MATLAB convolution is a mathematical operation that combines two sequences to produce a third sequence, representing how one sequence affects the other, which can be easily computed using the `conv` function.
Here’s a simple example demonstrating how to perform convolution in MATLAB:
% Define two input sequences
x = [1 2 3]; % First sequence
h = [0.5 1 0.5]; % Second sequence (filter)
% Perform convolution
y = conv(x, h);
% Display the result
disp(y);
What is Convolution?
Definition of Convolution
Convolution is a mathematical operation that combines two functions to form a third function. It expresses the way one function modifies another and is fundamental in various fields such as signal processing, image analysis, and control systems. In essence, convolution provides a means of filtering signals or images, which is crucial when analyzing or manipulating data.
Applications of Convolution
Convolution has numerous real-world applications. In audio signal processing, convolution can apply effects such as reverb or echo. In image processing, it is used for filtering operations, edge detection, and even blurring effects. For engineers and data scientists, understanding how to effectively use convolution in their work is vital for producing high-quality outputs.
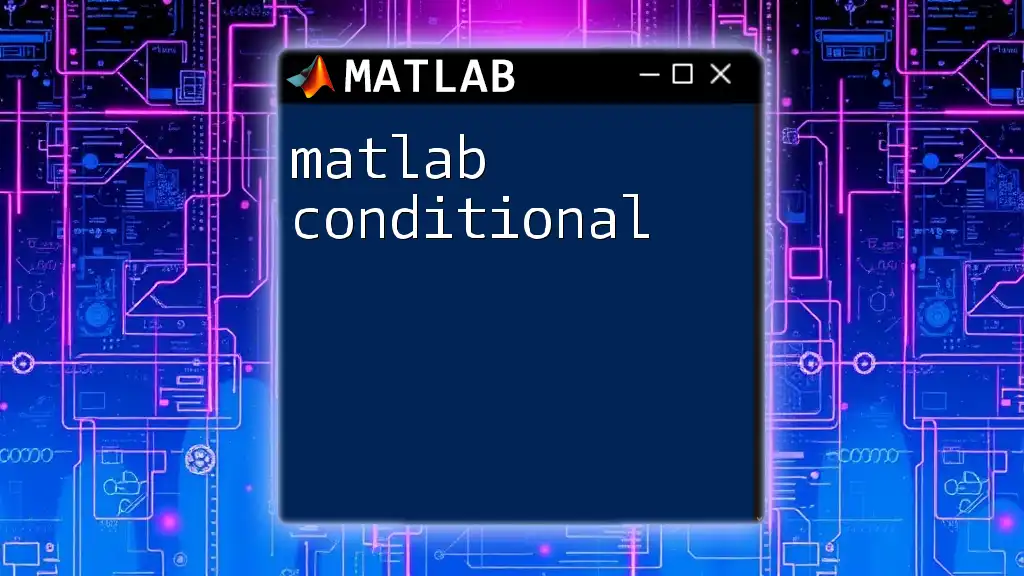
Theoretical Background of Convolution
Mathematical Representation
The convolution of two signals \( f(t) \) and \( g(t) \) is represented mathematically as:
\[ (f * g)(t) = \int_{-\infty}^{\infty} f(\tau) g(t - \tau) d\tau \]
In this equation, \( f(t) \) is typically the input signal, \( g(t) \) is the kernel or filter, and the resulting function represents the output signal. The convolution operation can be viewed as a means of applying a filter to a signal, adjusting the characteristics of the input based on the defined kernel.
Convolution Theorem
The Convolution Theorem states that convolution in the time domain is equivalent to multiplication in the frequency domain. This theorem is essential because it allows one to analyze signals efficiently by transforming them into the frequency domain where operations can be performed more easily. This principle is manifested in various applications such as Fast Fourier Transform (FFT) algorithms.
