The `plot` function in MATLAB is used to create 2D line plots of data, allowing for easy visualization of mathematical relationships or trends.
Here’s a simple example of how to use the `plot` function in MATLAB:
x = 0:0.1:10; % Generate x values from 0 to 10 with an increment of 0.1
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Create a plot of y versus x
xlabel('X-axis'); % Label for the x-axis
ylabel('Y-axis'); % Label for the y-axis
title('Sine Wave'); % Title of the plot
grid on; % Add grid lines for better readability
Understanding the Basics of the Plot Function
What is the `plot` Function?
The MATLAB plot function is a fundamental tool used for visualizing data in two-dimensional space. It allows users to create graphs by plotting points on an X and Y axis. The primary purpose of the `plot` function is to provide a graphical representation of numerical data, which can enhance the understanding and analysis of patterns, trends, and relationships within the data.
Syntax of the Plot Function
The basic syntax for using the `plot` function is as follows:
plot(X, Y)
In this syntax:
- X is the data for the horizontal axis.
- Y is the data for the vertical axis.
These inputs can be vectors of the same length, allowing each element in X to correspond to an element in Y.
Required vs Optional Parameters
While the X and Y data are required, the `plot` function also supports various optional parameters. These parameters can be used to adjust the appearance and behavior of the plot. For example, you can specify line styles, colors, and markers to customize how your data is presented.
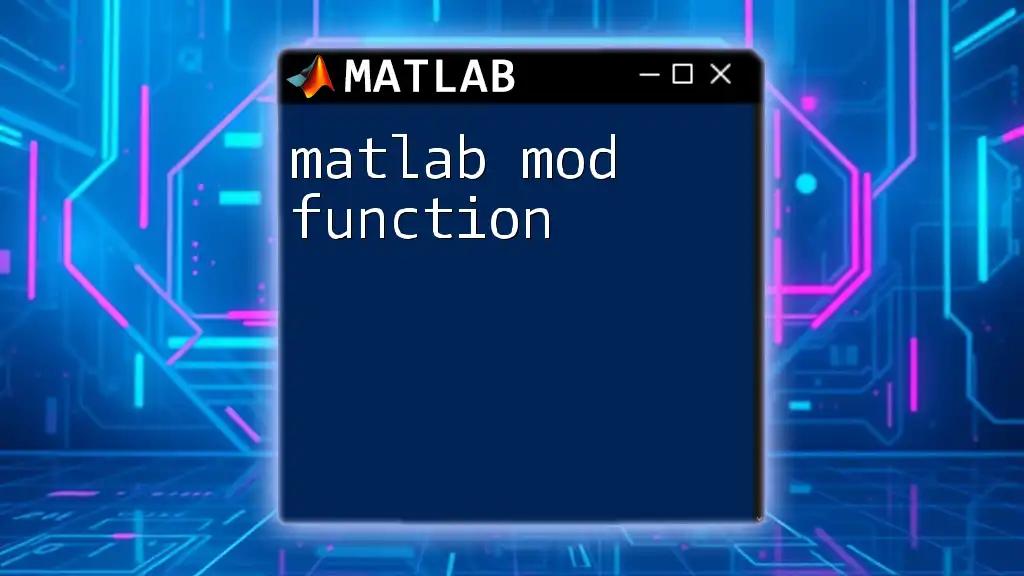
Creating Your First Plot
Step-by-Step Guide
To get started with creating your first plot in MATLAB, follow this simple step-by-step guide. Ensure that your MATLAB environment is set up correctly and that you have a script or command window ready to input commands.
Here’s a basic example:
x = 0:0.1:10; % Creating a range for x
y = sin(x); % Computing the sine of x
plot(x, y) % Creating the plot
In this example:
- We define an array x that ranges from 0 to 10 in increments of 0.1.
- The sine values for these x values are calculated and stored in y.
- Finally, the `plot` function is called to display the sine wave.
Customizing the Graph
Line Styles
MATLAB allows you to customize the style of the line used in your plots. You can choose from various styles such as solid, dashed, or dotted lines. Here’s how you can use them:
plot(x, y, '--'); % Dashed line
The string argument `'--'` instructs MATLAB to draw a dashed line. Other options include `'-'` for solid lines and `':'` for dotted lines.
Color Customization
Customizing colors can make your graph more visually appealing. You can use RGB color specifications or MATLAB’s predefined color names. For example:
plot(x, y, 'Color', [0 0.5 0]); % Dark green line
Here, `[0 0.5 0]` corresponds to the RGB values for dark green.
