Matrix division in MATLAB can be performed using the backslash `\` operator for solving equations or the forward slash `/` operator for pre-multiplying matrices, enabling efficient computation of solutions based on the context.
% Example of matrix division using backslash operator
x = A \ b; % Solves the equation Ax = b
% Example of matrix division using forward slash operator
y = b / A; % Solves the equation yA = b
Understanding Matrix Division
What is Matrix Division?
Matrix division refers to the process of using matrices to solve equations, akin to dividing numbers. In MATLAB, this division is not just a straightforward mathematical operation; it serves as a powerful tool for solving linear equations, manipulating datasets, and performing advanced numerical analysis. Matrix division is substantial in engineering, physics, data science, and statistics, playing a vital role in computations ranging from simulations to optimizations.
While scalar division involves a direct arithmetic operation, matrix division takes a more nuanced approach, considering the properties and dimensions of the matrices involved, often framing the solution space of linear equations.
Types of Matrix Division
In MATLAB, there are two primary forms of matrix division: left division and right division.
- Left Division (`A \ B`): This operation finds a matrix X such that it solves the equation \(A \cdot X = B\).
- Right Division (`A / B`): This operation finds a matrix X such that it solves the equation \(X \cdot B = A\).
Understanding when and how to use each type is crucial for effective computations.
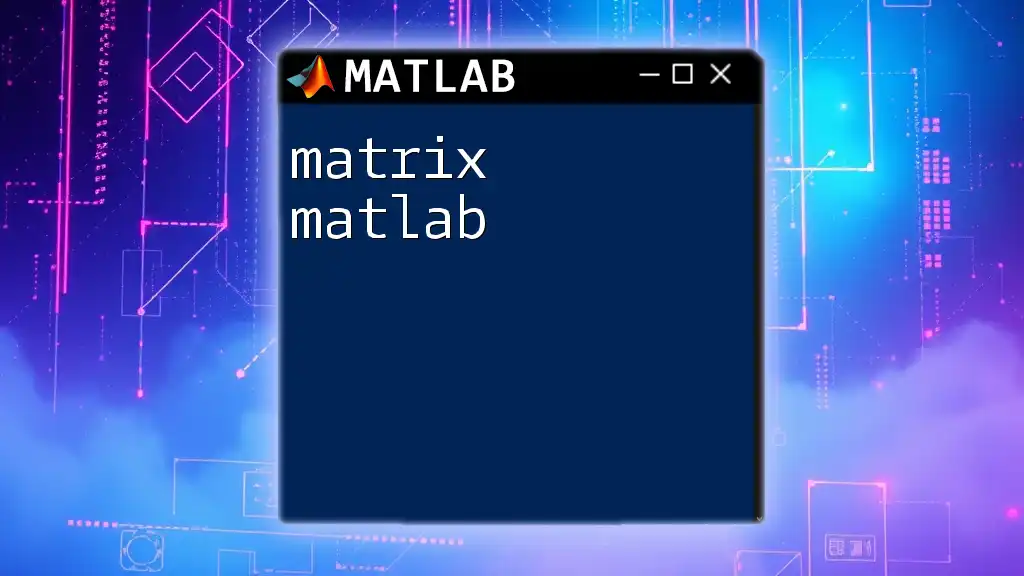
Left Division in MATLAB
Syntax and Basics
The basic syntax for performing left division in MATLAB is as follows:
X = A \ B
When you execute this command, MATLAB computes the matrix \(X\) such that the product of \(A\) and \(X\) equals \(B\). It's an efficient way to solve linear equations without directly calculating the inverse of \(A\), avoiding potential numerical instability in the results.
Use Cases of Left Division
One of the most common applications of left division is solving systems of linear equations. For example, consider the following matrices:
A = [1, 2; 3, 4];
B = [5; 11];
X = A \ B;
In this example, MATLAB finds the matrix \(X\) which satisfies the equation \(A \cdot X = B\). Here, \(X\) will contain the values that solve this system of equations, crucial for understanding variables' relationships in such mathematical contexts.
