MATLAB vectorization is the process of rewriting code to use vector and matrix operations instead of loops, which enhances performance and efficiency in computations.
% Example of vectorization: Calculate the square of each element in an array
% Original code using a loop
A = [1, 2, 3, 4, 5];
B = zeros(size(A));
for i = 1:length(A)
B(i) = A(i)^2;
end
% Vectorized code
B = A.^2;
What is Vectorization?
In MATLAB, vectorization refers to the process of converting operations that would normally use loops into operations that work on whole arrays or matrices at once. This approach capitalizes on MATLAB’s strengths in handling matrix computations directly, leading to more efficient and faster code execution.
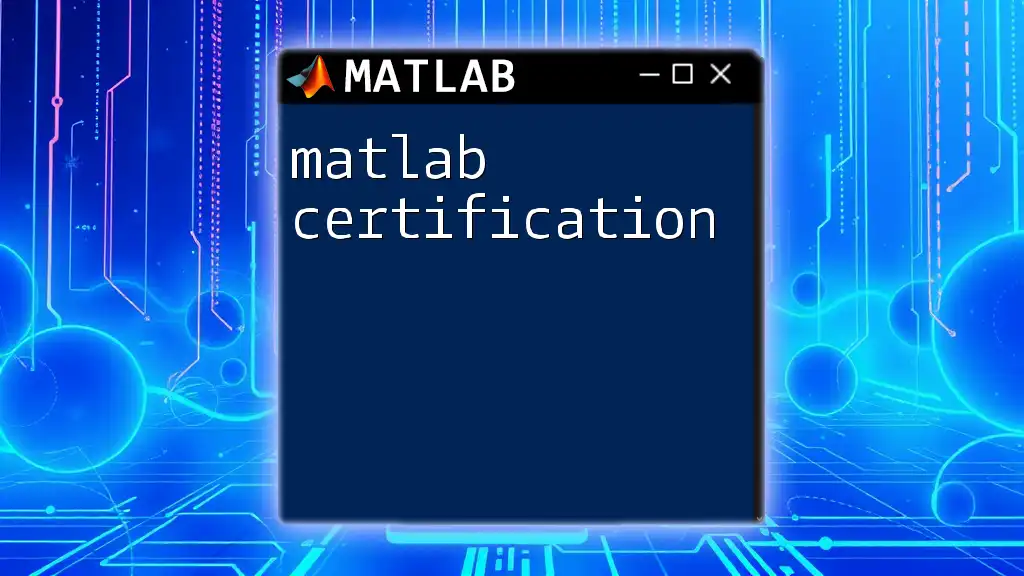
Why Use Vectorization?
Vectorization is crucial for optimizing performance in MATLAB. When you replace loops with vectorized operations, you can achieve significant speed improvements, especially with large datasets. For example, performing a calculation on a vector of one million elements using a for loop might take several seconds, whereas a vectorized version can complete the same task in milliseconds. Performance improvements can be crucial when working on large-scale data analytics or complex simulations.
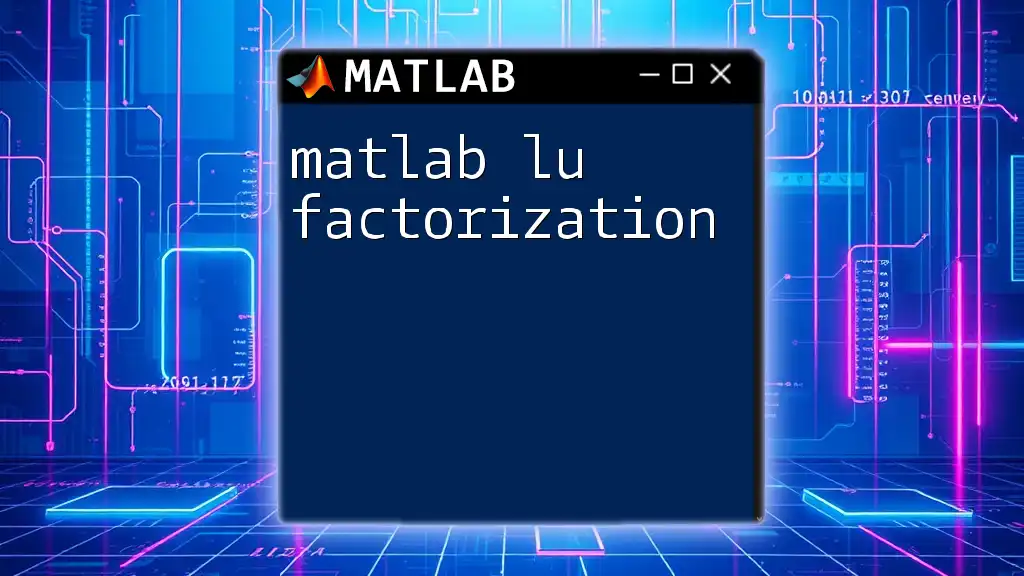
Understanding MATLAB Arrays
Types of Arrays in MATLAB
Row and Column Vectors
- Row Vectors are 1×N arrays.
