A MATLAB dictionary is a data structure that stores key-value pairs, allowing for efficient data retrieval and organization.
% Example of creating and using a dictionary in MATLAB
dict = containers.Map({'key1', 'key2', 'key3'}, {'value1', 'value2', 'value3'});
value = dict('key2'); % Retrieves 'value2'
What is a MATLAB Dictionary?
A MATLAB dictionary is a data structure that stores data in the form of key-value pairs. It allows for efficient storage and retrieval of data, making it invaluable for various programming tasks. The primary component of a dictionary is its keys, unique identifiers for the values that they map to. In MATLAB, dictionaries are created using the `containers.Map` class, which provides a robust framework for handling associative arrays.

Understanding the Basics of Dictionaries in MATLAB
The Concept of Key-Value Pairs
In a dictionary, each entry consists of two main components: the key and the value. The key is a unique identifier used to access the corresponding value. For example, in a contact list, names can be keys, while phone numbers serve as the associated values. This organization simplifies retrieving data, particularly when datasets grow large and complex.
Creating a Dictionary
To create a dictionary in MATLAB, you utilize the `containers.Map` function. This allows for the initialization of a dictionary with predefined keys and values. Here’s a basic example of how to create a dictionary with some initial data:
% Example of creating a dictionary
myDict = containers.Map({'key1', 'key2'}, {'value1', 'value2'});
In this example, we create a dictionary named `myDict` with two key-value pairs: `'key1'` maps to `'value1'`, and `'key2'` maps to `'value2'`.
Inspecting the Contents of a Dictionary
Once a dictionary is created, you may want to see its contents. You can display all key-value pairs through the command window using the following code:
% Display contents of the dictionary
disp(myDict);
This command will output the keys along with their associated values, providing a clear view of the dictionary's contents.
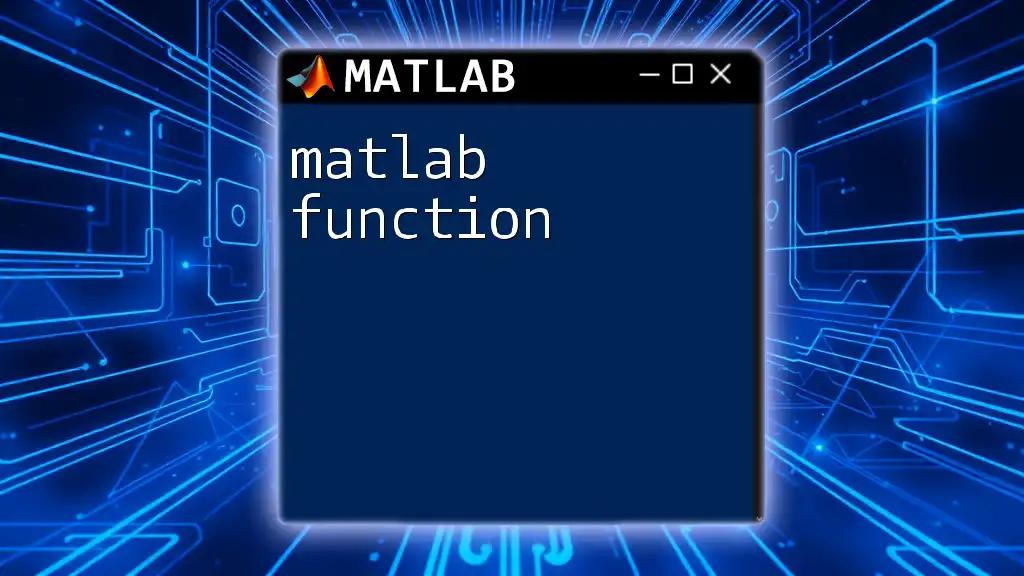
Core Dictionary Operations
Adding and Updating Entries
Adding New Key-Value Pairs
Once you have your dictionary established, you can easily add new entries. To add a new key-value pair, simply assign a value to a new key in the dictionary:
% Adding a new entry
myDict('key3') = 'value3';
Updating Existing Entries
You may also need to update existing values. For instance, if you wish to change the value associated with `'key2'`, you can do so as follows:
% Updating an existing value
myDict('key2') = 'newValue2';
This operation directly modifies `myDict`, replacing the previous value of `'key2'` with `'newValue2'`.
