The smoothness of a sequence in MATLAB refers to the reduction of noise or fluctuations in data, often achieved using filtering techniques or smoothing functions.
Here’s a simple example using the `smoothdata` function to smooth a noisy sequence:
% Generate a noisy sequence
x = 0:0.1:10;
y = sin(x) + 0.5*randn(size(x));
% Smooth the data using a moving mean method
smoothed_y = smoothdata(y, 'movmean', 5);
% Plotting the original and smoothed data
figure;
plot(x, y, 'r.', 'DisplayName', 'Noisy Data'); hold on;
plot(x, smoothed_y, 'b-', 'DisplayName', 'Smoothed Data');
legend show;
title('Smoothness of Sequence in MATLAB');
xlabel('X-axis'); ylabel('Y-axis');
This code snippet first generates a noisy sine wave, then applies a moving average smoothing technique, and finally visualizes both the noisy and smoothed data.
Key Concepts in MATLAB Smoothness
What is a Sequence?
A sequence in the context of MATLAB refers to an ordered list of numbers, which can be generated through various mathematical expressions or data collection methods. Sequences are fundamental in numerical analysis and are often used to represent data points in graphical forms, computations, and simulations.
For instance, a simple numerical sequence could be the result of sampling a function at specific intervals, such as:
x = 0:0.1:10; % Define the domain
y = sin(x); % A sequence based on the sine function
This creates a sequence of `y` values corresponding to `x`, which can be analyzed or manipulated using MATLAB functions.
What Does Smoothness Mean?
Smoothness can be defined as the quality of a sequence where adjacent points do not display abrupt changes, thus providing a visually appealing and analytically useful representation of data. Characteristics of a smooth sequence include continuity and a differentiable nature, which helps in depicting trends without noise or fluctuations that can mislead interpretations.
To understand smoothness, consider the graphical representation of a sinusoidal function such as sine or cosine. These functions are inherently smooth. In contrast, noisy data obtained from sensors or experiments may contain irregularities that obscure underlying trends.
By making sequences smooth, we enhance our ability to discern patterns that are significant in data analysis.
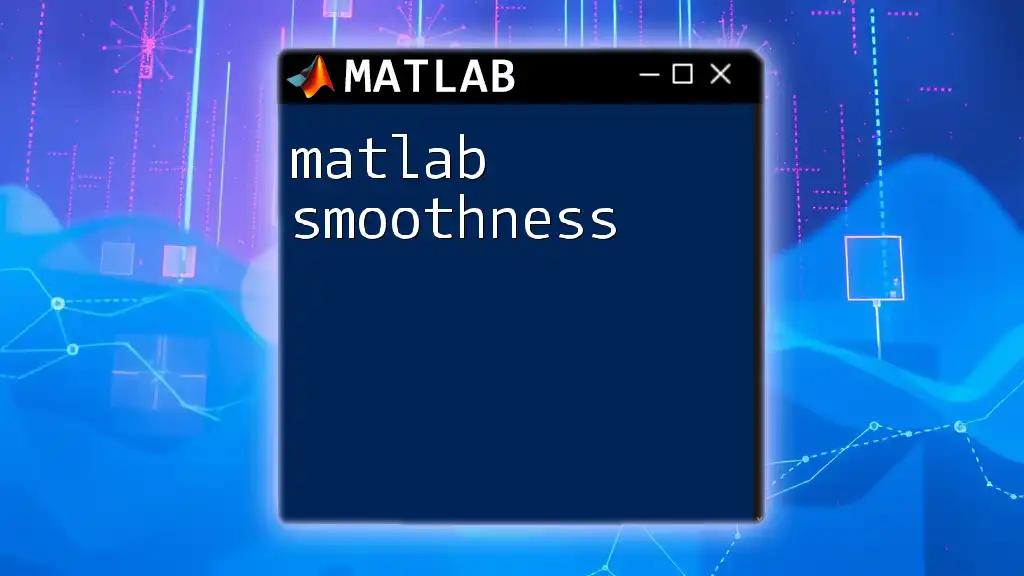
Methods to Achieve Smoothness in MATLAB
Applying Moving Average
The moving average is a straightforward technique used to smooth a sequence by averaging a defined number of surrounding data points. This method is particularly useful when attempting to reduce noise.
