A MATLAB rotation matrix is a mathematical construct used to rotate points in a two-dimensional or three-dimensional space about an origin or an axis, and here’s a simple example of creating a 2D rotation matrix for an angle θ:
theta = pi/4; % 45 degrees
R = [cos(theta) -sin(theta); sin(theta) cos(theta)];
What is a Rotation Matrix?
A rotation matrix is a mathematical tool used to perform rotations in a coordinate space. It transforms vectors or points to perform a clockwise or counterclockwise rotation around a fixed point or axis. The primary concept here is the use of matrices to represent geometric transformations effectively.
Mathematical Representation
The rotation of points in space can be represented mathematically through rotation matrices. In 2D spaces, the rotation by an angle \( \theta \) is represented as follows:
R2D = [cos(theta), -sin(theta);
sin(theta), cos(theta)];
In 3D, the representation becomes more complex, as it involves rotation about three different axes: the X-axis, Y-axis, and Z-axis.
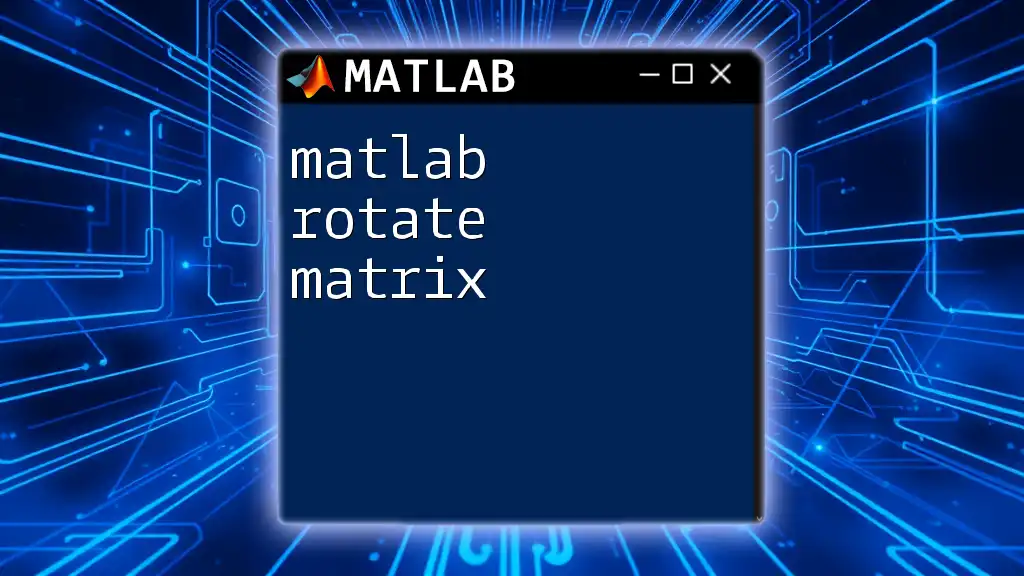
Types of Rotation Matrices
2D Rotation Matrix
In 2D space, the rotation matrix is quite straightforward. The rotation matrix for rotating points around the origin (0,0) by an angle \( \theta \) is defined as:
R2D = [cos(theta), -sin(theta);
sin(theta), cos(theta)];
Here, the values cos(theta) and sin(theta) are computed using the angle in radians. This transformation allows for rotating any point \( (x, y) \) by the specified angle.
3D Rotation Matrices
3D rotation matrices extend the concept of rotation beyond the XY-plane, enabling rotations around three axes.
Rotation about the X-axis
The rotation matrix for a rotation around the X-axis by an angle \( \theta \) is given by:
Rx = [1, 0, 0;
0, cos(theta), -sin(theta);
0, sin(theta), cos(theta)];
This matrix is crucial in simulations and robotics, where it represents the orientation of objects in three-dimensional space.
Rotation about the Y-axis
Similarly, for rotation around the Y-axis, the matrix is represented as:
Ry = [cos(theta), 0, sin(theta);
0, 1, 0;
-sin(theta), 0, cos(theta)];
This format is particularly useful in computer graphics, where adjusting the viewpoint or reorienting models requires such transformations.