Accessing Values
To retrieve values from a dictionary, you can use the associated key. This operation is straightforward and usually executed in a single line:
% Retrieve a value
value = myDict('key1');
This code retrieves the value corresponding to `'key1'` from `myDict`, storing it in the variable `value`.
Checking for Keys
To ensure a key exists before trying to retrieve its value, MATLAB provides the `isKey` function. This can prevent runtime errors associated with accessing non-existent keys:
% Check if a key exists
if isKey(myDict, 'key1')
disp('Key1 exists in the dictionary.');
end
Removing Entries
If you need to remove an entry from your dictionary, you can use the `remove` function. This function requires the dictionary and the key of the entry you want to delete:
% Removing an entry
remove(myDict, 'key2');
Executing this command will remove the key-value pair for `'key2'`, ensuring that it no longer exists within `myDict`.
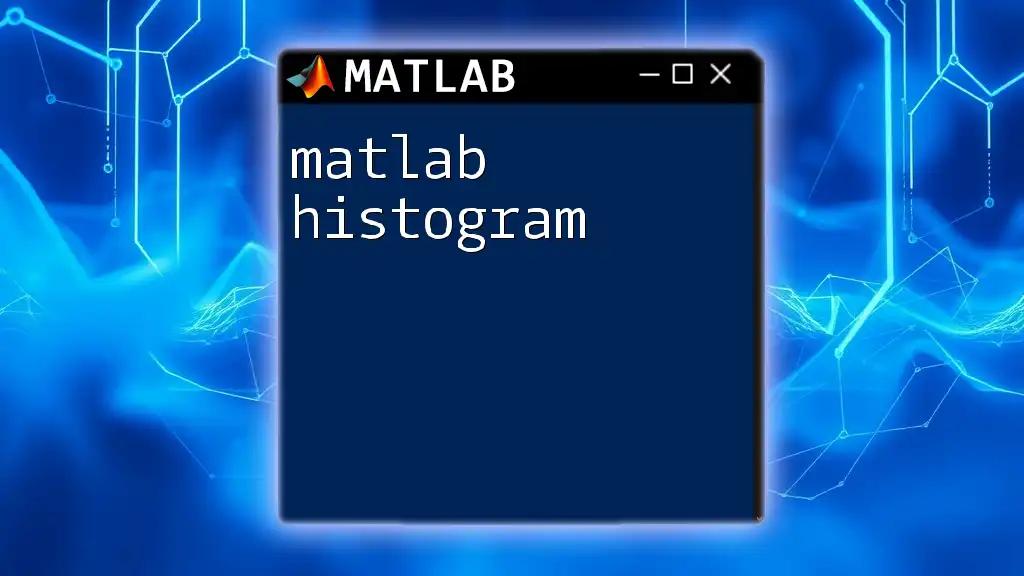
Advanced Dictionary Features
Iterating Through Dictionaries
Dictionaries in MATLAB also allow for iteration over keys and values, which is useful for scenarios where you need to process or analyze all entries within a dictionary.
To get all keys as an array, you can use:
% Get all keys
allKeys = keys(myDict);
Similarly, to retrieve all values, you can use:
% Get all values
allValues = values(myDict);
This flexibility enables you to efficiently handle data within the dictionary.
