In MATLAB, you can solve a system of linear equations using matrix operations, specifically leveraging the backslash operator (`\`) to find the values of the variables efficiently.
Here's a code snippet demonstrating how to solve the system of equations \(2x + 3y = 5\) and \(4x - y = 1\):
A = [2, 3; 4, -1]; % Coefficient matrix
B = [5; 1]; % Right-hand side matrix
X = A \ B; % Solve for X
In this example, `X` will contain the values of `x` and `y` that satisfy both equations.
Understanding Systems of Equations
Definition of Systems of Equations
A system of equations is a collection of two or more equations with the same set of unknowns. Systems can be classified into two primary categories: linear and nonlinear.
-
Linear systems consist of equations that can be represented graphically as straight lines. For example: \[ \begin{align*} 2x + 3y &= 6 \\ 4x - y &= 5 \end{align*} \]
-
Nonlinear systems involve equations that graphically represent curves or shapes other than straight lines. Solving these can be more complex and often requires iterative methods.
Examples of Systems of Equations
Consider the following simple linear system: \[ \begin{align*} 2x + 3y &= 6 \\ 4x - y &= 5 \end{align*} \] Solving these equations will yield a unique solution if the lines they represent intersect at a single point.
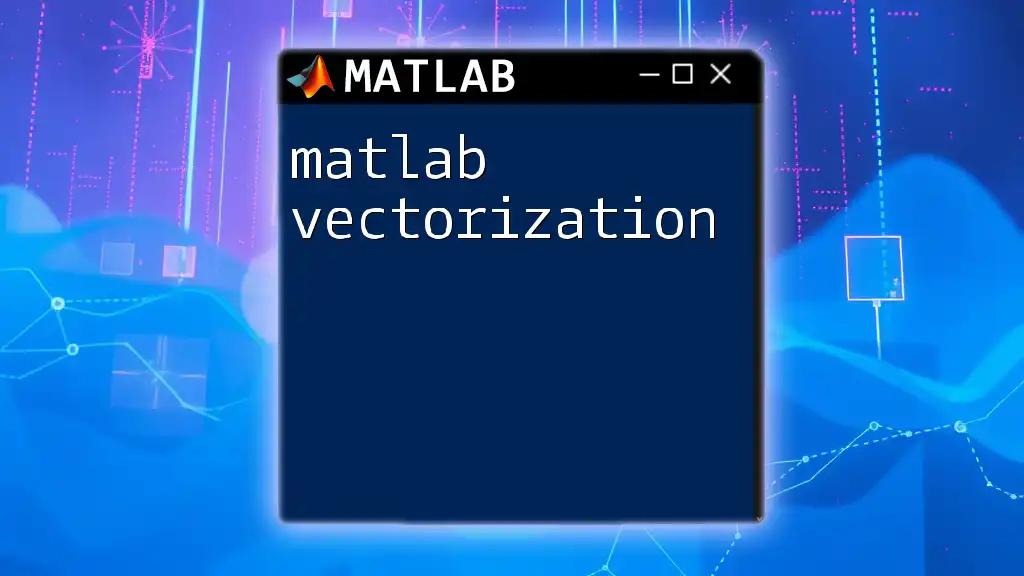
Why Use MATLAB for Solving Equations?
Advantages of Using MATLAB
MATLAB is a powerful tool for solving systems of equations for several reasons:
- It has a user-friendly interface that simplifies the coding process.
- It offers built-in functions that handle complex calculations efficiently.
- MATLAB is exceptionally proficient for managing large systems, making it an ideal choice in engineering and scientific research.
Common Applications
MATLAB's ability to solve systems of equations is invaluable across various fields, including:
- Engineering problems such as circuit analysis and structural engineering.
- Economic models that analyze data trends and predictions.
- Scientific research where modeling complex relationships is often necessary.
