In MATLAB, conditional statements allow you to execute certain blocks of code based on whether a specified condition is true or false.
Here’s a simple example using an if-else statement:
x = 10;
if x > 5
disp('x is greater than 5');
else
disp('x is less than or equal to 5');
end
Understanding Logical Conditions
What are Logical Conditions?
Logical conditions are crucial components in programming that allow developers to control the flow of the program based on certain criteria. In the context of MATLAB, logical conditions enable users to execute specific blocks of code if particular conditions are met. This allows for dynamic and flexible programming, which is essential for developing complex algorithms and functionalities.
Types of Logical Conditions
In MATLAB, there are several common logical operators that can be used to construct conditions:
- AND (&&): Evaluates to true only if both expressions are true.
- OR (||): Evaluates to true if at least one of the expressions is true.
- NOT (~): Reverses the logical state of its operand.
These operators can be combined to create more complex conditional statements, making them powerful tools for decision-making in your MATLAB scripts.
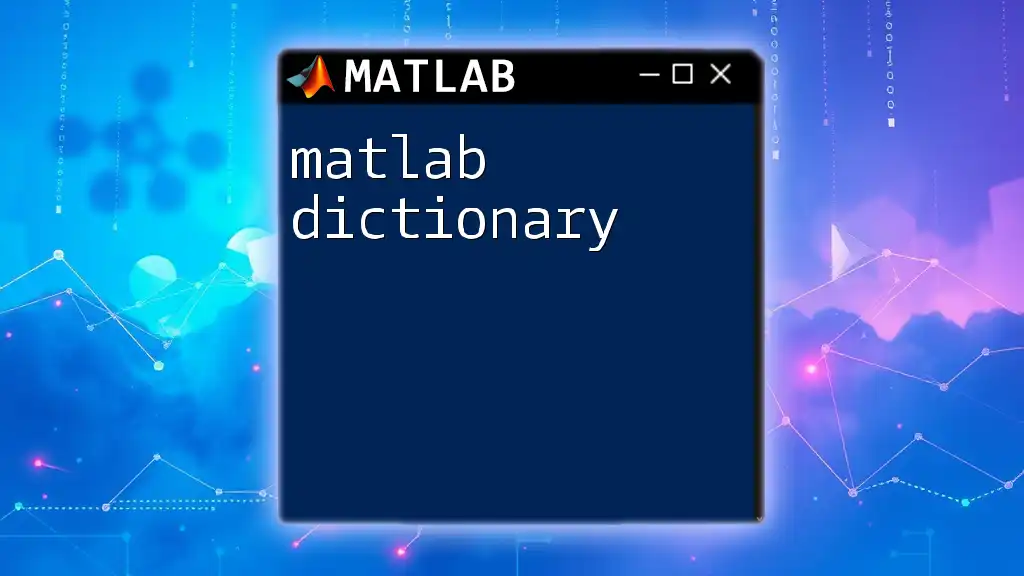
The If Statement
Syntax and Structure
The if statement is one of the most fundamental elements of conditional programming in MATLAB. This statement allows you to execute a block of code based on whether a specified condition evaluates to true. The basic structure is as follows:
if condition
% execute code
end
If the `condition` is true, the code between `if` and `end` will run; if it’s false, the code will not execute.
Using If-Else
Basic If-Else Structure
To manage two possible outcomes, use the if-else statement. This allows for one block of code to run if the condition is true, and another block of code to run if the condition is false. Here’s a simple example:
if number > 0
disp('Number is positive');
else
disp('Number is not positive');
end
In this example, if `number` is greater than zero, it displays "Number is positive"; otherwise, it outputs "Number is not positive".
Nested If Statements
Sometimes, you may need to evaluate additional conditions based on the result of the first condition. This can be achieved through nested if statements. Here’s how it works:
if condition1
% code block 1
elseif condition2
% code block 2
else
% code block 3
end
This structure allows for multiple conditions to be assessed sequentially. If `condition1` is true, `code block 1` executes. If not, MATLAB checks `condition2`, executing `code block 2` if it’s true. If neither condition is true, `code block 3` will execute.
