Matlab smoothness refers to techniques for reducing noise in data to enhance visual representation or analytical accuracy, often achieved using the `smooth` function.
Here’s a simple code snippet demonstrating how to use the `smooth` function:
% Example Data
x = 1:10;
y = [10 12 13 8 5 10 7 15 20 25] + rand(1,10); % noisy data
% Smoothing the data using a moving average method
y_smooth = smooth(y, 3); % 3 is the span
% Plotting the results
figure;
plot(x, y, 'ro-', x, y_smooth, 'b-', 'LineWidth', 2);
legend('Noisy Data', 'Smoothed Data');
title('Data Smoothing Example');
xlabel('X');
ylabel('Y');
Understanding Smoothness in MATLAB
What is Smoothness?
In the context of data analysis and signal processing, smoothness refers to the degree to which a signal fluctuates. A smooth signal typically has fewer abrupt changes or noise, allowing for easier interpretation and analysis. Understanding and applying smoothness in your data can lead to more reliable insights, especially in fields like engineering, finance, and science, where data interpretation is crucial.
Key Concepts Relevant to Smoothness
When discussing smoothness, two key concepts emerge—noise and signal. Noise is the unwanted variability in a dataset that can obscure the desired signal. A critical distinction is made between local and global smoothness, with local smoothness focusing on small sections of data and global smoothness considering the dataset as a whole. Understanding these concepts can help you choose the right smoothing techniques.

MATLAB Functions for Data Smoothing
Built-in Smoothing Functions
MATLAB provides several built-in functions to facilitate data smoothing, allowing users to achieve desired smoothness levels quickly.
smooth() Function
The `smooth()` function in MATLAB is a versatile tool used for data smoothing. Its flexibility allows users to select various methods, including moving average, local regression (loess), and others.
Here's an example code snippet demonstrating the use of the `smooth()` function:
data = randn(1,100); % Generate random data
smoothedData = smooth(data, 'loess');
plot(data); hold on; plot(smoothedData, 'r-'); % Plotting the results
In this example, we generate a random set of data, apply the loess smoothing method, and plot both the raw and smoothed data. The loess method is particularly effective as it employs local regression to smoothen data without significantly altering its original form.
Moving Average Smoothing
The moving average technique is another prevalent method for smoothing data. This technique averages a fixed number of data points, providing a simplified view.
Here's an example of how to implement moving average smoothing in MATLAB:
windowSize = 5;
movingAverage = movmean(data, windowSize);
plot(data); hold on; plot(movingAverage, 'g-');
In this code, we define a window size and compute the moving average for the dataset. While moving averages can help eliminate noise, they can also oversimplify data, leading to potential loss of important details.
Savitzky-Golay Smoothing
The Savitzky-Golay filter is another powerful method known for preserving the features of the data while smoothing it. This method fits successive sub-sets of adjacent data points with a low-degree polynomial.
You can apply the Savitzky-Golay filter with the following code snippet:
sgFiltered = sgolayfilt(data, 3, 11);
plot(data); hold on; plot(sgFiltered, 'b-');
In this example, the `sgolayfilt` function applies the filter with a polynomial order of 3 and a frame length of 11. This technique is particularly beneficial for preserving maxima and minima in the data.