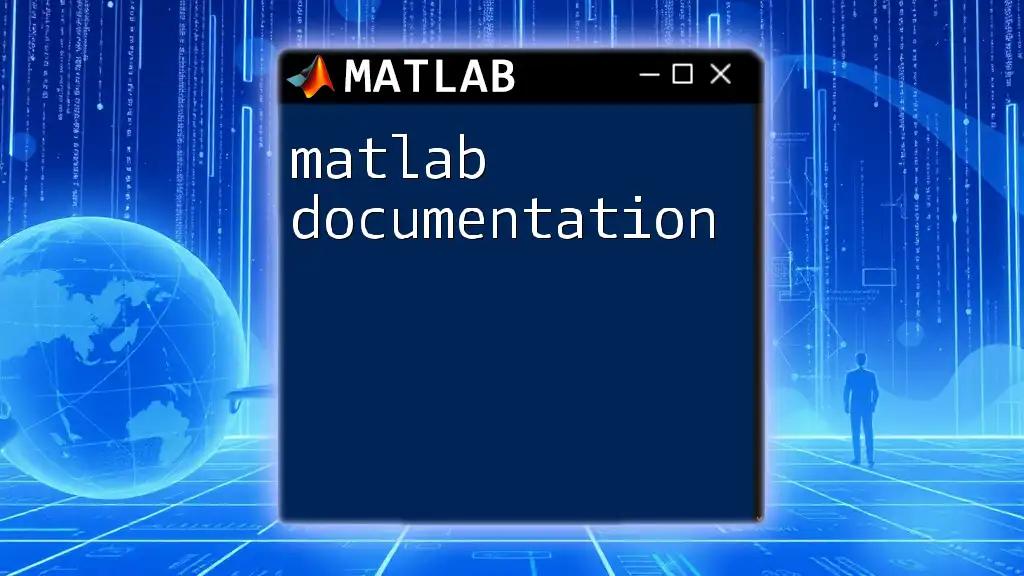
Methods to Solve Systems of Equations in MATLAB
Using Inline Commands
MATLAB allows you to address systems of equations using direct commands in the Command Window. The `linsolve` function is straightforward for smaller systems. Here’s an example:
A = [2, 3; 4, -1];
B = [6; 5];
X = linsolve(A, B);
In this code:
- `A` is the matrix of coefficients.
- `B` is the matrix of constants.
- `X` is the output, representing the solution vector containing the values of the unknowns.
Using the Backslash Operator
The backslash operator (`\`) is another efficient method to solve linear systems in MATLAB. It is often preferred due to its ease of use and speed:
X = A\B;
This command computes the same solution as `linsolve`, providing a simple yet powerful approach to solving equations.
Using MATLAB Functions: `solve`, `linsolve`, and `fsolve`
Using `solve`
The `solve` function is particularly useful when dealing with symbolic mathematics in MATLAB. Here’s how you can apply it:
syms x y
eq1 = 2*x + 3*y == 6;
eq2 = 4*x - y == 5;
sol = solve([eq1, eq2], [x, y]);
In this example:
- `syms` defines symbolic variables.
- Each equation is represented with the equality operator.
- The `solve` function outputs the solution in terms of the symbolic variables.
Using `linsolve`
The `linsolve` function is specifically designed for linear systems. It provides a robust way to handle these equations, especially when the coefficient matrix is large. An example is as follows:
A = [2, 3; 4, -1];
B = [6; 5];
X = linsolve(A, B);
Using `linsolve` is particularly advantageous when working with square matrices, where performance and numerical stability are critical.
Using `fsolve`
When confronting nonlinear equations, `fsolve` becomes a vital tool. It utilizes iterative numerical methods to approach a solution. Here’s an example:
fun = @(x)[2*x(1) + 3*x(2) - 6; 4*x(1) - x(2) - 5];
x0 = [0; 0];
x = fsolve(fun, x0);
In this example:
- The function `fun` defines the system of nonlinear equations.
- `x0` is the initial guess for the solution.
- `fsolve` returns the values of `x` where the equations equal zero.
