MATLAB interpolation is a technique used to estimate unknown values from known data points, enabling smoother data representation and analysis.
% Example of linear interpolation using MATLAB's interp1 function
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
xi = 1.5; % Point to interpolate
yi = interp1(x, y, xi);
disp(yi); % Output: 2.5
Understanding Interpolation
What is Interpolation?
Interpolation is a mathematical technique used to estimate unknown values that fall within the range of known data points. By connecting these known points with a specific formula, interpolation can create a continuous function that accurately represents the relationship between the data points. It is especially valuable when one needs to predict values, fill in gaps in datasets, or smooth data for analysis.
When to Use Interpolation?
Interpolation is particularly useful in various scenarios:
- Data Analysis: When working with incomplete datasets, interpolation can estimate missing values.
- Signal Processing: Interpolation aids in reconstructing signals from sampled data.
- Engineering and Science: It is commonly employed in computational simulations and modeling.
The benefits of interpolation include increased data accuracy and enhanced visualization, making it a critical tool in many technical fields.
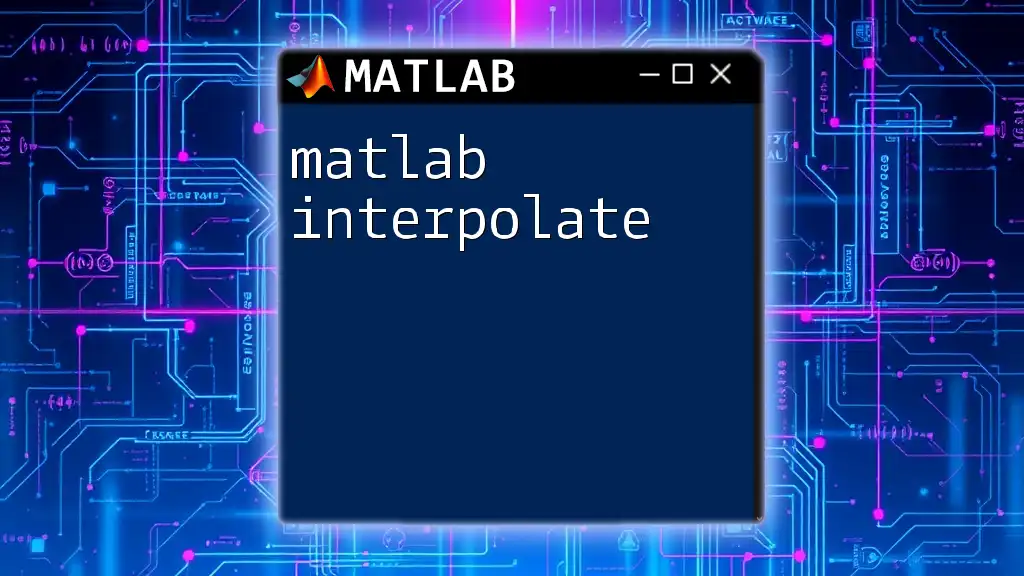
MATLAB and Interpolation
Why Use MATLAB for Interpolation?
MATLAB is a powerful programming environment known for its extensive mathematical capabilities. The platform stands out for the following reasons:
- Built-in Functions: MATLAB has numerous built-in functions specifically for various types of interpolation, making it efficient and user-friendly.
- Ease of Use: Its straightforward syntax allows beginners to quickly learn and apply interpolation techniques without extensive programming experience.
- Extensive Documentation: MATLAB provides comprehensive documentation that guides users through the intricacies of interpolation methods and functions.
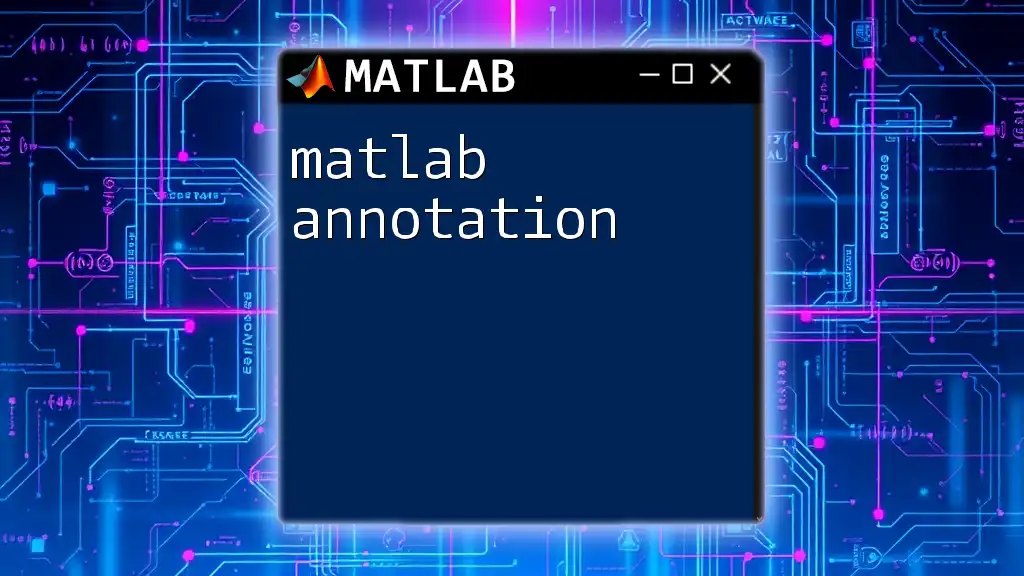
Types of Interpolation in MATLAB
Linear Interpolation
Definition and Explanation
Linear interpolation involves estimating unknown values based on the linear relationship between surrounding data points. It is the simplest form of interpolation, suitable when data points are relatively close to each other.
