The Jacobian in MATLAB is used to compute the matrix of partial derivatives of a vector-valued function, which can be essential for optimization and sensitivity analysis.
Here's a simple example of how to calculate the Jacobian of a function using MATLAB:
syms x y;
f = [x^2 + y; x + y^2];
J = jacobian(f, [x, y]);
disp(J);
Understanding the Jacobian Matrix
Definition of the Jacobian Matrix
The Jacobian matrix is a fundamental concept in multivariable calculus defined as a matrix of first-order partial derivatives of a vector-valued function. When dealing with a function that maps \(\mathbb{R}^n\) to \(\mathbb{R}^m\), the Jacobian provides insights into the local behavior of the function around a given point.
For a function \(\mathbf{f}:\mathbb{R}^n \rightarrow \mathbb{R}^m\) represented as:
\[ \mathbf{f} = \begin{bmatrix} f_1(x_1, x_2, \ldots, x_n) \\ f_2(x_1, x_2, \ldots, x_n) \\ \vdots \\ f_m(x_1, x_2, \ldots, x_n) \end{bmatrix} \]
the Jacobian matrix \(J\) is given by:
\[ J = \begin{bmatrix} \frac{\partial f_1}{\partial x_1} & \frac{\partial f_1}{\partial x_2} & \ldots & \frac{\partial f_1}{\partial x_n} \\ \frac{\partial f_2}{\partial x_1} & \frac{\partial f_2}{\partial x_2} & \ldots & \frac{\partial f_2}{\partial x_n} \\ \vdots & \vdots & \vdots & \vdots \\ \frac{\partial f_m}{\partial x_1} & \frac{\partial f_m}{\partial x_2} & \ldots & \frac{\partial f_m}{\partial x_n} \end{bmatrix} \]
Importance in Multivariable Calculus
The Jacobian matrix is crucial in various fields such as robotics, machine learning, and control systems. Its applications include:
-
Robotics: In kinematics, the Jacobian relates the velocities of the joints of a robot to the end-effector's velocity, enabling precise control and path planning.
-
Machine Learning: It plays a key role in optimization methods for training neural networks by providing gradients that help in minimizing loss functions.
-
Control Systems: The Jacobian is used for linearization around operating points, simplifying the design of controllers by approximating nonlinear systems with linear ones.
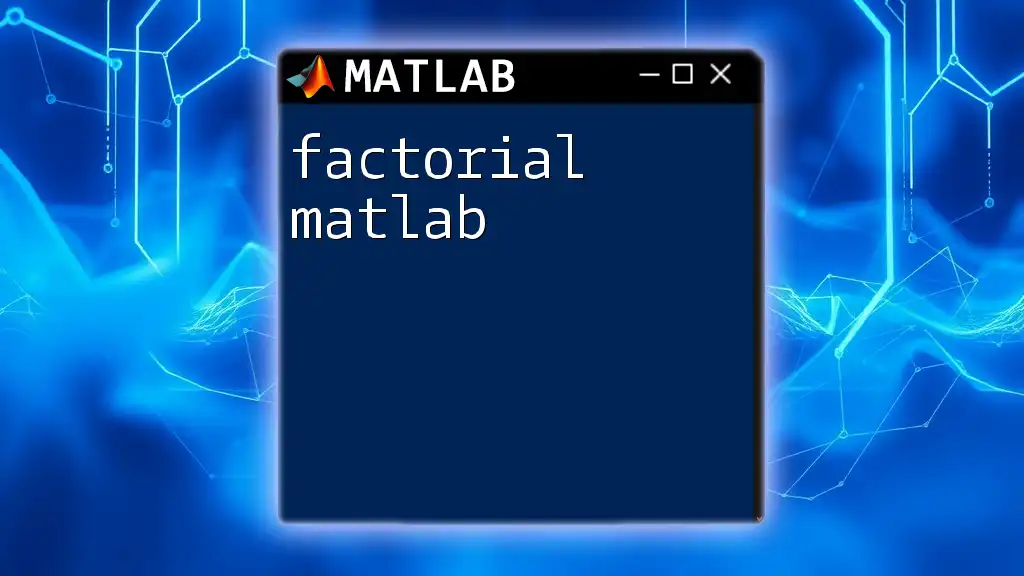
Jacobian in MATLAB
Basic MATLAB Syntax for the Jacobian
MATLAB provides a powerful function, `jacobian`, that can compute the Jacobian matrix of a symbolic function directly. To utilize this, you need to define the variables and the function.