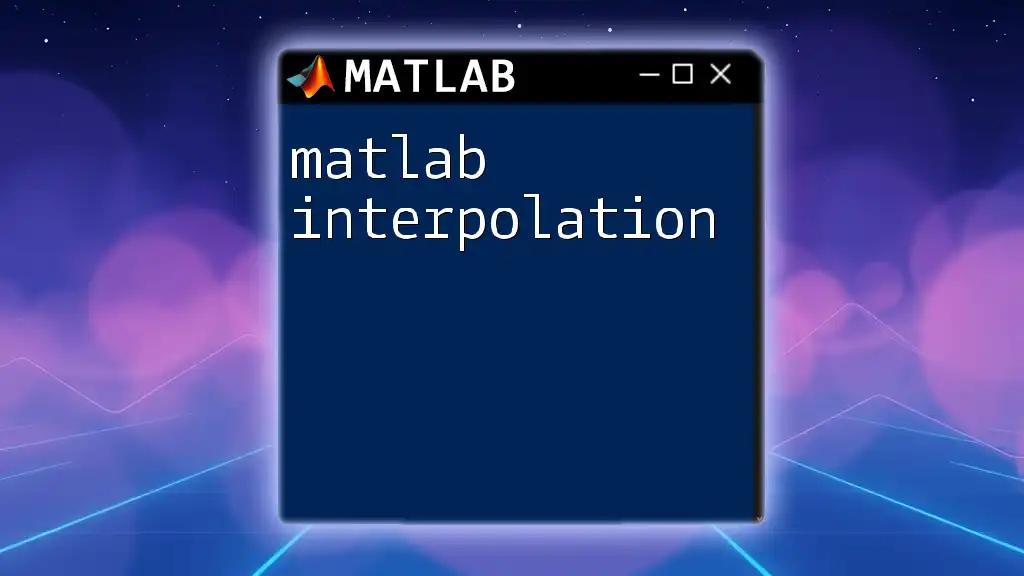
Handling Special Cases
Underdetermined Systems
An underdetermined system has more variables than equations. In such cases, the system may have infinitely many solutions. For example:
A = [1, 1, 0; 0, 1, 1];
B = [4; 3];
[X, freeVars] = linsolve(A, B);
Here, `X` contains a basic solution, while `freeVars` represent the parameters that signify the degrees of freedom within the solution.
Overdetermined Systems
An overdetermined system carries more equations than variables. These often have no solution or a unique solution obtained through relatively straightforward means, such as least squares. Here’s an example:
A = [1, 2; 2, 4; 3, 6];
B = [1; 2; 3];
X = A\B; % Least squares solution
In this code, MATLAB computes a least-squares solution, yielding the best approximate solution when an exact solution does not exist.
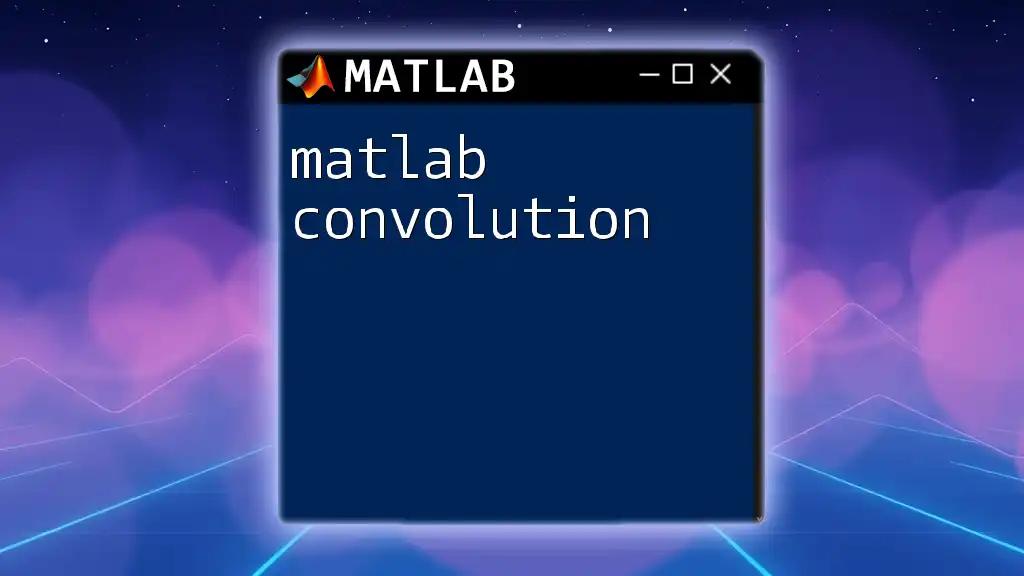
Visualizing Multiple Solutions
Visualizing systems of equations can significantly enhance understanding and interpretation of solutions. MATLAB’s plotting capabilities allow for effective graphics representation.
