The `zeros` function in MATLAB creates an array filled with zeros, with the specified dimensions provided as arguments.
A = zeros(3, 4); % Creates a 3x4 matrix of zeros
Introduction to the Zeros Function
The MATLAB Zeros Function is an essential tool for creating arrays filled with zeros, often used for initializing matrices and vectors in your calculations. Understanding this function is fundamental for anyone looking to perform matrix operations efficiently in MATLAB. The zeros function is particularly useful in scenarios where you require placeholder arrays, especially when building algorithms that depend on matrix dimensions.
Syntax of the Zeros Function
Understanding the syntax of the `zeros` function is crucial for proper usage.
The basic syntax of the function can be broken down as follows:
- `Z = zeros(n)` – This creates an n-by-n matrix of zeros.
- `Z = zeros(m, n)` – This generates an m-by-n matrix filled with zeros.
- `Z = zeros(size(A))` – This will create an array of zeros that matches the dimensions of matrix `A`.
Input Arguments Explained:
- n: A scalar that defines a square matrix.
- m and n: Scalars defining the dimensions of a 2D array.
- A: An existing matrix whose size will be used to construct a new zero array.
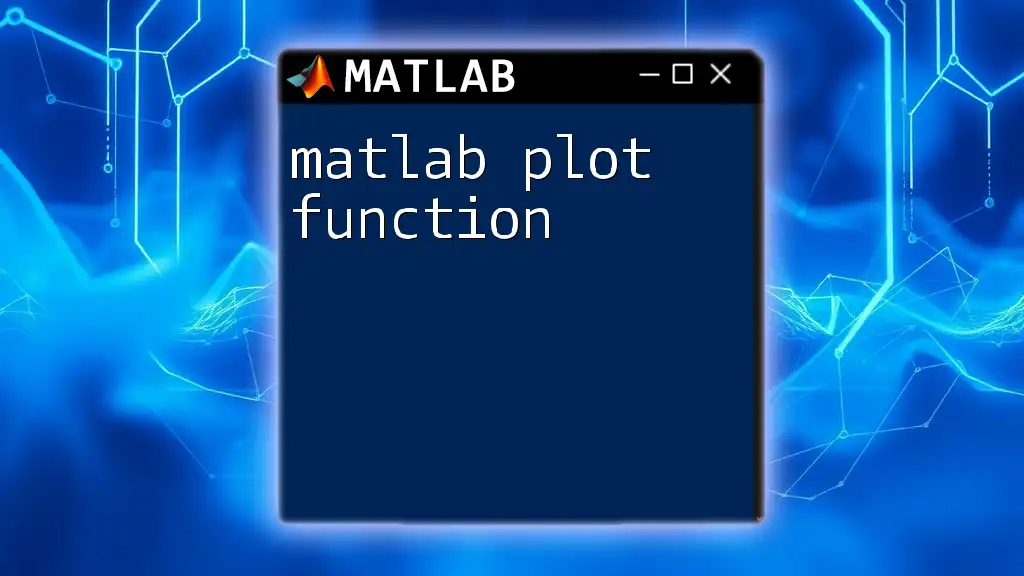
Creating Arrays with the Zeros Function
Creating a 1D Array
You can create a one-dimensional array using the zeros function. For example, to create a row vector with five elements, you can use the following code:
Z = zeros(1, 5);
This command will yield a row vector: `[0 0 0 0 0]`.
Creating a 2D Array
Shifting focus to a two-dimensional array, you can generate, for example, a 3x4 matrix of zeros:
Z = zeros(3, 4);
This results in a matrix that looks like this:
0 0 0 0
0 0 0 0
0 0 0 0
Creating an N-D Array
If you're interested in creating a higher-dimensional array, such as a 2x3x4 matrix, the syntax remains the same:
Z = zeros(2, 3, 4);
This will create a three-dimensional array filled with zeros, allowing for versatile data manipulation.
Creating an Array Based on Another Array
A practical feature of the zeros function is its ability to mimic the dimensions of an existing matrix. For example:
A = [1 2; 3 4];
Z = zeros(size(A));
In this case, `Z` will have the same dimensions as `A`, resulting in a 2x2 matrix of zeros.