Here’s how you can calculate the Jacobian in MATLAB:
syms x y
f = [x^2 + y; x*y^2];
J = jacobian(f, [x, y])
In this example, `f` is a vector-valued function consisting of two components, and `J` will be the resultant Jacobian matrix. The output will reveal the structure of partial derivatives, demonstrating how the function changes with respect to each variable.
Detailed Coverage of Jacobian in MATLAB
Symbolic vs. Numeric Jacobian
MATLAB can perform both symbolic and numeric calculations for the Jacobian, depending on the problem context.
Understanding Symbolic Computation
Utilizing the symbolic toolbox in MATLAB allows you to derive exact expressions for the Jacobian. This is incredibly useful for theoretical analysis and when precise formulas are required.
Here’s an example illustrating symbolic computation:
syms x y
f = [x^2 + y; x*y^2];
J_symbolic = jacobian(f, [x, y])
The result will yield a symbolically defined Jacobian matrix, showcasing how each component of `f` is dependent on `x` and `y`.
Performing Numeric Jacobian Calculations
In many practical scenarios, particularly when dealing with numerical data, you may wish to use numeric approximations for Jacobian calculations. One common method involves finite differences.
To compute a numeric Jacobian, first, substitute specific values into the symbolic Jacobian:
x_val = [1; 2]; % Example point
J_numeric = double(subs(J_symbolic, [x, y], x_val));
By providing specific values for `x` and `y`, MATLAB computes a numeric approximation of the Jacobian at that point. This is useful in simulations where exact derivatives are less important.
Visualizing the Jacobian
Visualization is a powerful tool for understanding mathematical functions and their derivatives. Graphing the functions along with their Jacobians can illuminate how changes in input values affect outputs.
Example: Plotting 2D Functions
You can create contour plots to visualize how a function behaves in relation to its variables. The following MATLAB code snippet illustrates this by plotting a simple function:
f_plot = @(x,y) x.^2 + y; % Define the function to plot
fcontour(f_plot, [-2, 2, -2, 2]);
title('Contour plot of f(x,y) = x^2 + y');
This code generates a contour plot for the function \(f(x,y)=x^2+y\) over the domain specified. Visual aids like this can significantly enhance the interpretation of how functions change, as well as provide insights into the role of the Jacobian in those changes.
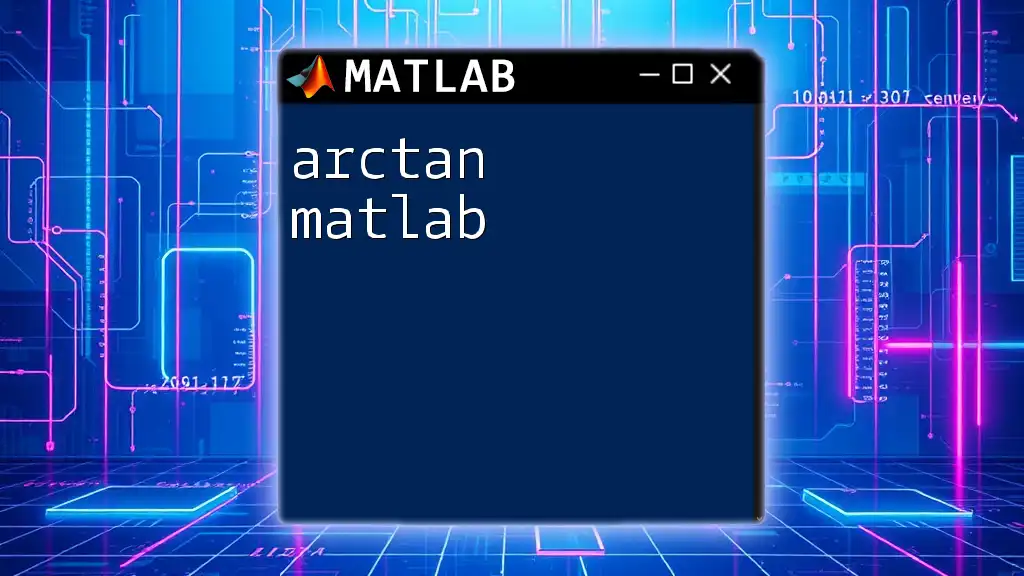
Advanced Techniques Using Jacobians in MATLAB
Jacobian for Nonlinear Systems
Complex systems often possess nonlinear relationships that can be analyzed using Jacobians. Obtaining the Jacobian for such systems typically involves deriving the partial derivatives at specific points in the state space.
