LU factorization in MATLAB is a technique used to decompose a matrix into a lower triangular matrix (L) and an upper triangular matrix (U), which can be performed using the `lu` function.
Here’s a code snippet to demonstrate LU factorization in MATLAB:
A = [4, 3; 6, 3];
[L, U] = lu(A);
Understanding the Concept of LU Factorization
What is LU Factorization?
LU Factorization is a mathematical technique that decomposes a square matrix \( A \) into two distinct matrices: a lower triangular matrix \( L \) and an upper triangular matrix \( U \). This means we can express the original matrix as:
\[ A = LU \]
Where:
- \( L \) is a lower triangular matrix (elements above the main diagonal are zero).
- \( U \) is an upper triangular matrix (elements below the main diagonal are zero).
Importance
LU Factorization is especially useful in solving linear equations, inverting matrices, and computing determinants efficiently. It transforms complex mathematical operations into simpler steps.
Why Use LU Factorization?
Efficiency in Computations is a hallmark of LU Factorization. When faced with a system of linear equations, decomposing the matrix into \( L \) and \( U \) allows us to solve the equations in two steps, namely forward and backward substitutions, thereby significantly reducing computational complexity.
When compared to other methods like Gaussian elimination, LU Factorization stands out because it allows us to reuse the decomposed matrices \( L \) and \( U \) for multiple right-hand sides of a linear system without repeating the entire elimination process.
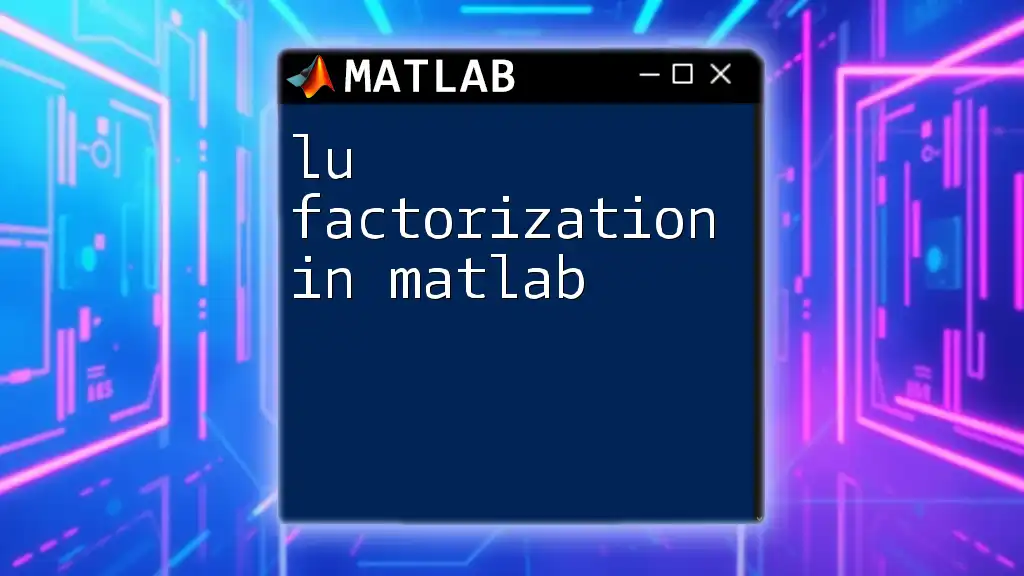
Implementing LU Factorization in MATLAB
Setting Up Your Environment
Before jumping into LU Factorization in MATLAB, it is crucial to ensure that the MATLAB environment is set up correctly. Launch MATLAB, and familiarize yourself with the interface. Ensure that you have access to required toolboxes, especially the MATLAB Basic Operations Toolbox, which is typically included in standard installations.
Using Built-in Functions for LU Factorization
MATLAB's `lu` Function
MATLAB provides a built-in function specifically for LU Factorization, known as `lu`. The function's syntax is straightforward, and its implementation is efficient, allowing for rapid decomposition of matrices.