- Column Vectors are Nx1 arrays.
For example:
row_vector = [1, 2, 3, 4, 5]; % Row vector
column_vector = [1; 2; 3; 4; 5]; % Column vector
Matrices
A matrix is a two-dimensional array. Here is an example:
matrix = [1, 2, 3;
4, 5, 6;
7, 8, 9];
Creating MATLAB Arrays
MATLAB provides several built-in functions for creating arrays. For instance:
zeros_array = zeros(3, 4); % 3x4 array filled with zeros
ones_array = ones(2, 5); % 2x5 array filled with ones
rand_array = rand(5); % 5x5 matrix with random values
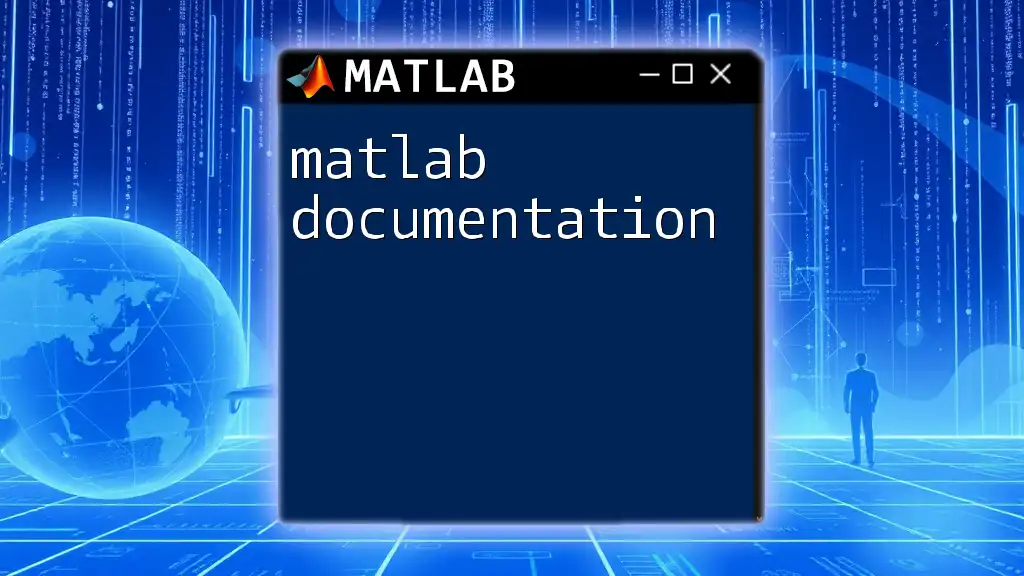
The Basics of Vectorized Operations
Element-wise Operations
Element-wise operations allow for calculations to be performed on each element of an array individually. In MATLAB, you can use operators with a dot (.) prefix to perform these operations.
