In MATLAB, you can compute the derivative of a function symbolically using the `diff` function, as shown in the following example:
syms x; % Define the symbolic variable
f = x^2 + 3*x + 2; % Define the function
derivative_f = diff(f, x); % Compute the derivative
disp(derivative_f); % Display the result
What is a Derivative?
In mathematics, a derivative represents the rate at which a function is changing at any given point. More formally, the derivative of a function at a particular point provides the slope of the tangent line to the function at that point. Understanding derivatives is crucial in various fields, including physics, engineering, and data analysis, as they offer insights into behavior and trends in relationships between variables.
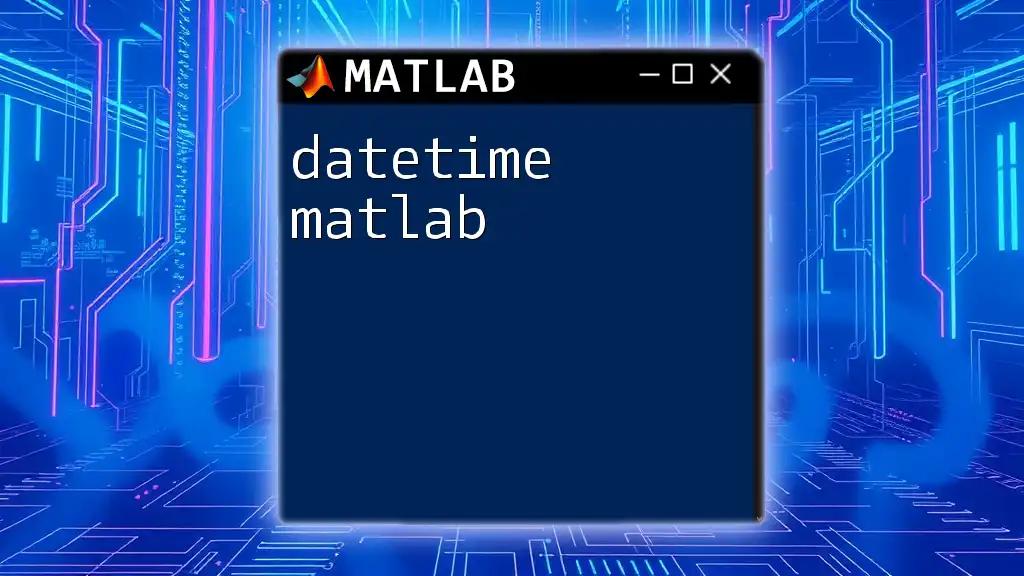
Why Use MATLAB for Derivatives?
MATLAB stands out as a powerful tool for calculating derivatives due to its extensive capabilities in numerical analysis and symbolic computation. With built-in functions specifically designed for these purposes, MATLAB enables users to perform derivative calculations quickly and efficiently, whether they require exact results or numerical approximations.

Understanding Derivative Concepts
Types of Derivatives
First Derivative
The first derivative of a function provides information about the rate of change of that function—the slope at a particular point. For example, for a distance function over time, the first derivative indicates velocity.
Higher-Order Derivatives
While the first derivative shows the rate of change, the second derivative illustrates how the rate of change itself is altering. Higher-order derivatives can be valuable in analyzing motion, curvature, and optimization.
Numerical vs. Symbolic Derivatives
Numerical Derivative
Numerical differentiation estimates the derivative at specific points based on the function's values nearby. This approach is useful when dealing with complex functions where an analytical solution is difficult to derive.
Symbolic Derivative
The symbolic derivative computes the derivative in an exact manner, allowing for the derivation of formulas that can be reused or simplified. This method is generally more powerful as it provides precise results as opposed to numerical approximations.
