Integration in MATLAB allows users to compute the definite and indefinite integrals of functions using built-in functions such as `integrate` and numerical methods like `integral`.
Here's a simple example of how to perform both symbolic and numerical integration in MATLAB:
% Symbolic integration
syms x
f = x^2;
indefinite_integral = int(f, x)
% Numerical integration
numerical_integral = integral(@(x) x.^2, 0, 1)
Understanding the Basics of Integration
What is Integration?
Integration is one of the fundamental concepts in calculus, signifying the process of finding the integral of a function, which can be understood as the "reverse" operation of differentiation. In mathematical terms, integration is used to calculate areas, volumes, and other quantities that arise from summing infinitesimal parts together.
There are two primary types of integrals:
- Definite Integrals: These evaluate the accumulation of a quantity between two specified bounds, yielding a numerical value representing the area under a curve.
- Indefinite Integrals: These represent a family of functions and include a constant of integration, often depicted as \( C \).
The Fundamental Theorem of Calculus
The Fundamental Theorem of Calculus connects the concept of differentiation with integration, indicating that if a function \( F \) is the integral of \( f \) on an interval \([a, b]\), then: \[ F(b) - F(a) = \int_{a}^{b} f(x) \, dx \]
This theorem is essential for evaluating definite integrals and highlights the relationship between the area under a curve and the antiderivative of a function.
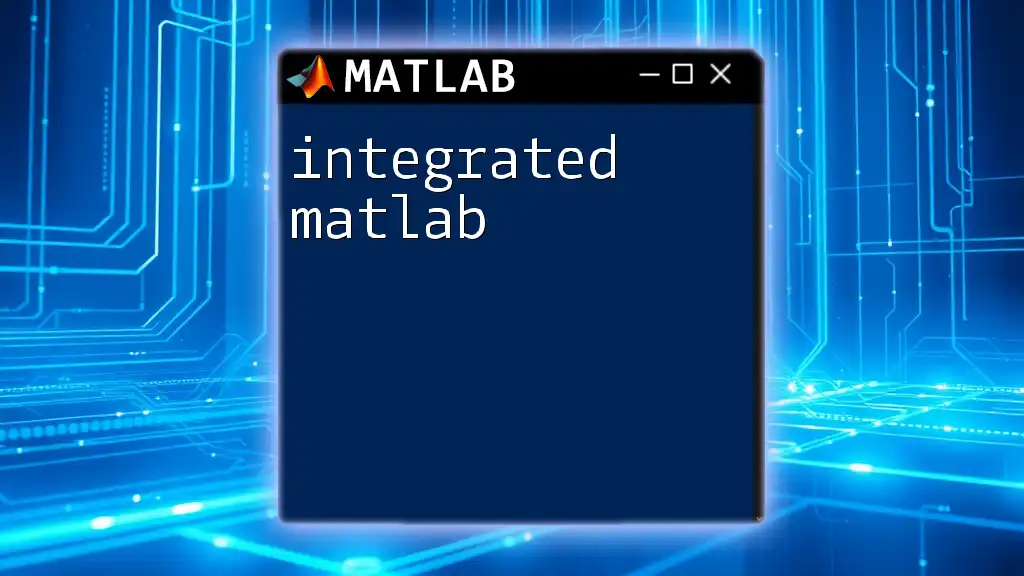
MATLAB's Integration Functions
Overview of MATLAB’s Integration Capabilities
MATLAB provides a wide variety of tools to perform integration, catering to both numerical and symbolic needs. The choice between symbolic and numerical integration often depends on the specific requirements of the problem at hand.
Symbolic Integration with `syms`
Using symbolic math in MATLAB allows you to derive exact mathematical expressions rather than numerical approximations. To utilize symbolic variables, you must first declare them with the `syms` command:
syms x
Following this, you can perform symbolic integration using the `int` function. Here’s an example:
integral_result = int(x^2, x)
The result of this command will provide the indefinite integral of \( x^2 \), which is \( \frac{x^3}{3} + C \). This illustrates the power of symbolic computation in obtaining precise answers.