Markers
Adding markers to your plot can highlight specific data points. You can specify types of markers, such as circles (`'o'`), squares (`'s'`), or stars (`'*'`). Here’s an example:
plot(x, y, 'o'); % Circular markers
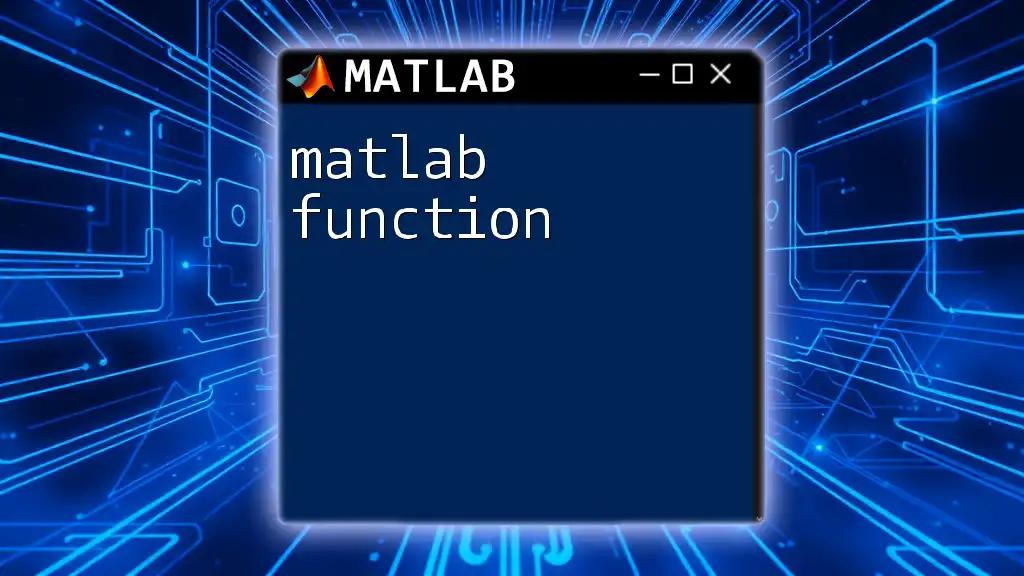
Enhancing the Plot
Adding Titles and Labels
Titles and labels are crucial for making your plots informative. They provide context, helping the viewer understand what the graph represents. Here is how to add them:
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
Using the `title`, `xlabel`, and `ylabel` functions, you can give your plot a title and label its axes, significantly improving clarity.
Legends and Annotations
Including a legend is essential when multiple datasets are plotted on the same graph. It helps identify which data series corresponds to which visualization. Here’s how to add a legend:
plot(x, y, 'DisplayName', 'Sine');
legend show;
In this code, the `DisplayName` property specifies what label will appear in the legend.
Moreover, you can annotate specific points on your plot to draw attention to significant values. Use the `text` function to place text at specified coordinates.
Grid and Axes Settings
Enabling a grid can enhance the readability of your plot. By using the following command, you can turn on the grid:
grid on;
Additionally, to customize the limits of your axes and focus on specific data ranges, you can set the X and Y axis limits as follows:
xlim([0, 10]);
ylim([-1, 1]);
This example sets the X-axis to span from 0 to 10 and the Y-axis from -1 to 1, allowing you to control the window through which your data is visualized.