MATLAB Implementation
To perform linear interpolation in MATLAB, use the `interp1` function. Below is an example:
x = [1, 2, 3, 4];
y = [2, 3, 5, 7];
xi = 2.5; % The point where we want to interpolate
yi = interp1(x, y, xi, 'linear'); % Linear interpolation
fprintf('Interpolated value at %.2f is %.2f\n', xi, yi);
In this example, we have a simple dataset defined by vectors `x` and `y`. The `xi` variable represents the point where we want to estimate the value. Using `interp1`, we calculate the interpolated value `yi`.
Visualizing Linear Interpolation
To illustrate the linear interpolation visually, one can create a plot:
xq = linspace(1, 4, 100);
yq = interp1(x, y, xq, 'linear');
plot(x, y, 'o', xq, yq, '-');
title('Linear Interpolation');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
This plot shows the original points as circles and the interpolated linear function as a continuous line.
Polynomial Interpolation
Definition and Explanation
Polynomial interpolation extends the concept of linear interpolation to higher-degree polynomials. It is beneficial for datasets that exhibit a more complex relationship. However, one must be cautious with the degree of the polynomial, as a high degree can lead to overfitting.
MATLAB Implementation
In MATLAB, one can use the `polyfit` function to fit a polynomial to the dataset, followed by `polyval` to evaluate the polynomial at specific points. Here’s an example:
x = [1, 2, 3, 4];
y = [2, 3, 5, 7];
p = polyfit(x, y, 3); % Cubic polynomial fitting
xi = 2.5;
yi = polyval(p, xi); % Get interpolated value
fprintf('Interpolated value at %.2f is %.2f\n', xi, yi);
The `polyfit` function computes the coefficients for a cubic polynomial that fits the data points, while `polyval` evaluates this polynomial at the point `xi`.
Visualizing Polynomial Interpolation
To visualize the polynomial interpolation, one can plot the data points against the fitted polynomial:
xq = linspace(1, 4, 100);
yq = polyval(p, xq);
plot(x, y, 'o', xq, yq, '-');
title('Polynomial Interpolation');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
This representation helps to illustrate how well the polynomial fits the given data points.
Spline Interpolation
Definition and Explanation
Spline interpolation, particularly cubic splines, involves connecting data points with piecewise polynomial functions. This approach provides a smoother curve compared to polynomial interpolation while avoiding the pitfalls of overfitting.
MATLAB Implementation
Using MATLAB's `interp1` function with the 'spline' option enables cubic spline interpolation. Here’s how it's done:
x = [1, 2, 3, 4];
y = [2, 3, 5, 7];
xi = 2.5;
yi = interp1(x, y, xi, 'spline'); % Spline interpolation
fprintf('Interpolated value at %.2f is %.2f\n', xi, yi);
The code above performs cubic spline interpolation, estimating the value at `xi`.
Visualizing Spline Interpolation
To visualize the smoothness of the spline interpolation:
xq = linspace(1, 4, 100);
yq = interp1(x, y, xq, 'spline');
plot(x, y, 'o', xq, yq, '-');
title('Spline Interpolation');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
This plot demonstrates the smooth curves achieved through spline interpolation compared to other methods.
