In MATLAB, a function is a reusable block of code designed to perform a specific task, enabling modular programming and improving code organization.
function result = addNumbers(a, b)
result = a + b;
end
Understanding Functions in MATLAB
What is a Function?
In MATLAB, a function serves as a fundamental building block for organizing code efficiently. It enables you to encapsulate a specific task into a reusable module, making your programming process smoother and enhancing code clarity. Unlike scripts that execute a series of commands in a single workspace, functions allow you to define inputs and outputs, creating a controlled environment for computations.
Anatomy of a Function
To understand how to create functions in MATLAB, start with the basic syntax. A function is defined using the `function` keyword, followed by the output variable, the function name, and input arguments. Here’s a general structure for defining a function:
function [output] = functionName(input)
% Function code here
end
This structure lays the groundwork for encapsulating operations, which can range from simple calculations to complex algorithms.
Function Inputs and Outputs
Input Arguments
Input arguments allow you to pass values into your function. They are crucial because they dictate the data that the function will process. Here's a simple example of a function that calculates the area of a rectangle:
function area = rectangleArea(length, width)
area = length * width;
end
In this case, `length` and `width` are the inputs that the function `rectangleArea` uses to compute the area, demonstrating how one can input different values and receive specific outputs.
Output Arguments
Functions can return one or more values via output arguments. This is particularly useful when you want a function to provide multiple results. Check out this example of a function that computes both the perimeter and area of a rectangle:
function [perimeter, area] = rectangleProperties(length, width)
perimeter = 2 * (length + width);
area = length * width;
end
Here, the function computes and returns both perimeter and area, showcasing how robust MATLAB functions can be.
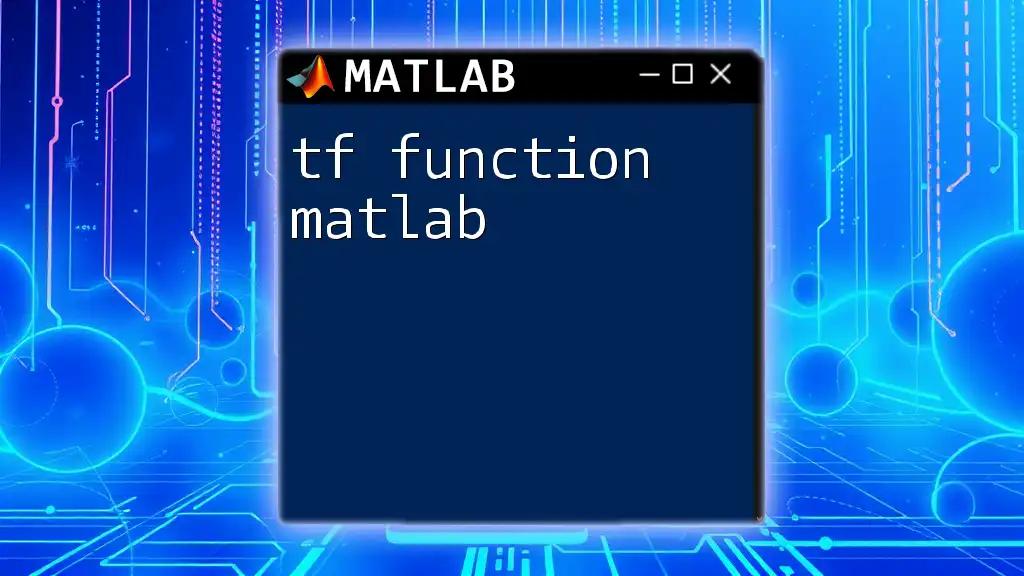
Creating Your First Function
Step-by-Step Guide
Creating a function in MATLAB is straightforward. Here’s a step-by-step approach:
Step 1: Open MATLAB and create a new script file in the editor.
Step 2: Write your function code using the correct syntax.
Step 3: Save the function with the same name as the function itself (e.g., `addNumbers.m`).
Step 4: Open the command window to call your new function and see it in action.
Practical Example: Function to Calculate the Sum of Two Numbers
Consider this simple function to add two numbers:
function total = addNumbers(a, b)
total = a + b;
end
This function takes two inputs, `a` and `b`, and returns their sum as `total`. Each step is essential for demonstrating how to define and call functions efficiently.