For example, if you have a noisy sequence generated randomly, you can apply a moving average using the following MATLAB code:
data = randn(1,100); % Generate random data
windowSize = 5; % Define window size for moving average
smoothData = movmean(data, windowSize); % Apply moving average
plot(data); hold on; plot(smoothData, 'r'); % Plot original and smooth data
title('Moving Average Smoothness');
legend('Original Data','Smooth Data');
In this example, the original data is plotted along with the smoothed data. By adjusting the `windowSize`, you can explore how the smoothness changes, providing insights into the balance between retention of features and noise reduction.
Savitzky-Golay Filter
The Savitzky-Golay filter is another popular smoothing technique that fits successive subsets of adjacent data points with a polynomial of a specified degree. This method preserves features of the data, such as relative maxima and minima, unlike simpler methods which may distort these characteristics.
To implement a Savitzky-Golay filter in MATLAB, use the following code:
data = randn(1,100); % Generate random data
smoothData = sgolayfilt(data, 3, 11); % Apply Savitzky-Golay filter
plot(data); hold on; plot(smoothData, 'g'); % Plot original and filtered data
title('Savitzky-Golay Filter Smoothness');
legend('Original Data','Filtered Data');
The degree of the polynomial and the frame size need to be chosen wisely. This flexibility allows you to adjust the resulting smoothness based on your data type and analysis requirements.
Interpolation Techniques
Interpolation methods are used not only for estimating unknown values between known data points but also for smoothing sequences. Linear interpolation is one of the simplest techniques and can provide a quick smoothing effect.
Here’s an example of linear interpolation in MATLAB:
x = 1:10; % Original data points
y = rand(1,10); % Random values
xq = 1:0.1:10; % Query points for interpolation
smoothData = interp1(x, y, xq, 'linear'); % Linear interpolation
plot(x, y, 'o', xq, smoothData, '-'); % Original and interpolated data
title('Linear Interpolation for Smoothness');
In this code, the `interp1` function fills in the gaps between original data points, producing a smoother appearance. Different interpolation methods, such as cubic or spline interpolation, can be tested to find the best fit for your specific use case.

Practical Applications of Smoothness in Data Analysis
Analyzing Experimental Data
In experimental science, achieving smoothness in data is crucial to deriving meaningful conclusions. Raw experimental data often contains noise due to external factors, and applying smoothing techniques like moving averages or Savitzky-Golay filters can enhance the clarity of trends.
For instance, you might analyze a sequence of temperature measurements over time which fluctuates slightly. By applying a smoothing technique, you can better visualize temperature trends and identify critical inflection points.
Time Series Analysis
Time series data, which consists of observations collected over time, also greatly benefits from smoothing methods. For example, stock market prices can be extremely volatile. By applying smoothing techniques, analysts can observe underlying trends and make more informed predictions about future movements.
Here's a simplified illustration using a moving average for time series analysis:
time = 1:100;
data = sin(time/10) + randn(1,100)*0.1; % Simulate noisy sinusoidal data
windowSize = 10;
smoothData = movmean(data, windowSize); % Smooth the data
plot(time, data, 'k'); hold on; plot(time, smoothData, 'b'); % Plot both
title('Time Series Smoothing Example');
legend('Original Data','Smoothed Data');
This type of analysis enables clearer insights and strategic decision-making based on prolonged trends rather than short-term fluctuations.
