The Pearson correlation in MATLAB measures the linear relationship between two sets of data, providing a value between -1 and 1 that indicates the strength and direction of the correlation.
Here’s a code snippet to compute the Pearson correlation coefficient between two vectors, `x` and `y`:
R = corrcoef(x, y);
Understanding Pearson Correlation
What is Pearson Correlation?
Pearson correlation measures the linear relationship between two datasets. It helps determine how closely the variables move in relation to one another. Understanding Pearson correlation is essential for anyone working with statistics and data analysis, as it enables insights into the strength and direction of relationships between variables.
It is crucial to acknowledge that correlation does not imply causation. While Pearson correlation can indicate a relationship, it does not confirm that changes in one variable cause changes in another.
The Pearson Correlation Coefficient
The Pearson correlation coefficient (often denoted as r) is calculated using the formula:
\[ r = \frac{cov(X, Y)}{\sigma_X \sigma_Y} \]
where:
- \( cov(X, Y) \) is the covariance of the variables \(X\) and \(Y\),
- \( \sigma_X \) and \( \sigma_Y \) are the standard deviations of the variables.
The coefficient ranges from -1 to 1:
- +1 indicates a perfect positive correlation,
- 0 indicates no correlation,
- −1 indicates a perfect negative correlation.
Visualizing this relationship through scatter plots can enhance understanding. A clustering of points in a linear pattern suggests a strong correlation, while a more dispersed pattern indicates a weaker relationship.
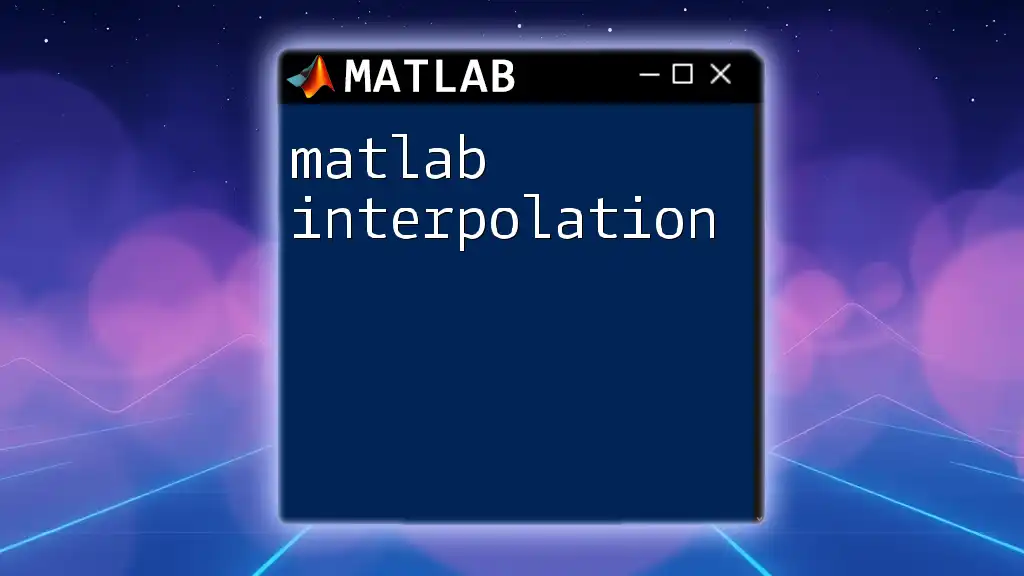
Getting Started with MATLAB
Setting Up MATLAB
To analyze data using MATLAB, you first need to install the software. Once installed, familiarize yourself with the MATLAB interface, which features the Command Window, Workspace, and Editor. Become comfortable using MATLAB commands to navigate through data analysis processes.