Here’s how you can plot the equations from the earlier example:
x = linspace(-10, 10, 100);
y1 = (6 - 2*x)/3; % First equation
y2 = 4*x - 5; % Second equation
plot(x, y1, 'r', x, y2, 'b');
title('Graphical Solution to the System of Equations');
xlabel('x-axis');
ylabel('y-axis');
legend('2x + 3y = 6', '4x - y = 5');
grid on;
This code displays the equations as lines on a graph, allowing you to visualize the intersection point, which corresponds to the solution of the system.
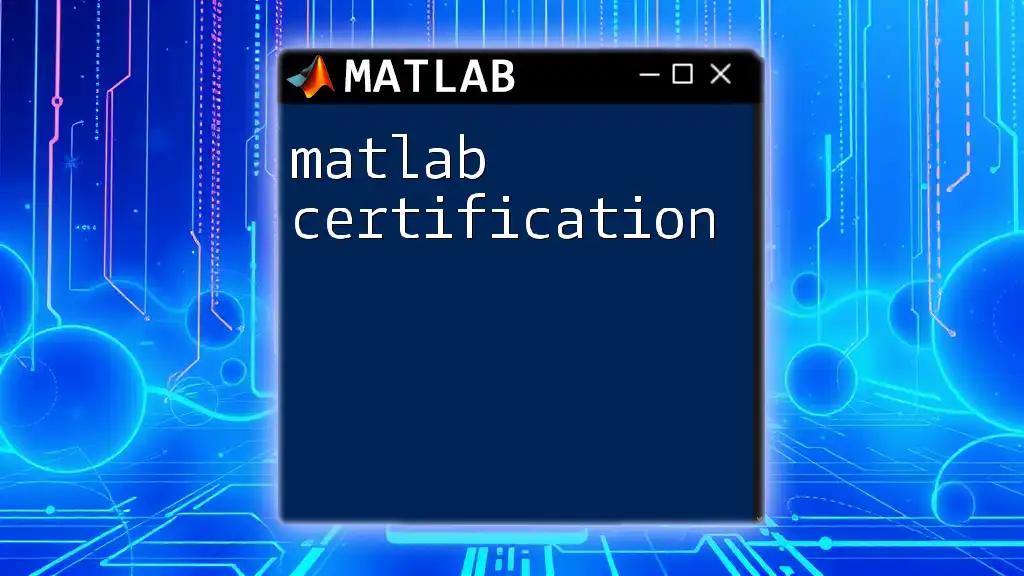
Conclusion
Solving a system of equations in MATLAB can be performed through various techniques, ranging from basic commands to advanced functions, each with unique advantages depending on the scenario. With its powerful functionality and flexibility, MATLAB is an essential tool for both students and professionals.
Practicing these techniques will help you gain proficiency in solving systems of equations efficiently. Make sure to explore additional resources and tutorials to deepen your understanding and skills in using MATLAB to solve systems of equations effectively.