Code Explanation
In the code example provided, the result of `X` will yield:
X =
1
2
This means that \(X\) equals 1 and 2, respectively, effectively representing the values that satisfy our original matrix equation.
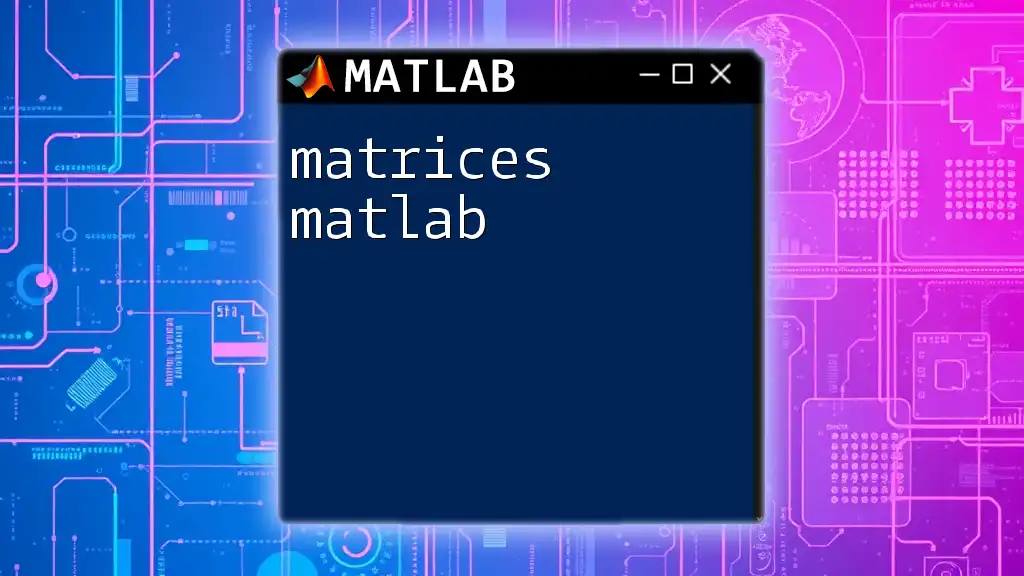
Right Division in MATLAB
Syntax and Basics
The right division syntax is structured similarly but changes the context slightly:
X = A / B
In this operation, MATLAB performs the equivalent of multiplying \(A\) by the inverse of \(B\), solving the equation \(X \cdot B = A\).
Use Cases of Right Division
Right division is commonly used for transformations or manipulations of matrices. Take a look at the following example:
A = [1, 2; 3, 4];
B = [5; 11];
X = B' / A;
In this instance, the transpose operator (`'`) is utilized to reshape \(B\), and with right division, we are essentially transforming it as per the matrix \(A\).
Code Explanation
After executing the code, `X` will result in a transformed solution based on the right division operation. This operation allows for quick adjustments to matrix operations without needing direct inverse computations, which can enhance performance in calculations involving larger matrices.
