repeated linear factors, the partial fraction decomposition will require additional terms representing these repetitions.
For example, if your function has \( (x - 2)^2 \) as part of the denominator, your decomposition may look like this:
f = x/((x - 2)^2*(x^2 + 1));
[b, a] = numden(f);
[R, P, K] = residue(b, a)
This will yield terms that correspond to both \( \frac{A}{x-2} \) and \( \frac{B}{(x-2)^2} \).
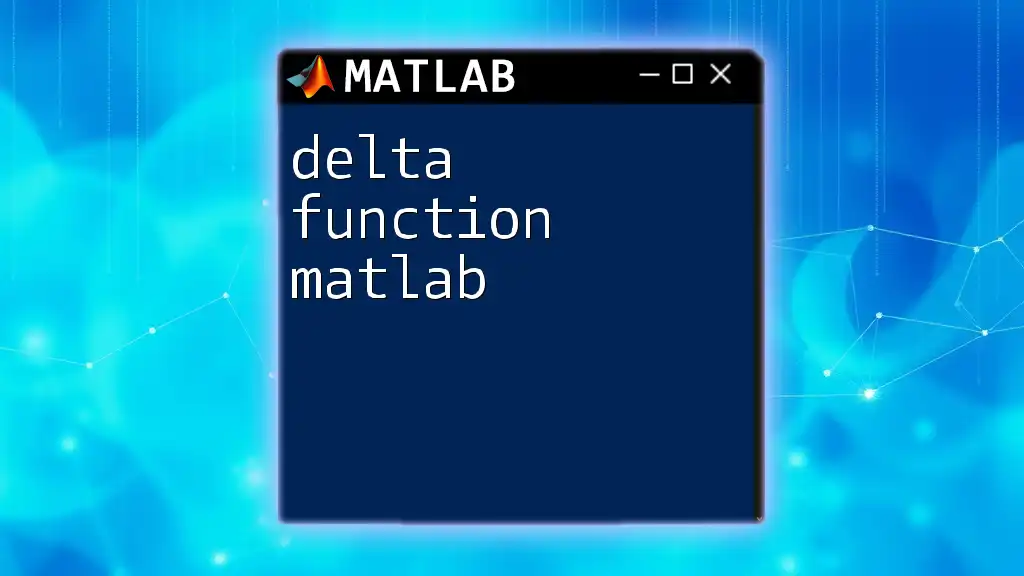
Visualizing Results
Once you have performed the decomposition, visualizing your function and its partial fractions can enhance understanding.
Plotting Functions in MATLAB
Utilize MATLAB's powerful plotting functions to visually represent your original function alongside its decomposed parts. For example, use the following code to plot:
fplot(f, [-10, 10])
This command will render a graph of the function over the interval [-10, 10]. You will be able to see how the rational function behaves and how it can be approximated with simpler terms.
Interpretation of the Plots
The plots generated from your calculations will illuminate the function’s nuances. By comparing the original with the partial fractions, you can gain insights into how each part contributes to the overall function and what impact it has on integration or further analysis.
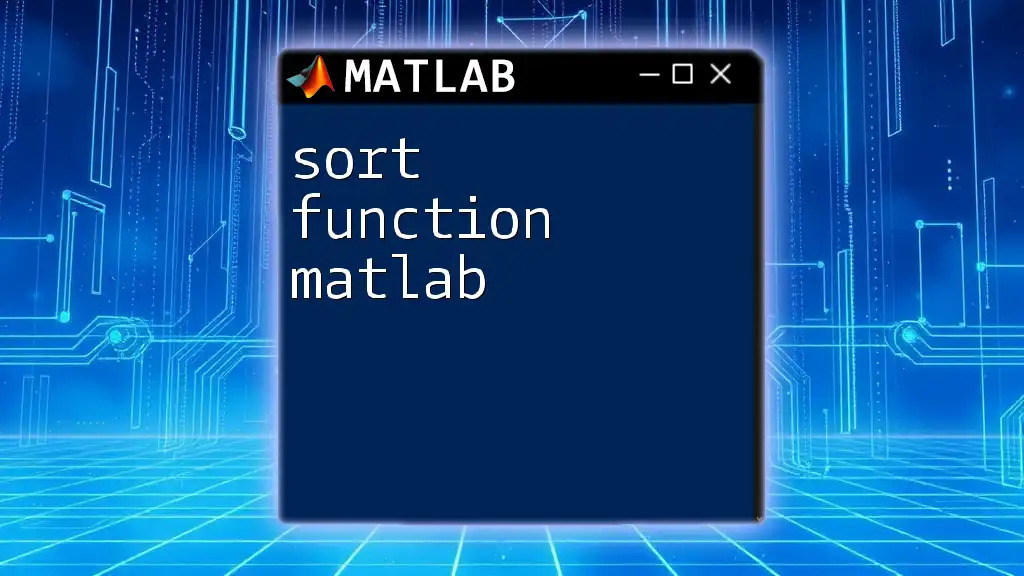
Common Errors and Troubleshooting
As you navigate working with partial fractions in MATLAB, you may encounter various challenges.
