The MATLAB `mod` function returns the remainder after division of one number by another, which is useful for various mathematical computations.
Here’s a code snippet demonstrating the usage of the `mod` function in MATLAB:
% Example: Calculate the remainder of 10 divided by 3
result = mod(10, 3);
disp(result); % Outputs: 1
Understanding the Basics of Modulus Operation
What is the Modulus Operation?
The modulus operation is a mathematical operation that finds the remainder after the division of one number by another. For example, when you divide 10 by 3, the quotient is 3, but the remainder is 1. Thus, \( 10 \mod 3 = 1 \).
This operation is widely applicable in many fields. It's used in computing to determine periodic behavior, in algorithms to handle circular structures, and in everyday mathematics where the concept of remainders is necessary.
How Modulus Translates to MATLAB
In MATLAB, the modulus operation is achieved through the `mod` function. This function serves the same purpose as the modulus operator in other programming languages such as C++ or Python, but with a straightforward syntax that is tailored for handling matrix and vector operations efficiently. The function consolidates the complexity of performing modulus operations on entire arrays with ease.
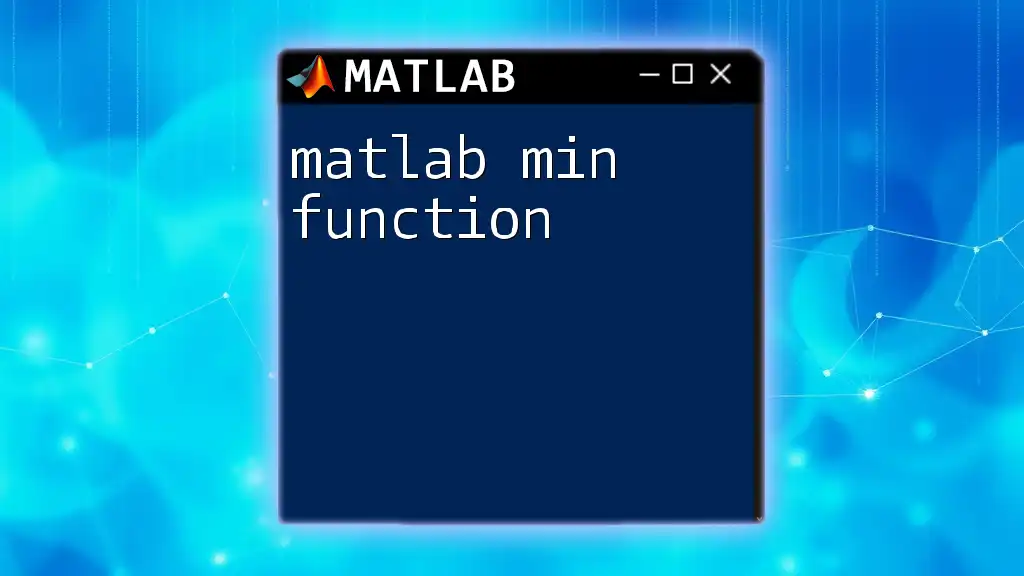
Syntax of the mod Function
Basic Syntax
The basic syntax for the `mod` function in MATLAB is:
mod(a, b)
Here, `a` is the dividend and `b` is the divisor. The function returns the modulus of `a` with respect to `b`. Both `a` and `b` can be scalars, vectors, or matrices, making this function versatile for various numerical contexts.
Return Value
The `mod` function returns the remainder after dividing `a` by `b`. If `a` and `b` are arrays, the operation applies element-wise, producing an array of the same size as `a`.
