The MATLAB `integrate` function is used for performing numerical integration on functions, allowing users to compute definite and indefinite integrals efficiently.
Here's a simple code snippet demonstrating the use of the `integrate` function in MATLAB:
syms x; % Define the variable
f = x^2; % Define the function
integral_value = int(f, x); % Calculate the indefinite integral
disp(integral_value); % Display the result
Understanding the Integrate Function in MATLAB
What is Integration?
Integration is a fundamental concept in mathematics that involves calculating the area under a curve. It can be categorized into two types: definite integrals, which compute the area between two specific limits, and indefinite integrals, which provide a general form of the area without specified limits. Integration plays a vital role in various fields such as physics, engineering, and economics, helping to determine quantities like distances, areas, volumes, and total accumulated values.
The Role of MATLAB in Integration
MATLAB, a powerful computing environment, simplifies the process of performing complex integral calculations. It offers a range of built-in functions that allow users to tackle both symbolic and numeric integration seamlessly, making it a go-to tool for engineers and scientists.
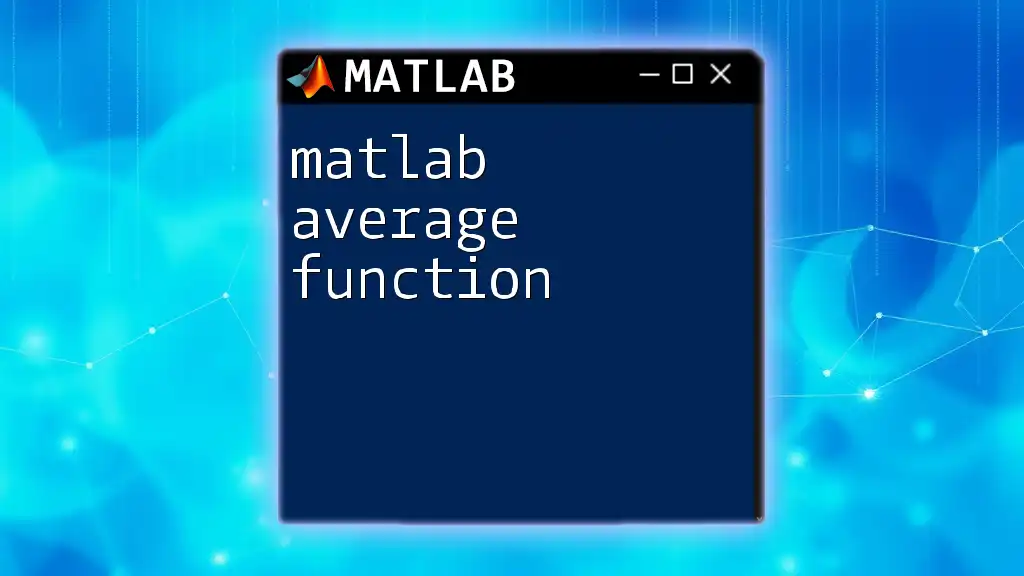
Using the integrate Function in MATLAB
Syntax and Structure
The syntax for the integrate function is structured as follows:
result = integrate(fun, var, limits)
In this syntax:
- fun is the function you want to integrate.
- var is the variable with respect to which you're integrating.
- limits specifies the limits of integration.
Types of Integrals in MATLAB
Indefinite Integrals
Indefinite integrals provide a general formula for the antiderivative of a function without specific limits. In MATLAB, this can be accomplished using the `int` function.
Here is an example code snippet to calculate the indefinite integral of a polynomial function:
syms x
f = x^2 + 3*x + 2;
indefinite_integral = int(f, x);
disp(indefinite_integral);
Upon execution, MATLAB will return the indefinite integral in symbolic form. Understanding this output is crucial, as it represents a family of functions whose derivative is the original function f(x).
Definite Integrals
Definite integrals, on the other hand, calculate the area under a curve between specified limits. This is also done using the `int` function, but with specified lower and upper bounds.
Here’s an example of how to compute a definite integral:
definite_integral = int(f, x, 0, 2);
disp(definite_integral);
In this case, MATLAB evaluates the polynomial function from 0 to 2, providing a numeric output that represents the shaded area under the curve between those limits.