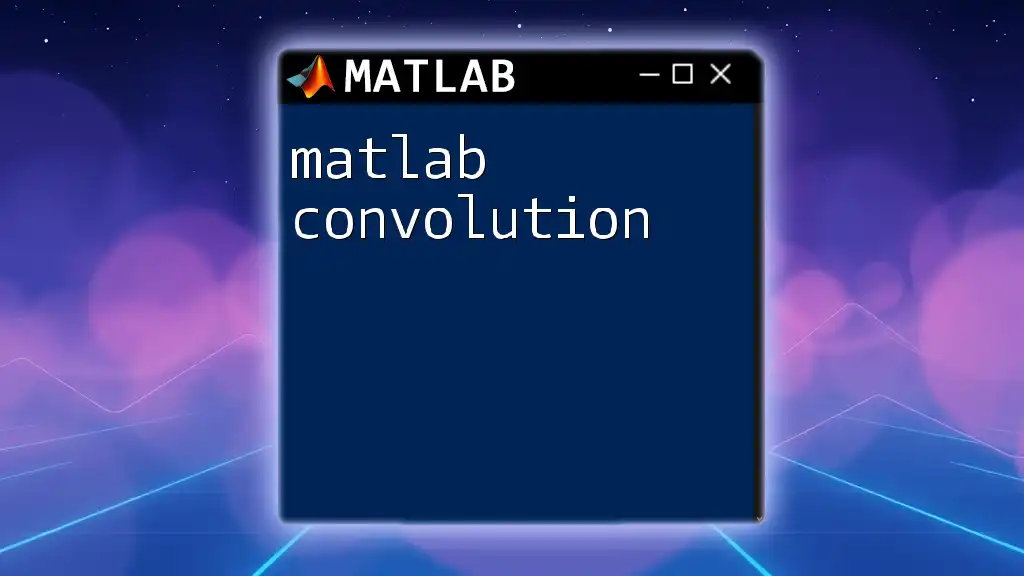
Choosing the Right Interpolation Method
Factors to Consider
Selecting the appropriate interpolation method depends on several factors:
- Data Characteristics: The relationship between data points and their proximity can dictate the method. Linear interpolation may suffice for closely spaced points, while splines can manage more complex datasets.
- Desired Accuracy: If a high level of precision is required, polynomial or spline methods may be more suitable.
- Computational Efficiency: Simpler linear interpolation is generally faster and requires less computation than higher-order polynomial or spline interpolation.
Comparison of Different Methods
Here’s a brief overview of the pros and cons of different interpolation methods:
-
Linear Interpolation
- Pros: Simple and fast; works well for small datasets.
- Cons: Can introduce errors with larger gaps.
-
Polynomial Interpolation
- Pros: Can fit a complex function well.
- Cons: Risk of overfitting and Runge's phenomenon for high degrees.
-
Spline Interpolation
- Pros: Smooth curves; avoids the overfitting issue.
- Cons: More complex to implement and can be computationally expensive.
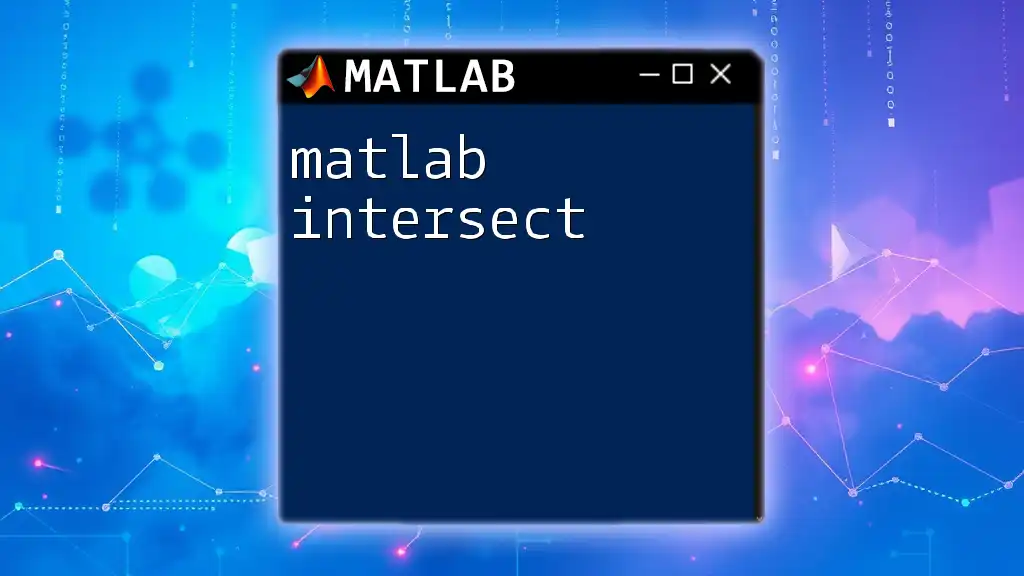
Common Pitfalls in Interpolation
Overfitting vs. Underfitting
When engaging with polynomial interpolation, overfitting is a common issue where a polynomial of too high a degree fits the data points exceedingly well but performs poorly on new data. Conversely, underfitting results from using a polynomial that is too simplistic, failing to capture the underlying trend. It’s crucial to strike a balance and possibly use validation sets to test the model’s effectiveness.