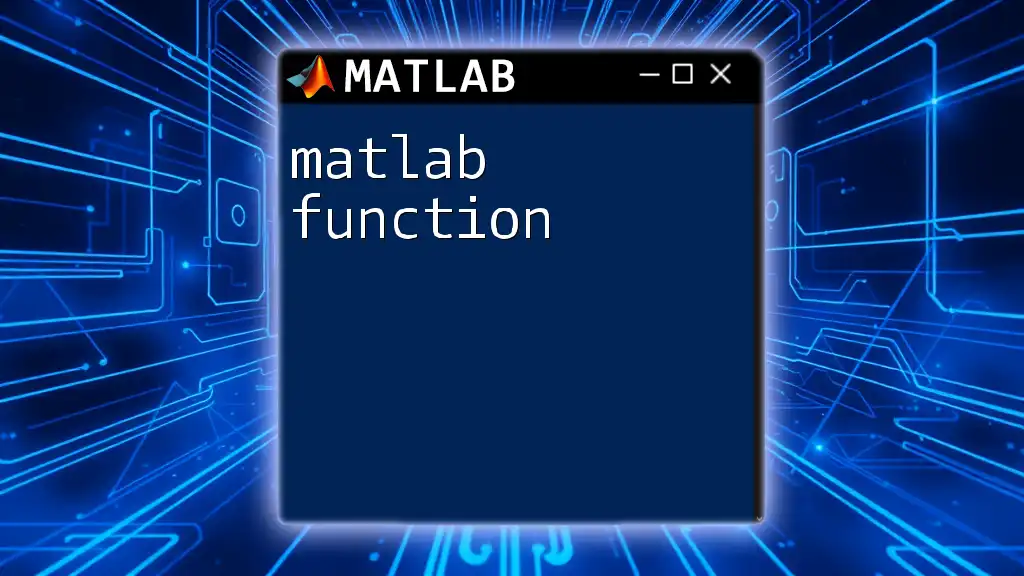
How to Use the mod Function
Single Value Modulus Operation
You can use the `mod` function for single numerical values effortlessly. For instance, when calculating:
result = mod(10, 3);
fprintf('The result is: %d\n', result); % Output: The result is: 1
This code snippet calculates the modulus of 10 and 3, where you will see that the result is 1. This is a simple and effective way to ascertain remainders directly in MATLAB.
Vectorized Modulus Operation
One of the powerful features of the MATLAB `mod` function is its ability to operate on arrays. When applying `mod` on an array:
array = [10, 20, 30, 40];
result = mod(array, 6);
disp(result); % Output: [4 2 0 4]
In this example, the function computes the remainder of each element in `array` divided by 6. The output will be an array where each element corresponds to the modulus of the respective input element, demonstrating MATLAB's ability to handle vectorized operations seamlessly.
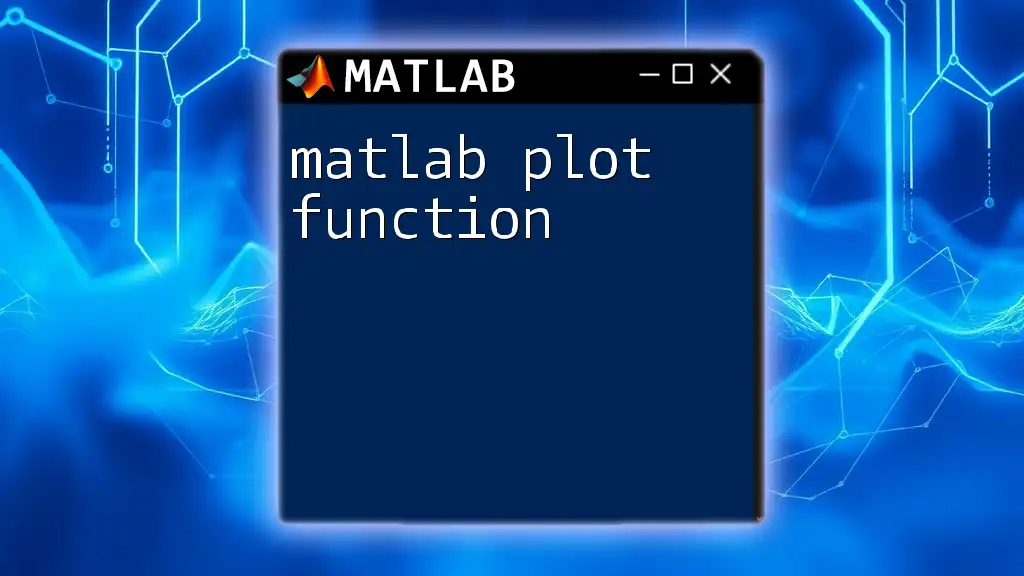
Practical Applications of Mod Function
Determining Even or Odd Numbers
A common practical application of the `mod` function is checking whether a number is even or odd. By employing the modulus with 2, you can easily identify odd and even numbers:
num = 15;
if mod(num, 2) == 0
disp('Even Number');
else
disp('Odd Number');
end
In this case, since `15 mod 2` equals 1, MATLAB will output "Odd Number". This simple conditional check is useful in various algorithms where the parity of a number is relevant.