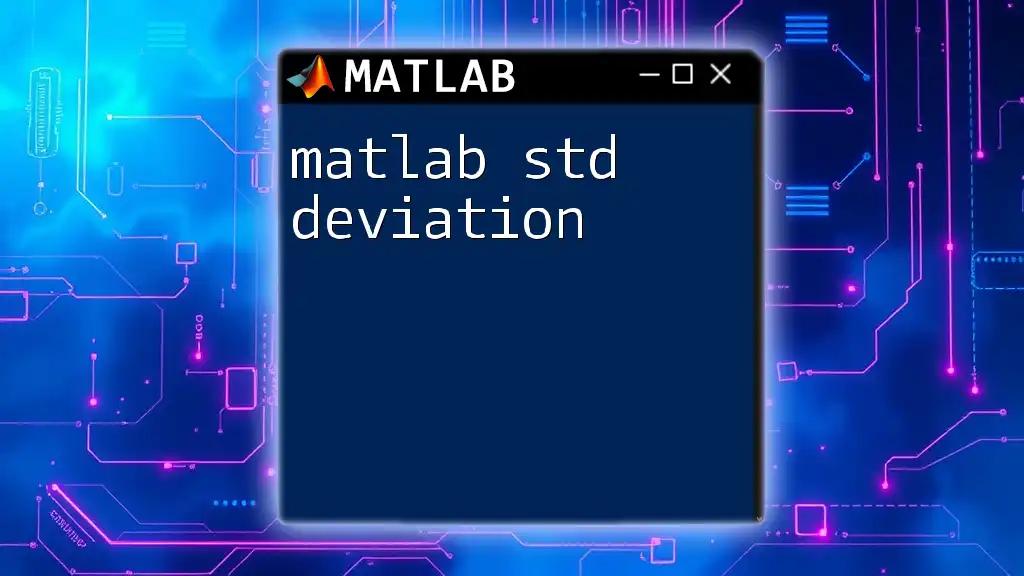
Additional Resources
Useful MATLAB Functions and Documentation
For further exploration, refer to the official MATLAB documentation which provides comprehensive guidance on these functions and additional capabilities.
Suggested Exercises for Practice
To reinforce your understanding, try solving different systems of equations by modifying the examples provided. Challenge yourself with both linear and nonlinear scenarios and explore how different functions yield results.
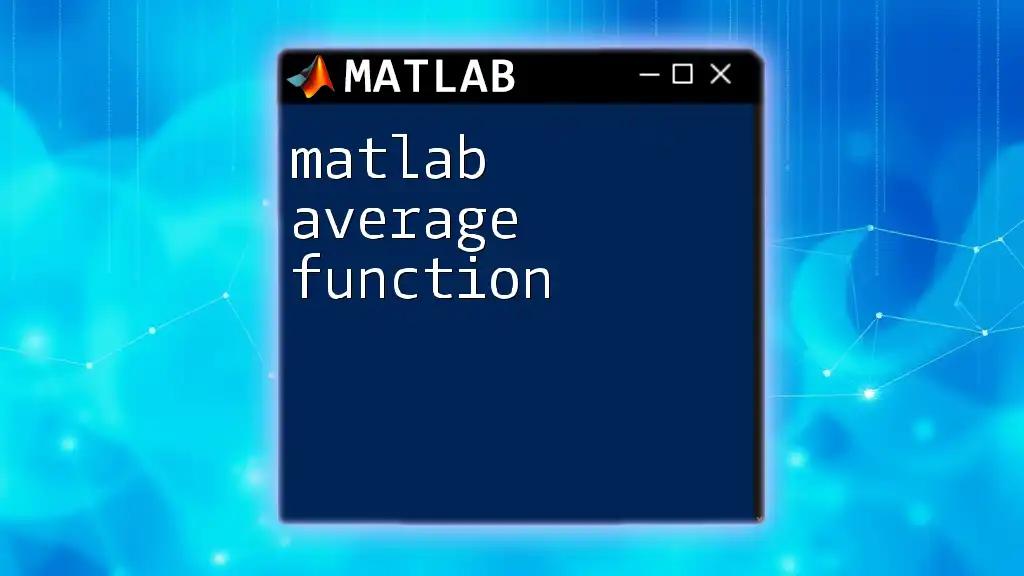
FAQs on Solving Systems of Equations in MATLAB
Feel free to post common questions or seek clarifications regarding any of the methods discussed in this guide. Engaging with your peers or community can enhance your learning experience.