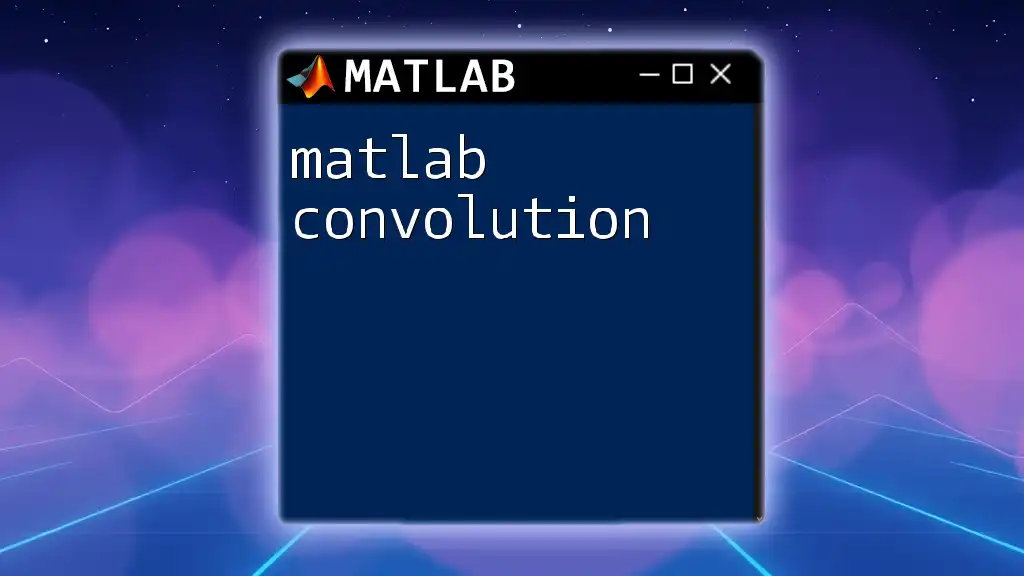
Steps to Compute Pearson Correlation in MATLAB
Preparing Your Data
Before you start computing Pearson correlation in MATLAB, data preparation is crucial. Ensure that you have clean datasets ready for analysis. Here’s an example of creating two simple datasets:
% Example data
x = [1, 2, 3, 4, 5];
y = [2, 3, 4, 5, 6];
Using MATLAB Built-in Functions
Correlation Coefficient Function
The `corrcoef()` function in MATLAB computes the Pearson correlation coefficient for two variables. Its syntax is straightforward, and it provides an output matrix displaying the correlation between each pair of variables:
% Calculate the correlation coefficient
R = corrcoef(x, y);
disp(R);
The output matrix will display the correlation coefficients. In this case, if \(R(1,2)\) equals 1, it indicates a perfect positive correlation between \(x\) and \(y\).
Visualizing Correlation
Scatter Plots
Visualizing your data enhances interpretation of correlations. Scatter plots are an excellent way to illustrate relationships between datasets. Here's how to create a scatter plot in MATLAB:
% Creating a scatter plot
scatter(x, y);
xlabel('X Data');
ylabel('Y Data');
title('Scatter Plot of X vs Y');
grid on;
In this example, the x-axis represents the variable \(x\) and the y-axis represents \(y\). The title and labels aid in understanding the plot. Look for linear trends in the scatter plot that indicate correlation.
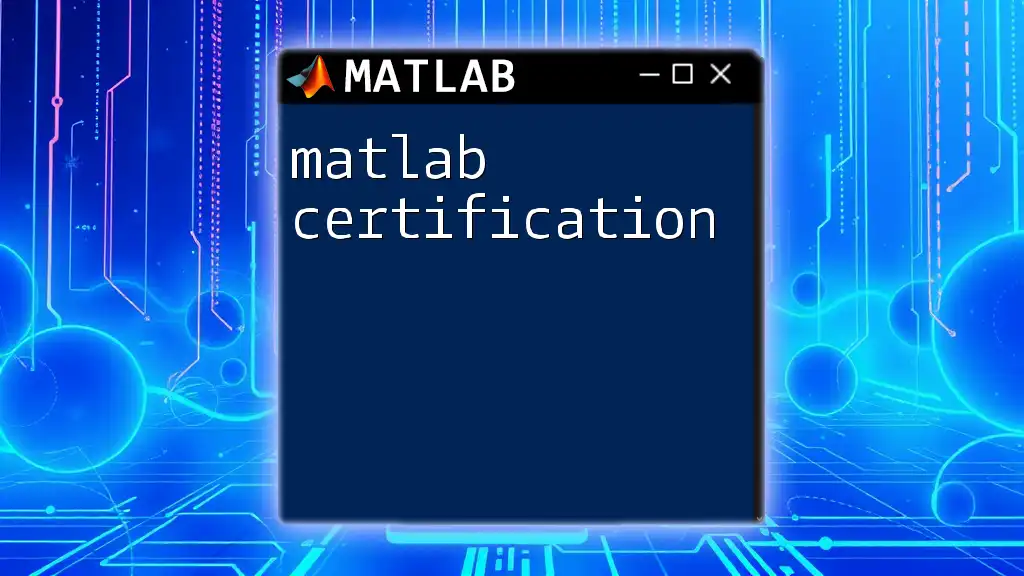
Advanced Topics
Handling Multiple Variables
When analyzing data with more than two variables, calculating pairwise correlations might be necessary. The `corrcoef()` function can also be applied to matrices. Here's a step-by-step example:
% Example with multiple variables
data = [1, 2, 3; 5, 6, 7; 9, 10, 11];
R_multi = corrcoef(data);
disp(R_multi);
This code will display a correlation matrix where each cell shows the correlation coefficient between pairs of variables in the matrix.