Circular Indexing
Another compelling use case for the `mod` function is circular indexing, which allows for accessing elements in a repeated manner. For instance, consider a list of items:
list = {'A', 'B', 'C', 'D'};
index = 5; % trying to access the 5th index
circular_index = mod(index-1, numel(list)) + 1;
disp(['Element: ' list{circular_index}]); % Output: Element: A
In this example, since the list has only four elements, using `mod` allows the index to wrap around, resulting in accessing the first element ('A') despite the original index being 5. This functionality is particularly useful in programming scenarios, such as cycling through states or items repeatedly.
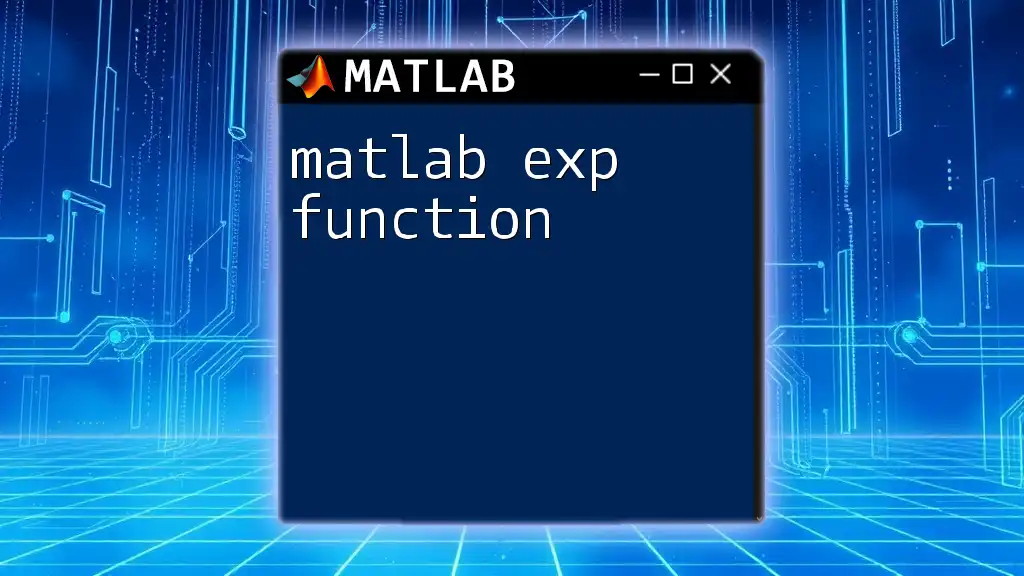
Advanced Features and Considerations
Handling Negative Numbers
One interesting aspect of the `mod` function in MATLAB is its behavior when dealing with negative numbers. For instance:
result = mod(-10, 3);
disp(result); % Output: 2
Here, `-10 mod 3` results in 2, which might seem counterintuitive. This behavior guarantees that the result of `mod` is always non-negative when the divisor is also positive. Understanding this is crucial, especially when performing mathematical computations that involve a mix of positive and negative integers.
Using the mod Function with Complex Numbers
The `mod` function can also handle complex numbers seamlessly. By applying it on the real part of a complex number, you can quickly obtain the modulus:
complexNum = 3 + 4i;
modResult = mod(real(complexNum), 2);
disp(modResult); % Output: 1
This example demonstrates how you can retrieve the modulus of the real component of a complex number. Understanding how to use `mod` with complex numbers expands its utility in advanced mathematical computations.