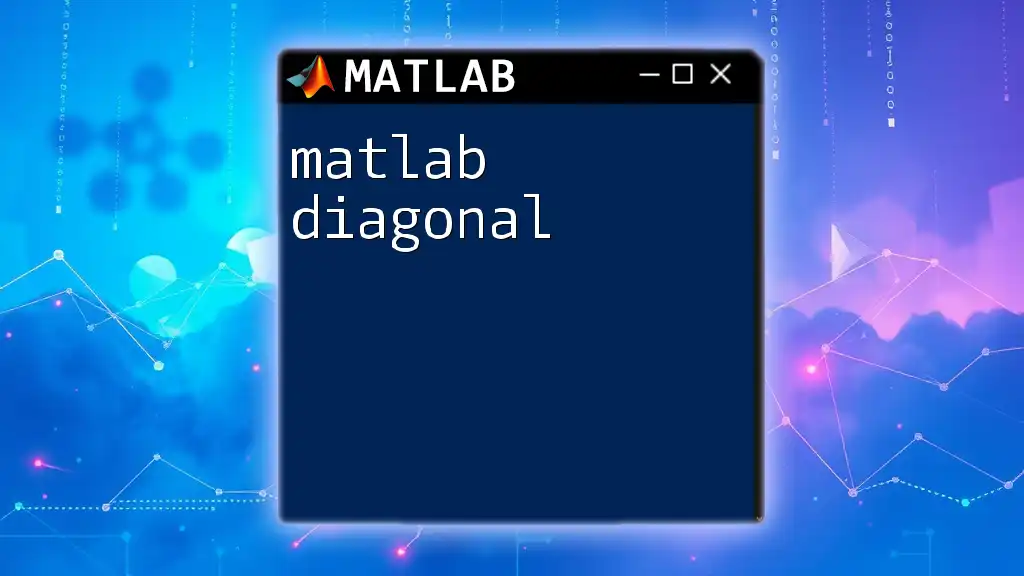
The Switch Statement
Introduction to Switch
For cases where you need to evaluate a single expression against multiple potential values, the switch statement can be a more readable alternative to lengthy if-else chains. The basic syntax of a switch statement is as follows:
switch expression
case value1
% code block 1
case value2
% code block 2
otherwise
% default code block
end
Example Use Case
Here’s an example that illustrates how a switch statement can simplify your code logic:
day = 'Monday';
switch day
case 'Monday'
disp('Start of the week!');
case 'Friday'
disp('End of the work week!');
otherwise
disp('Just another day.');
end
In this case, if the variable `day` contains "Monday," MATLAB will display "Start of the week!" and follow similarly for other cases or fallback to the default message.
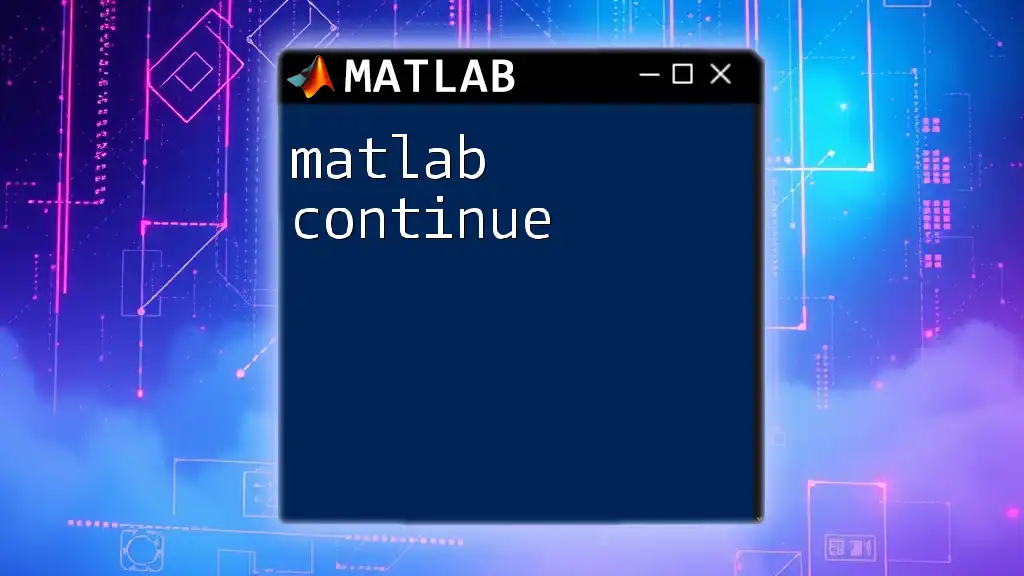
Logical Indexing in MATLAB
What is Logical Indexing?
