The `intersect` function in MATLAB is used to find common elements between two arrays, returning the intersection of the two sets.
A = [1, 2, 3, 4];
B = [3, 4, 5, 6];
C = intersect(A, B); % C will be [3, 4]
Understanding Intersection: Definition and Applications
What is Intersection?
In mathematical and programming contexts, intersection refers to the common elements shared between two or more sets. In the realm of MATLAB, the intersection operation is vital for various applications, allowing users to identify and analyze shared data points. For instance, when comparing datasets, finding overlapping values or structures is crucial for drawing meaningful insights, especially in data analysis, image processing, and geometry.
Practical Scenarios for Intersection in MATLAB
The intersection function in MATLAB can be applied in numerous scenarios, such as:
- Data Analysis: When working with large datasets, you may want to find common data points across multiple groups, which helps in comparative analysis.
- Image Processing: Overlapping shapes, whether they represent objects in an image or regions of interest in data visualization, can be efficiently handled using intersection methods.
- Geometry: For tasks involving geometric figures, such as lines and curves, determining points of intersection is fundamental for further analysis.
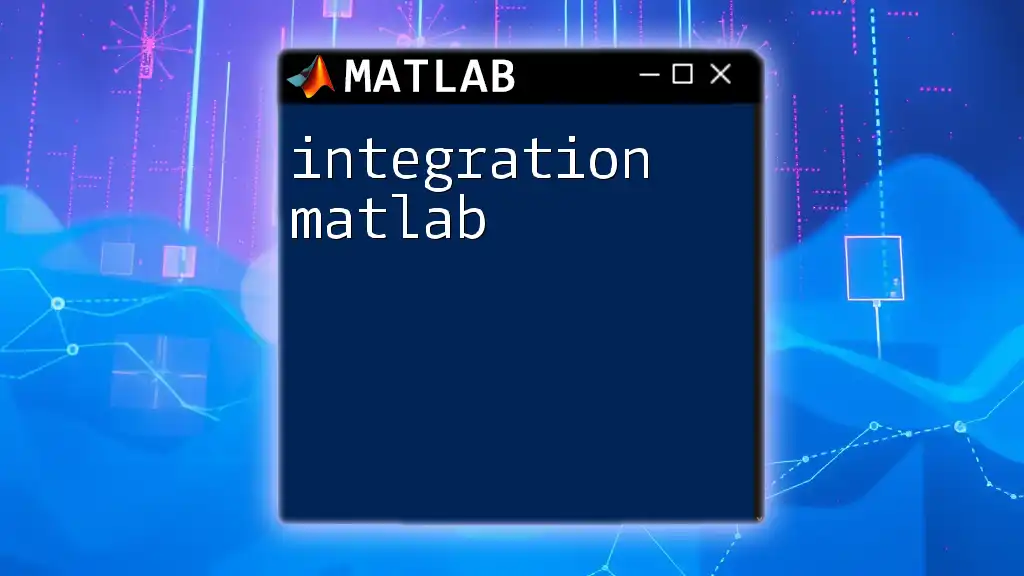
Key Intersection Functions in MATLAB
`intersect` Function
The intersect function is the cornerstone for finding common elements in MATLAB. It efficiently identifies shared elements between two arrays.
Function Syntax:
C = intersect(A, B)
Here, `A` and `B` represent the two arrays you want to compare. The variable `C` will contain the elements common to both arrays.
Basic Example of Using `intersect`
To illustrate the basic usage of the intersect function, consider the following simple example:
A = [1, 2, 3, 4, 5];
B = [4, 5, 6, 7, 8];
C = intersect(A, B);
disp(C);
In this example, MATLAB will display:
4 5
This output signifies that the numbers 4 and 5 are present in both arrays A and B, demonstrating a basic but essential function of intersection.
Additional Options with `intersect`
Finding Indices of Intersection
Beyond simply finding common elements, you might also want to know their original indices within the arrays. You can accomplish this using the following syntax:
[C, IA, IB] = intersect(A, B)
Parameters Breakdown:
- `C` contains the common elements,
- `IA` provides the indices of `A` where the elements of `C` can be found,
- `IB` provides the indices of `B`.
Here's how it plays out in code:
A = [1, 2, 3, 4, 5];
B = [4, 5, 6, 7, 8];
[C, IA, IB] = intersect(A, B);
disp(IA); % Indices of A
disp(IB); % Indices of B
Expect the output:
4 5
1 2
This indicates that the elements of `C` are located at indices 4 and 5 in A and at indices 1 and 2 in B.
Combining `intersect` with Other Functions
Using `intersect` with Sorting
It is sometimes advantageous to ensure that your arrays are sorted, especially when dealing with larger datasets or certain algorithms. For example:
A = sort([10, 20, 30, 40, 50]);
B = sort([30, 40, 50, 60, 70]);
C = intersect(A, B);
In this case, sorting arrays A and B helps maintain order and ensures that the intersection is correctly computed without redundant comparisons.
