The MATLAB Optimization Toolbox provides a collection of functions for solving various optimization problems, enabling users to efficiently find the minimum or maximum of objective functions.
Here’s a simple example of how to use the `fminunc` function to find the minimum of a given function:
% Example function to minimize
objFun = @(x) (x(1)-2)^2 + (x(2)-3)^2;
% Initial guess
x0 = [0, 0];
% Call to fminunc for optimization
[x, fval] = fminunc(objFun, x0);
disp(['Minimum point: ', mat2str(x)]);
disp(['Function value at minimum: ', num2str(fval)]);
Key Features of the Optimization Toolbox
The MATLAB Optimization Toolbox offers extensive functionality for various optimization techniques. It is designed to cater to both simple and complex scenarios, making it suitable for a wide range of applications in engineering and data science.
Extensive Functionality
-
Linear Programming: The toolbox supports linear optimization problems, where the objective function and constraints are linear. This is particularly useful in scenarios such as resource allocation, supply chain management, and production planning.
-
Nonlinear Programming: Nonlinear optimization allows for problems where either the objective or the constraints are nonlinear. With functions like `fminunc` and `fmincon`, users can address a variety of real-world challenges, from engineering design to financial modeling.
-
Mixed-Integer Programming: This feature allows for optimization problems that involve both continuous and discrete variables. This capability is critical in applications where decisions must be made in whole units, such as scheduling, routing, and facility location problems.
Multi-objective Optimization
What is Multi-objective Optimization?: In many real-world problems, you may need to optimize multiple conflicting objectives simultaneously. For example, maximizing profit while minimizing environmental impact. The MATLAB Optimization Toolbox provides tools to efficiently handle these scenarios.
How the Toolbox Supports Multi-objective Problems: The toolbox offers functions like `gamultiobj` and `paretosearch`, which utilize evolutionary algorithms and other heuristics to find a set of optimal trade-offs known as the Pareto front.
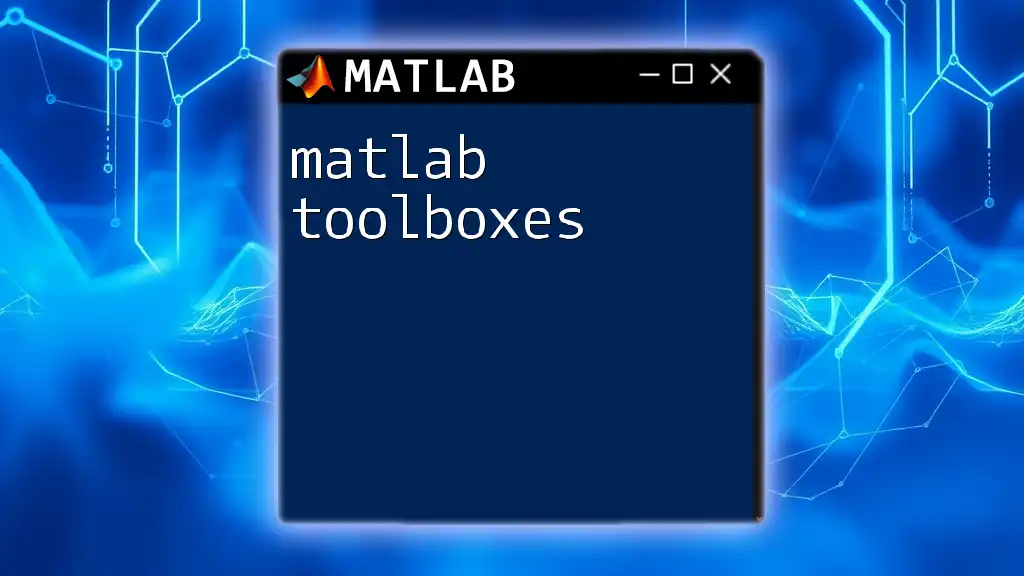
Getting Started with the Optimization Toolbox
Installation and Setup
To begin using the MATLAB Optimization Toolbox, you need to ensure that it's installed. You can do this via the Add-On Explorer in MATLAB. Simply search for "Optimization Toolbox", click on the installation link, and follow the prompts. Once installed, verify the installation by typing the following command in your MATLAB command window:
ver
Basic Commands and Functions
An essential first step in using the toolbox is to familiarize yourself with crucial commands. `optimoptions` allows users to set options for solvers. The most commonly used functions include:
- `fminunc`: For unconstrained nonlinear optimization.
- `fmincon`: For constrained nonlinear optimization.
- `linprog`: For linear programming problems.