Rotation about the Z-axis
Lastly, the rotation matrix for the Z-axis is defined as follows:
Rz = [cos(theta), -sin(theta), 0;
sin(theta), cos(theta), 0;
0, 0, 1];
This rotation is often used in 3D modeling applications to implement camera transformations and animations.
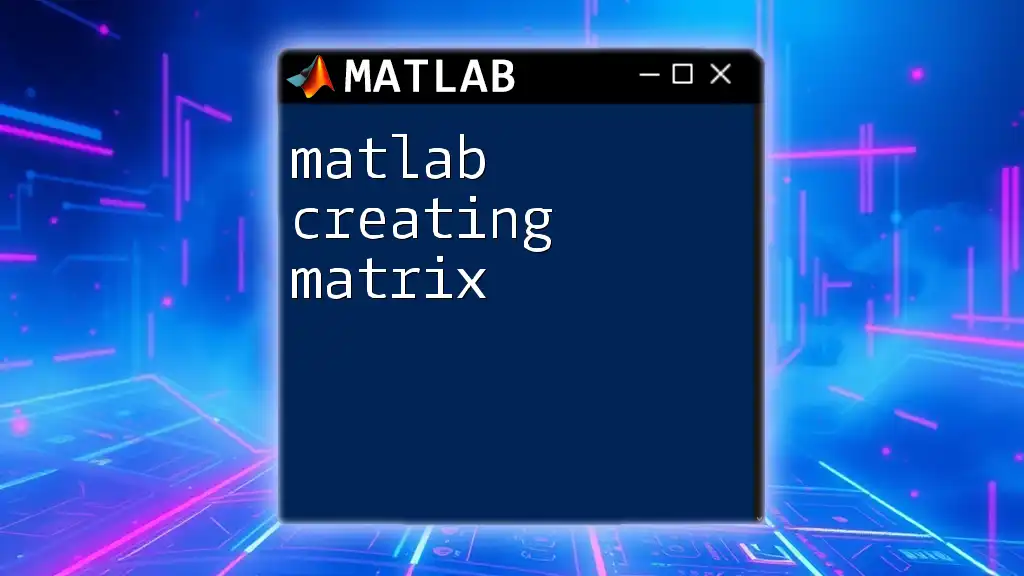
Creating and Using Rotation Matrices in MATLAB
To work with rotation matrices in MATLAB, you can utilize simple commands to define and manipulate these matrices.
Basic Commands for Rotation Matrices
Define a rotation matrix in MATLAB by specifying an angle \( \theta \). For example, to create a matrix for a rotation of 45 degrees (converted to radians):
theta = pi/4; % Example angle in radians
R = [cos(theta), -sin(theta);
sin(theta), cos(theta)];
This code snippet allows you to store the rotation matrix \( R \) for later use in transforming points.
Applying Rotation Matrices to Points
2D Point Rotation
After defining your rotation matrix, applying it to a point is straightforward. Consider a point at \( (1, 0) \):
point = [1; 0];
rotated_point = R * point;
The result, `rotated_point`, gives the new coordinates after the rotation. Here, the output reflects how the original point has been transformed according to the rotation defined.
3D Point Rotation
For 3D points, the process is similar but uses the corresponding matrix. For instance, if you have a point \( (1, 1, 1) \) and you want to rotate it around the Z-axis:
point3D = [1; 1; 1];
rotated_point3D = Rz * point3D; % Example using Z-axis rotation
This transformation will output the new location of `point3D` after rotation, adapting its position based on the specified angle and axis of rotation.