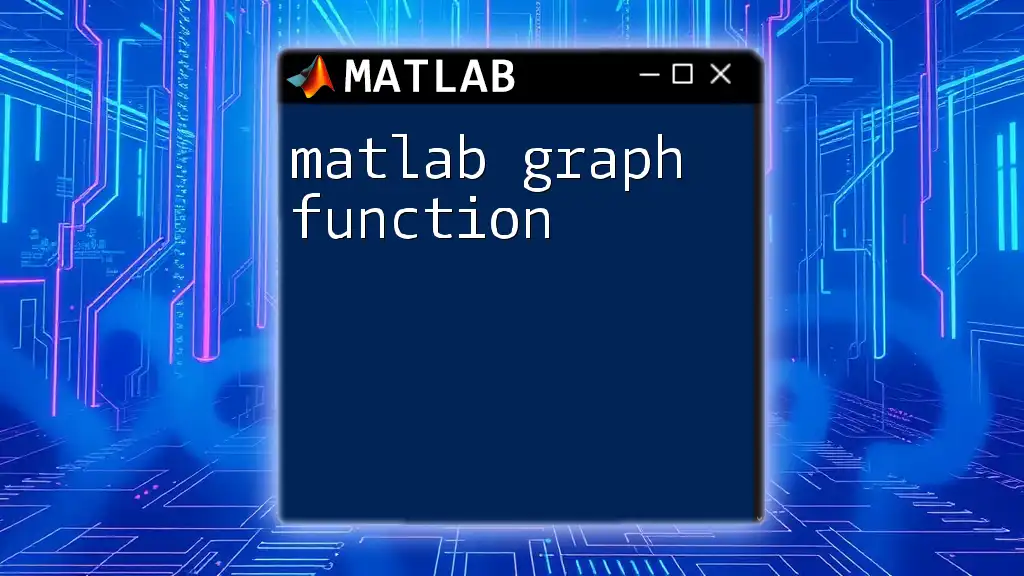
Advanced Features of the Integrate Function
Numerical Integration
Importance of Numerical Methods
There are instances where symbolic integration is inefficient or impossible, particularly with complex functions or when an explicit solution does not exist. In such cases, MATLAB provides numerical integration capabilities that can yield approximate results with high accuracy.
Example Code for Numerical Integration
The `integral` function is often employed for numerical integration. Here’s an example:
f_numeric = @(x) sin(x) ./ x;
numeric_result = integral(f_numeric, 0, 10);
disp(numeric_result);
This code approximates the integral of \( \frac{\sin(x)}{x} \) from 0 to 10. The output gives a numerical value representing the area under the curve over that interval, providing an effective and fast method to solve integrals that might otherwise resist analytical solutions.
Integration with Multivariable Functions
Double Integrals
Double integrals are crucial when dealing with functions of two variables, allowing for the computation of volumes under surfaces.
Here’s how to compute a double integral in MATLAB:
syms x y
f_double = x*y;
double_integral = int(int(f_double, y, 0, 1), x, 0, 1);
disp(double_integral);
This code calculates the volume under the surface defined by \( f(x, y) = xy \) over the unit square. The first `int` computes the integral with respect to y, while the outer `int` integrates with respect to x.
Triple Integrals
Similarly, triple integrals extend the concept to three dimensions, which can be valuable in diverse applications such as calculating volumes of solids in physical sciences.
Here’s an example code snippet for calculating a triple integral:
syms x y z
f_triple = x*y*z;
triple_integral = int(int(int(f_triple, z, 0, 1), y, 0, 1), x, 0, 1);
disp(triple_integral);
In this case, MATLAB calculates the volume underneath the body defined by the function \( f(x, y, z) = xyz \) in the bounds given.