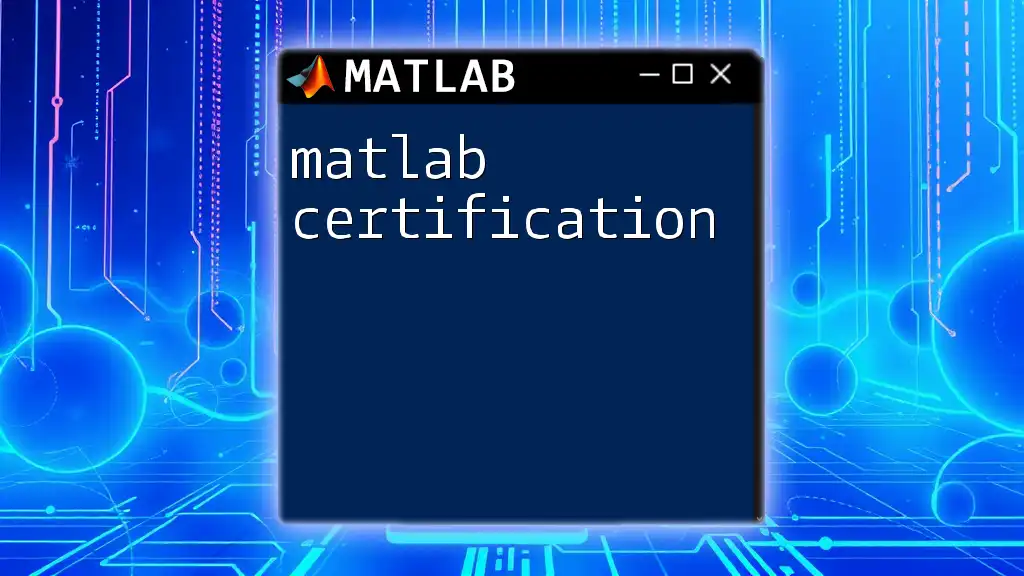
Types of Optimization Problems
Linear Optimization
Understanding linear programming involves defining objective functions and constraints within a linear framework. A typical example is solving supply chain optimization problems, minimizing costs while meeting demand.
% Example MATLAB Code for Linear Programming
f = [-4, -3]; % Objective Function Coefficients
A = [1, 2; 4, 1]; % Coefficients for Inequality Constraints
b = [8; 12]; % Bounds
lb = zeros(2,1); % Lower Bounds
options = optimoptions('linprog','Display','none');
[x, fval] = linprog(f, A, b, [], [], lb, [], options);
disp(x); % Display Solution
In this snippet, we define the objective coefficients, constraints, and call the `linprog` function to solve the problem, capturing the optimal solution in `x`.
Nonlinear Optimization
Nonlinear optimization is frequently used for problems where the relationship between variables is not linear. For instance, consider minimizing the following function:
% Example MATLAB Code for Nonlinear Optimization
fun = @(x) (x(1)-1)^2 + (x(2)-2)^2; % Objective Function
x0 = [0, 0]; % Initial Guess
options = optimoptions('fminunc','Display','iter');
[x,fval] = fminunc(fun,x0,options);
disp(x); % Display Solution
In this example, a function is defined and optimized to find its minimum. The variable `x` holds the optimal inputs that minimize the function.
Mixed-Integer Optimization
Mixed-integer programming is essential when dealing with problems that require both integer and continuous decision variables. A common application is in scheduling.
% Example MATLAB Code for Mixed-Integer Programming
f = [-3, -2]; % Objective Function
intcon = 1; % Specify integer constraint
A = [1, 1; -1, 1; 1, -1]; % Constraints
b = [5; 2; 2];
lb = zeros(2,1); % Lower Bounds
[x,fval] = intlinprog(f,intcon,A,b,[],[],lb,[]);
disp(x); % Display Solution
This code showcases how to solve a mixed-integer problem with specified objectives and constraints, returning the optimal decision variables.