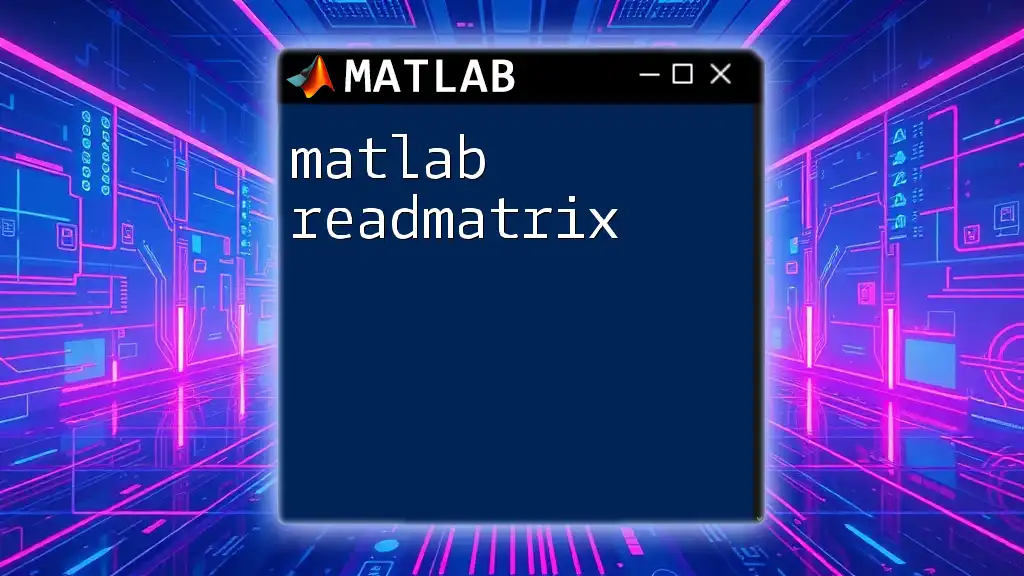
Visualizing Rotation Matrices
Plotting Rotated Points
A critical part of learning to use rotation matrices effectively involves visual representation. Plotting both original and rotated points helps illustrate the impact of these transformations.
To plot a 2D rotation, you can use MATLAB's built-in plotting functions:
figure;
hold on;
plot(point(1), point(2), 'ro'); % Original Point
plot(rotated_point(1), rotated_point(2), 'bo'); % Rotated Point
title('2D Rotation of a Point');
legend('Original Point', 'Rotated Point');
axis equal;
This code generates a visual where you can clearly see the original and rotated points, reinforcing your understanding of how rotation affects position.
Animation of Rotations
Creating animations of rotations offers additional insights into the behavior of rotation matrices.
This example demonstrates a continuous rotation visualization, cycling through angles from 0 to \( 2\pi \):
for t = 0:0.1:2*pi
R = [cos(t), -sin(t); sin(t), cos(t)];
rotated_point = R * point;
plot(rotated_point(1), rotated_point(2), 'bo');
pause(0.1); % Delay for visualization
end
This loop modifies the angle applied to the rotation matrix and displays the rotated point, effectively creating an animated effect.
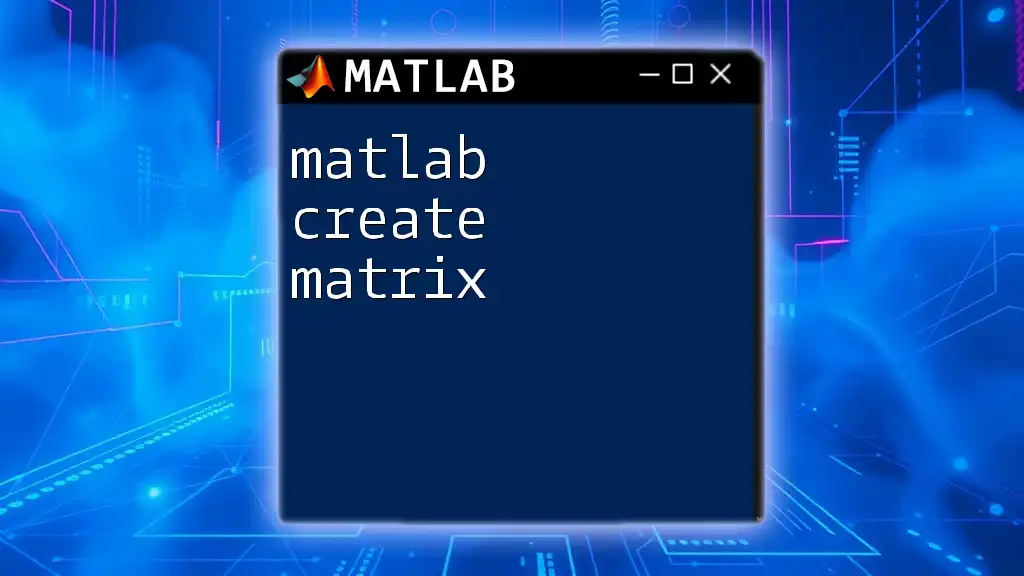
Practical Applications of Rotation Matrices
In Robotics
Rotation matrices are integral in robotics, particularly in motion planning and control systems. They help define the orientation of robotic arms and any objects they manipulate, ensuring precise movements within various spatial dimensions.