Logical indexing allows you to reference elements of arrays or matrices based on conditions represented by logical values (true or false). This feature enhances data manipulation efficiency by enabling selections without explicitly looping through elements.
Using Logical Indexing
Here’s a brief illustration:
A = [1, 2, 3, 4, 5];
index = A > 3; % returns [false, false, false, true, true]
B = A(index); % returns [4, 5]
In this example, `index` is a logical array that identifies the elements in `A` that are greater than 3. The result stored in `B` will be the array containing only elements satisfying this condition.
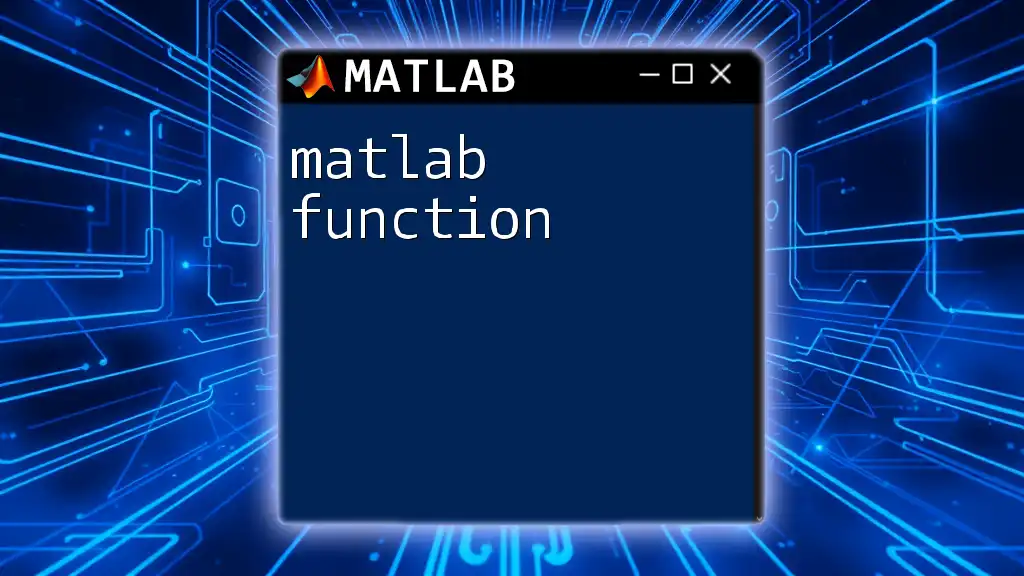
Conditional Functions
Introduction to Conditionals in Functions
Incorporating conditionals within MATLAB functions allows you to produce different outputs based on varying inputs. This capability is vital in creating flexible and reusable code.
Example Function with Conditionals
Here’s a simple function that utilizes an if statement to check whether a number is even or odd:
function checkEvenOdd(num)
if mod(num, 2) == 0
disp('Even');
else
disp('Odd');
end
end
When you call `checkEvenOdd(4)`, it will return "Even"; whereas `checkEvenOdd(7)` will return "Odd".