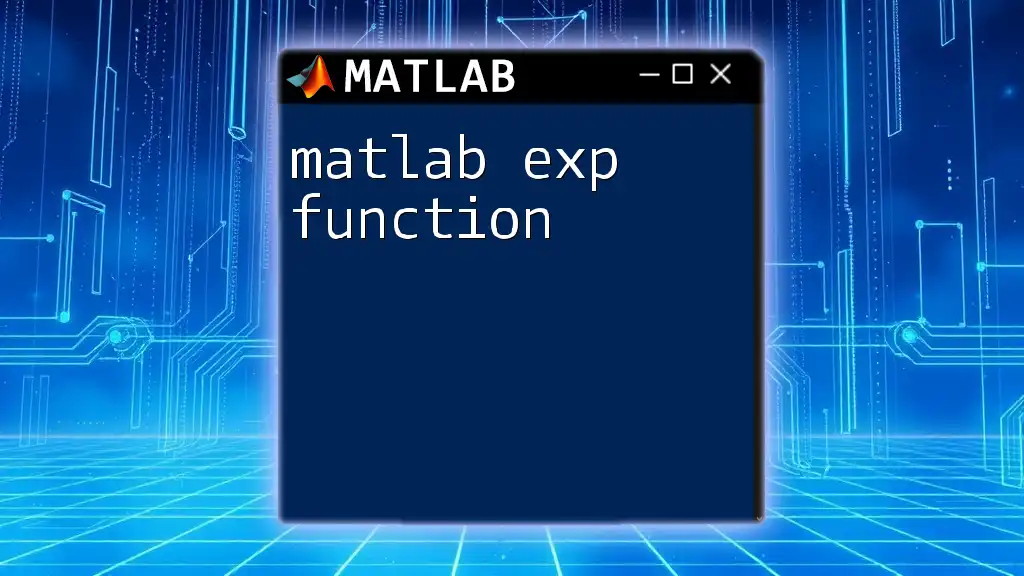
Data Types and Zeros Function
Default Data Type
When discussing the MATLAB zeros function, it's important to note that the default data type for the created zeros is `double`. This can be illustrated with the following snippet:
Z = zeros(3, 4); % Z is of type double
disp(class(Z));
This command confirms that `Z` is indeed a double type matrix.
Specifying Data Types
In newer versions of MATLAB, you can specify the data type of the resulting array using the 'like' argument. This is particularly useful when you want to ensure consistency in your variable types. For example:
B = int32(zeros(2, 2, 'like', A));
Here, you are creating a 2x2 zero matrix of type `int32` that matches the type of `A`.
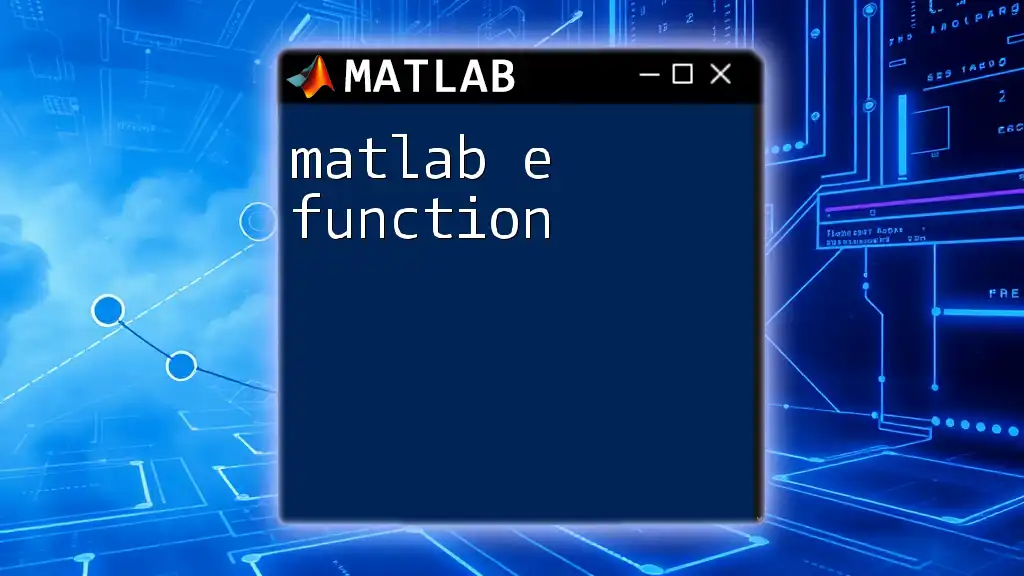
Working with Zeros Function in Applications
Zero Matrices in Linear Algebra
In linear algebra, zero matrices play a significant role, especially when solving systems of linear equations. They can act as identity elements in matrix operations. Consider this example:
A = [1 2; 3 4];
B = zeros(size(A));
In this code, `B` serves as a neutral element, allowing further operations such as addition or multiplication.