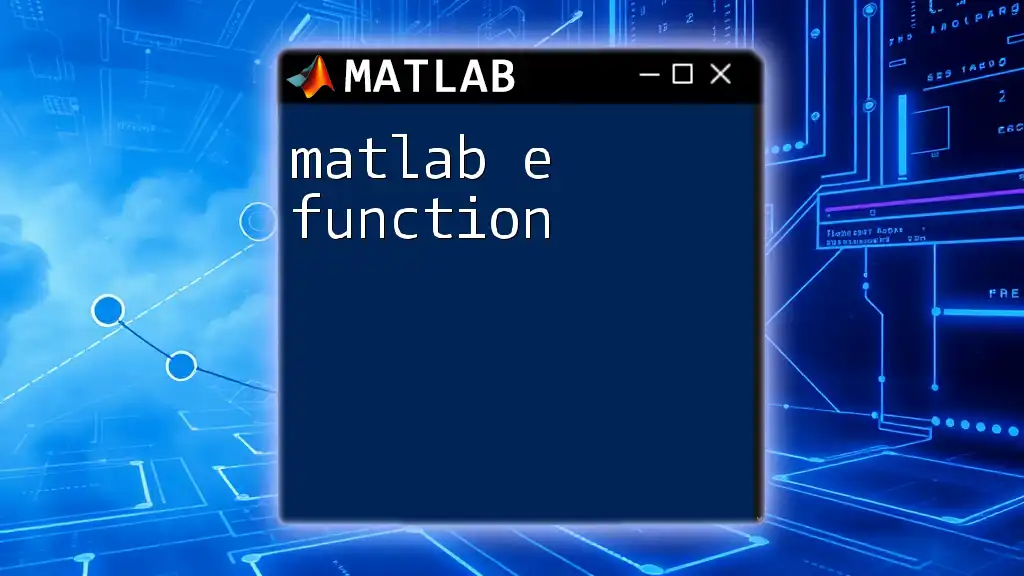
Conclusion
The MATLAB mod function is a powerful tool that simplifies calculations where modulus operations are needed. From determining even and odd numbers to enabling circular indexing and handling complex numbers, its applications are vast and diverse.
To become proficient in utilizing the `mod` function, regular practice is advisable, as it will strengthen your understanding and integration of this function into various programming scenarios.
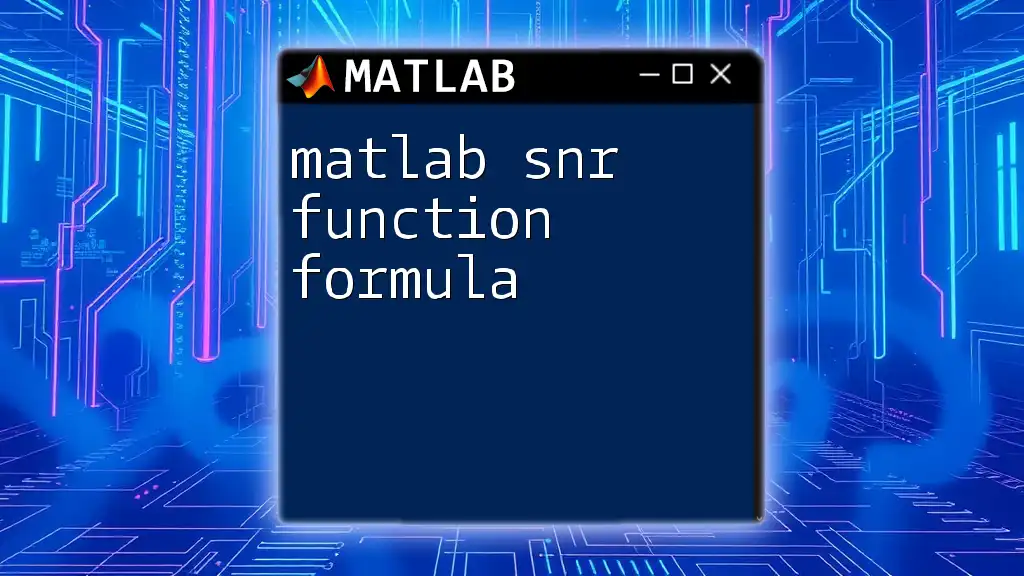
Additional Resources
Further Reading and Tutorials
For those looking to further their knowledge, the official MATLAB documentation offers detailed insights and examples on using the `mod` function effectively.
Community and Support
Engaging with the MATLAB community online, whether through forums or dedicated groups, provides additional support and learning resources, allowing for deeper dives into both the `mod` function and wider MATLAB capabilities.