In MATLAB, you can define a function using the `function` keyword, followed by the output variables, the function name, and the input arguments, allowing you to create reusable code for various computations.
Here's a simple example of defining a function that sums two numbers:
function result = addNumbers(a, b)
result = a + b;
end
Understanding Functions in MATLAB
What is a Function?
In programming, a function is a reusable block of code designed to perform a specific task. In MATLAB, functions facilitate better organization of code and promote reusability across different scripts and projects. By defining functions, programmers can break complex problems into smaller, manageable components, leading to cleaner and more efficient code.
Basic Structure of a Function
A MATLAB function generally follows a specific syntax:
function [output1, output2, ...] = functionName(input1, input2, ...)
% Function documentation
% Code for the function
end
- Function Keyword: Every function begins with the keyword `function`.
- Output Variables: These are wrapped in square brackets and allow the function to return results.
- Function Name: The name should be descriptive enough to convey its purpose.
- Input Parameters: These are the values passed into the function for processing.
Advantages of Using Functions
Using functions in MATLAB provides several benefits:
- Improved Readability: Functions encapsulate code, making it more understandable.
- Easier Debugging: Isolating code into functions makes identifying issues simpler.
- Enhanced Organization: Structuring code into functions leads to a cleaner and more systematic workflow.
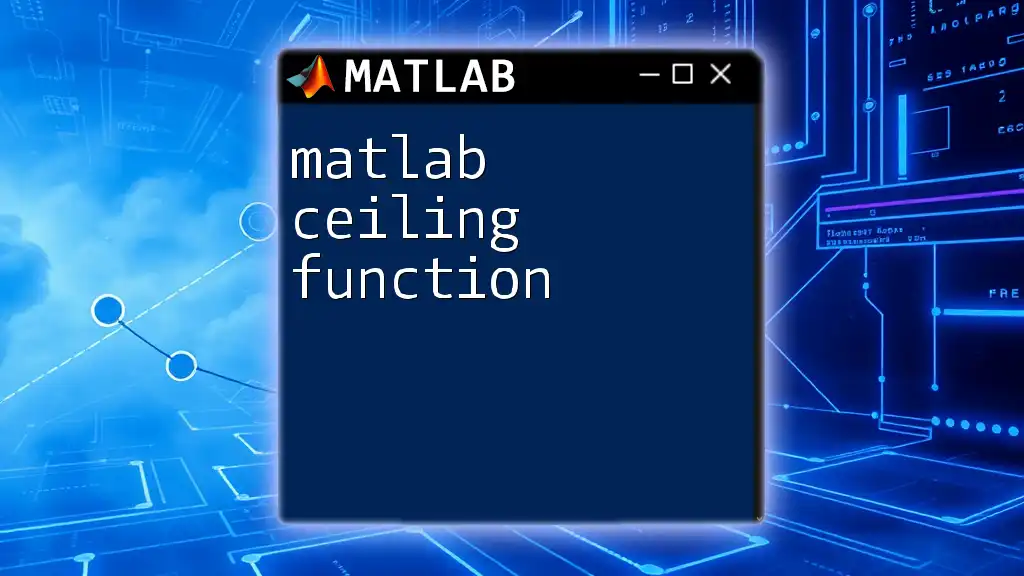
Defining a Function in MATLAB
Creating Your First Function
Creating a function in MATLAB is straightforward. Consider a simple function that squares an input value:
function output = myFunction(input)
output = input^2; % This function squares the input value
end
This function, `myFunction`, takes one input and returns its square. When you call `myFunction(3)`, the output will be `9`.
Function Naming Conventions
Choosing an appropriate name for a function is essential. A good function name should be:
- Descriptive and indicative of its action, such as `calculateSum` or `findMaxValue`.
- Adhering to MATLAB’s naming rules, which typically discourage special characters and spaces.