Common Mistakes
One of the typical errors includes incorrectly defining the numerator and denominator, which can lead to misleading results. Ensure you are using `numden` properly to avoid this pitfall.
Debugging Tips
If you find yourself facing unexpected outputs, consider the following:
- Verify that the degrees of the numerator and denominator are correctly identified.
- Check if the function is proper before attempting decomposition.
- Utilize MATLAB’s debugging tools, such as `disp`, to check intermediate values.
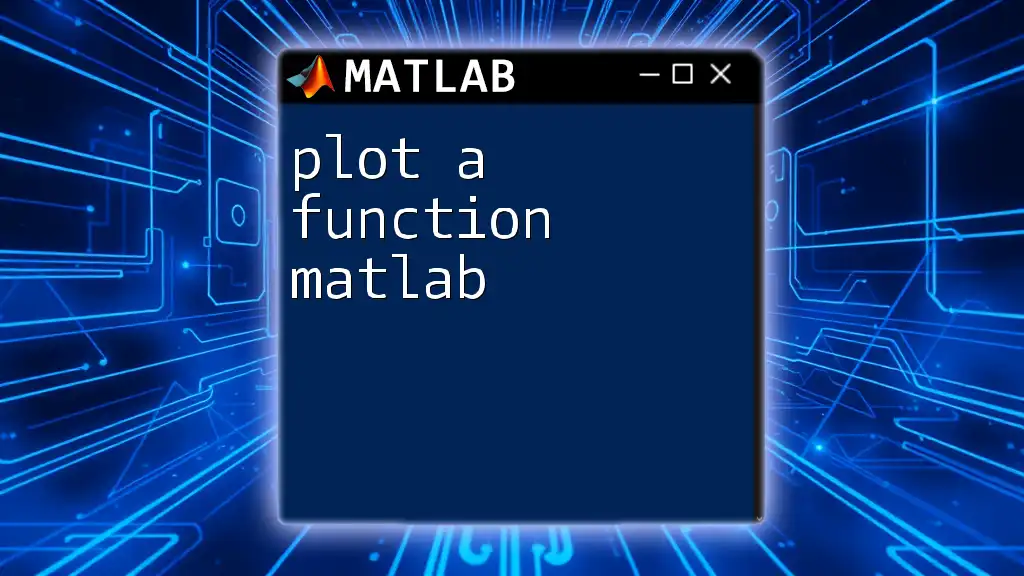
Conclusion
In summary, mastering the method of partial fraction decomposition using MATLAB can significantly enhance your mathematical toolkit. By understanding the underlying principles, implementing practices correctly, visualizing outputs, and troubleshooting common errors, you can leverage this powerful technique for a variety of applications.
Next Steps for Learners
Continue exploring MATLAB's capabilities by practicing with increasingly complex rational functions. Experiment with different types of polynomials and their decompositions to gain confidence in your skills.