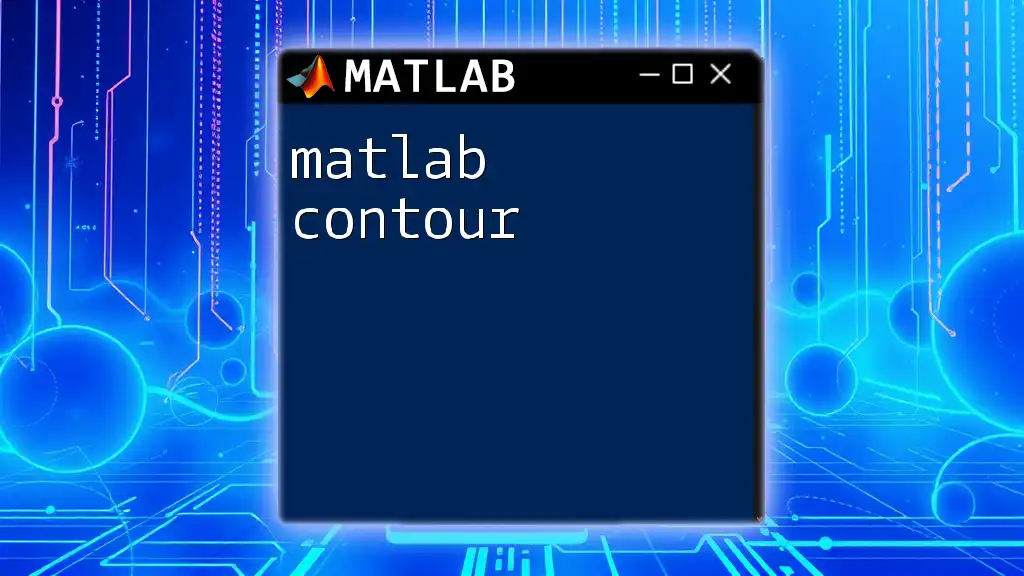
Best Practices for Using Conditionals
Code Readability
It's essential to maintain clear and logical structure in your conditionals. Well-structured code not only facilitates understanding but also supports easier debugging and enhancements in the future.
Avoiding Complexity
Avoid creating overly complex conditional logic, as it can become difficult to read and maintain. When conditions become unwieldy, consider breaking them down into smaller, more manageable functions or using switch statements to enhance clarity.
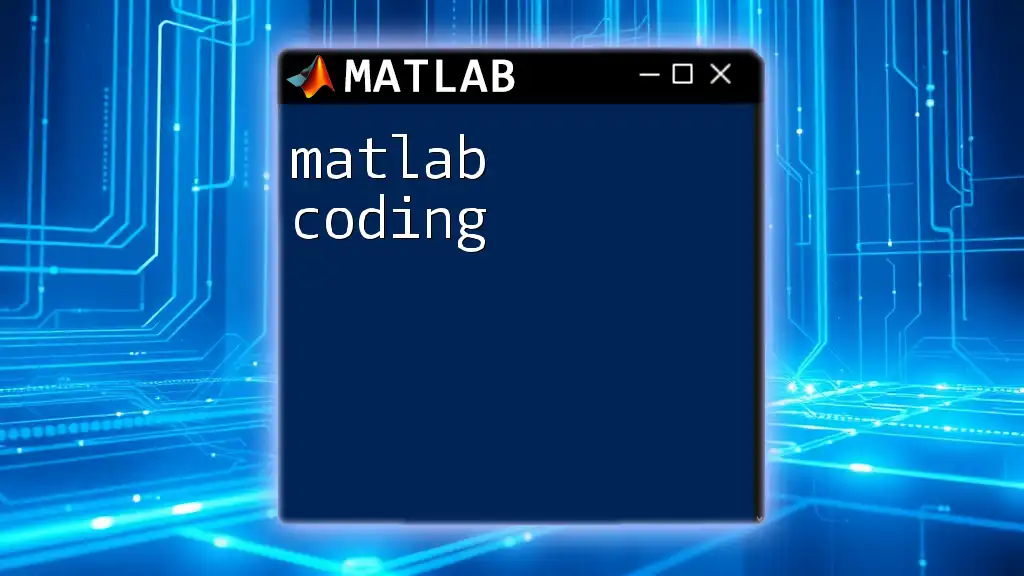
Conclusion
MATLAB conditionals—a combination of if statements and switch statements, supported by logical operators—are essential in defining the behavior of scripts and functions. Understanding how to effectively utilize these constructs will empower you to make decisions in your code dynamically.