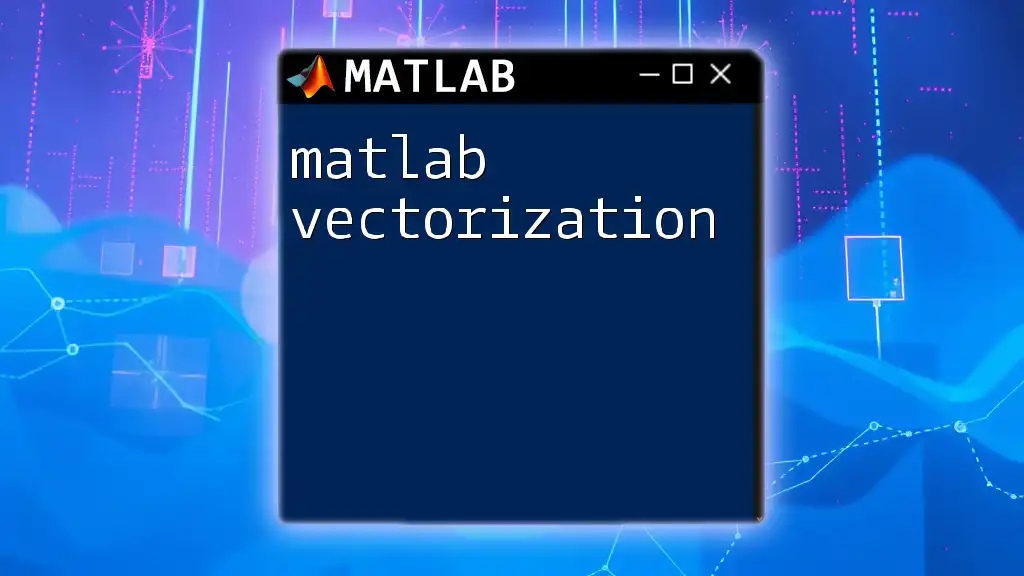
Implementing Optimizations in MATLAB
Using Optimization Options
The `optimoptions` structure allows customization and tuning of solvers to enhance performance and convergence rates. Options like tolerances, maximum iterations, and display settings can all be modified to suit the specific needs of your optimization task.
Interpreting Results
Interpreting the results of an optimization can be as crucial as the optimization itself. Understanding the output functions, such as constraints, objective values, and reason for convergence, helps in refining models and making informed decisions. Additionally, visualizing optimization results, through plotting paths or objective function values, can provide deeper insights.
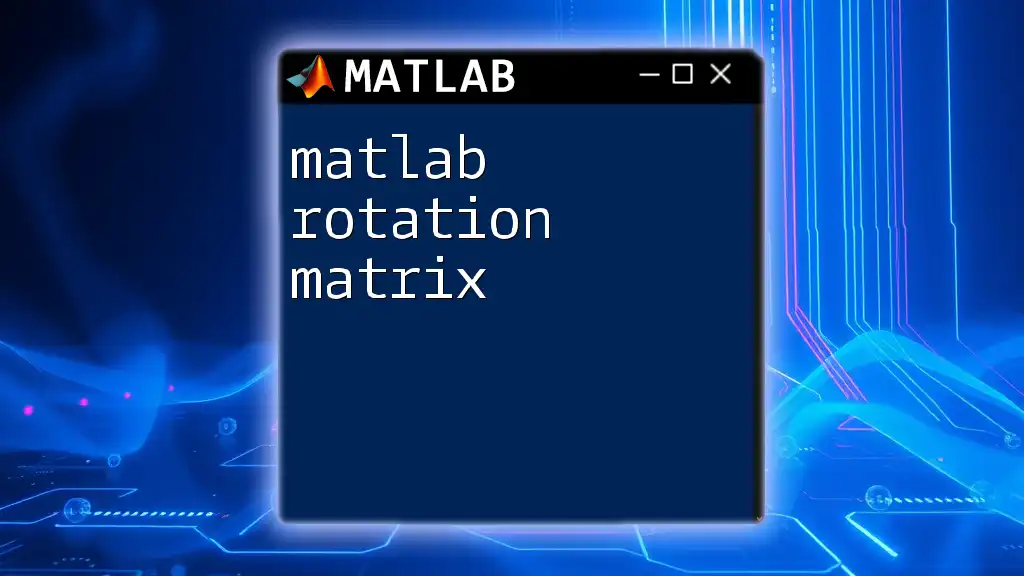
Use Cases of Optimization Toolbox in Industries
Engineering Applications
In engineering, optimization is key for structural and design efficiencies. The toolbox can be used to optimize material usages, load distributions, and overall designs reducing weights without compromising safety.
Data Analytics
In the realm of data analytics, optimization plays a fundamental role in model development. Techniques such as hyperparameter tuning in machine learning models directly benefit from capabilities in the Optimization Toolbox.
Finance Optimization
Portfolio optimization techniques utilize uncertainty and return profiles to maximize returns while minimizing risk. By applying the toolbox, financial analysts can solve for the best asset allocation strategies.