Handling Multiple Arrays
Intersection in Multidimensional Arrays
If you are working with multidimensional data, MATLAB also allows you to find intersections across the rows of matrices. You can achieve this using:
C = intersect(A, B, 'rows')
For example:
A = [1, 2; 3, 4; 5, 6];
B = [3, 4; 7, 8; 5, 6];
C = intersect(A, B, 'rows');
The result for this operation identifies the common rows and demonstrates how you can work with multidimensional data efficiently.
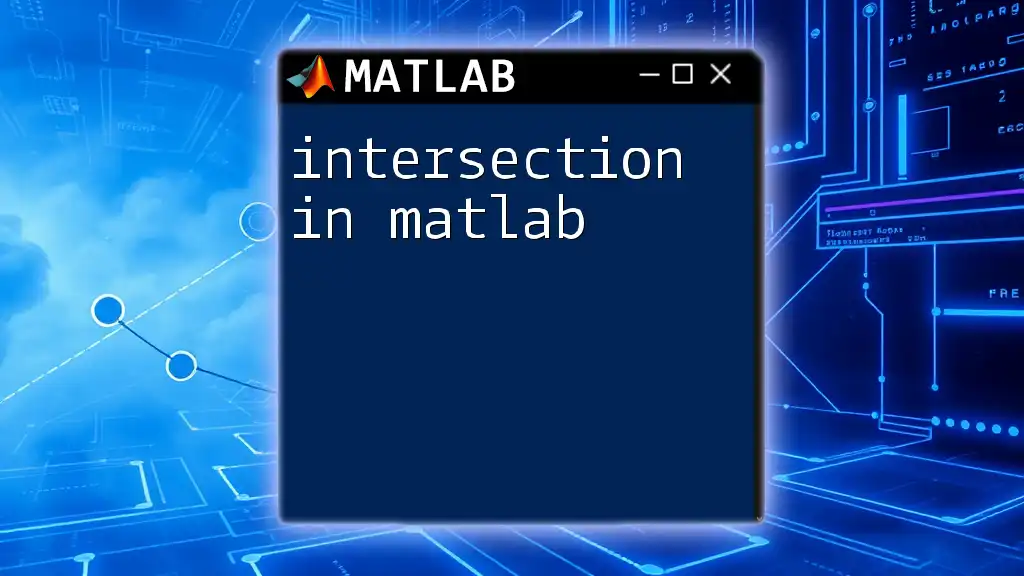
Advanced Intersection Concepts
Intersection in Sets
Understanding set theory can provide deeper insights into how intersections work in MATLAB. In scenarios where effectiveness is essential, leveraging logical indexing can simplify your task of finding intersections while enabling cleaner code.
Geometric Intersections
Determining intersections in geometric contexts, such as finding the point where two lines cross, can be accomplished through algebraic methods within MATLAB. Consider the following example:
Suppose you want to find the point of intersection between the lines given by the equations: y = 2x + 1 and y = -x + 4.
You can solve this using symbolic computation:
syms x y;
eq1 = y == 2*x + 1;
eq2 = y == -x + 4;
sol = solve([eq1, eq2], [x, y]);
disp(sol);
This will provide you the coordinates of the intersection point, which might be used in many geometrical applications.
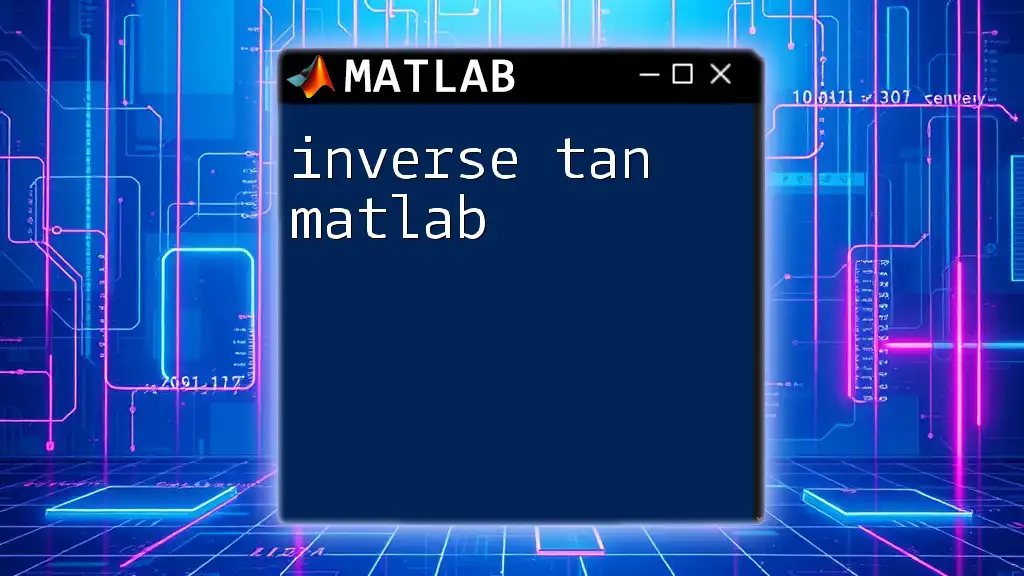
Common Issues and Troubleshooting
General Problems with `intersect`
While utilizing the intersect function, users may come across data type mismatches, particularly when pairs of arrays have different types (e.g., integer arrays vs. string arrays). Always ensure the data types match to avoid errors.