Extrapolation Caution
Extrapolation—estimating values outside the range of known data points—presents its own unique risks. Interpolation functions are only reliable within the span of the existing data, and extending them beyond these bounds can lead to significant inaccuracies.
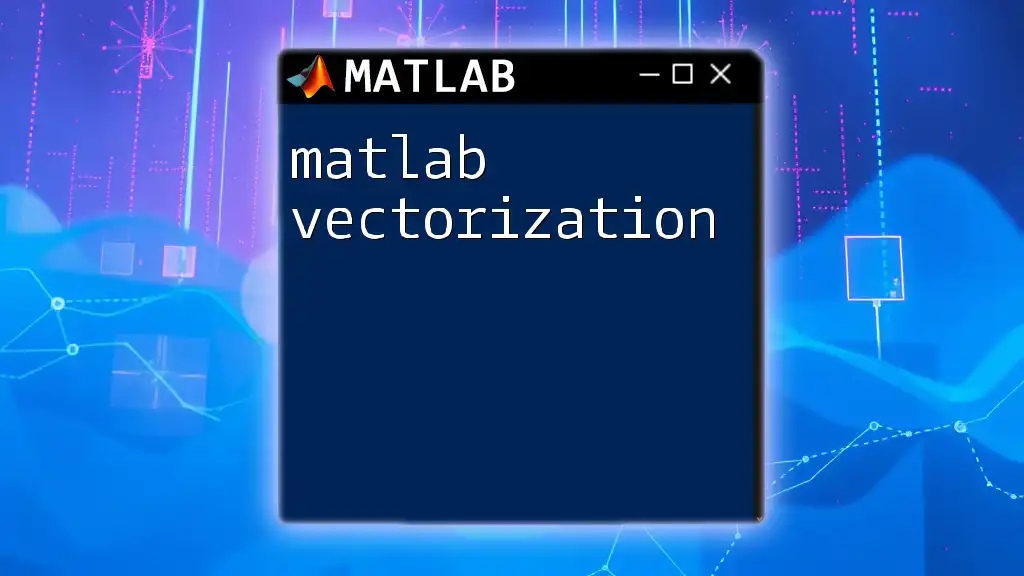
Conclusion
In summary, MATLAB interpolation offers various techniques—linear, polynomial, and spline—each with its strengths and weaknesses. Understanding the context and characteristics of your data is crucial when selecting the right interpolation method. Mastering these techniques can enhance your data analysis skills and pave the way for more sophisticated projects.
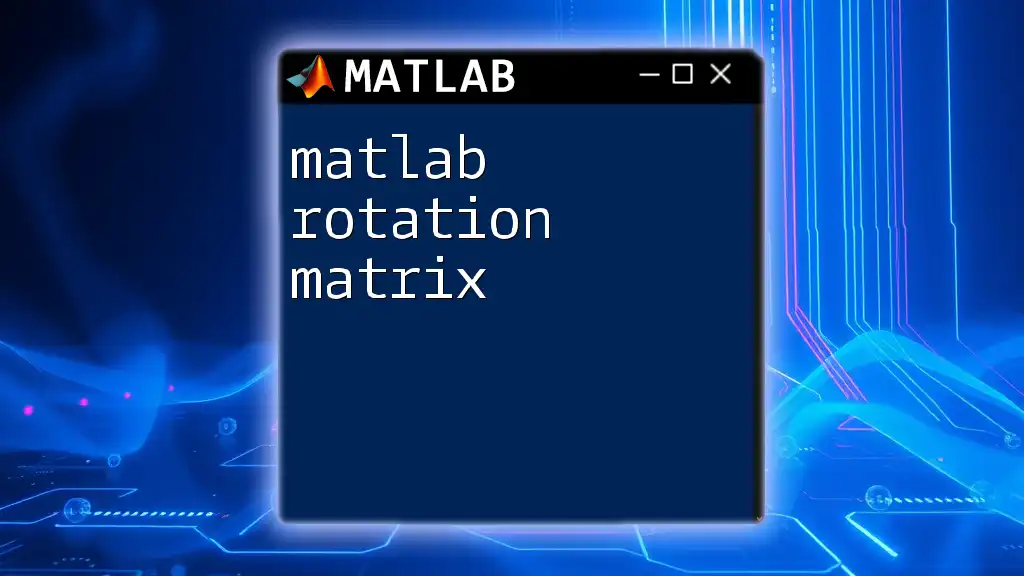
Additional Resources
Explore MATLAB's official documentation for deeper insights and additional examples related to `interp1`, `polyfit`, and visualization techniques. Engaging in community forums can also provide valuable insights and support as you continue to hone your MATLAB skills.