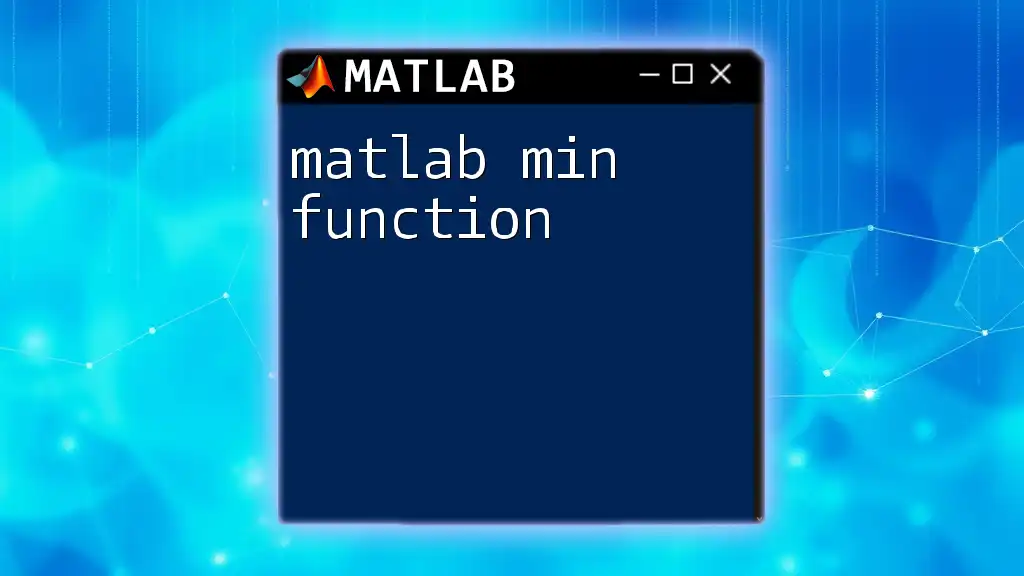
Input and Output Arguments
Types of Input Arguments
Functions can accept various input types:
- Required Inputs: Necessary for the function to operate correctly.
- Optional Inputs: Can enhance flexibility, often managed using the `nargin` function.
This allows functions to handle different numbers of inputs, catering to a broader range of scenarios.
Handling Output Arguments
You can define multiple output arguments in a MATLAB function. Here's how:
function [area, perimeter] = rectangleProperties(length, width)
area = length * width;
perimeter = 2 * (length + width);
end
By calling `rectangleProperties(5, 3)`, you receive both the area `15` and the perimeter `16`.
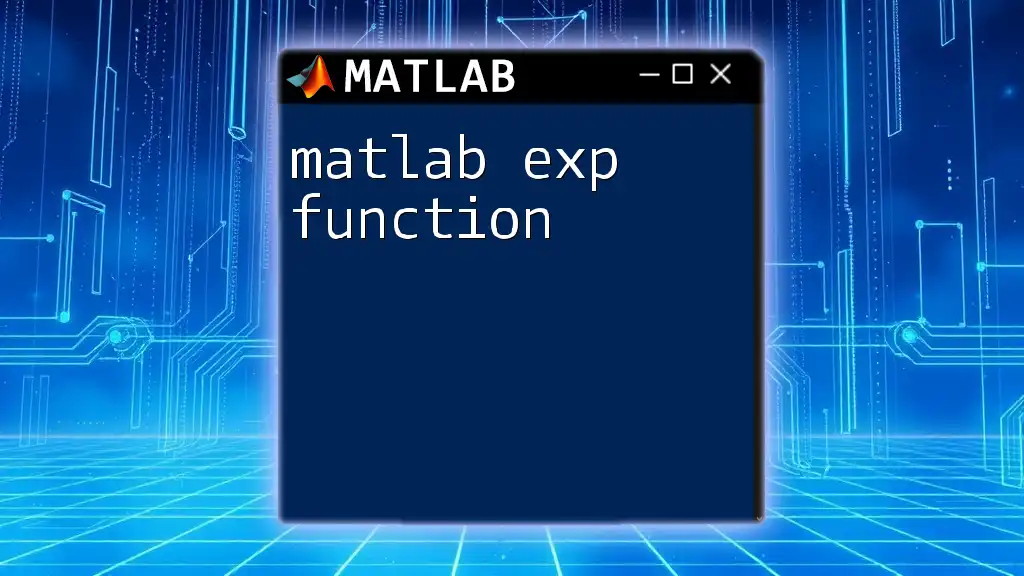
Best Practices for Writing Functions
Commenting and Documentation
Writing clear and informative comments within your code is crucial for maintainability. Utilize MATLAB's help feature to document your function, enabling users to understand its purpose and usage without diving into the code.
Managing Function Scope
Understanding variable scope is critical. In MATLAB:
- Local Variables: These are accessible only within the function itself. They help prevent inadvertent interference with variables in other parts of the code.
- Global Variables: If a variable needs to be accessed across different functions, it can be declared as global. However, this approach should be used judiciously to avoid unexpected behaviors.
Example: A Complete Function Development
Let’s explore creating a function that calculates the factorial of a number. The recursion method is a natural fit:
function f = factorial(n)
if n == 0
f = 1; % Base case: 0! is 1
else
f = n * factorial(n - 1); % Recursive case
end
end
Using this function, calling `factorial(5)` yields `120`, showcasing how functions can simplify complex calculations.
Optimizing Function Performance
When writing functions, it's essential to consider performance. Here are a few strategies:
- Avoid complex calculations inside loops when possible. Pre-calculate values outside the loop instead.
- Minimize function calls in performance-critical code, especially in tight loops. Consider vectorization, a powerful feature in MATLAB that allows for operating on entire arrays simultaneously rather than element by element.