For example, to find the Jacobian of a nonlinear system dynamically defined by \(\mathbf{f}\):
syms x1 x2
f_nonlin = [x1^2 + x2^3; sin(x1*x2)];
J_nonlin = jacobian(f_nonlin, [x1, x2])
This calculates the Jacobian matrix for the nonlinear function providing insights into changes in its output as the input varies, which can be vital in simulations and stability analysis.
Jacobians in Optimization Problems
The Jacobian is also utilized in optimization contexts, particularly in gradient descent algorithms, where it provides the necessary gradients for navigating the solution space efficiently.
Example: Using the Jacobian in Optimization
In a practical case, consider you need to minimize a function defined as:
f_obj = @(x) x(1)^2 + x(2)^2; % Objective function
options = optimoptions('fminunc', 'Algorithm', 'quasi-newton', 'GradObj', 'on');
x0 = [1, 1]; % Initial guess
[x_opt, fval] = fminunc(f_obj, x0, options);
Here, `fminunc` optimizes the function defined by `f_obj`, utilizing the gradient (which relates back to the Jacobian) to determine how to adjust the current guess `x0` toward the minimum of the function. This demonstrates the effectiveness of the Jacobian in convergence methods for optimization problems.
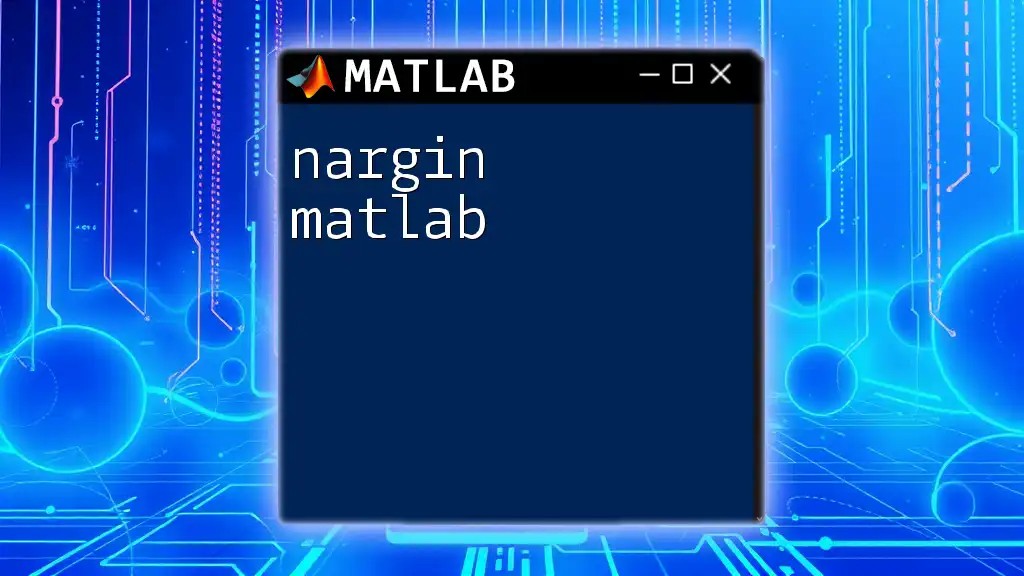
Conclusion
The Jacobian matrix is a crucial mathematical tool that unlocks powerful applications across various domains, from robotics to optimization. MATLAB’s robust features make it an ideal platform for calculating and visualizing Jacobians. By mastering the use of the `jacobian` function and understanding both symbolic and numeric computations, as well as their applications in visualizations and optimizations, you position yourself for success in fields that heavily rely on multivariable calculus.