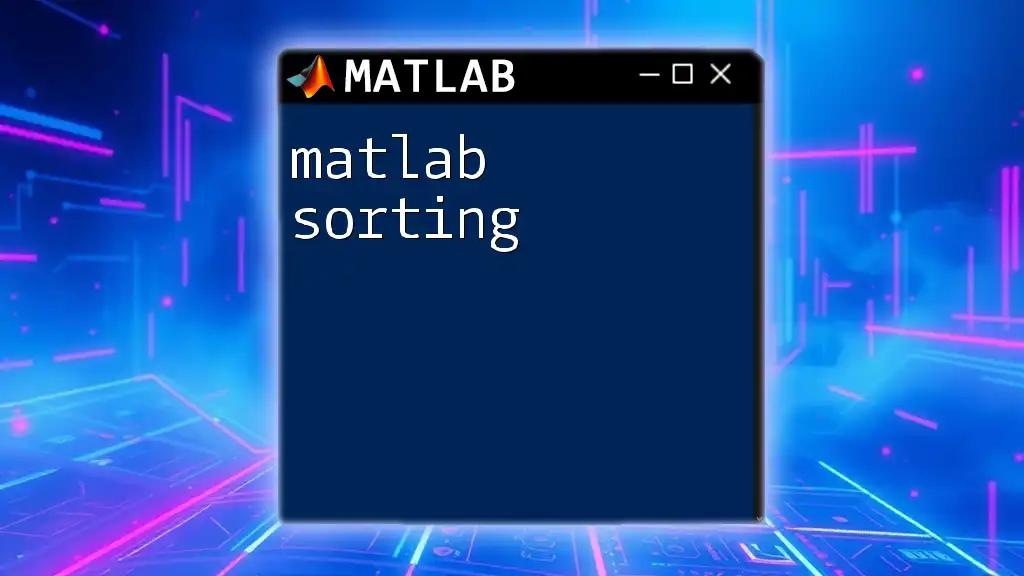
Techniques for Evaluating Smoothness
Visual Inspection
Visualizing smoothed and raw data is essential for assessing the effectiveness of smoothing techniques. MATLAB's plotting functions make it easy to compare these datasets.
Here’s a code snippet for visual comparison:
figure; subplot(2,1,1);
plot(data); title('Raw Data');
subplot(2,1,2);
plot(smoothedData); title('Smoothed Data');
This visual inspection can provide a quick understanding of how well the smoothing technique has worked.
Quantitative Measures of Smoothness
Beyond visual analysis, quantitative measures such as mean squared error (MSE) can offer a more precise evaluation of smoothness accuracy. A lower MSE indicates a better fit between the smoothed data and the original dataset.
You can calculate MSE using this code:
mse = mean((data - smoothedData).^2);
disp(['Mean Squared Error: ', num2str(mse)]);
The output will aid in determining whether the chosen smoothing method is suitable for your specific needs.

Advanced Smoothing Techniques
Wavelet Transform for Smoothing
Wavelet transforms provide an advanced approach to smoothing that captures data behavior at multiple scales. By decomposing a signal into various frequency components, wavelets can effectively diminish noise while maintaining important patterns.
This sample code applies wavelet transform smoothing:
[c, l] = wavedec(data, 5, 'db1');
smoothedWavelet = waverec(c, l, 'db1');
plot(data); hold on; plot(smoothedWavelet, 'm-');
The `wavedec` function decomposes the signal, while `waverec` reconstructs the smoothed signal. This multiscale representation allows for effective denoising without a significant loss of detail.
Custom Smoothing Functions
For those who need tailored smoothing solutions, creating a custom smoothing function in MATLAB can be highly beneficial. For example, you can define an exponential smoothing function as follows:
function smoothedData = exponentialSmoothing(data, alpha)
smoothedData = zeros(size(data));
smoothedData(1) = data(1);
for t = 2:length(data)
smoothedData(t) = alpha * data(t) + (1 - alpha) * smoothedData(t-1);
end
end
This function allows for control over the smoothing parameter `alpha`, enabling fine-tuning based on the specific characteristics of your data.
