In MATLAB, you can compute the summation of an array or matrix elements using the `sum` function, which efficiently adds up all the elements along a specified dimension. Here's a code snippet demonstrating its use:
% Calculate the sum of array elements
A = [1, 2, 3, 4, 5];
total = sum(A); % total will be 15
Understanding Summation
Summation is a fundamental mathematical operation used to calculate the total or sum of a series of numbers. In the realm of programming, particularly with summation in MATLAB, understanding how to effectively compute sums can significantly enhance data analysis capabilities. MATLAB provides a versatile environment for mathematical computations, making it an ideal choice for anyone looking to learn efficient summation techniques.
Basic Summation Commands in MATLAB
Using the `sum` Function
The most straightforward way to perform summation in MATLAB is by utilizing the built-in `sum` function. The syntax is simple:
totalSum = sum(array);
This command sums all elements in the specified array. Let's look at a basic example:
% Example: Simple summation of a vector
A = [1, 2, 3, 4];
totalSum = sum(A);
disp(totalSum); % Output: 10
In this case, MATLAB sums the elements of vector A, resulting in a total of 10.
Summing Different Dimensions
MATLAB allows you to sum along specific dimensions of matrices, which is particularly useful in multi-dimensional data analysis. By specifying the dimension as an additional argument, you can control whether you want to sum across rows or columns.
% Example: Summing along different dimensions
B = [1, 2; 3, 4];
rowSum = sum(B, 1); % Sum across rows
columnSum = sum(B, 2); % Sum across columns
disp(rowSum); % Output: 4 6
disp(columnSum); % Output: 3; 7
Here, `sum(B, 1)` results in the total for each column, while `sum(B, 2)` provides the total for each row.
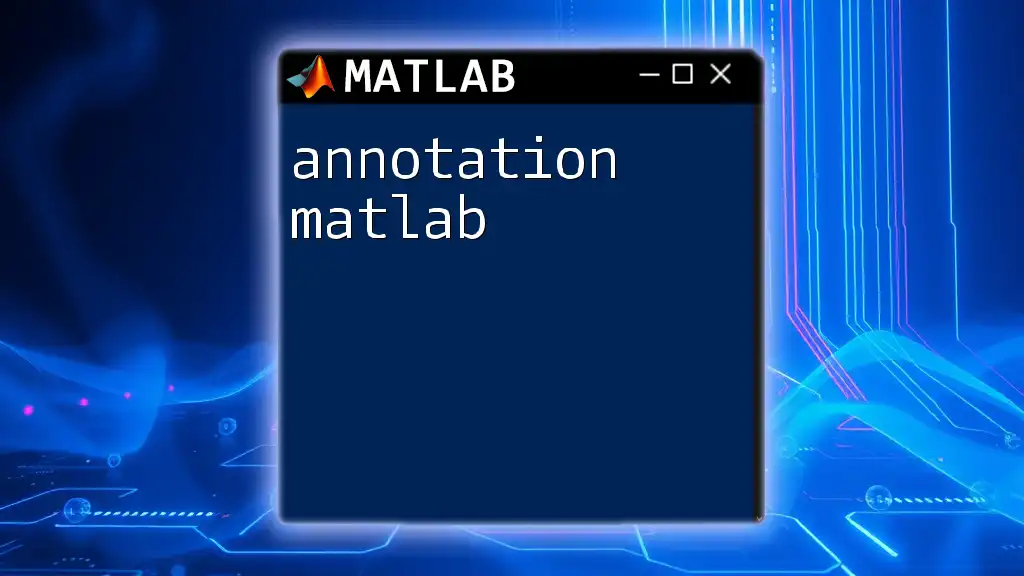
Advanced Summation Techniques
Cumulative Summation
Another powerful function in MATLAB is `cumsum`, which performs cumulative summation. This function generates an array where each element is the sum of the elements up to that index.
% Example: Cumulative sum of elements
C = [1, 2, 3, 4];
cumulativeSum = cumsum(C);
disp(cumulativeSum); % Output: 1 3 6 10
In this example, each position in the output array represents the total sum of the elements leading up to that index, providing insights into the accumulation of values.