Here's a simple example of how to use the `lu` function:
A = [4 3; 6 3];
[L, U] = lu(A);
disp('Lower Triangular Matrix L:');
disp(L);
disp('Upper Triangular Matrix U:');
disp(U);
In the above example, we determine \( L \) and \( U \) for the matrix \( A \). The resulting matrices can be displayed directly in the MATLAB command window.
Interpreting the Output
The output matrices \( L \) and \( U \) show the decomposition result:
- \( L \) contains the multipliers used during the elimination process.
- \( U \) retains the echelon form of \( A \).
This decomposition allows for easy resolution of linear equations subsequently.
Manual LU Factorization
Step-by-Step Process
Engaging with manual LU Factorization promotes deeper understanding. Consider the following sample matrix \( A \):
A = [2 3 1; 4 8 2; 6 10 3];
To perform LU Factorization manually, follow these steps:
- Initialization: Set \( L \) as an identity matrix and \( U \) as the original matrix \( A \).
n = size(A, 1);
L = eye(n); % Initialize L as an identity matrix
U = A; % Start U as the original matrix A
- Row Operations: Using nested loops, perform the necessary row operations to create matrices \( L \) and \( U \).
for i = 1:n
for j = i+1:n
L(j,i) = U(j,i) / U(i,i);
U(j,:) = U(j,:) - L(j,i) * U(i,:);
end
end
This snippet effectively modifies \( U \) through subtraction while updating \( L \) with the scaling factors.
Handling Pivoting
What is Pivoting?
Numerical stability is of utmost importance in computations. Pivoting refers to rearranging the rows (or columns) of the matrix to ensure the largest element is used as a pivot, thus improving accuracy and mitigating rounding errors during calculations.
Partial Pivoting in MATLAB
In MATLAB, partial pivoting can be easily included using the same `lu` function by obtaining a permutation matrix \( P \), such that:
[L, U, P] = lu(A);
The permutation matrix \( P \) allows the original matrix \( A \) to stay aligned with the reordered \( L \) and \( U \), ensuring better numerical properties.
Understanding the Permutation Matrix
The permutation matrix \( P \) serves as a corrective factor to aid in accurately transforming \( A \) into \( L \) and \( U \). It allows for managing cases of zero or small pivot entries effectively.
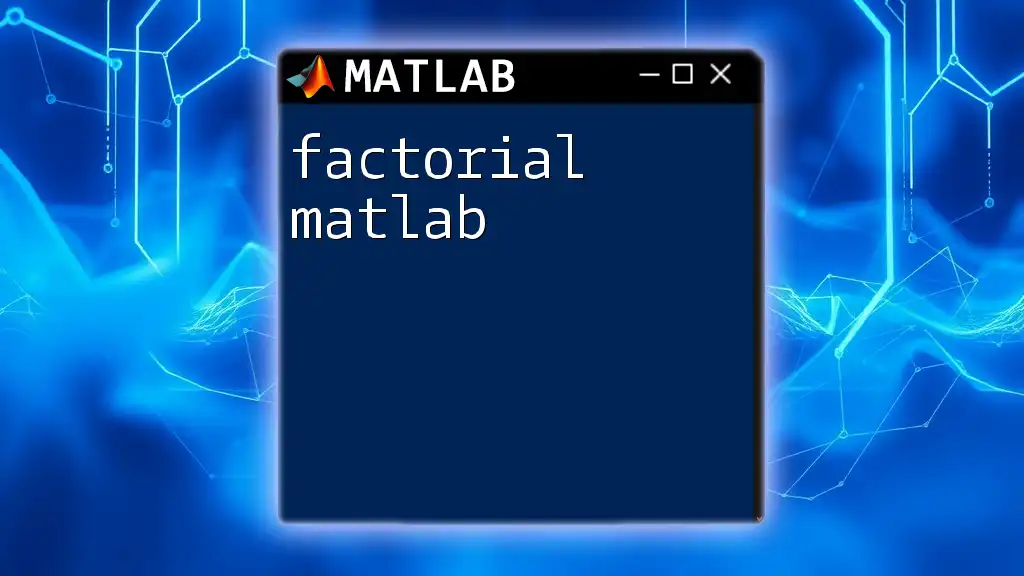
Applications of LU Factorization
Solving Systems of Linear Equations
One of the most common applications of LU Factorization is in solving systems of linear equations. Let's consider the following system represented in matrix form:
b = [5; 9; 12];
To solve for \( x \) in \( Ax = b \) using LU Factorization, you first conduct forward substitution to find \( y \):
y = L \ (P * b); % Forward substitution
Then, backward substitution to find \( x \):
x = U \ y; % Back substitution
This two-step process is much faster than solving the entire system directly using methods like Gaussian elimination.