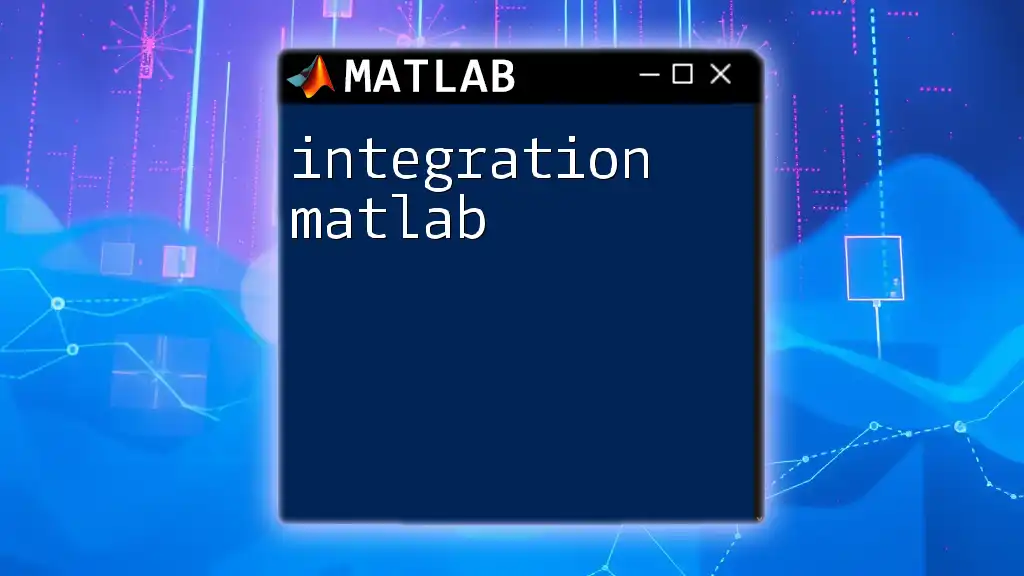
Additional Resources
For further learning, consider visiting MATLAB's official documentation, engaging with user forums, or enrolling in specialized courses that focus on both symbolic mathematics and MATLAB programming. These resources will provide additional insights and techniques to enhance your understanding of partial fractions.
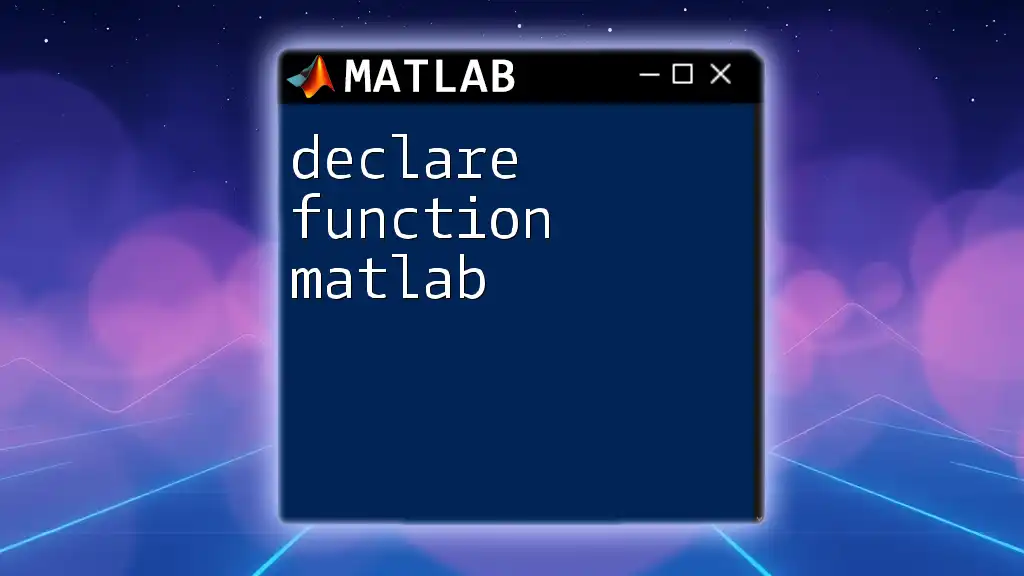
FAQs about Partial Fraction Decomposition in MATLAB
Frequently Asked Questions
-
What if my numerator is of the same degree as my denominator?
- If the degrees are equal or the numerator is of a higher degree, you must first perform polynomial long division.
-
Can MATLAB handle multiple variables in partial fractions?
- While the primary focus here is on single-variable functions, MATLAB can also manage partial fraction decomposition for multivariable rational functions, though it may require different approaches or functions.
By applying these techniques and insights, you’ll be well-equipped to use partial fraction decomposition in MATLAB effectively, unlocking new doors in both analysis and applied mathematics.