Dealing with Missing Data
In many real-world datasets, handling missing data is a common challenge. In MATLAB, functions such as `nanmean` and `nanstd` ignore `NaN` values, allowing effective analysis even when data is missing. Here's how to compute correlation while ignoring missing values:
% Example with missing values
x_with_nan = [1, 2, 3, NaN, 5];
y_with_nan = [2, NaN, 4, 5, 6];
corr_with_nan = corrcoef(x_with_nan, y_with_nan, 'Rows', 'complete');
disp(corr_with_nan);
Utilizing the `'Rows', 'complete'` option ensures that only complete cases are analyzed, providing a clear correlation coefficient despite gaps in the data.
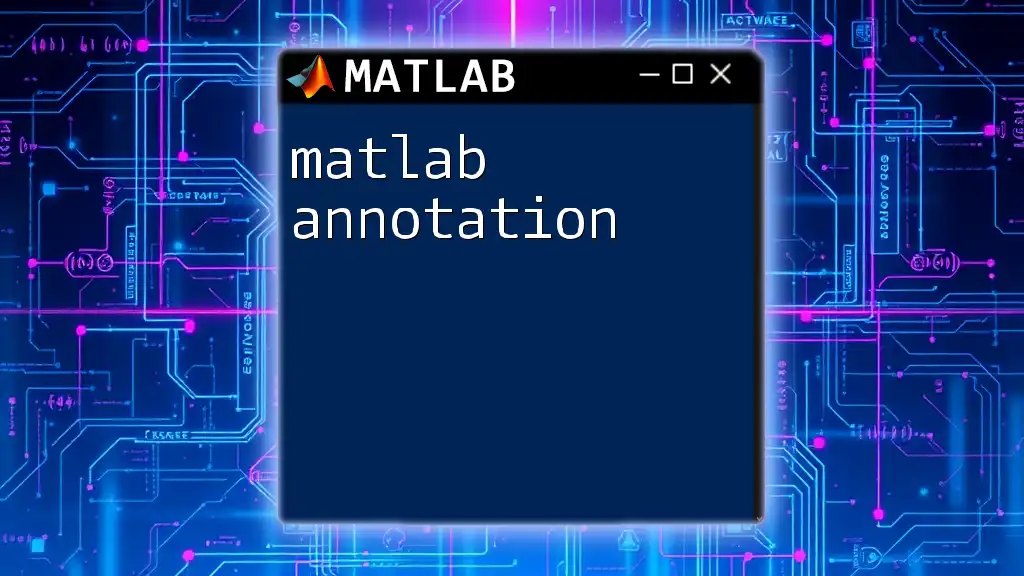
Applications of Pearson Correlation
Fields and Use Cases
Pearson correlation is widely applied across fields such as finance, where it can assess the relationship between stock prices. In biology, researchers may analyze gene expression data to find correlations between genes. Social sciences also utilize Pearson correlation to understand relationships among survey responses.