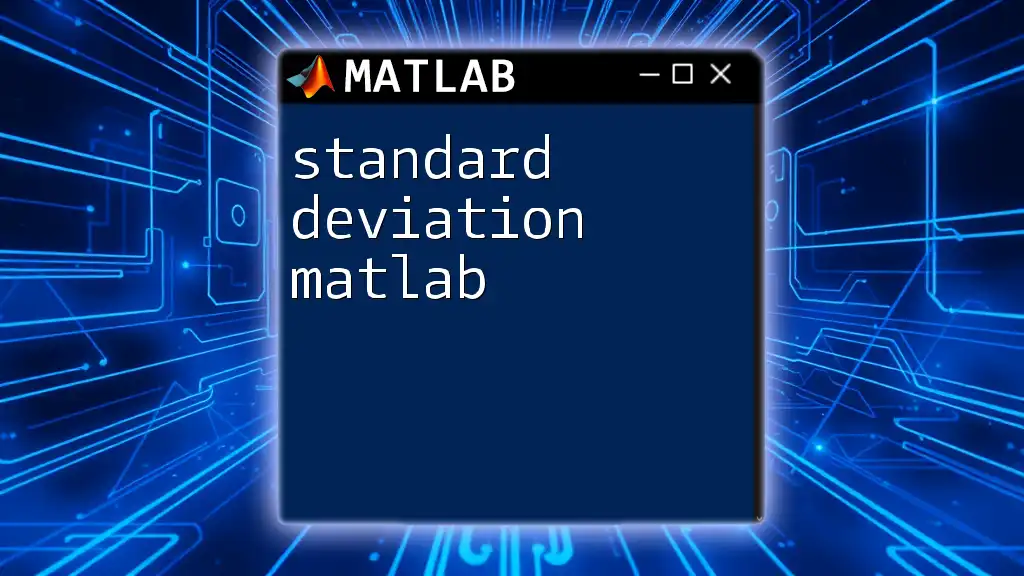
Handling Errors in Matrix Division
Common Errors
When working with matrix division, you may encounter several common errors:
- Rank Deficiency: This occurs when a matrix does not have full rank, causing complications in determining unique solutions. A singular matrix indicates that the corresponding system of equations may either have no solutions or infinitely many solutions.
- Dimension Mismatch: Every matrix must be of compatible dimensions for division to be valid, meaning \(A\) must have the same number of rows as \(B\) has columns, or vice versa.
Tips to Avoid Errors
To minimize these errors, ensure that your matrices are compatible by checking their dimensions before attempting division. Furthermore, you can confirm the rank of a matrix using the following command:
rank(A);
By pre-checking, you can ensure the matrices behave as expected during division operations.
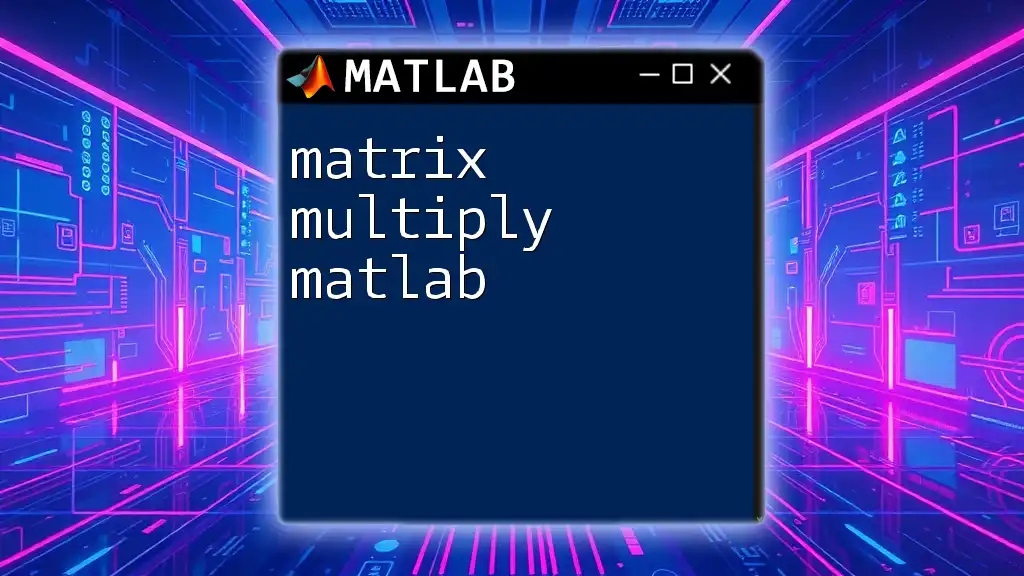
Optimization Techniques
Performance Considerations
When performing matrix operations, efficiency is critical. MATLAB's matrix division operations are designed for efficiency, so it is recommended to leverage these built-in capabilities rather than resorting to manually finding inverses.