Weighted Summation
In many applications, you may want to compute a weighted sum, where each element's contribution to the total sum is multiplied by a specific weight. This scenario arises in statistics and data analysis frequently.
% Example: Computing a weighted sum
weights = [0.1, 0.2, 0.3, 0.4];
D = [10, 20, 30, 40];
weightedSum = sum(weights .* D);
disp(weightedSum); % Output: 30.0
In the above code, each value in array D is multiplied by the corresponding weight, and then the sums of these products are computed, resulting in a 30.0 weighted total.
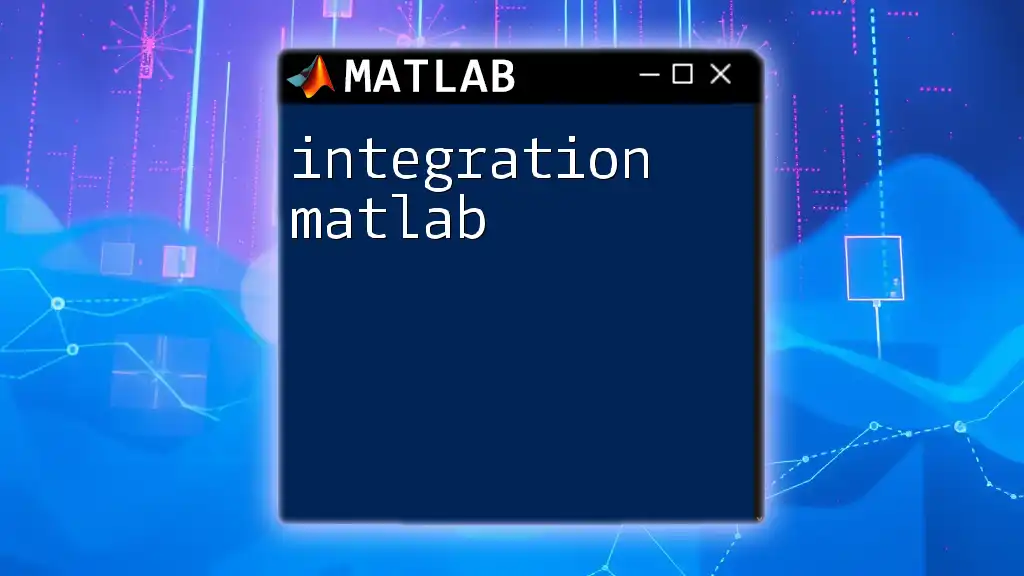
Working with Summation in Data Structures
Summation in Tables and Timetables
MATLAB also facilitates summation in data structures like tables and timetables. You can leverage the `sum` function to perform operations on data stored in these structures seamlessly.
% Example: Summation in a table
T = table([1; 2; 3], [4; 5; 6], 'VariableNames', {'A', 'B'});
totalA = sum(T.A);
disp(totalA); % Output: 6
This example illustrates how to sum values in a specific column of a table named T, showcasing the flexibility of data types in MATLAB.
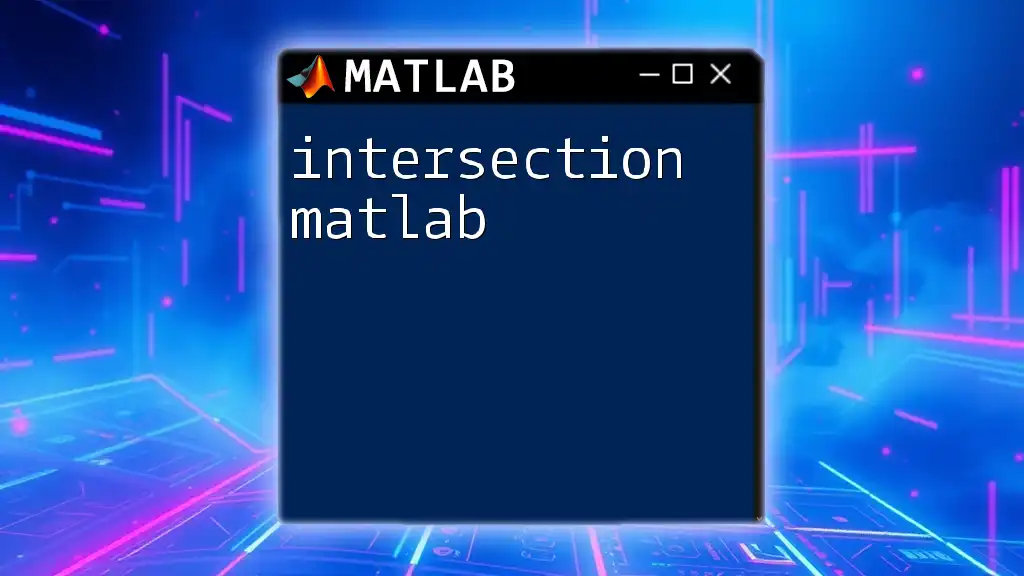
Custom Summation Functions
Sometimes, you might require a custom summation operation tailored to specific business logic or unique datasets. You can easily create a custom function for this purpose.