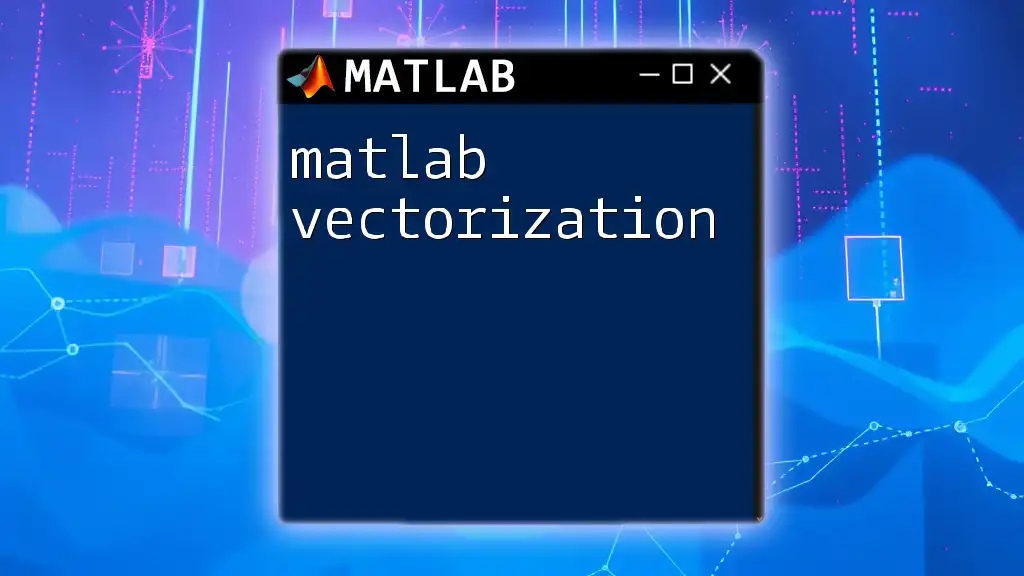
Common Mistakes and Misinterpretations
Misunderstanding Correlation
It is essential to recognize the limitations of Pearson correlation. It may suggest a relationship where none exists (false positives) and may fail to capture nonlinear relationships. Causation cannot be inferred solely based on correlation; a more comprehensive analysis must follow.
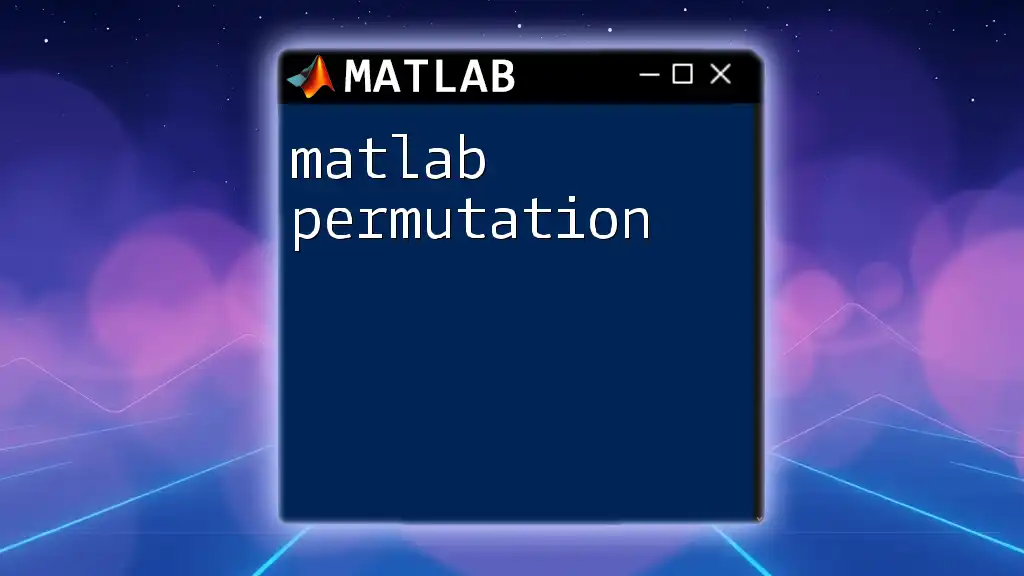
Conclusion
Pearson correlation, as computed in MATLAB, is a powerful statistical tool for analyzing relationships between datasets. By understanding this correlation, you can derive meaningful insights from data while acknowledging its limitations. Experimenting with code examples and visualizations will deepen your understanding and enhance your analytical skills.