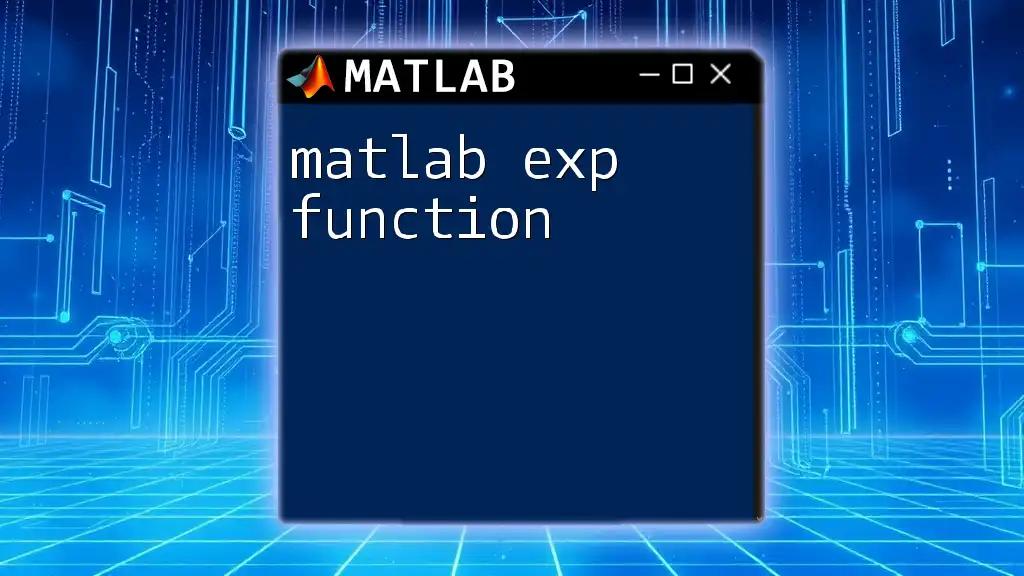
Advanced Plotting Techniques
Multiple Plots in One Figure
In many scenarios, you may want to overlay multiple plots in a single figure. You can achieve this easily in MATLAB using `hold on` and `hold off`. Here is an illustration:
hold on;
plot(x, y, 'b-'); % Sine wave
plot(x, cos(x), 'r--'); % Cosine wave
hold off;
Using `hold on`, you allow multiple datasets to be plotted on the same figure without clearing the previous ones. The `hold off` command, in contrast, ends this holding state.
Subplots
When comparing different datasets or visualizations side by side, subplots are a great solution. MATLAB makes it simple to create subplots in a grid format. For example:
subplot(2, 1, 1);
plot(x, sin(x));
title('Sine Wave');
subplot(2, 1, 2);
plot(x, cos(x));
title('Cosine Wave');
In this example, two subplots are created, displaying a sine wave in the top half of the figure and a cosine wave in the bottom half.
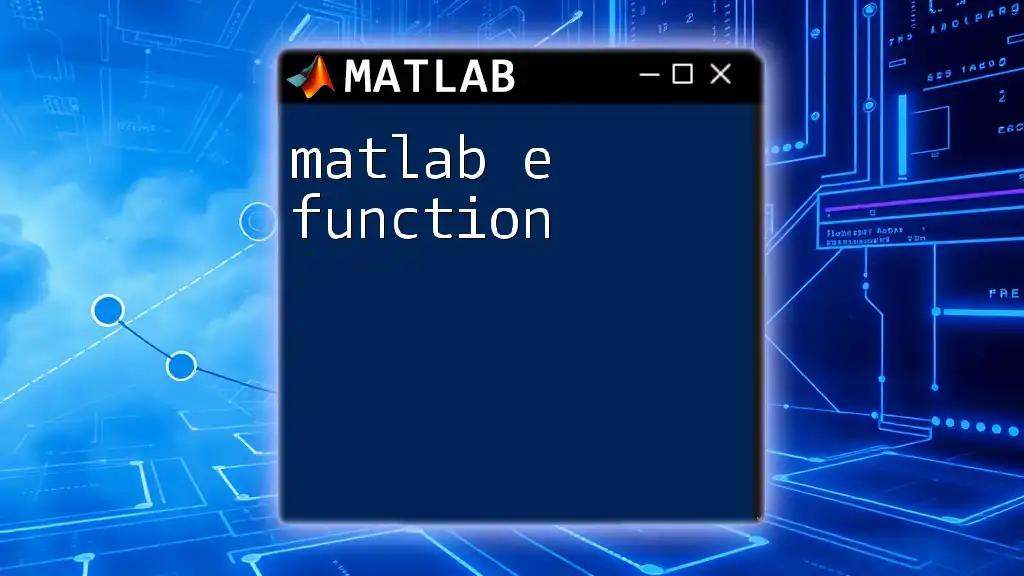
Exporting and Saving Plots
Saving as an Image File
Once you’ve spent time crafting your plot, you may want to save it as an image for use in reports or presentations. MATLAB allows you to save figures in various formats such as PNG, JPEG, or PDF. Here’s a quick example:
saveas(gcf, 'myPlot.png'); % Save current figure as PNG
By using `gcf`, you reference the current figure, and `saveas` saves it as a PNG image.
Copying to Clipboard
Another useful feature is the ability to copy the figure directly to your clipboard. This is helpful for quick pasting into presentations or documents.