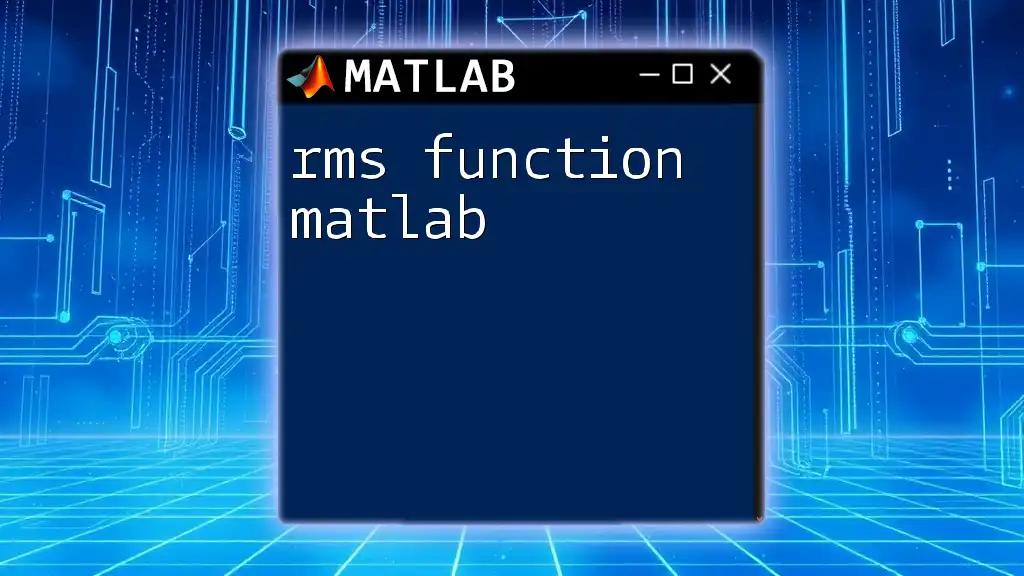
Advanced Function Features
Default Input Arguments
Sometimes, you may want to give input arguments default values. MATLAB offers flexibility in handling fewer inputs. Here’s how to set up defaults:
function result = calculate(a, b)
if nargin < 2
b = 10; % Default value if b is not provided
end
result = a + b;
end
In this function, if only `a` is provided, `b` automatically assumes a default value of `10`, showcasing the convenience this feature can offer.
Varargin and Varargout
Varargin
If you want to allow a function to accept a variable number of input arguments, you can use `varargin`. Here’s an example that sums an arbitrary number of values:
function total = sumAll(varargin)
total = sum([varargin{:}]);
end
In this case, `varargin` collects all input arguments, allowing the function to process any number of values.
Varargout
Similarly, `varargout` enables functions to return variable numbers of output arguments. This feature enhances flexibility in your functions. Here’s an example:
function [sum, avg] = operations(data)
sum = sum(data);
avg = mean(data);
end
With `varargout`, users can request one or both outputs depending on their need.

Best Practices for Writing MATLAB Functions
Code Readability
Writing clear and readable code is crucial in programming. Use descriptive naming conventions for your functions and parameters, which convey the purpose of the function. Additionally, engaging comments can guide users through your code.
Employ the MATLAB "%%" to separate sections within long functions, improving organization and readability.
Efficiency Considerations
Efficiency is key in programming. Avoid using global variables, as they can lead to side effects that are challenging to debug. Instead, ensure that functions operate within their defined scope. Also, aim for vectorization rather than loops to enhance performance. MATLAB is optimized for operating on arrays, and vectorized operations typically outperform their loop-based counterparts.
Testing Your Functions
Testing your functions is essential to ensure they perform as intended. Using MATLAB's built-in functions can simplify this process. Here’s a quick example of using `assert` to validate your function:
assert(addNumbers(5, 3) == 8) % This will pass if addNumbers correctly adds 5 and 3
Assertions help confirm the correctness of your functions, providing peace of mind.