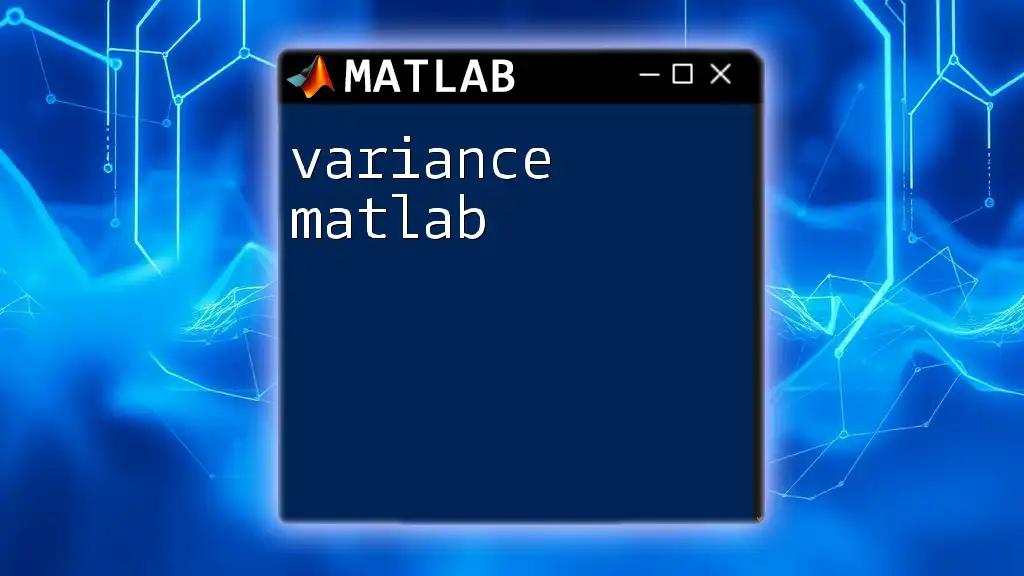
Calculating Derivatives Using MATLAB
Built-in Functions for Derivatives
Using `diff` for Symbolic Derivation
MATLAB provides the `diff` function for symbolic differentiation, which allows users to differentiate functions precisely. Below is an example:
syms x;
f = x^2 + 3*x + 5;
df = diff(f, x);
disp(df);
This code computes the symbolic derivative of the function \( f(x) = x^2 + 3x + 5 \) with respect to \( x \). The result, displayed in the command window, illustrates how the slope of the function changes.
Using `gradient` for Numerical Derivation
The `gradient` function is used for calculating numerical derivatives. A basic example is as follows:
x = 0:0.1:10;
y = sin(x);
dy = gradient(y, 0.1);
plot(x, dy);
title('Numerical Derivative of sin(x)');
xlabel('x');
ylabel('dy/dx');
In this example, we compute the numerical derivative of the sine function and visualize it. The plot illustrates how the derivative yields the function’s slope at various points, an essential concept in calculus.
Custom Functions for Derivative Calculation
Creating a custom numerical derivative function allows for tailored applications based on specific needs. Here's a straightforward function to perform numerical differentiation:
function df = numerical_derivative(f, x, h)
df = (f(x+h) - f(x-h)) / (2*h);
end
In this function, `f` is the function to be differentiated, `x` represents the point of interest, and `h` is the step size. You can use this function in various contexts, providing increased flexibility in differentiating more complex functions that may not have a straightforward derivative.
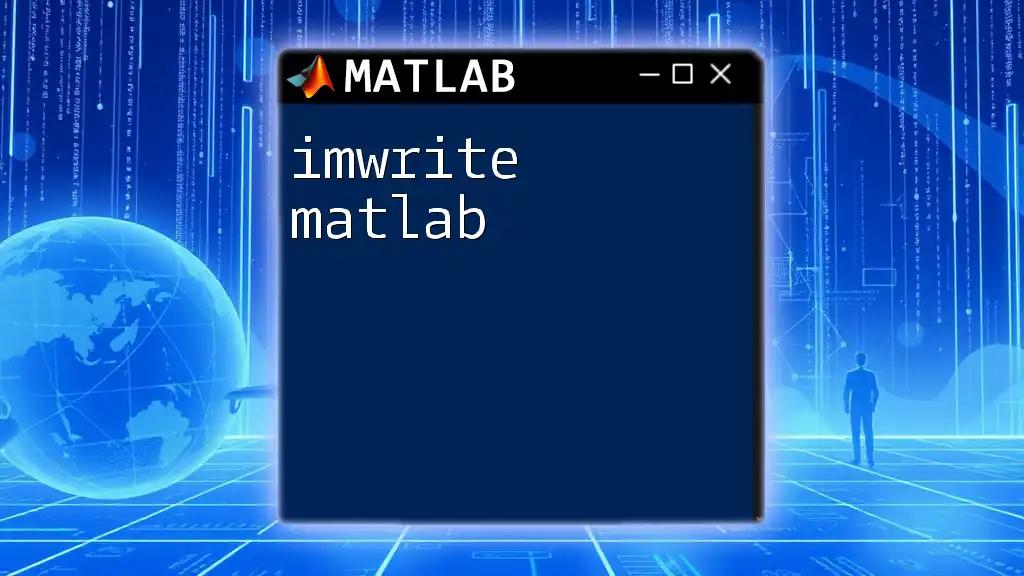
Applications of Derivatives in MATLAB
Optimization Problems
Derivatives play a crucial role in optimization problems, especially when identifying local maxima and minima. By finding points where the derivative equals zero (critical points), we can determine the nature of the function. For instance:
syms x;
f = -1 * (x^2 - 4 * x + 4); % Function to maximize
df = diff(f, x);
criticalPoints = solve(df == 0, x);
disp(criticalPoints);
This code finds critical points of the function \( f(x) = -1(x^2 - 4x + 4) \), identifying where the function reaches its maximum. Understanding these critical points is vital in fields like economics and engineering for optimizing outputs.