Best Practices for Matrix Division
To maximize performance during matrix division:
- Avoid using the `inv()` function: Using matrix inversion for division can lead to unnecessary computational expense and numerical instability. Instead, always prefer left and right division methods for solving matrix equations.
- Utilize built-in functions effectively: MATLAB's internal optimizations make direct use of functions like `\` and `/`, resulting in faster computations and improved accuracy.
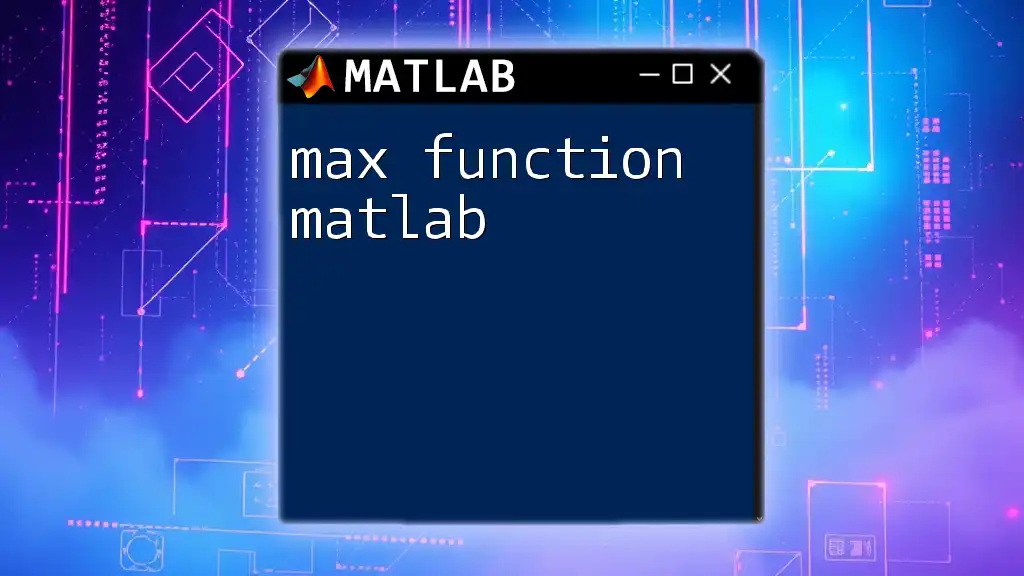
Conclusion
Matrix division in MATLAB is a cornerstone concept that enables you to solve linear systems and performs numerous calculations effectively. By understanding the nuances of left and right division, as well as avoiding common pitfalls, you can harness the full power of matrix operations in your projects. Practice with diverse examples to solidify your comprehension, and explore additional resources to enhance your MATLAB skills further.