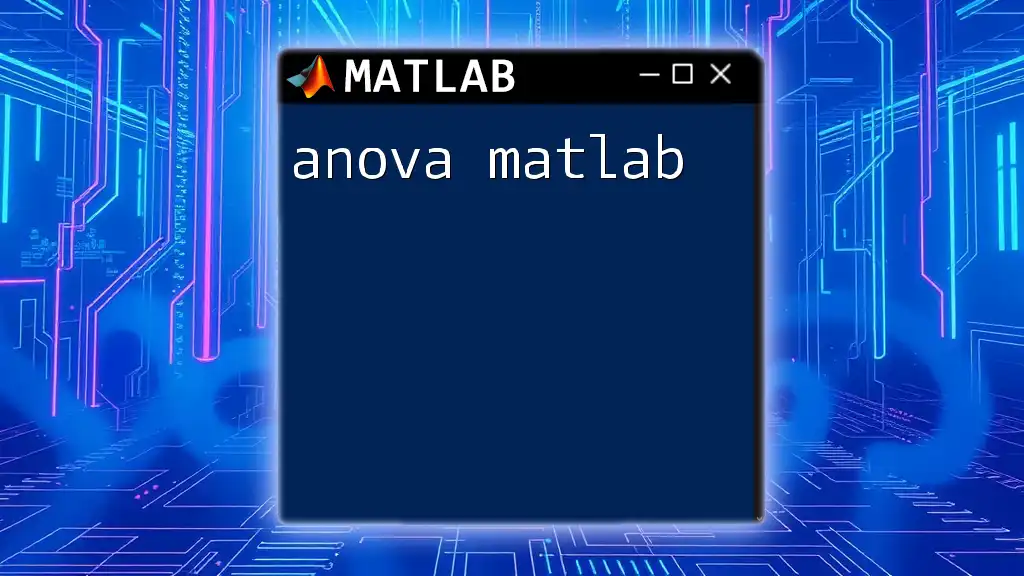
Additional Resources
To further enhance your understanding of Jacobians and their applications within MATLAB, consider consulting the following resources:
- MATLAB Documentation on Jacobians
- Online tutorials and courses specific to MATLAB
- Books focusing on multivariable calculus and robotics
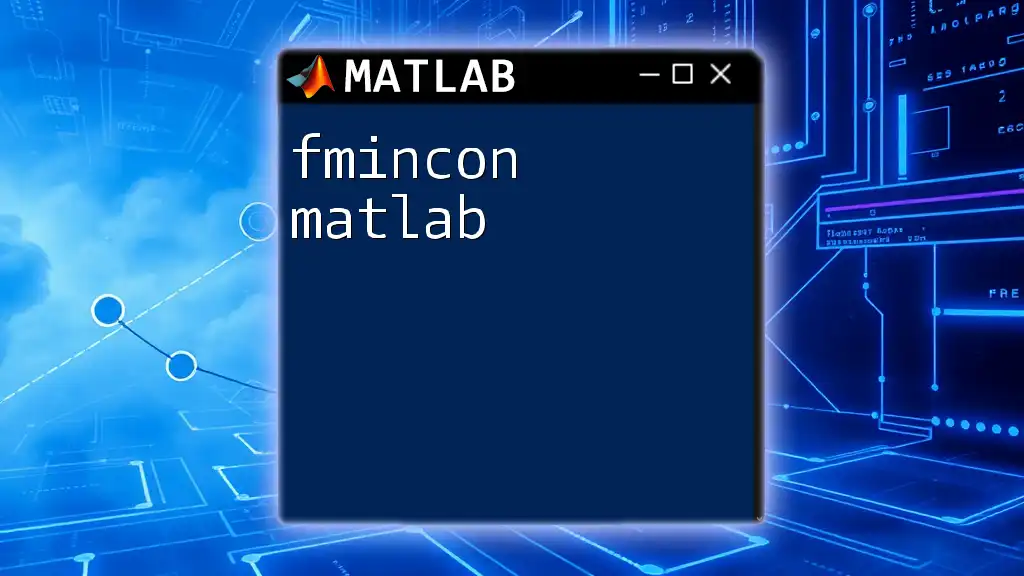
Call to Action
Ready to take your MATLAB skills to the next level? Join our MATLAB learning community and unlock the potential to master commands like the Jacobian swiftly and effectively!