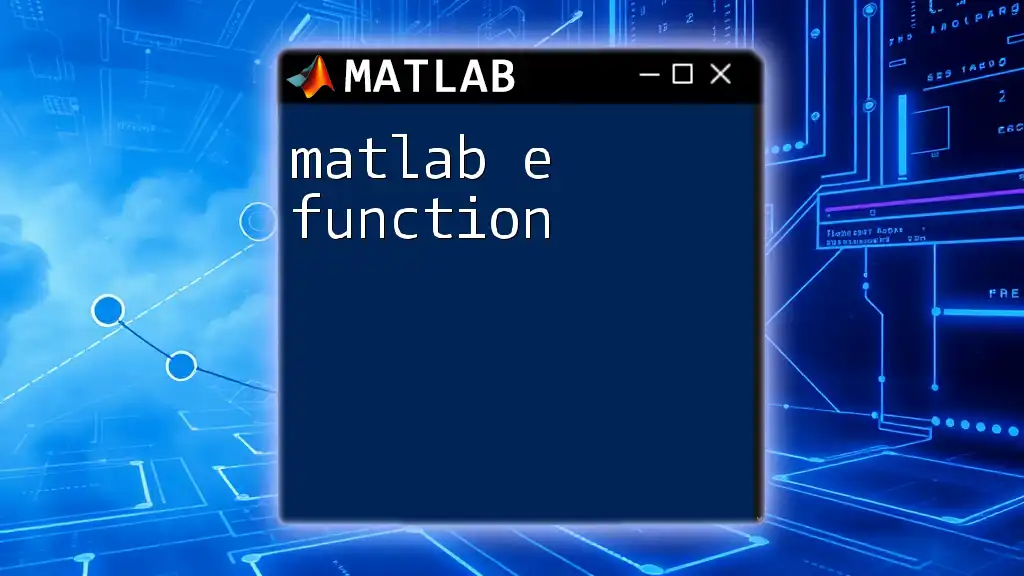
Debugging Functions in MATLAB
Common Errors in Functions
While creating functions, programmers can encounter a few common errors:
- Syntax Errors: Mistakes like missing `end` statements can lead to compilation errors. MATLAB will typically highlight the line where the error occurred, making it easier to correct.
- Logical Errors: These occur when the code runs but does not produce the expected output. These can be trickier to identify and may require systematic testing and debugging.
Using MATLAB Debugging Tools
MATLAB offers robust debugging tools to aid in identifying issues:
- Breakpoints: Set breakpoints by clicking on the left margin of the editor. This pauses execution, allowing you to inspect variables and flow.
- Step Execution: Use stepping commands to run your code line by line, providing insights into how data changes across your function.
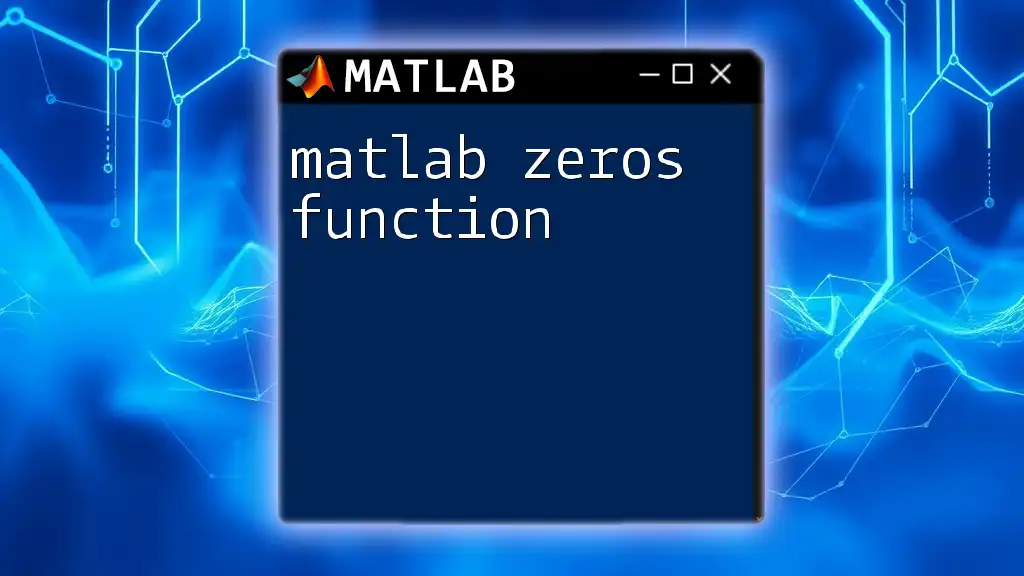
Conclusion
Understanding how to MATLAB define function is essential for anyone looking to harness the power of MATLAB effectively. By knowing how to create, document, and debug functions, you can improve your programming efficiency and capability tremendously. With practice, you will find that functions not only enhance the readability of your code but also pave the way for more complex and robust programming solutions.