Initializing Variables and Arrays for Algorithms
Zero initialization is a common practice in many algorithms. For instance, if you need to collect results over several iterations, using zeros to initialize your results array can greatly enhance performance and readability:
results = zeros(1, num_iterations);
This code snippet prepares a results vector, ensuring it is filled with zeros before computation begins.
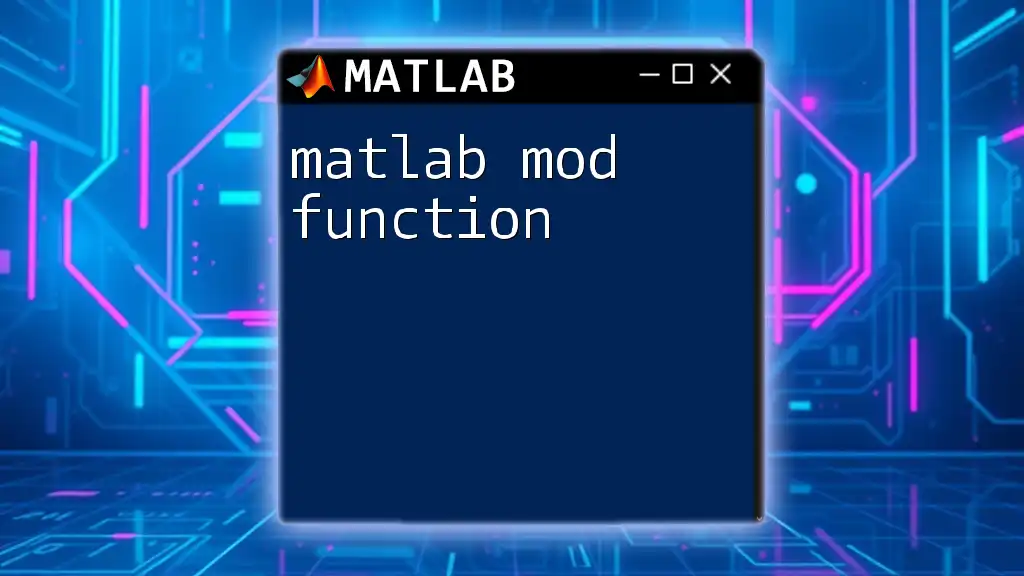
Common Mistakes and Troubleshooting
Oversized Arrays
One common issue with the `zeros` function arises when attempting to create very large matrices, which can lead to memory allocation problems. Therefore, it is recommended to check available memory and optimal matrix size before execution.
Mismatched Dimensions
Another potential pitfall is attempting to perform operations on matrices with mismatched dimensions. For instance:
% Attempting to add matrices of different sizes with Z = zeros(3, 2);
This can result in errors, emphasizing the need to ensure compatibility during mathematical operations.
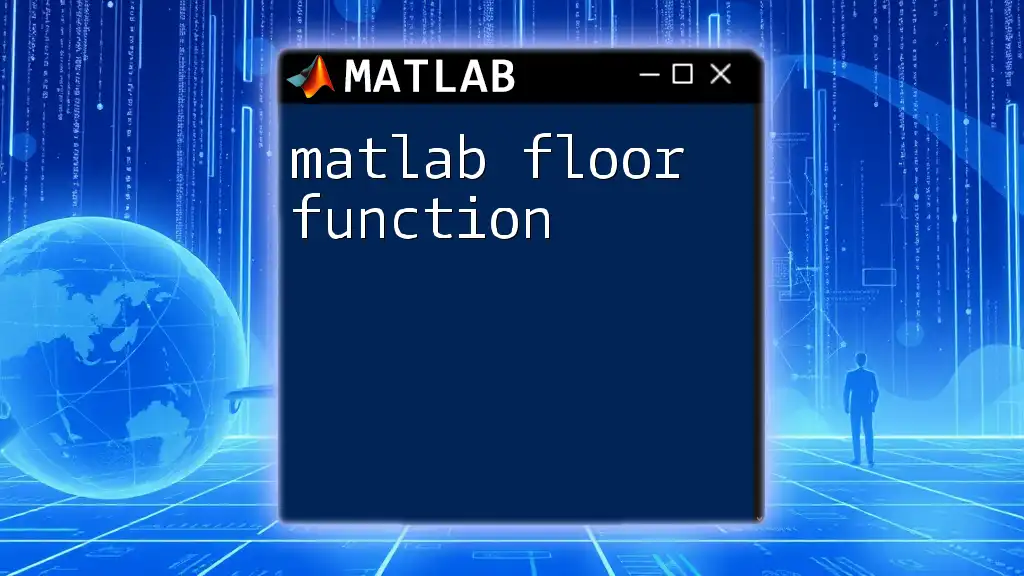
Summary
In summary, the MATLAB zeros function is not just a simple command; it is a powerful tool for creating arrays filled with zeros efficiently and effectively. From initializing matrices to playing a pivotal role in linear algebra, this function provides versatility in programming and data manipulation.