In Computer Graphics
In computer graphics, rotation matrices are pivotal for rendering scenes accurately. They allow for transformations of objects in three-dimensional space, enabling realistic animations and perspective adjustments.
In Attitude Control Systems
Attitude control in aerospace emphasizes the use of rotation matrices. These matrices manage the orientation of spacecraft and drones, facilitating highly accurate responses to orientation changes and movements in various flight scenarios.
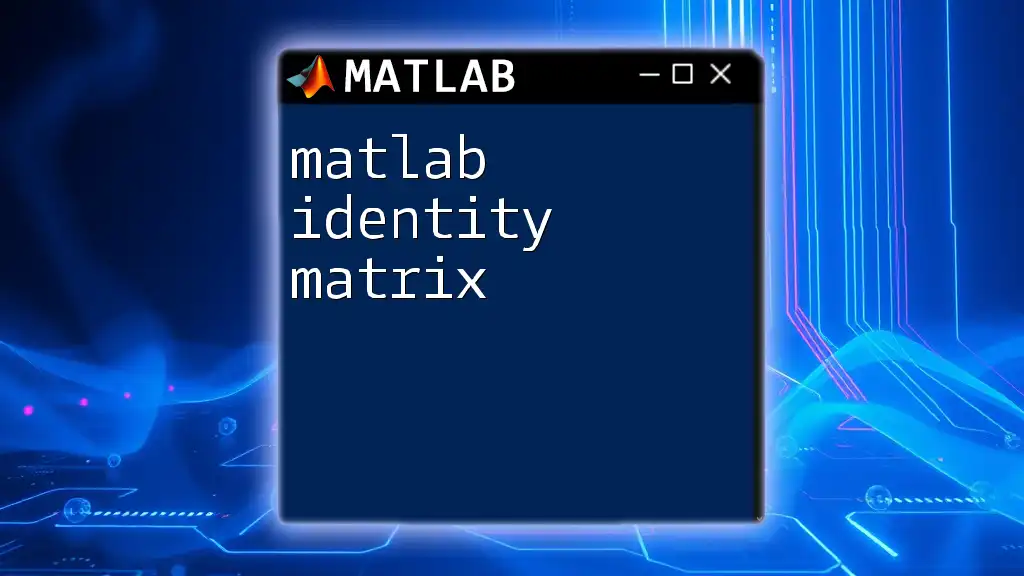
Conclusion
Understanding the concept of a MATLAB rotation matrix opens up a multitude of possibilities across various disciplines such as robotics, computer graphics, and aerospace. By mastering how to create and apply these matrices, you can significantly enhance your ability to manipulate spatial representations in MATLAB. Experiment with the code snippets and visualization techniques provided, and you’ll soon appreciate the power and utility of rotation matrices in real-world applications.