function total = customSum(vector)
total = 0;
for i = 1:length(vector)
total = total + vector(i);
end
end
This user-defined function iterates through each element of the input vector and calculates the total sum manually, providing a clear understanding of the summation process and allowing for customization.
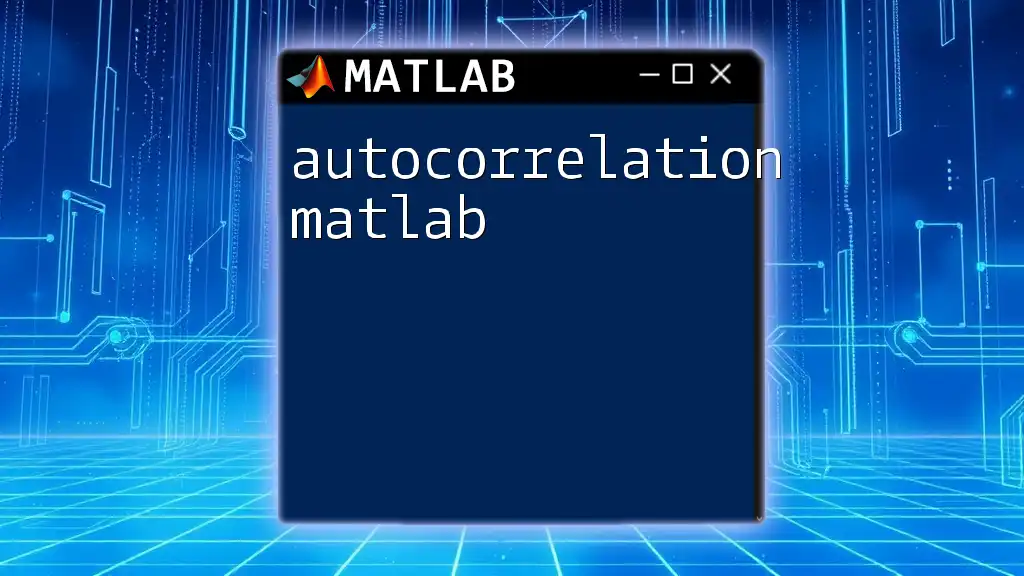
Error Handling in Summation
While performing summation operations, some common errors may arise, particularly regarding dimension mismatches in arrays. For example, trying to sum non-conforming dimensions can lead to unexpected results or runtime errors. It’s essential to ensure the arrays you are summing are properly aligned in their dimensions.
When debugging summation code, using the `size` and `length` functions to check the dimensions of the arrays can save time:
% Check dimensions
disp(size(A));
disp(length(A));
This way, you can confirm that the arrays comply with your summation logic before execution.