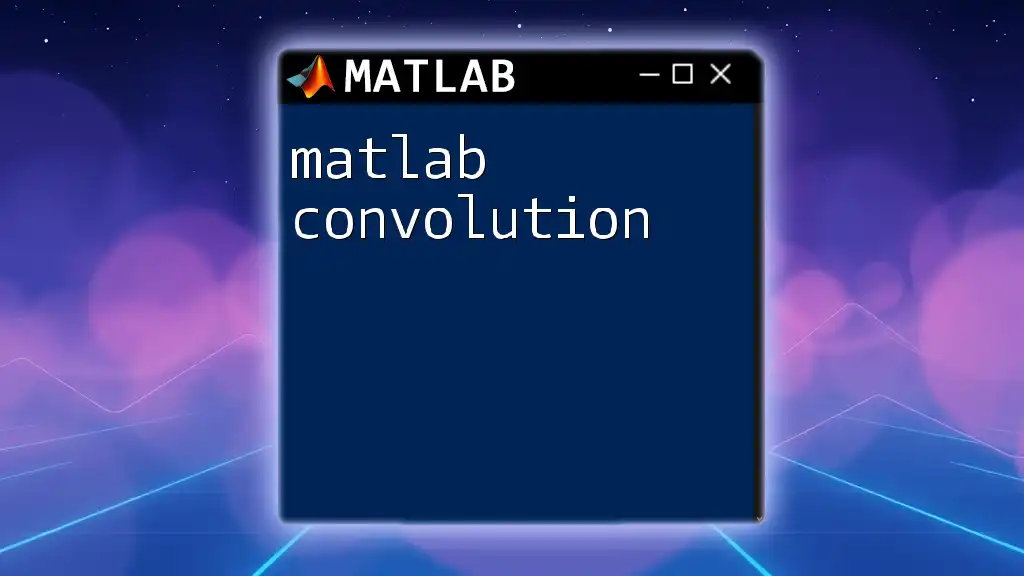
Call to Action
Subscribe for more tips and concise guides on MATLAB and share your experiences or queries related to MATLAB conditionals. Engaging with the community can elevate your learning experience!
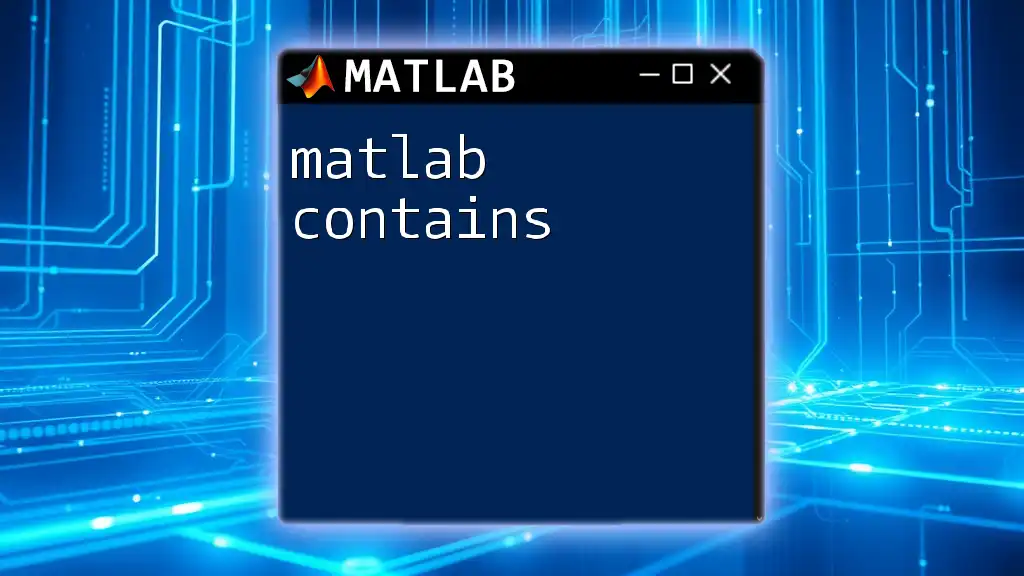
Additional Resources
For deeper exploration, refer to the official MATLAB documentation on conditionals, alongside recommended books and online courses designed to enhance your MATLAB programming skills.