Numerical Integration Methods
Numerical integration techniques are vital when you cannot find an antiderivative or when working with empirical data. MATLAB offers several functions for this purpose.
Using `integral()`
The `integral` function is a modern and versatile choice for performing definite integrals. Its usage includes defining a function handle and specifying limits:
f = @(x) x.^2; % Function handle definition
area = integral(f, 0, 1) % Computes the definite integral from 0 to 1
By default, `integral()` is adaptive, automatically adjusting its method based on the behavior of the function to improve accuracy.
Using `quad` and `quadl`
While `integral` is typically recommended for most cases, MATLAB also includes legacy functions like `quad` and `quadl` for numerical integration. Here is how you can implement each:
area = quad(@(x) x.^2, 0, 1); % Traditional quad integration
area_l = quadl(@(x) x.^2, 0, 1); % Quadl for fewer oscillations
Both functions will calculate the area under \( x^2 \) within the defined limits, but `quadl` tends to be more efficient for smoother functions.
Integrating Multiple Dimensions with `integral2` and `integral3`
For multidimensional integration, MATLAB has specialized functions known as `integral2` for double integrals and `integral3` for triple integrals. Here's how to implement each:
area_double = integral2(@(x,y) x.*y, 0, 1, 0, 1); % Double integral
volume = integral3(@(x,y,z) x.*y.*z, 0, 1, 0, 1, 0, 1); % Triple integral
The first command calculates the area under the surface defined by \( xy \) over the unit square, while the second computes the volume under the surface \( xyz \) within the unit cube.
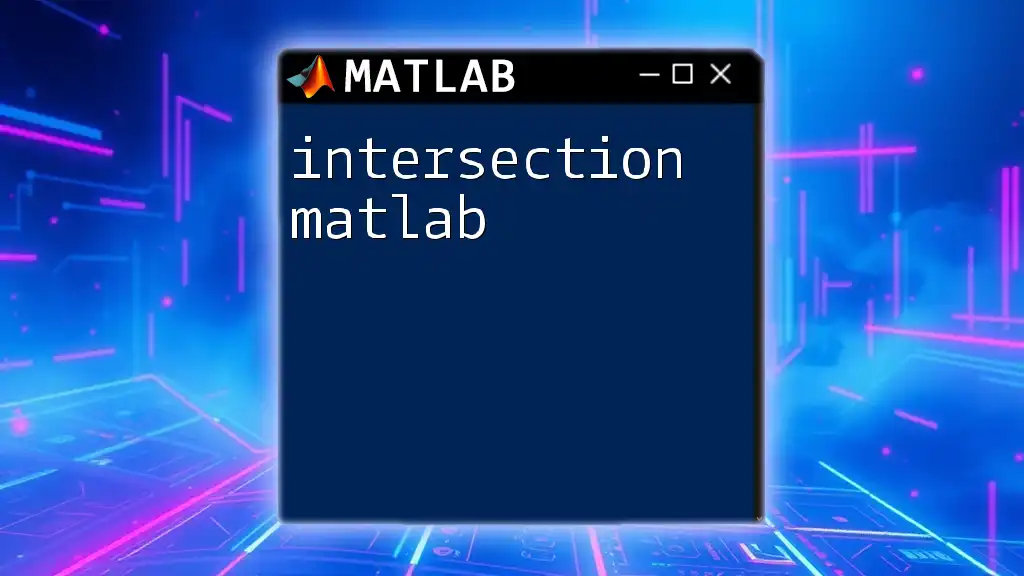
Special Integration Techniques in MATLAB
Adaptive Quadrature Methods
MATLAB's numerical integration methods primarily rely on adaptive quadrature, which refines the partitioning of an interval where the function may behave unpredictably (e.g., having sharp peaks). By allowing MATLAB to make these adjustments automatically, you ensure high accuracy with fewer computational resources.