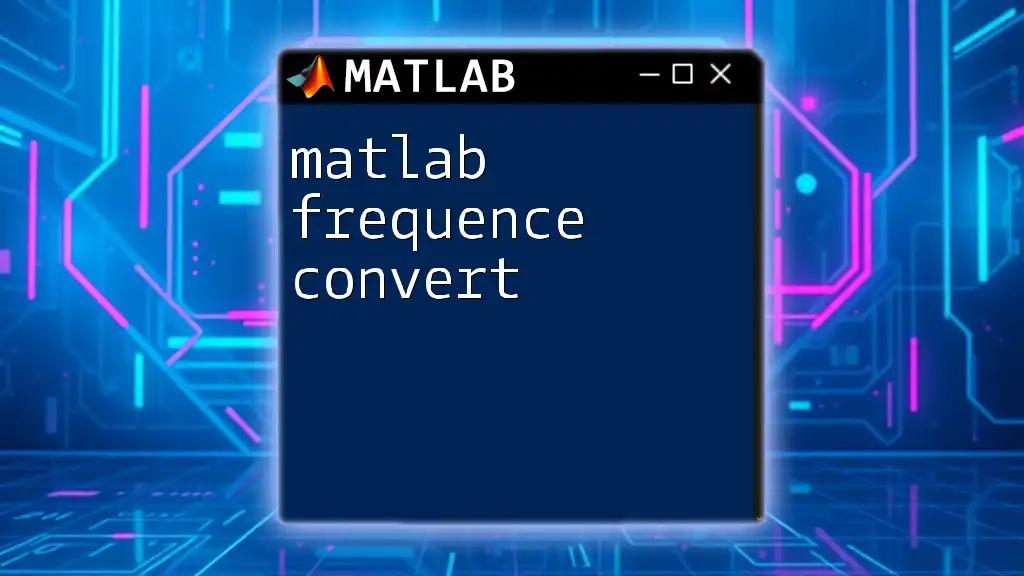
Best Practices for Smoothness in MATLAB
Choosing the Right Smoothing Method
Selecting the appropriate smoothing method depends on various factors, including the nature of your data, the level of noise present, and the specific features of interest. For instance, if you need to maintain peak values in a dataset, the Savitzky-Golay filter may be preferred, whereas for noisy datasets where trends are important, a moving average might suffice.
Performance Considerations
When working with large datasets, consider the computational efficiency of the smoothing methods. Some techniques may introduce delays or require extensive processing time. Evaluating the size, type of data, and required smoothness should guide your choice of method to ensure a balance between accuracy and performance.
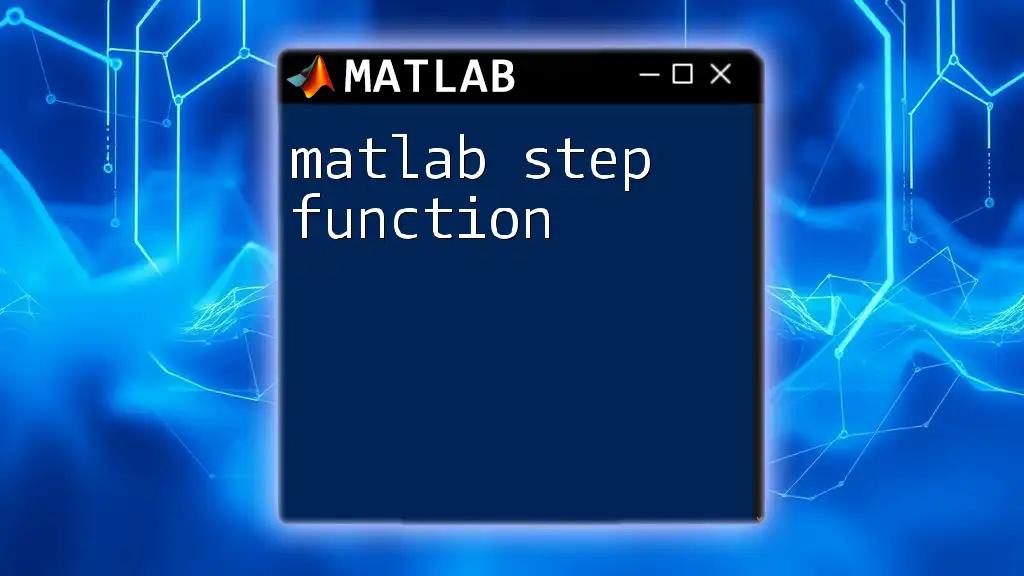
Conclusion
Achieving smoothness in sequences is a vital aspect of data analysis in MATLAB, providing clearer insights and enabling more informed decisions across various fields. Armed with knowledge of different smoothing techniques like moving averages, the Savitzky-Golay filter, and interpolation methods, you can effectively mitigate noise and enhance the clarity of your analyses.
By following the practices outlined in this guide, you can apply these methods proficiently in your projects, ensuring that your data presentation is both informative and visually appealing. Explore further learning resources to deepen your understanding of MATLAB’s capabilities and enhance your skills in data analysis.