For example:
A = [1, 2, 3];
B = [4, 5, 6];
C = A + B; % Result: [5, 7, 9]
D = A .* B; % Result: [4, 10, 18]
Logical Indexing
Logical indexing allows you to manipulate arrays based on conditions. For example, you can select elements greater than a certain value:
A = [1, 2, 3, 4, 5];
B = A(A > 3); % Result: [4, 5]
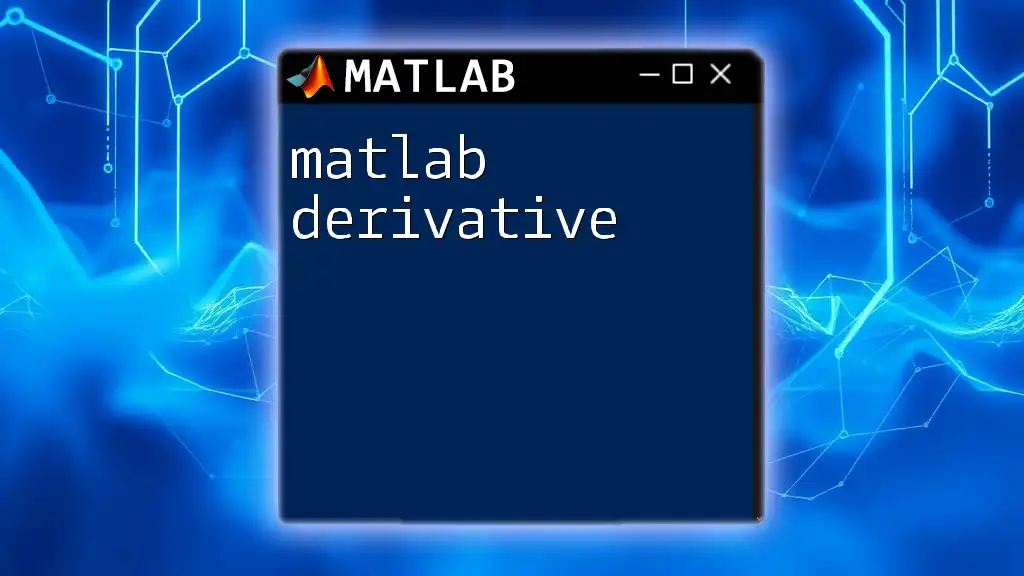
Transforming For Loops into Vectorized Code
Understanding For Loops in MATLAB
For loops are commonly used for iterative computations, but they can be slow. For instance, consider the following code that calculates the square of the first five integers:
result = zeros(1, 5);
for i = 1:5
result(i) = i^2;
end
Replacing For Loops with Vectorized Solutions
This can be converted to a vectorized form as follows:
result = (1:5).^2; % Result: [1, 4, 9, 16, 25]
This vectorized approach is not only shorter but performs better.
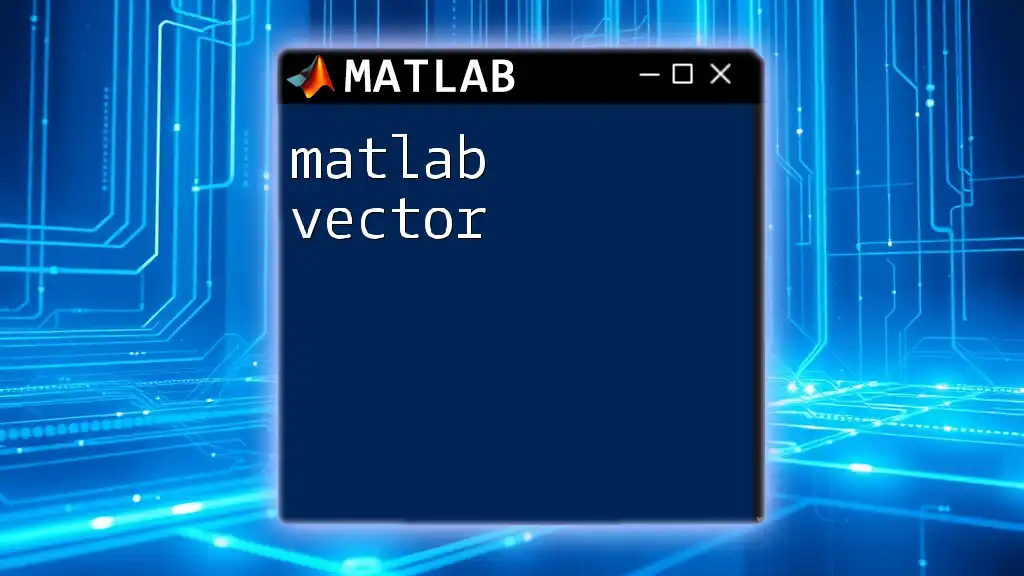
Advanced Vectorization Techniques
Vectorized Functions
MATLAB has built-in functions that are already vectorized for speed. Consider the following example, which computes the sum and mean of an array:
data = [1, 2, 3, 4, 5];
total = sum(data); % Result: 15
average = mean(data); % Result: 3
Arrayfun vs. Vectorization
The `arrayfun` function applies a function element-wise but can be slower than pure vectorization. For example:
data = [1, 2, 3];
result = arrayfun(@(x) x^2, data); % Each element squared
While this works, the vectorized approach remains preferred for better performance:
result = data.^2;
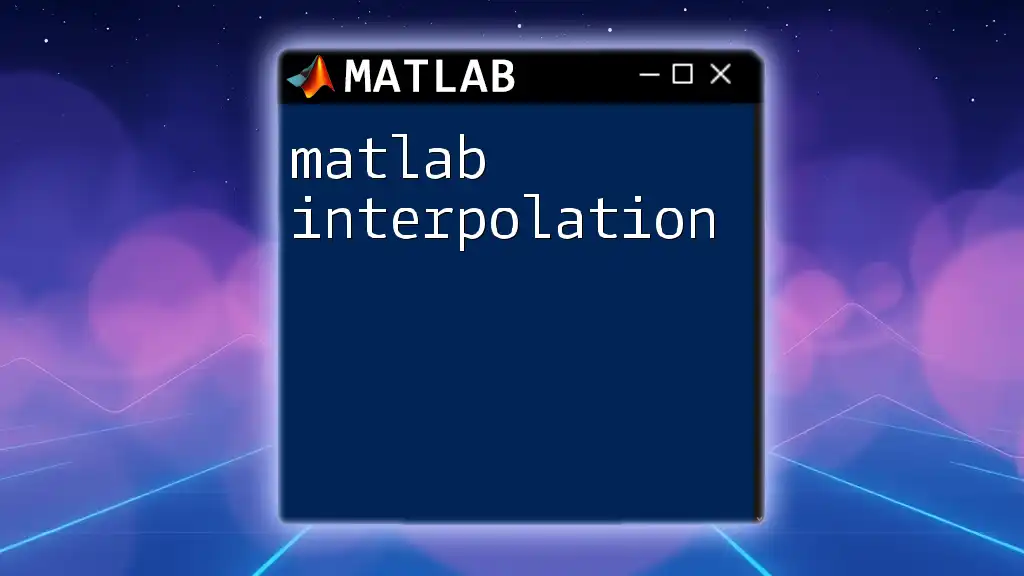
Practical Examples of Vectorization
Statistical Analysis
You can perform statistical analyses quickly using vectorization. Here’s a calculation of the mean, median, and standard deviation of an array:
data = [1, 2, 3, 4, 5];
mean_value = mean(data); % Result: 3
median_value = median(data); % Result: 3
std_dev = std(data); % Result: Standard Deviation
Mathematical Transformations
Vectorization is essential in performing matrix operations:
A = [1, 2; 3, 4];
B = A'; % Transpose of A
C = inv(A); % Inverse of A (if A is square and non-singular)
For solving linear equations, you can use:
% Ax = b
A = [2, 1; 1, 3];
b = [1; 2];
x = A\b; % Solution to the equation
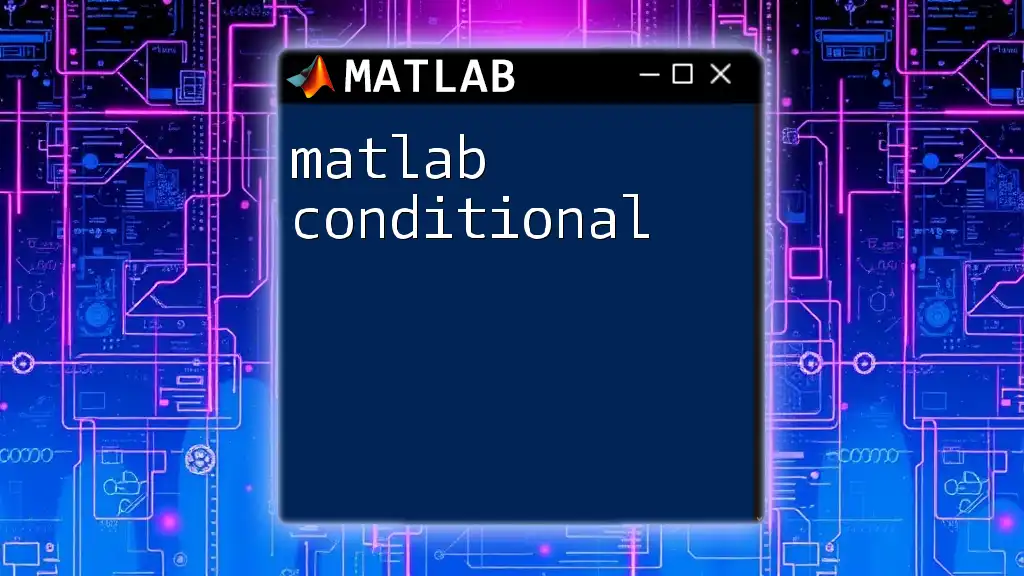
Best Practices for Vectorization
When to Avoid Vectorization
Despite the many advantages of vectorization, there are scenarios where it may not be beneficial. For example, if the operation includes conditionals that cannot be easily applied to the whole array, a loop may be preferred. Large datasets with heavy conditional checks might also slow down if vectorized.