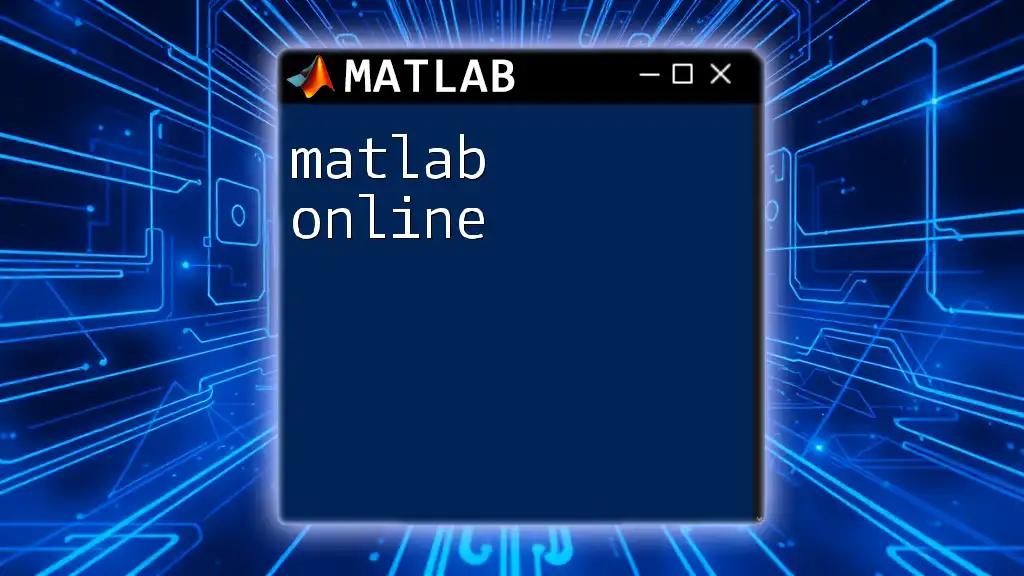
Practical Applications of MATLAB Smoothness
MATLAB smoothness techniques are versatile and applicable across various fields. In engineering, smoother signals can improve the accuracy of system response analyses. In finance, smoothing algorithms help in predicting stock trends by filtering out noise. Similarly, in environmental science, smoothness techniques can make weather data more interpretable, aiding in better decision-making.
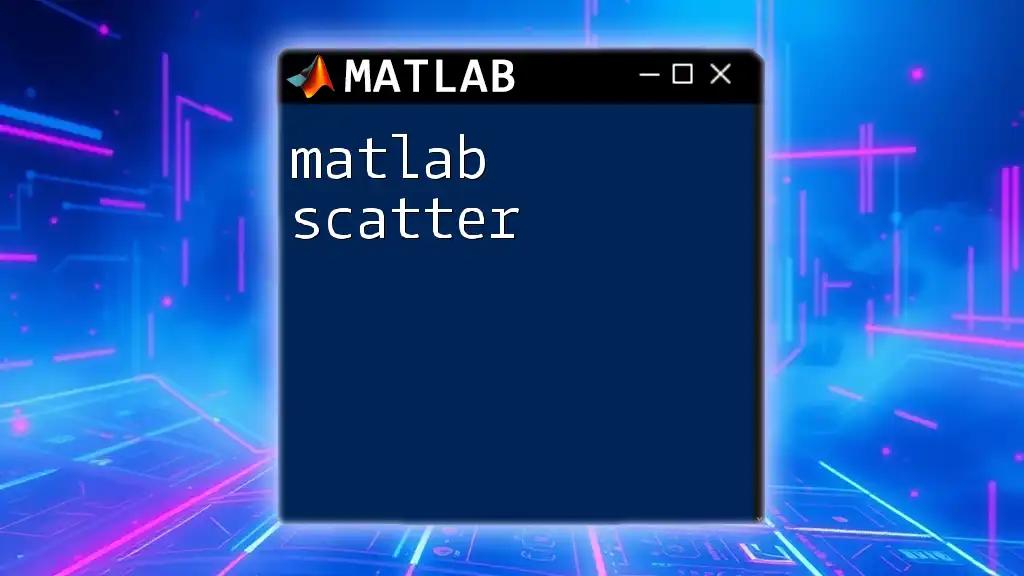
Troubleshooting Common Smoothing Issues
A common challenge in data smoothing is the balance between over-smoothing and under-smoothing. Over-smoothing can strip essential features, while under-smoothing may leave too much noise. Identifying these issues often requires iterative adjustments of parameters in your chosen smoothing function.
A good practice is to engage in thorough parameter tuning based on visual inspection and quantitative measures. Trying multiple smoothing methods can also help identify the most suitable approach for your specific dataset.