Performance Considerations
In situations involving large datasets, performance becomes a critical factor. Efficient coding practices, such as pre-sorting and minimizing the number of array comparisons, can significantly enhance the speed of intersection calculations.
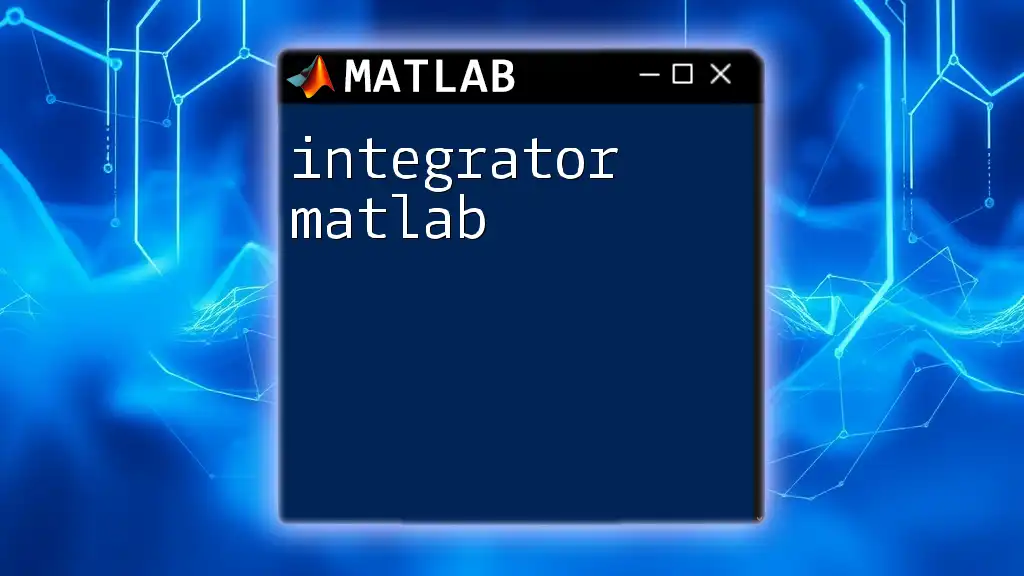
Conclusion
The ability to find intersections using the `intersect` function in MATLAB enables users to explore data effectively, draw connections between datasets, and manage logical operations seamlessly. It serves not just as a simple command but as a powerful tool in various applications from analyzing data trends to processing geometric figures.
Additional Resources
For further exploration, consider diving into the official MATLAB documentation to understand more about the intersect function and its applications. Additional reading from books or formal courses can enhance your skills and provide a more comprehensive learning experience.
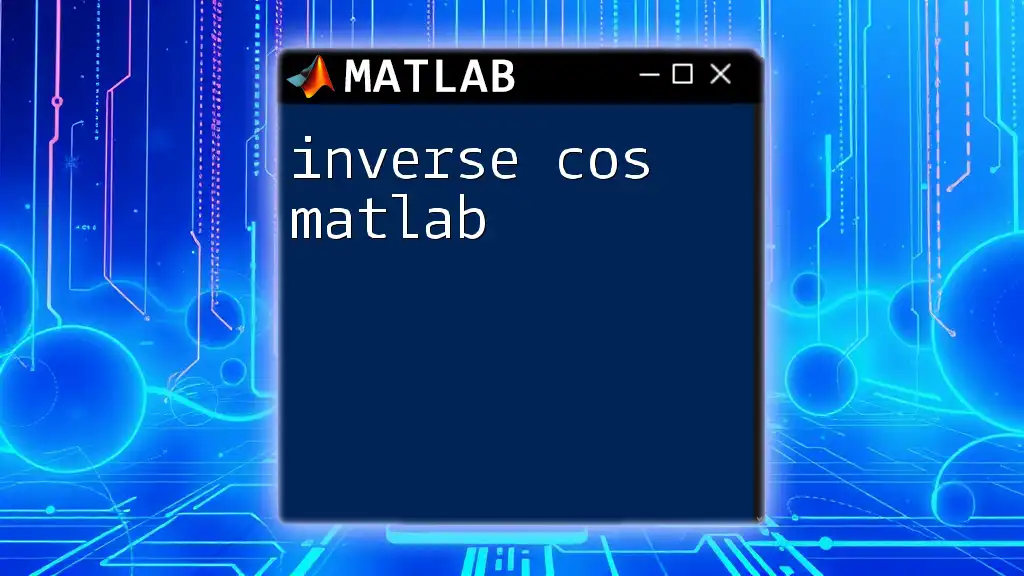
Call to Action
Want to enhance your MATLAB skills? Stay updated by signing up for our courses and following us on social media, where we share tips, tricks, and additional resources to sharpen your programming prowess!