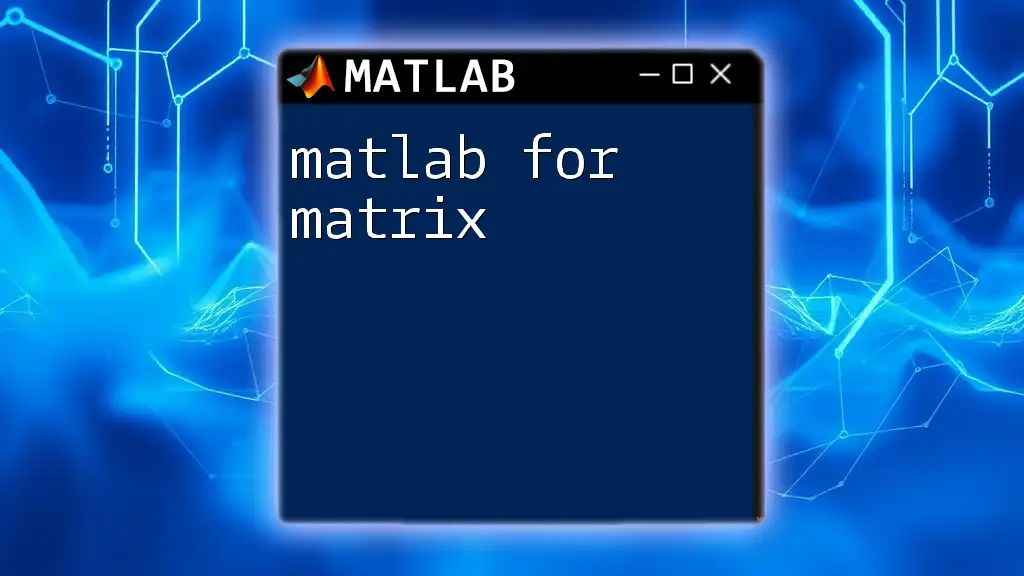
Additional Resources
For an enriched learning experience, refer to the official MATLAB documentation on matrices and transformations. Dive deeper into books or online articles specialized in 3D transformations and the geometric algebra that supports these operations.
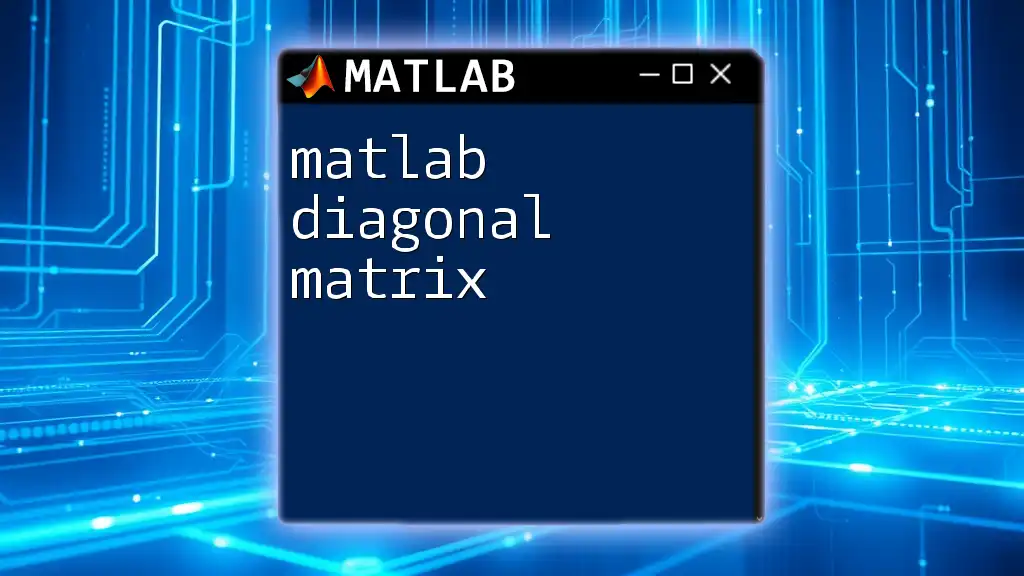
FAQs
Common Questions About Rotation Matrices in MATLAB
-
What is the significance of using radians vs. degrees? Radians provide a natural measure for angles in MATLAB, aligning closely with the mathematical definitions used in trigonometric functions.
-
How do I interpolate between two rotation matrices? This often involves using quaternion representations or spherical linear interpolation (slerp) techniques.
-
Can I combine multiple rotations into a single matrix? Yes, by multiplying the corresponding rotation matrices in the correct order, you can achieve a combined rotation effect on your points or objects.