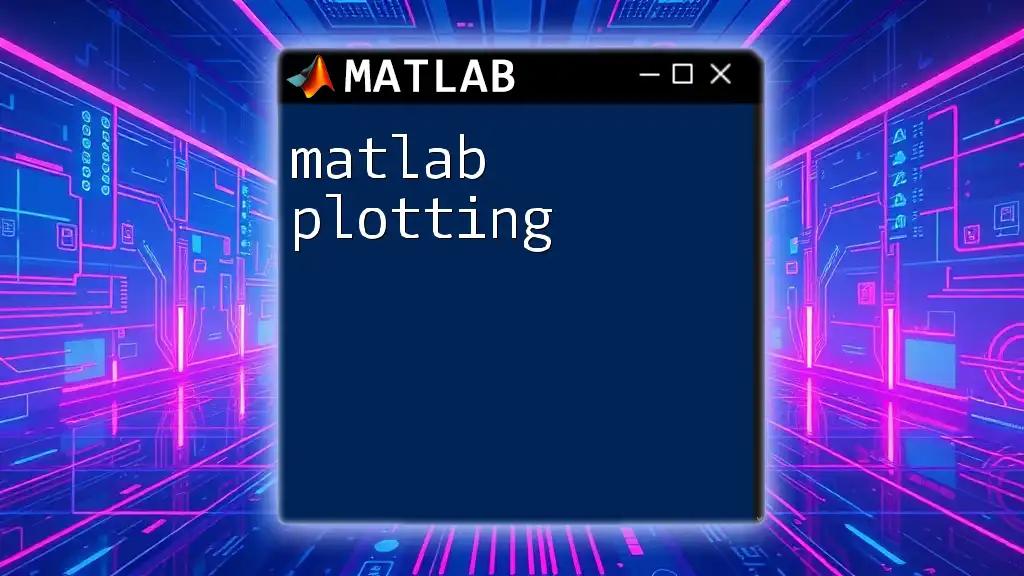
Conclusion
Understanding and applying MATLAB smoothness techniques is crucial for effective data analysis. With the various functions and methods available, from built-in options like `smooth()` to advanced techniques like wavelet transforms, users can significantly enhance their data's interpretability. By evaluating data visually and quantitatively, practitioners can ensure they select the most appropriate smoothing methods tailored to their specific needs.
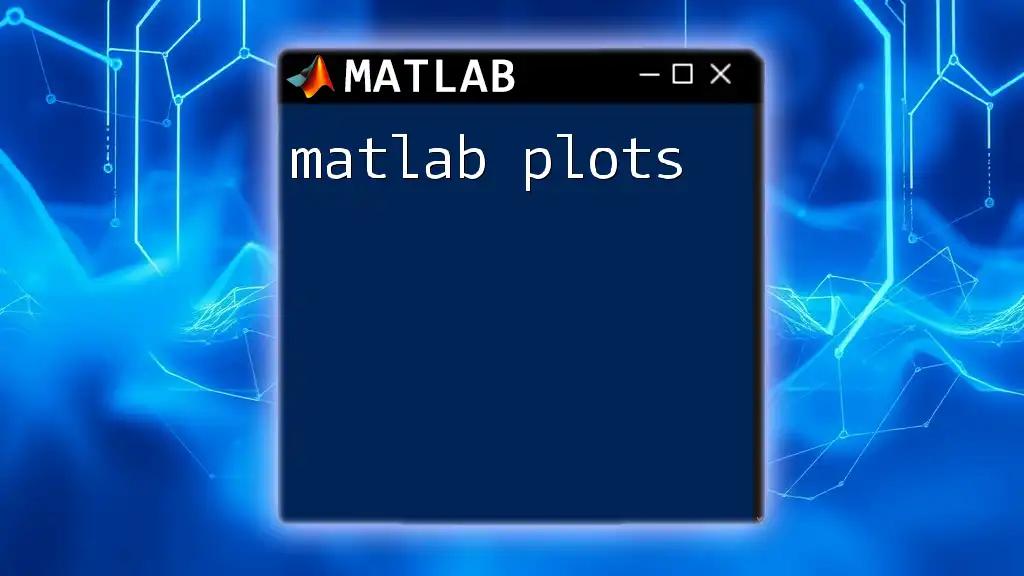
Additional Resources
To further explore MATLAB smoothness, users are encouraged to check out MATLAB’s official documentation, online tutorials, and community forums, where they can engage with fellow MATLAB enthusiasts and expert practitioners.