Solving Differential Equations
Derivatives serve as fundamental components of ordinary differential equations (ODEs). MATLAB's `ode45` solver efficiently handles these equations, as shown below:
f = @(t, y) -2*y + 1; % ODE definition
[t, y] = ode45(f, [0 5], 0); % Initial condition y(0) = 0
plot(t, y);
title('Solution of ODE dy/dt = -2y + 1');
xlabel('Time t');
ylabel('y(t)');
Here, we define a simple linear ODE and solve it under a given initial condition. The resultant plot visualizes how the solution evolves over time, exemplifying the relationship between the function and its derivatives.
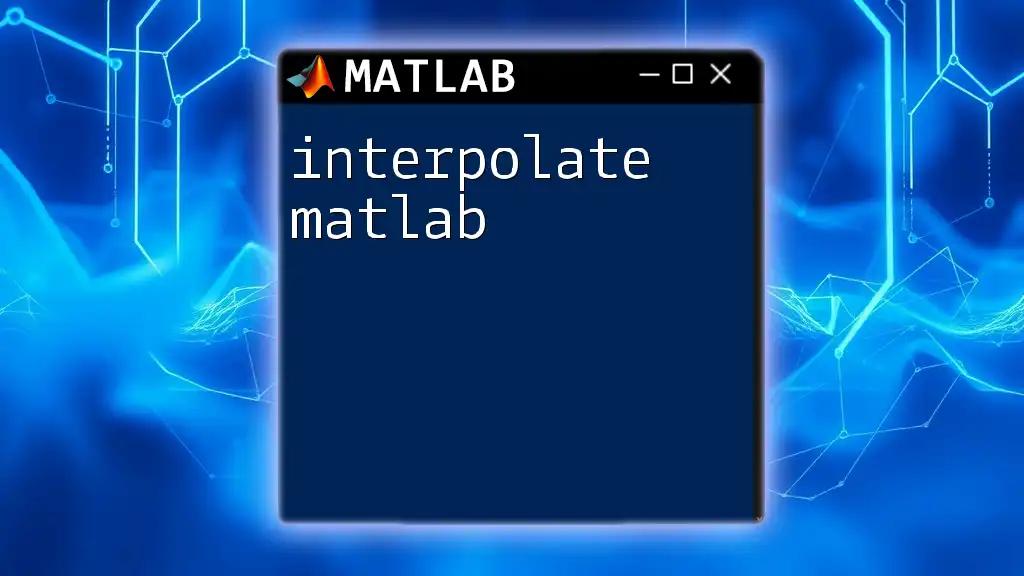
Visualizing Derivatives
Graphical Representation
Visualizing both the function and its derivative provides insights into their relationships. The following example plots a function alongside its derivative:
x = linspace(-2, 2, 100);
f = x.^3 - 3*x.^2 + 2;
df = gradient(f, x);
plot(x, f, 'b', x, df, 'r');
legend('Function', 'Derivative');
title('Function and Its Derivative');
In this visualization, the blue curve represents the original function, while the red curve denotes its derivative. Observing this interaction helps clarify how the changes in the function manifest as fluctuations in the derivative, bringing deeper understanding to calculus principles.