Optimizing Vectorized Code
To achieve the best performance, ensure that the vectorized expressions are simplified and avoid unnecessary computations. For instance, caching results where feasible can improve efficiency:
A = rand(1, 1e6);
result = A(A > 0.5) .* 2; % Vectorized expression
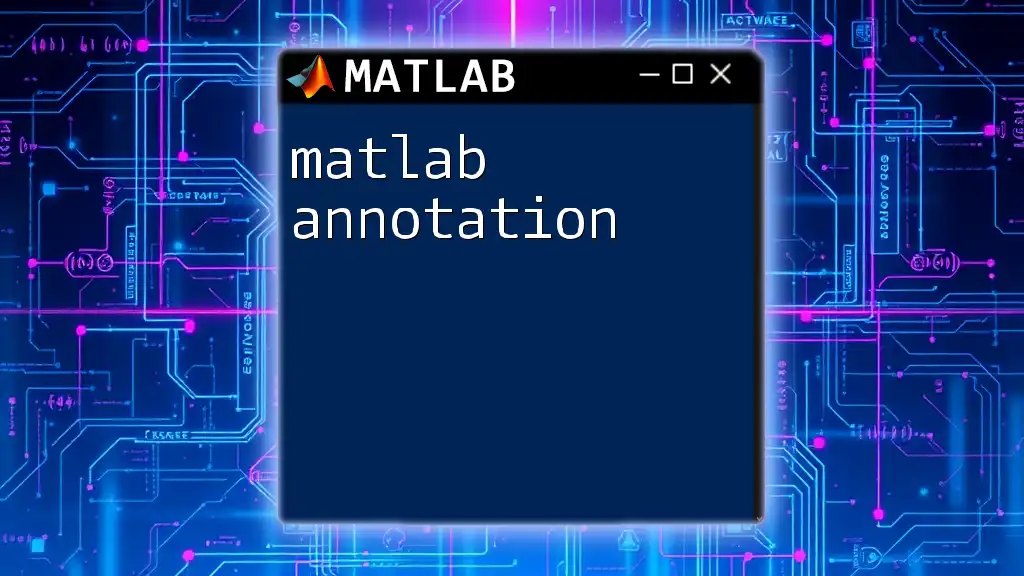
Conclusion
Understanding MATLAB vectorization is crucial for anyone aiming to write efficient and fast code. By embracing vectorized operations, you can streamline calculations and significantly enhance performance. The techniques discussed in this guide can be applied to your projects, providing a solid foundation for tackling complex problems effectively.
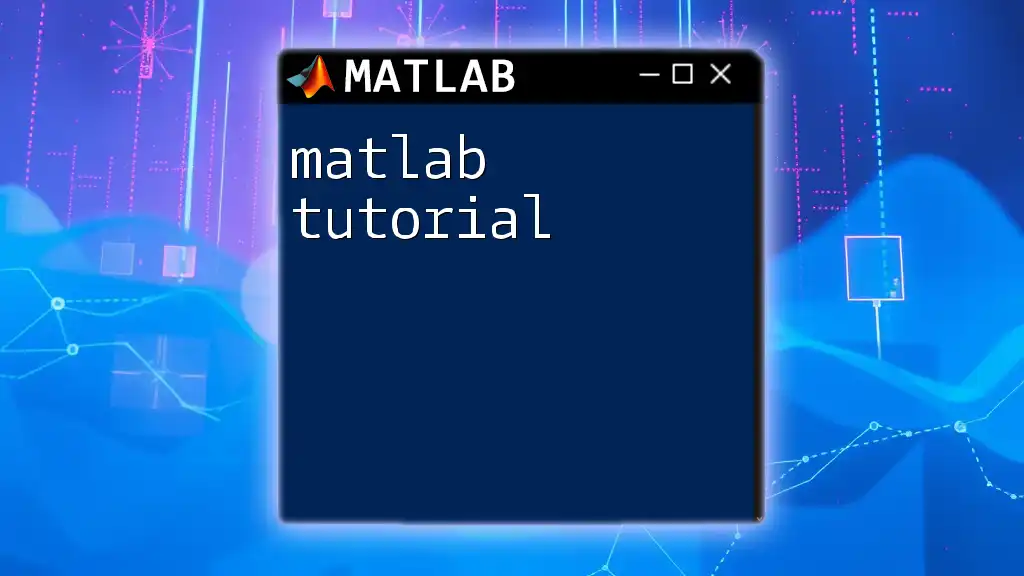
Next Steps
Practice these vectorization techniques in your coding endeavors. Experiment with vectorized transformations in your analyses and simulations, and push the boundaries of your MATLAB skills. For further learning, explore the official MATLAB documentation and consider resources that delve deeper into advanced MATLAB programming and optimization techniques.