Handling Numerical Issues: Improper Integrals
Improper integrals, which extend infinitely or have infinite discontinuities, can also be computed in MATLAB. As an example, consider the integral of \( \frac{1}{x^2} \) from 1 to infinity:
improper_area = integral(@(x) 1./(x.^2), 1, Inf); % Integral from 1 to infinity
This command effectively computes the area under the curve as \( x \) approaches infinity, an essential tool for many applications in physics and engineering.
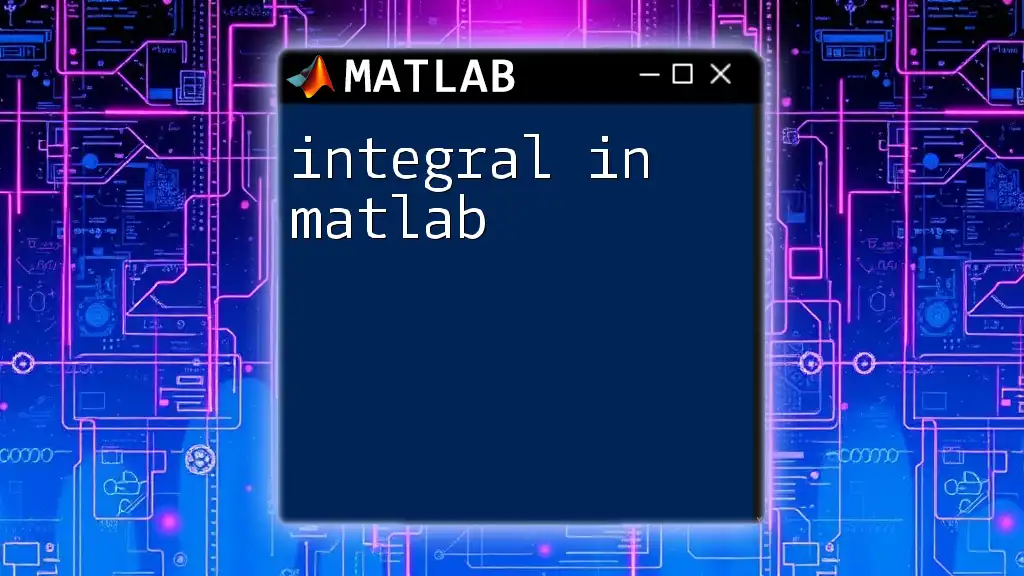
Visualization of Integration Results
Plotting Functions and Areas
Visualizing the results of integration not only aids in understanding but also provides insight into the behavior of functions. MATLAB's powerful plotting capabilities can be used to create clear representations:
f = @(x) x.^2;
fplot(f, [0 1]);
hold on; % Retain current plot for overlay
x = linspace(0, 1, 100);
fill([x 1 0], [0 f(x) 0], 'r', 'FaceAlpha', 0.5) % Fill under the curve
In this code, `fplot` graphs the function \( x^2 \) from 0 to 1, while the `fill` command shades the area beneath the curve for visual clarity.