Nested Dictionaries
MATLAB also supports creating nested dictionaries, which can allow for more complex data structures. You can create a dictionary where the values themselves are dictionaries. For instance:
% Create a nested dictionary
nestedDict = containers.Map('KeyType', 'char', 'ValueType', 'any');
nestedDict('outerKey') = containers.Map({'innerKey1', 'innerKey2'}, {'innerValue1', 'innerValue2'});
In this case, the key `'outerKey'` maps to another dictionary that contains its own keys and values.
Performance Considerations
When working with large datasets, a MATLAB dictionary can significantly improve performance. Its hash-table underlying structure allows for quick lookups, making it ideal for applications requiring frequent data access. It’s particularly useful when you have unordered collections of data that require fast insertion and retrieval.
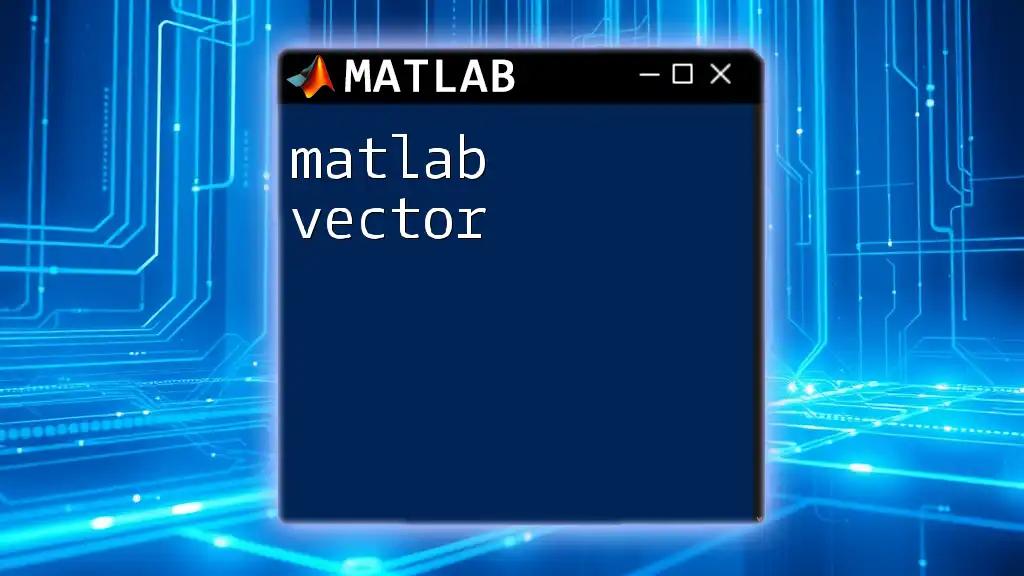
Practical Applications of Dictionaries in MATLAB
Data Organization
As projects grow in complexity, organizing data becomes crucial. A dictionary’s ability to map keys to values efficiently allows for the effective organization of related data. For example, a dictionary can hold configuration settings for an application, mapping each setting name to its value.
Mapping Data for Machine Learning
Dictionaries can be particularly useful in machine learning applications, where features are often mapped against values representing their importance or categorization.
Real-World Example
Consider a simple contact list application that can be implemented using a MATLAB dictionary. You could store names as keys and email addresses as values:
% Example code for a contact list
contacts = containers.Map();
contacts('John Doe') = 'john@example.com';
contacts('Jane Smith') = 'jane@example.com';
With this structure, retrieving or updating contact information becomes a seamless process.