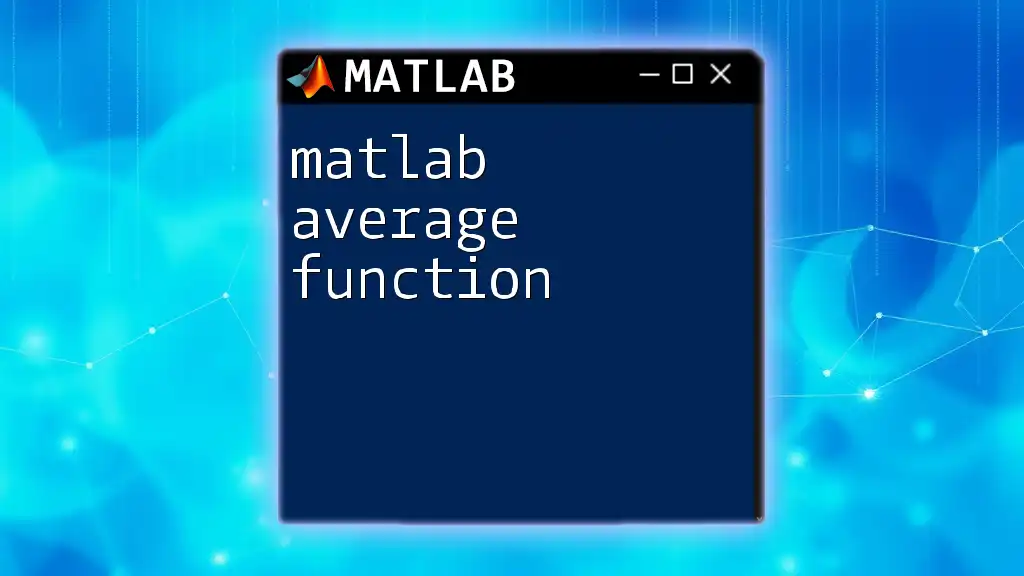
Additional Resources
Learning More About MATLAB Functions
To further enhance your skills, consider looking into the following resources:
- MATLAB’s official documentation provides comprehensive insights and examples.
- Online courses, tutorials, and forums offer community support and additional learning opportunities.
Community and Forums
Engaging with the MATLAB community through forums and platforms is a great way to learn from experienced users. Sharing questions and solutions in these spaces can offer valuable insights.
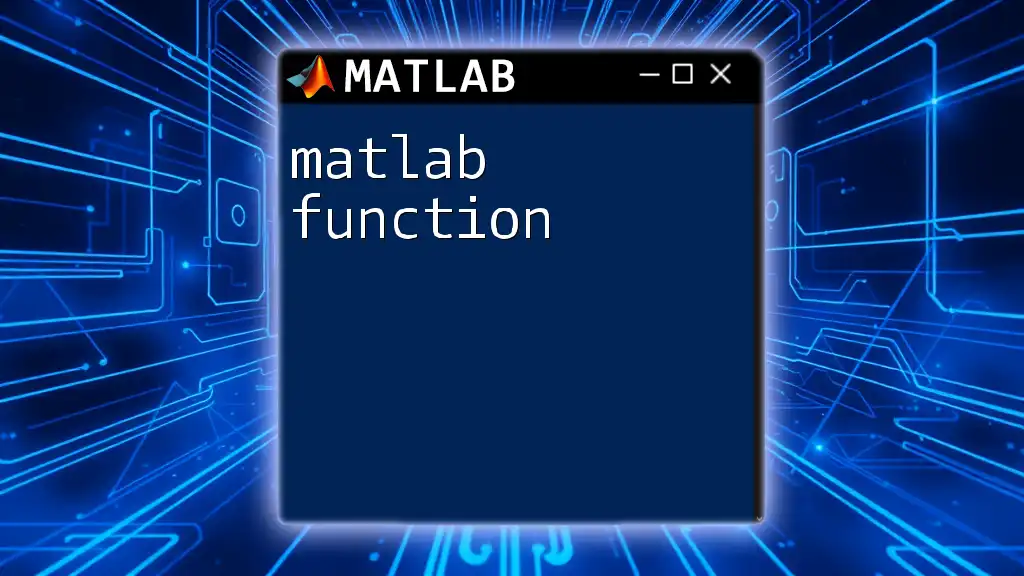
Frequently Asked Questions (FAQ)
When learning to define functions in MATLAB, newcomers often have questions regarding syntax, naming conventions, and best practices. Don't hesitate to reach out for support when you encounter challenges on your programming journey!