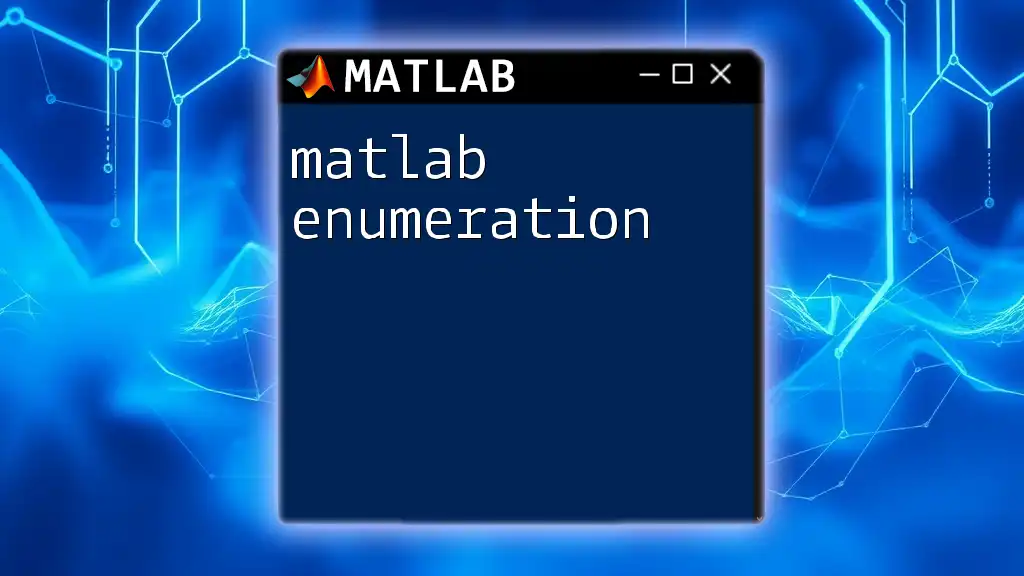
Additional Resources
Further Reading and Tools
For further mastery of MATLAB and statistical analysis, explore the [MATLAB documentation on correlation](https://www.mathworks.com/help/stats/correlation.html). Here, you will find extensive resources and examples that can enhance your learning.
Community Support and Forums
Engaging with the MATLAB community is an excellent way to continue your education. Consider joining platforms like [MATLAB Central](https://www.mathworks.com/matlabcentral/) to connect with other users, share ideas, and seek assistance.
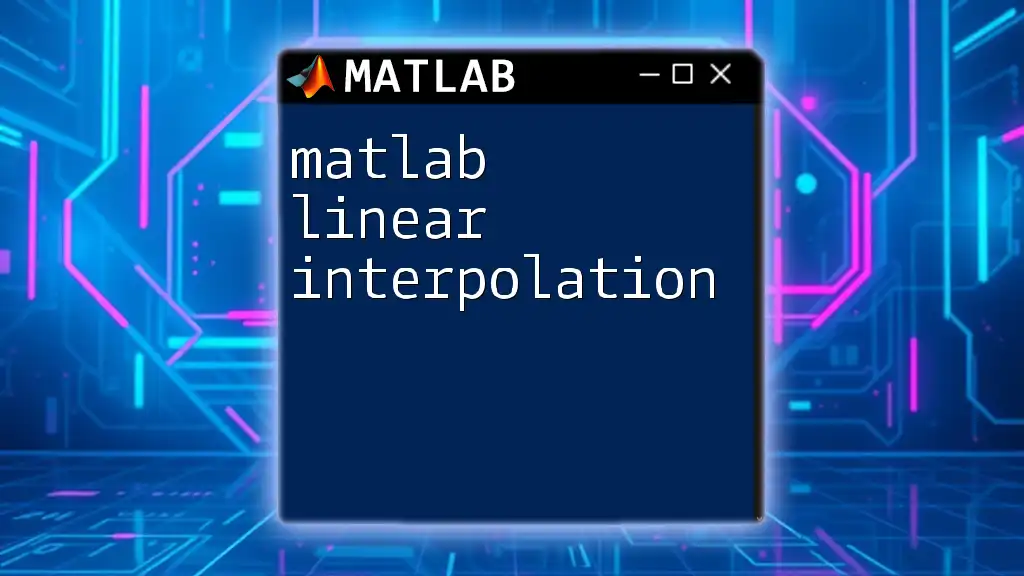
Call to Action
Take the plunge and practice using the MATLAB commands covered in this article. Challenge yourself to analyze different datasets and uncover interesting correlations. Share your insights or pose questions in the comments section, and let’s foster a community of learning together!