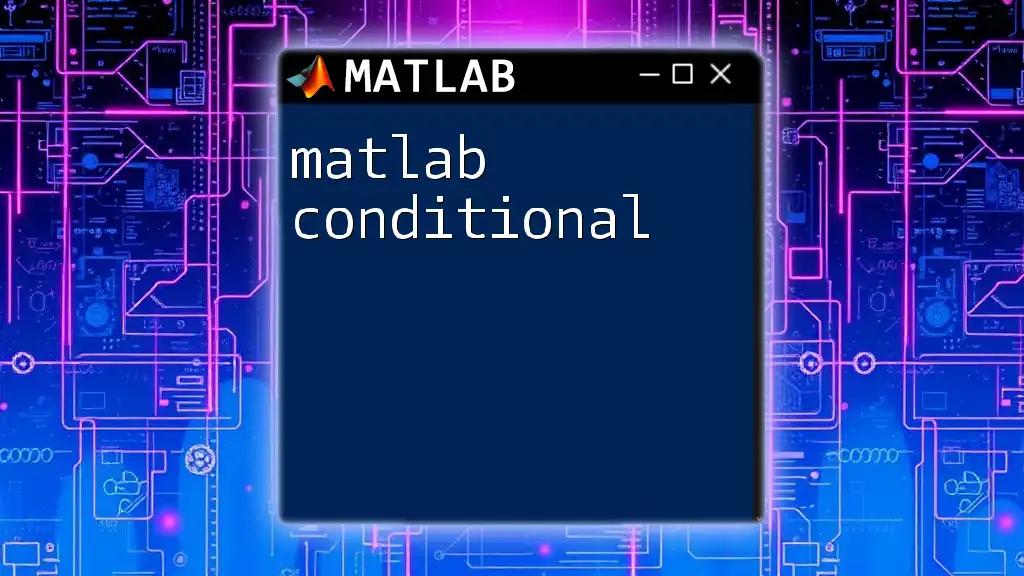
Conclusion
In summary, the MATLAB dictionary is a powerful data structure that allows users to store and manage data efficiently using key-value pairs. Its functionality—including creation, retrieval, updating, and deletion of entries—enables intuitive and organized programming practices. By practicing with dictionaries, you can enhance your data management skills in MATLAB, making your programming tasks easier and more effective.
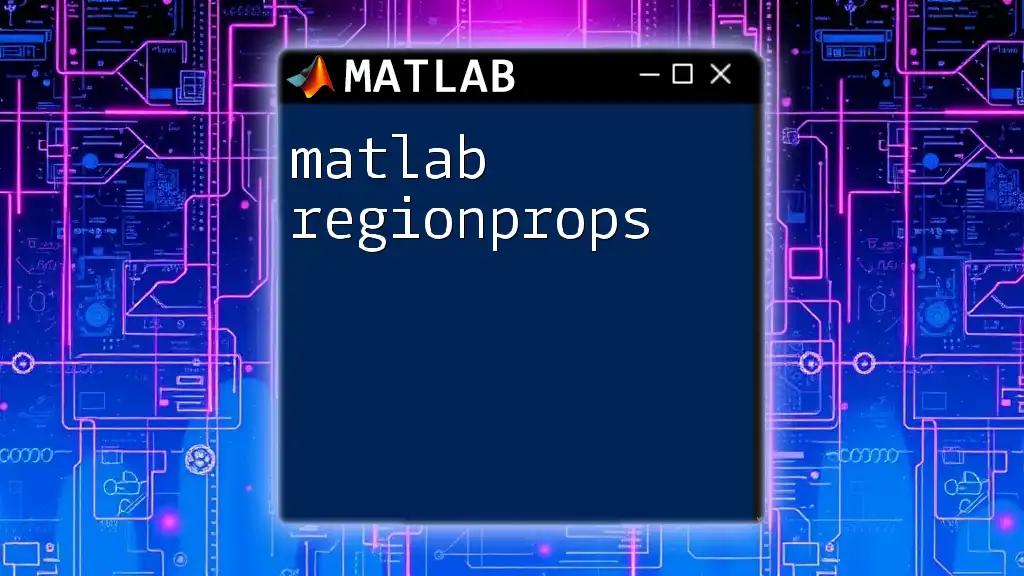
Additional Resources
For those looking to expand their knowledge further, explore MATLAB’s official documentation on `containers.Map` for comprehensive details. Additionally, numerous online tutorials and courses can provide deeper insights into using dictionaries effectively in MATLAB programming.