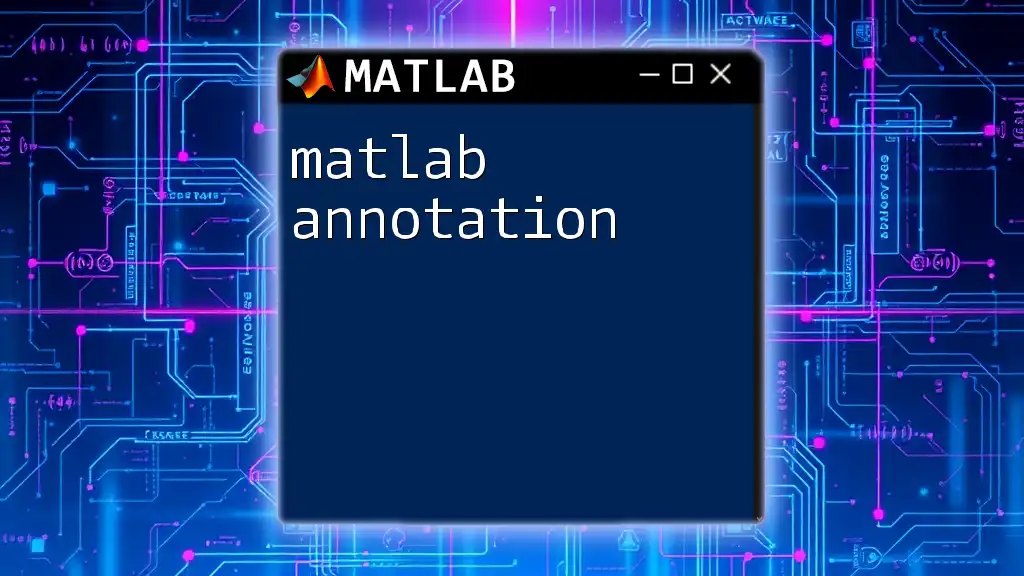
MATLAB’s Implementation of Convolution
Introduction to MATLAB Functions for Convolution
MATLAB provides several built-in functions for executing convolution operations, the most prominent being `conv`, `conv2`, and `filter`. Each of these functions serves different purposes and can be applied in varying contexts.
Syntax and Parameters
The `conv` Function
The `conv` function is typically used for 1D convolution. Its basic syntax is:
C = conv(A, B)
Here, `A` represents the first input vector (signal), and `B` is the second input vector (filter). This function computes the convolution of the two vectors and returns the result in `C`.
Example:
A = [1, 2, 3]; % Input signal
B = [0.2, 0.5, 0.3]; % Filter
C = conv(A, B); % Perform convolution
disp(C); % Displays the output
The `conv2` Function
The `conv2` function is designed for performing 2D convolutions, essential in image processing. It utilizes the same input syntax but operates specifically on matrices.
C = conv2(A, B)
Using `conv2`, one can apply a kernel to an image to filter it.
Example:
A = magic(3); % 3x3 matrix (image)
B = [1, 0; 0, -1]; % Simple kernel for edge detection
C = conv2(A, B); % Perform 2D convolution
disp(C); % Displays the filtered output
Advanced Functions Related to Convolution
The `filter` Function
The `filter` function is a powerful tool in MATLAB for applying digital filters represented by difference equations. Its syntax is as follows:
Y = filter(b, a, X)
Here, `b` contains the numerator coefficients of the filter, `a` contains the denominator coefficients, and `X` is the input signal.