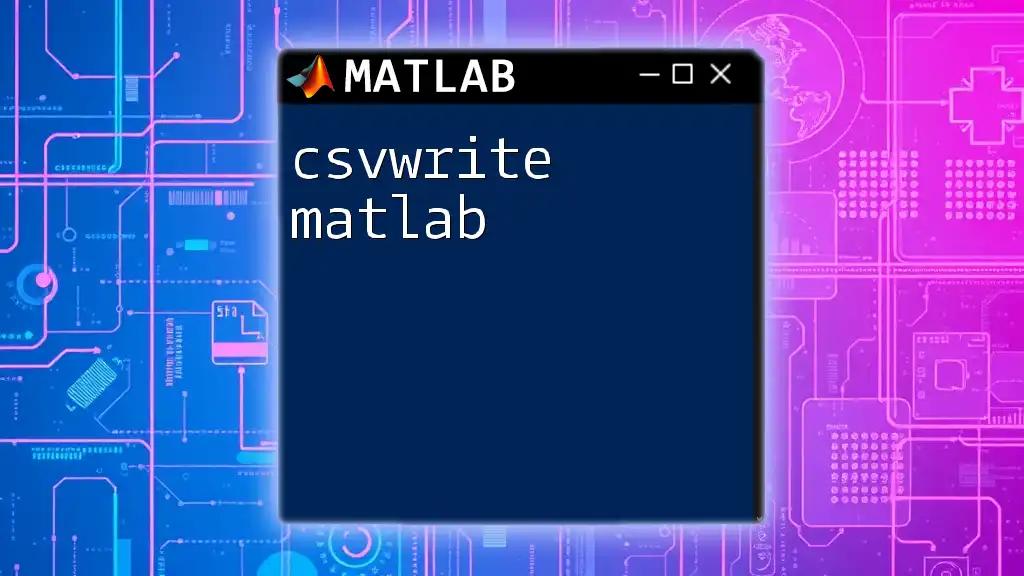
Conclusion
Through this comprehensive guide, we have explored the concept of derivatives within MATLAB, emphasizing their importance, calculation techniques, and applications. With powerful built-in functions, custom functions, and seamless integration into various problems, MATLAB serves as an excellent platform for derivative computations.
For those looking to further enhance their understanding of derivative MATLAB, practicing coding, solving real-world problems, and experimenting with derivatives in different contexts will solidify these concepts. The world of derivatives is vast, and with MATLAB as a tool, the possibilities are endless.
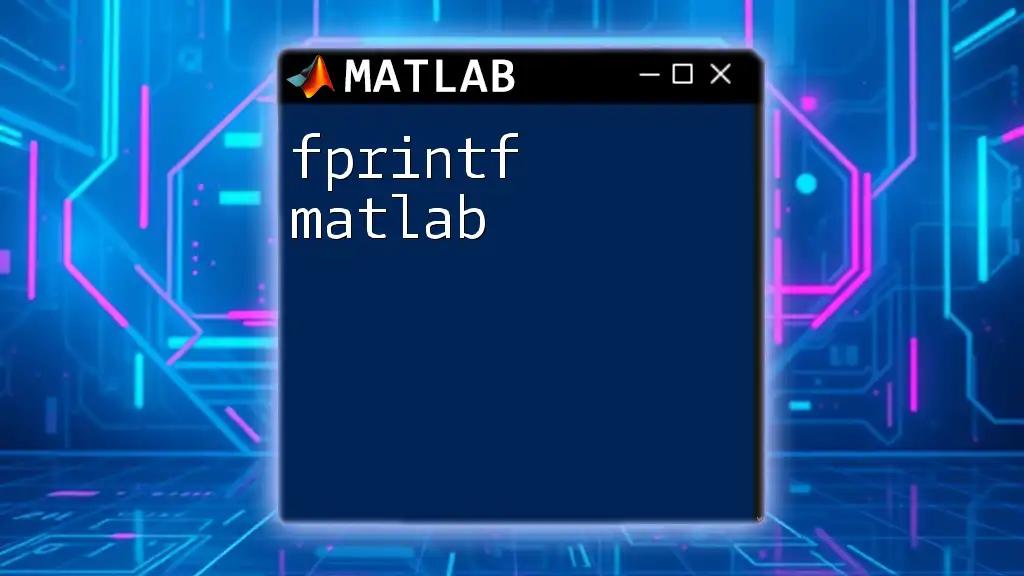
FAQs
Common queries about derivatives and their applications in MATLAB can help clarify concepts. Topics like numerical vs. symbolic derivatives, methods for visualizing derivative behavior, and specific use cases of derivatives across different domains are frequently discussed, providing a wealth of information for learners.