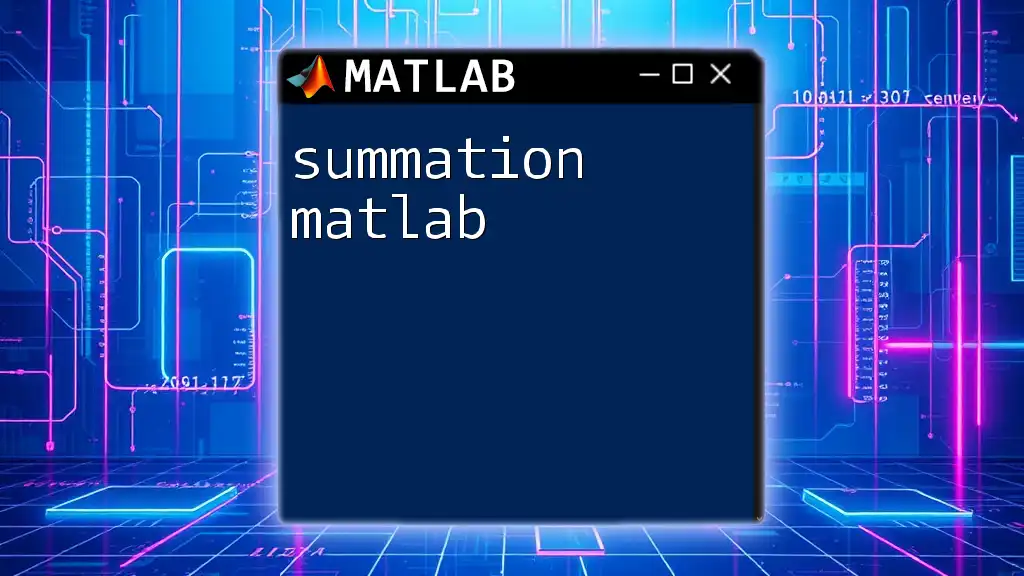
Additional Resources
To deepen your knowledge on matrix division further, consider exploring the official MATLAB documentation, sign up for online courses dedicated to MATLAB, or join community forums where MATLAB enthusiasts collaborate and share insights.
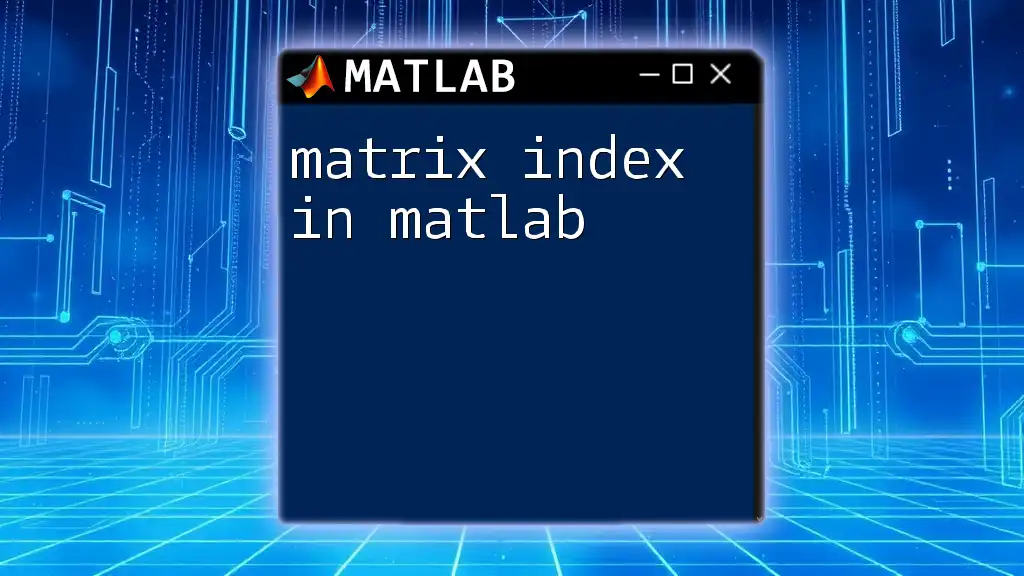
Frequently Asked Questions (FAQs)
What is the difference between left and right division?
The key difference between left and right division is in their operational context. Left division (`A \ B`) solves for \(X\) such that it can be seen as \(A \cdot X = B\), while right division (`A / B`) operates where \(X \cdot B = A\). It’s essential to choose the correct operation based on the specific equation you are solving.
When should I use matrix division?
Matrix division should be your go-to method whenever you are dealing with system equations in a matrix form. It becomes especially useful when you're working with larger datasets or need to solve multiple equations efficiently.
Is it okay to use matrix inversion instead of division?
Using matrix inversion is generally discouraged due to the potential for loss of numerical precision and increased computational workload. Left and right division often yield better performance and stability.