Example:
b = [1, 0.5]; % Numerator coefficients
a = [1, -0.8]; % Denominator coefficients
X = randn(1, 10); % Random input signal
Y = filter(b, a, X); % Execute filtering operation
disp(Y); % Displays the filtered signal
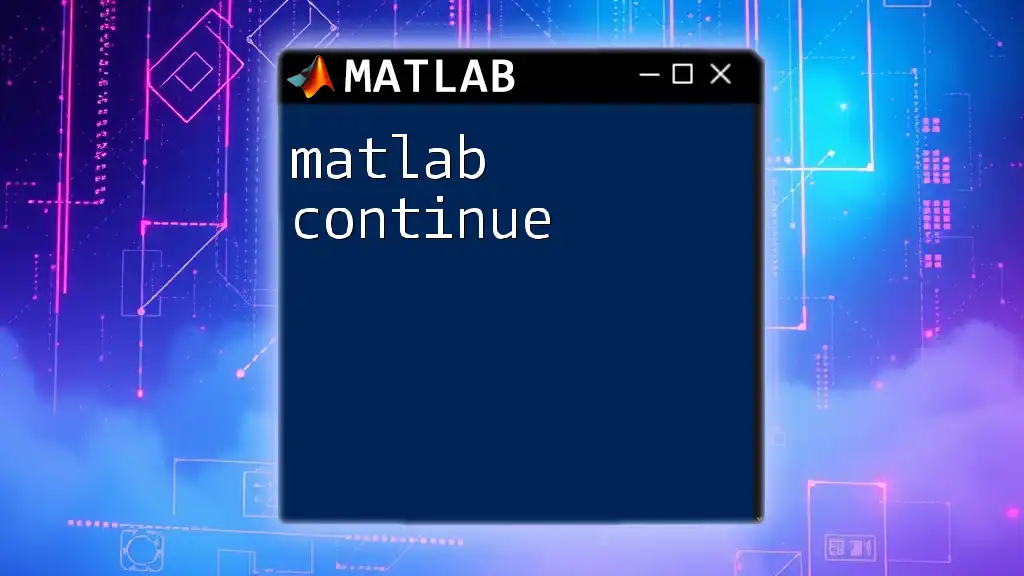
Visualizing Convolution Results
Plotting Convolution Outputs
Visual representation is pivotal in understanding the effects of convolution on signals. MATLAB offers comprehensive plotting capabilities, which can be utilized to compare the original and convolved signals.
Example:
t = 0:0.01:1; % Time vector
x = sin(2*pi*5*t); % Original signal (sinusoidal)
h = [0.2, 0.5, 0.2]; % Moving average filter
y = conv(x, h, 'same'); % Perform convolution with 'same' option
plot(t, x, t, y); % Plot original and convolved signals
legend('Original Signal', 'Convolved Signal'); % Legends for clarity
title('Convolution Result'); % Title for the plot
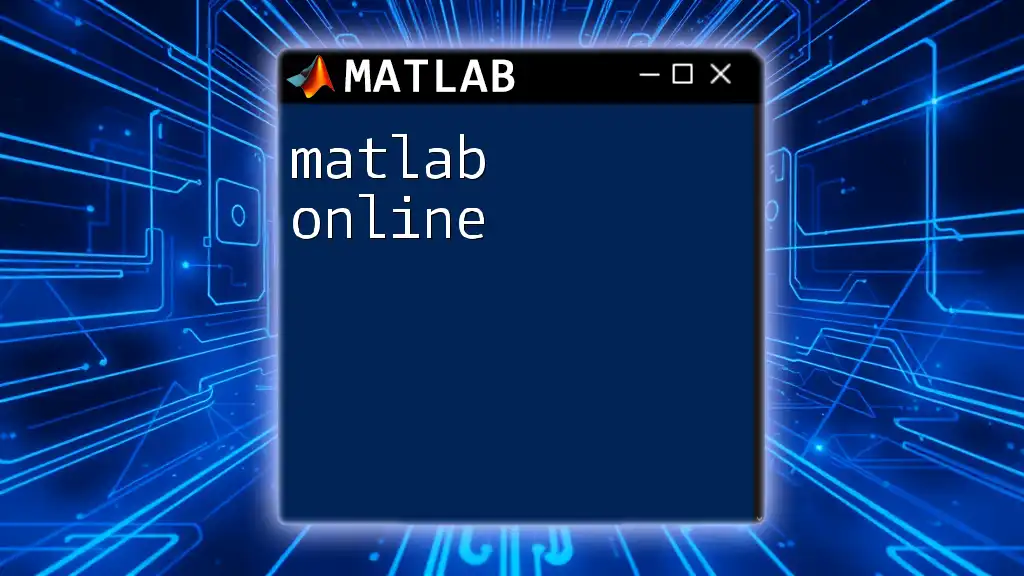
Convolution in Different Dimensions
1D Convolution Example
1D convolution is widely used for signals represented as one-dimensional arrays. It is vital to understand the nature of the signal and the kernel used. The convolution process sums the products of the overlapping values, thus allowing a detailed analysis of signal characteristics.