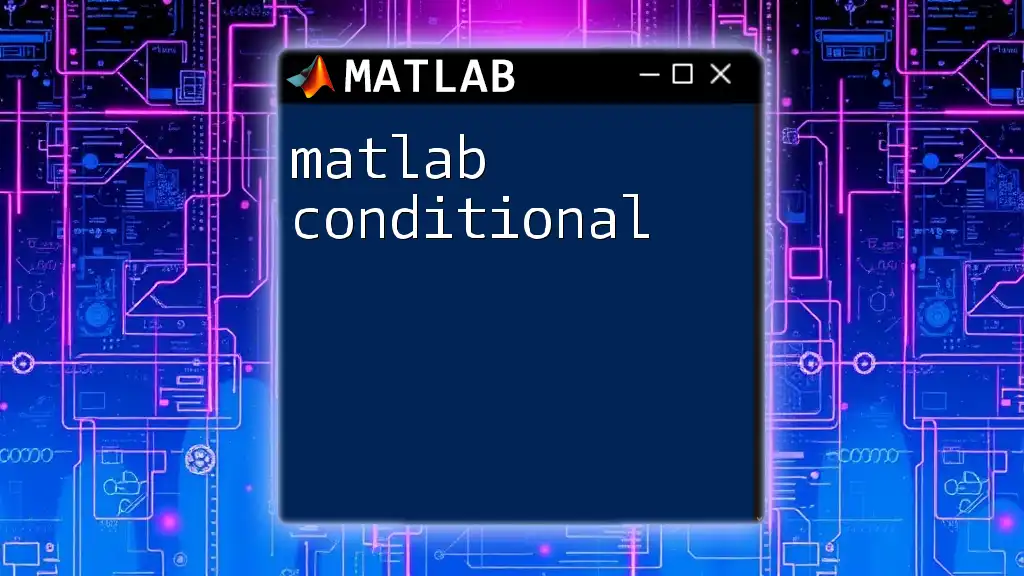
Advanced Topics in Optimization
Sensitivity Analysis
Sensitivity analysis examines how the variability in output can be attributed to changes in input variables. Using the toolbox, users can determine how sensitive their optimization results are to the changes in parameters, enabling better risk assessment.
Parallel Computing in Optimization
Parallel computing can significantly enhance computational efficiency in optimization problems. By distributing calculations across multiple processors, large-scale problems can be solved more quickly. The toolbox supports parallel processing through functions like `parfor` and `MultiStart`, allowing users to explore multiple initial conditions simultaneously.
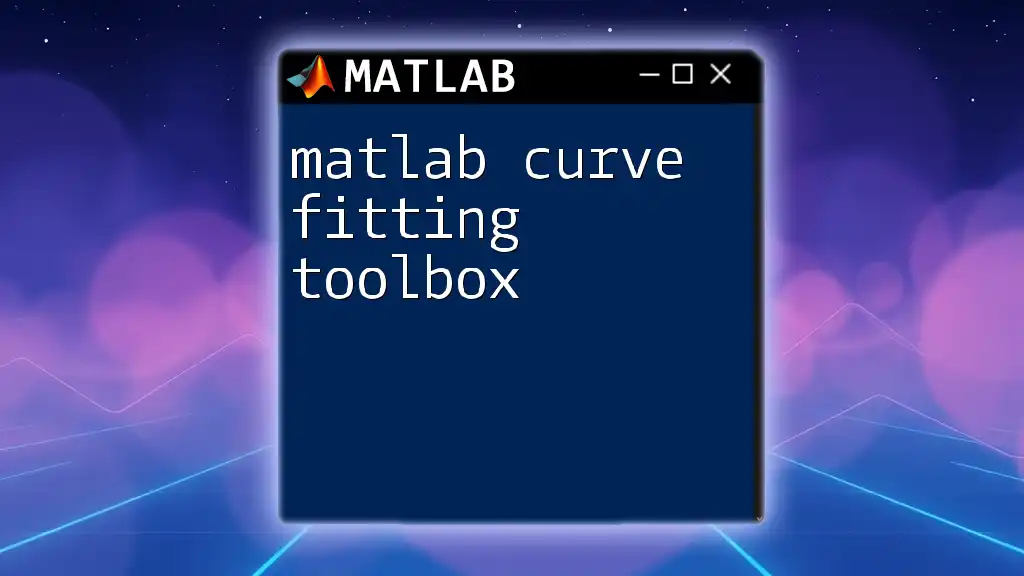
Tips and Best Practices
Common Pitfalls in Optimization
Navigating the optimization landscape often involves pitfalls such as falling prey to local minima. Using global optimization strategies available in the toolbox, such as `globalSearch` or `multistart`, can help mitigate these risks.