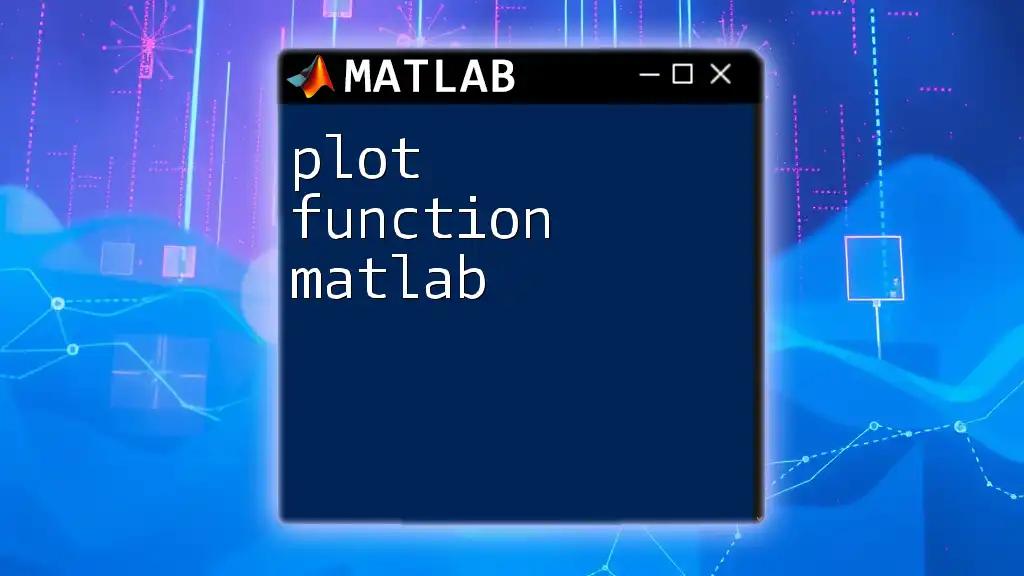
Common Pitfalls in Function Creation
Forgetting to Save the File
One common mistake is forgetting to save your function before executing it. Unsaved changes mean you might run an outdated version, leading to unexpected results.
Misnaming the Function File
Ensure that the name of the `.m` file matches the function name precisely. If inconsistencies arise, MATLAB won't recognize the function, resulting in errors.
Failing to Understand Scope
Another pitfall is misunderstanding the difference in variable scope between the function and base workspace. Variables defined in a function only exist within that function’s scope unless explicitly declared as global. This can lead to confusion and unintended bugs.
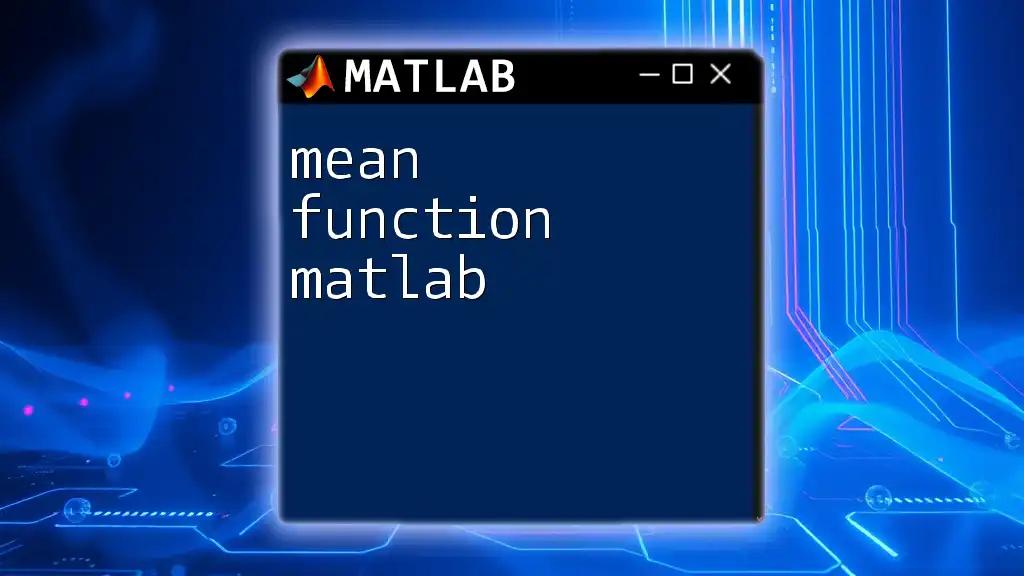
Conclusion
Mastering functions in MATLAB is instrumental in becoming an efficient programmer and data analyst. This comprehensive guide has armed you with the knowledge to create, test, and optimize your functions effectively. Remember to experiment and build your MATLAB functions to deepen your understanding!

Additional Resources
For further reading on MATLAB functions and honing your skills, consider exploring resources like the official MATLAB documentation, online courses, and community forums where you can learn and exchange ideas.

FAQs on MATLAB Functions
What are anonymous functions in MATLAB?
Anonymous functions offer a compact way to create simple functions without having to create a separate file. Here’s an example:
square = @(x) x^2;
Anonymous functions are particularly useful for one-off calculations or when paired with functions like `arrayfun`.
How do I debug a function in MATLAB?
Debugging is crucial for maintaining code quality. MATLAB provides robust debugging tools allowing you to set breakpoints, step through code, and inspect variables. Utilize these tools to catch errors early in your function development process.
By covering these essential aspects of functions in MATLAB, you will be well on your way to developing efficient and effective code, ultimately elevating your programming skills to new heights.