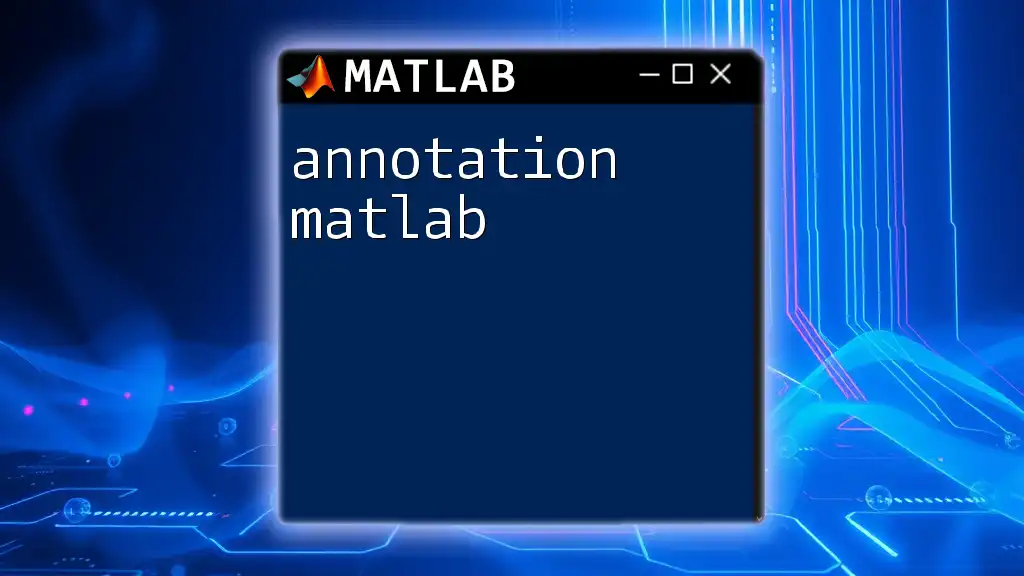
Practical Applications of Integration in MATLAB
Real-world Examples
Area under a curve: Engineers often use integration to calculate quantities like material stress, fluid pressure, and electrical charge.
Statistical applications: Integrating probability density functions can yield cumulative distribution functions, vital in statistics and data analysis.
Case Study: Velocity and Acceleration
Integration plays a critical role in physics, particularly concerning motion. For instance, when you have a velocity function, integrating it allows you to derive displacement over time:
syms t
v = t^2; % Define velocity function
displacement = int(v, t) % Calculate displacement
In this example, you calculate the total displacement by integrating the velocity function.
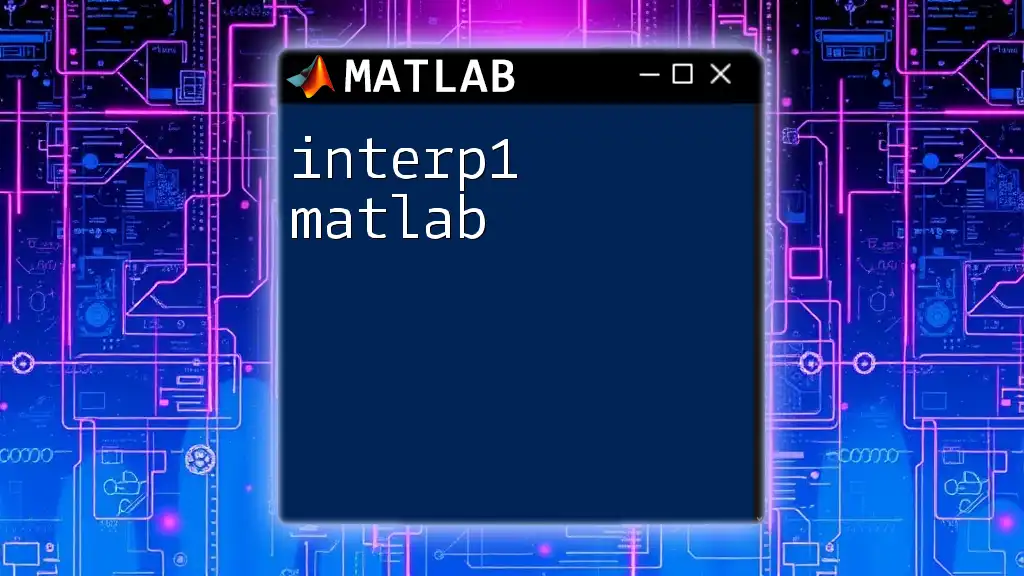
Conclusion
This guide provides a comprehensive overview of integration in MATLAB, covering symbolic and numerical methods along with practical applications. Using MATLAB for integration not only enhances computational efficiency but also allows for deeper engagement with mathematical concepts. By mastering these techniques, you can tackle a wide range of problems in engineering, physics, statistics, and beyond.