Practical Tips for Troubleshooting
When issues arise, debugging optimization problems becomes essential. Use diagnostic outputs and visualizations to identify convergence issues, problematic constraints, or unsuitable initial guesses, allowing for effective troubleshooting.
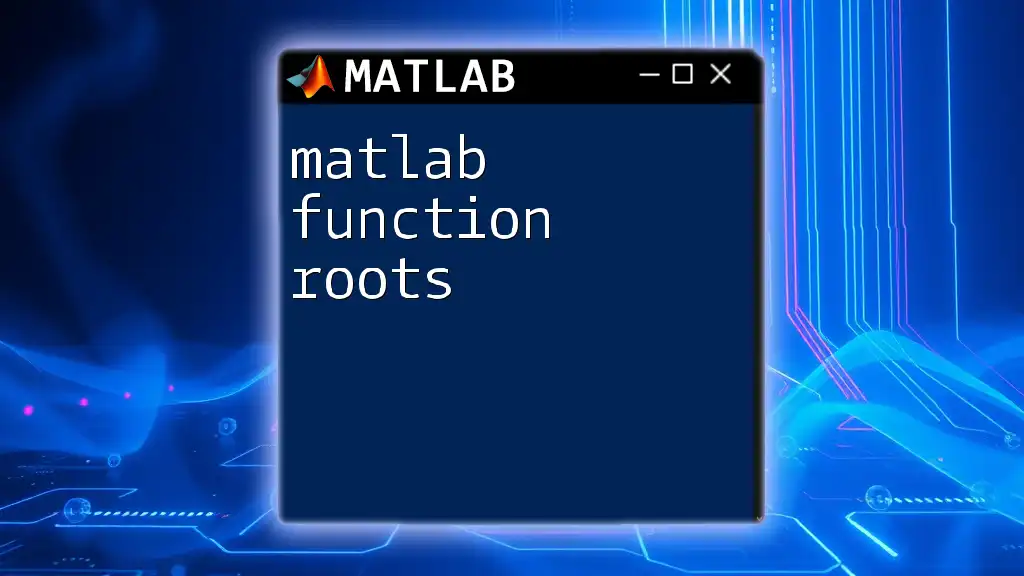
Conclusion
The MATLAB Optimization Toolbox is a powerful suite of tools designed for tackling a diverse range of optimization problems, from linear programming to complex mixed-integer challenges. By understanding its features and functionalities, users can effectively enhance decision-making processes across various fields. Experimenting with different types of optimization scenarios will deepen your mastery of the toolbox, paving the way for innovative solutions to real-world problems.
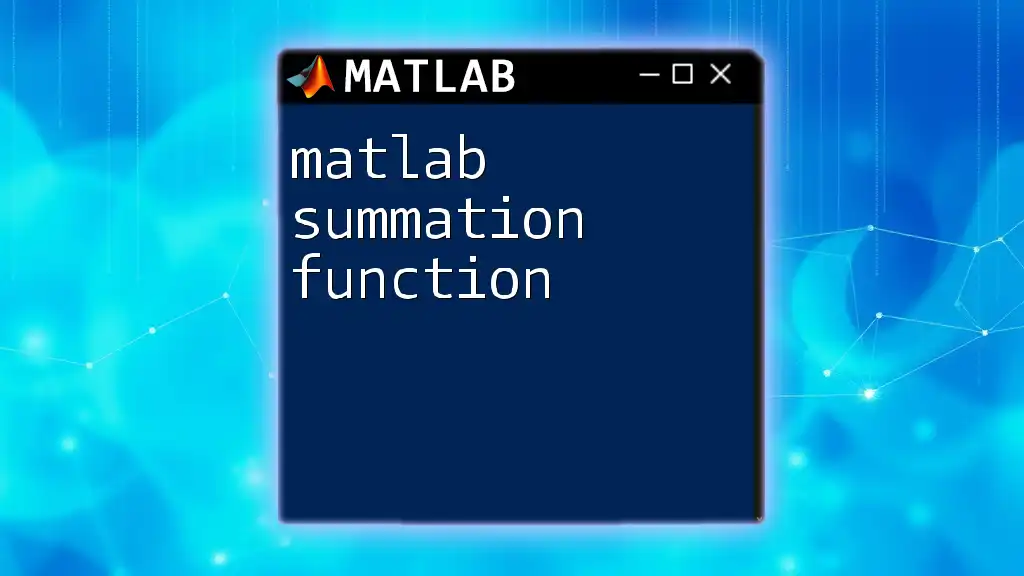
References and Further Reading
For additional learning and exploration, users can refer to the official MATLAB documentation, recommended texts on optimization techniques, and online courses focusing on practical applications of the Optimization Toolbox.