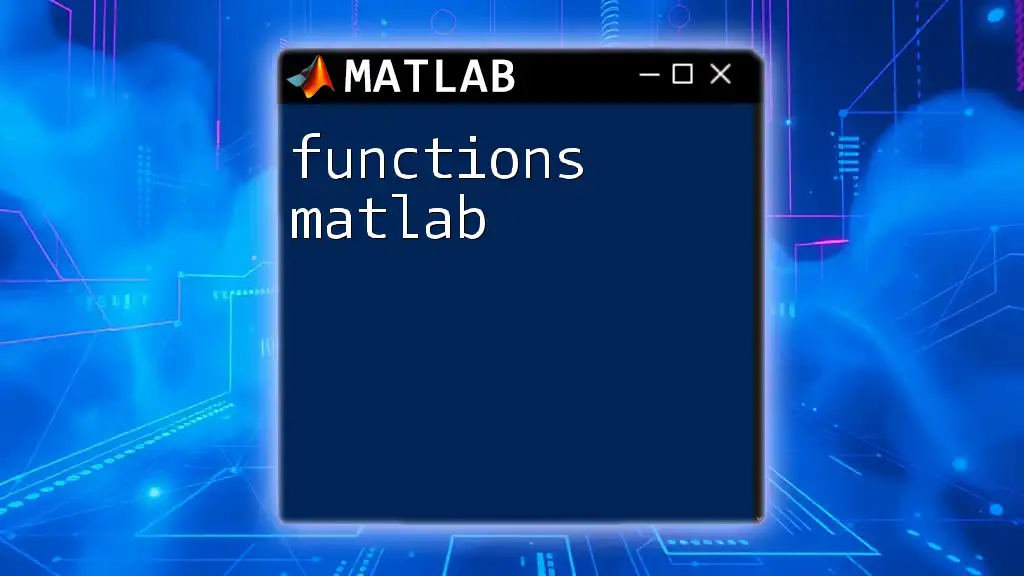
Practical Applications of Summation in MATLAB
Real-Life Use Cases of Summation
Understanding summation in MATLAB has vast real-world applications. In statistics, summation is integral for calculating means and variances. In engineering, it can be vital for analyzing signal processing data, while in finance, it implements calculations for sums of payments and expenses over time.
Case Study: Summation in Data Analysis
Let's consider a case study of analyzing sales data. By summing values in a dataset, businesses can gauge total sales over various time periods and make informed decisions regarding inventory management or marketing strategies.
Summation serves as a foundational skill for any data analyst or engineer, allowing one to extract significant insights and make data-driven decisions effectively.
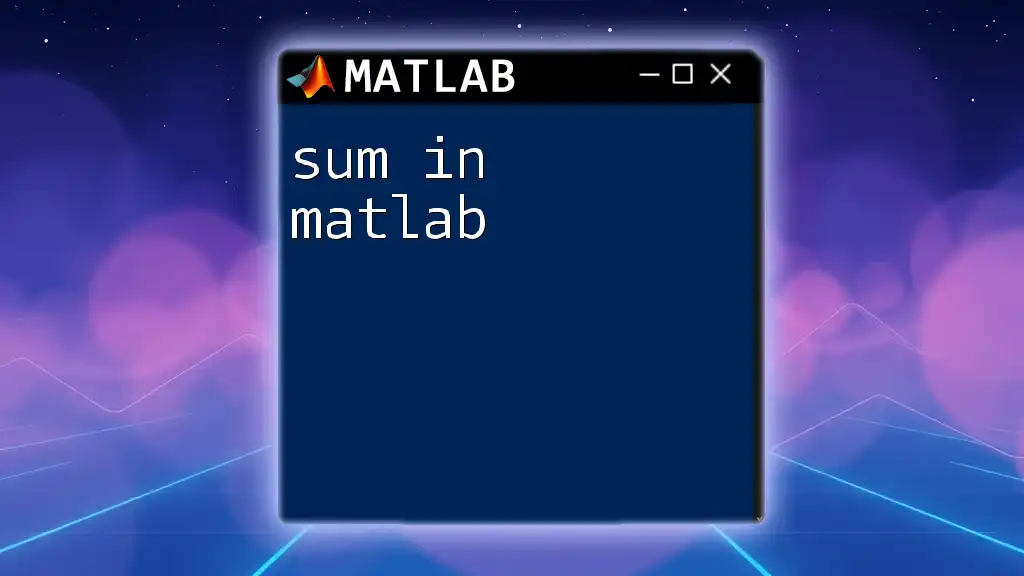
Conclusion
In conclusion, mastering summation in MATLAB unlocks a plethora of possibilities in data analysis and mathematical computation. From basic operations using the built-in `sum` function to advanced techniques like cumulative and weighted summation, the tools at your disposal can facilitate extensive and nuanced data insights. Practice these commands and explore their diverse applications to enhance your MATLAB proficiency.