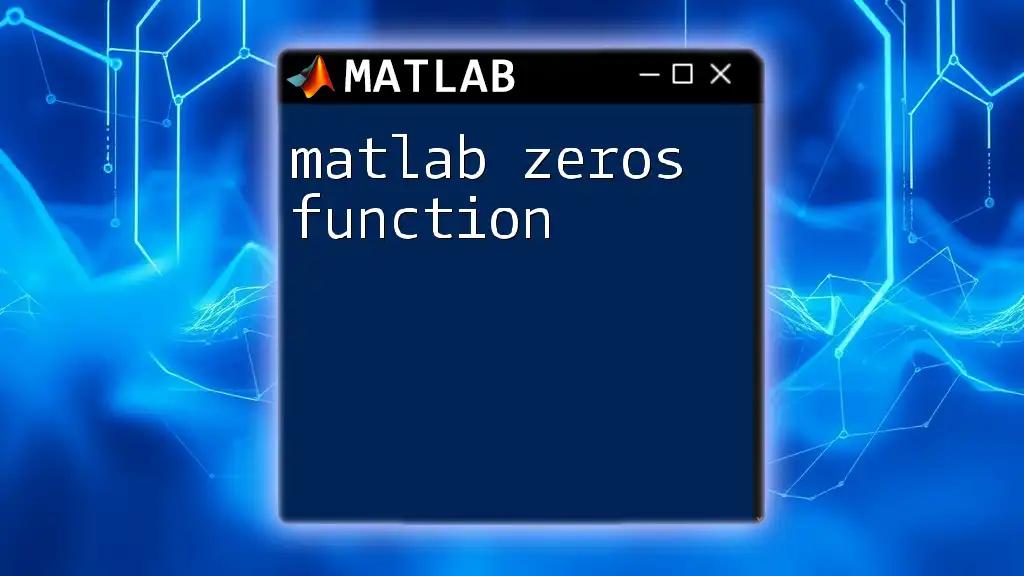
Common Errors and Troubleshooting Tips
Frequently Encountered Errors
When working with the MATLAB plot function, some common errors may arise, such as data dimension mismatches or undefined variables. For instance, if you have two vectors of different lengths and attempt to plot them, MATLAB will return an error indicating that the dimensions do not match.
Solutions and Best Practices
To avoid these issues, always ensure that your X and Y inputs are of the same length before plotting. Moreover, labeling your plots and checking variable definitions can help complement your understanding and clarity.
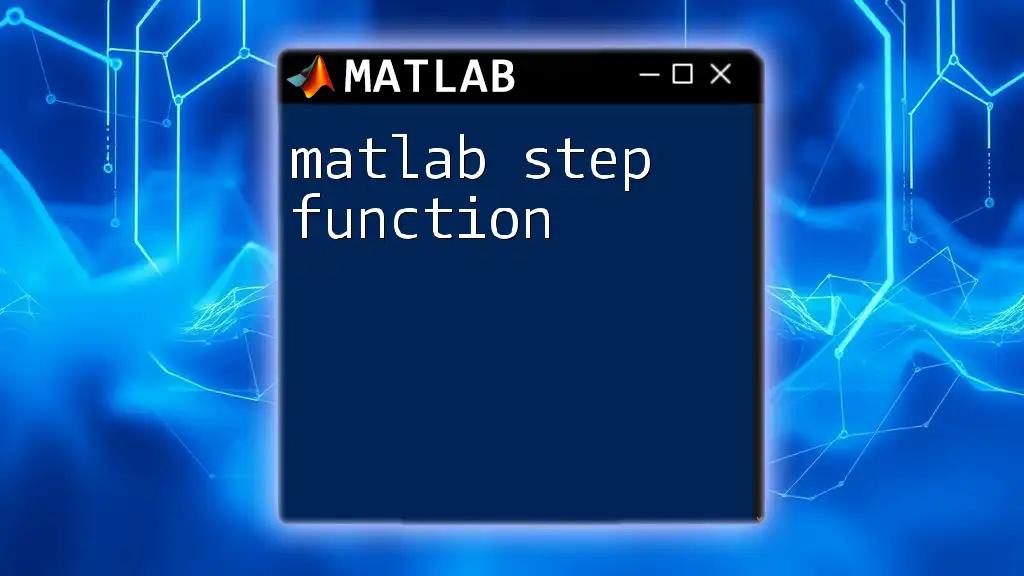
Conclusion
The MATLAB plot function plays a vital role in data visualization, serving as a foundation upon which users can build sophisticated graphical representations of their datasets. By mastering the basics and exploring advanced features, you can significantly enhance your ability to analyze and present data effectively. Remember to practice with the various functionalities covered in this guide to become proficient with MATLAB’s capabilities in graphing and plotting.