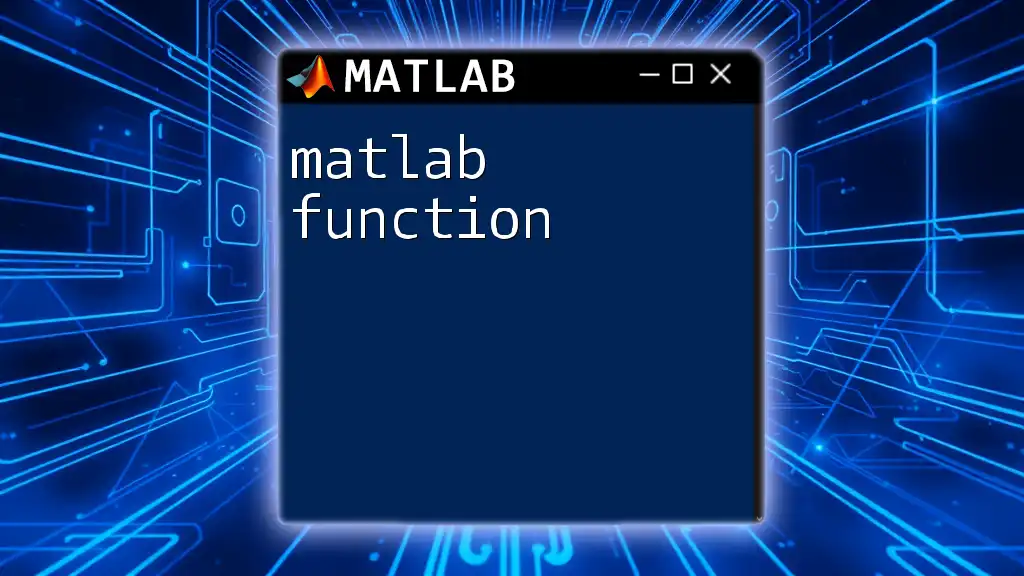
Additional Resources
To further enhance your understanding, refer to the official MATLAB documentation for the zeros function, explore interactive tutorials, and seek out additional resources focused on matrix operations.
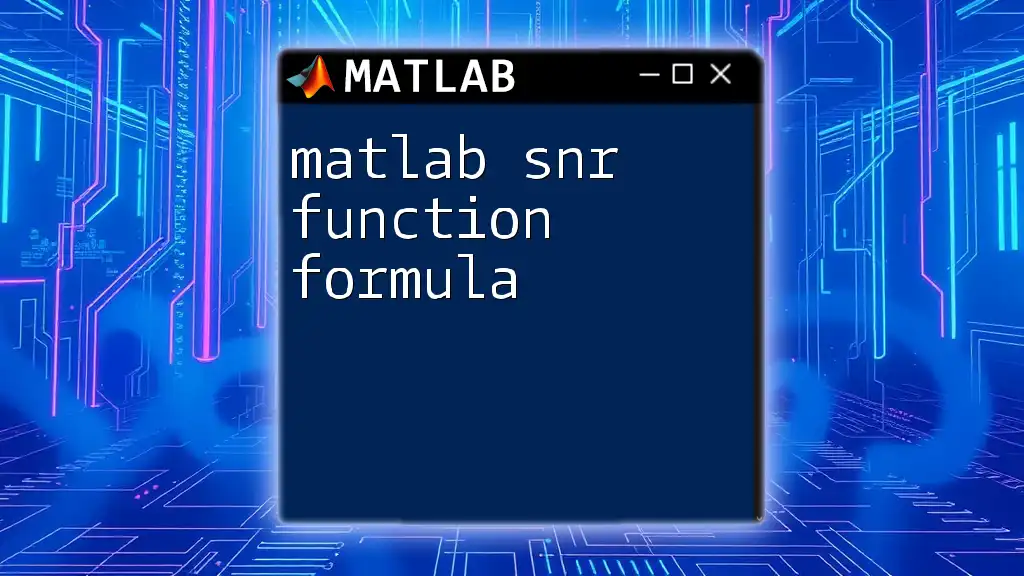
Conclusion
Mastering the zeros function in MATLAB is a step towards efficient programming and effective data handling. As you experiment with this function, you will find it to be an invaluable asset in your MATLAB toolkit.