2D Convolution Example
2D convolution is frequently applied in image processing. It involves applying a filter (kernel) through every pixel of the image, effectively altering the image's properties such as edges and textures.
Example:
img = imread('image.png'); % Load an image
kernel = fspecial('sobel'); % Sobel edge detection filter
filtered_img = conv2(double(rgb2gray(img)), kernel, 'same'); % Apply filter
imshow(uint8(filtered_img)); % Display the filtered image
title('Filtered Image Using 2D Convolution'); % Title for the display
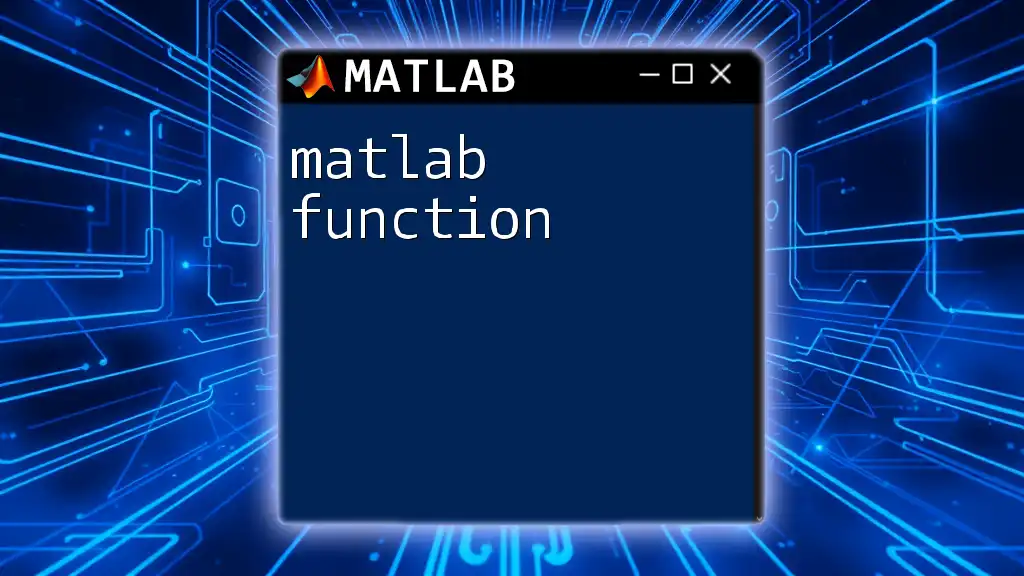
Tips for Effective Convolution in MATLAB
Choosing the Right Filter
The selection of the filter is crucial; a poorly chosen filter can degrade the quality of the output. Consider the application and the intended effect on the signal or image when choosing a filter.
Performance Considerations
When working with large datasets, the convolution process can become computationally expensive. To optimize performance, consider using the FFT method which allows you to achieve convolution in the frequency domain more effectively.
Common Errors and Troubleshooting
While implementing convolution, users may encounter common issues such as mismatched dimensions or unexpected results. Ensure that the input signals are appropriately sized and that the operation adheres to MATLAB's requirements. Checking for integration errors and debugging step-by-step in MATLAB can help identify problems.