Numerical Stability and Condition Number
Understanding Numerical Stability
In numerical methods, numerical stability is vital. LU Factorization aids in this by maintaining rounded errors manageable, especially when dealing with ill-conditioned matrices.
Condition Number
The condition number of a matrix indicates how changes in input can affect changes in output. A matrix with a high condition number is considered ill-conditioned, making direct solutions prone to errors. Implementing LU Factorization can help maintain stability in these scenarios, as we mitigate computational errors through strategic decomposition.
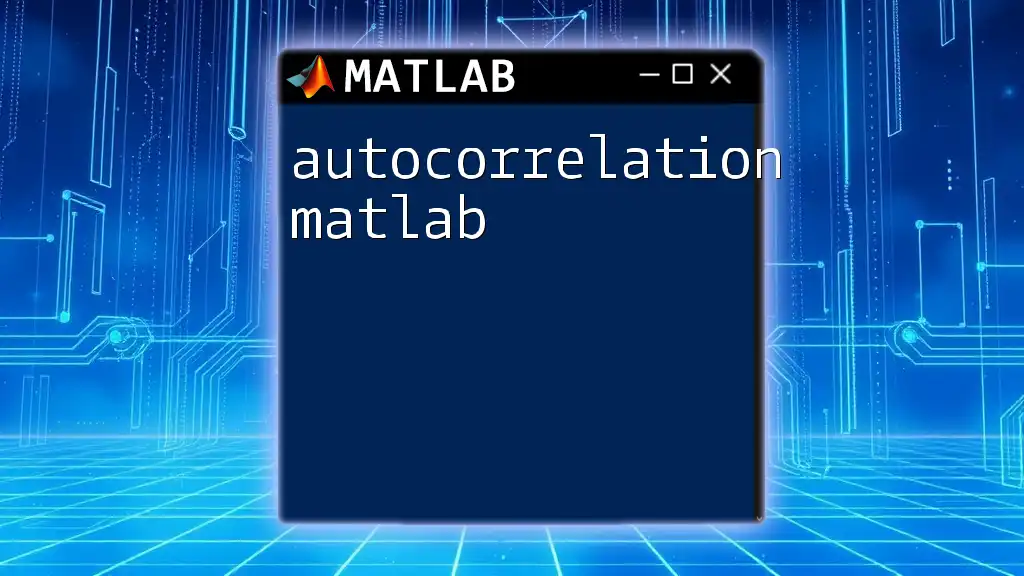
Conclusion
In this guide, we've journeyed through the intricacies of LU Factorization in MATLAB. From understanding the decomposition of matrices into \( L \) and \( U \) to leveraging built-in functions for efficient computations, the advantages of mastering LU Factorization cannot be overstated.
Practical application of these concepts will undoubtedly bolster your confidence and capabilities in handling linear algebra tasks using MATLAB. Engage with various matrices and practice the processes outlined here to solidify your understanding and proficiency in LU Factorization techniques.
The world of numerical computations awaits you, and whether you are solving linear equations or aiming for numeric stability, the skill of LU Factorization will serve you well.