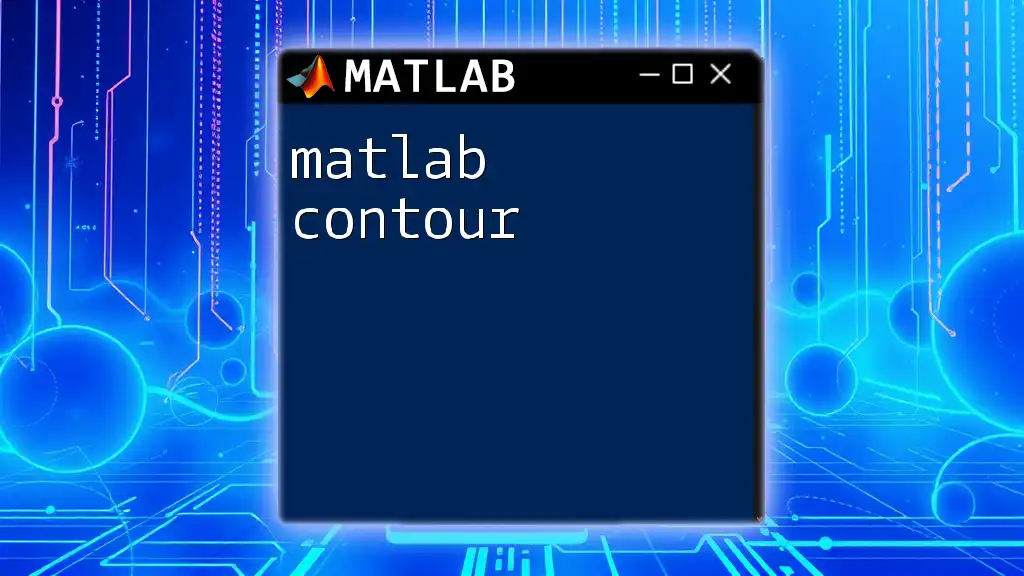
Conclusion
In summary, mastering MATLAB convolution is essential for effective signal and image processing. Understanding the theoretical background, practice with built-in functions, and visualizing results will enhance your ability to manipulate and analyze data effectively. Convolution is not only a powerful mathematical tool; it also forms the foundation for advanced data analysis techniques. Explore the capabilities of MATLAB, and don't hesitate to experiment with real datasets to solidify your understanding and skills in convolution operations.
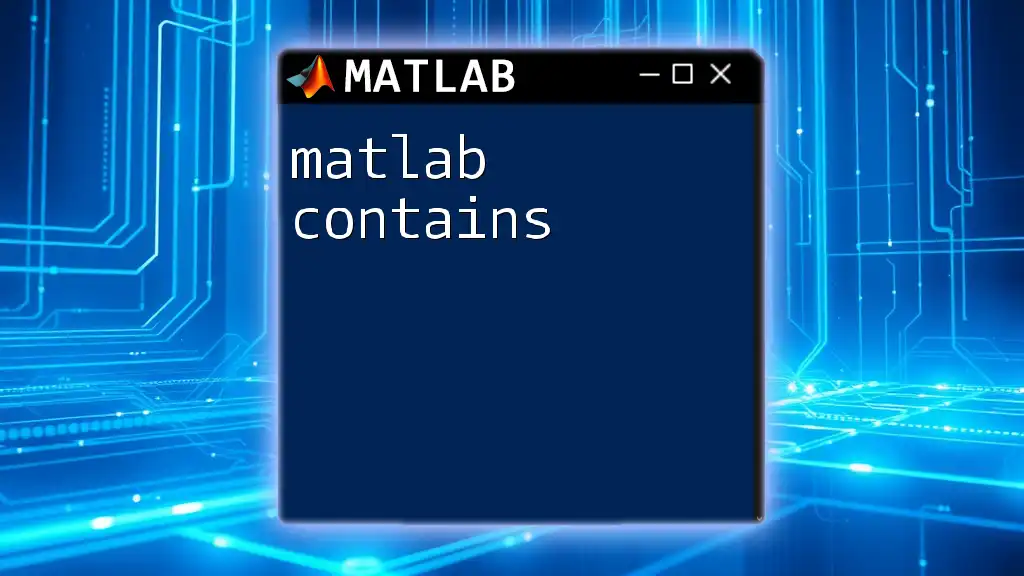
Additional Resources
Recommended Reading
To deepen your knowledge of convolution and its applications, explore texts on digital signal processing and MATLAB programming. Online courses can also provide practical, hands-on experience.
Communities and Forums
Engage with the MATLAB community through forums like MATLAB Central, where you can ask questions, share insights, and learn from experienced users.