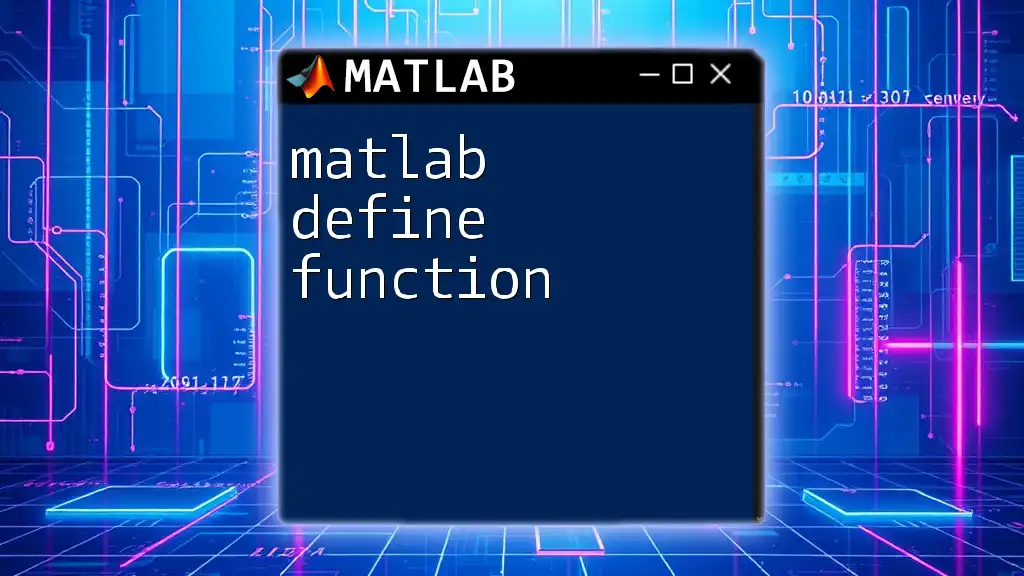
Tips for Effective Use of the Integrate Function
Common Pitfalls
While using the MATLAB integrate function, users should be cautious about:
- Improper limits of integration, which can lead to incorrect results.
- Function definitions that may not be entirely specified or are overly complex for MATLAB to handle symbolically.
Best Practices
To maximize your efficiency with the matlab integrate function:
- Choose wisely between symbolic and numerical integration: Use symbolic methods when possible for exact results, but switch to numerical methods for complex or unsolvable integrals.
- Verify your results: Whenever feasible, cross-check the outputs with known integrals or perform graphical analysis.
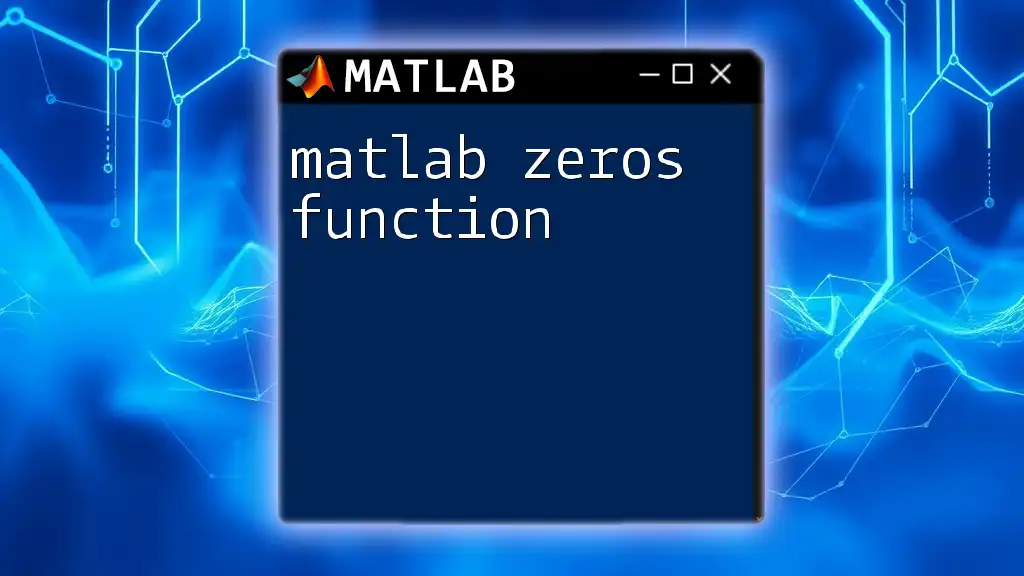
Real-World Examples of Integration using MATLAB
Engineering Applications
Consider the task of calculating the area under the curve that represents the load distribution on a beam. By applying the matlab integrate function, engineers can derive critical insights about the structural performance.
Scientific Applications
In physics, integration might be used to compute the total electric field in a region influenced by distributed charges. The matlab integrate function effectively simplifies these complex calculations, allowing for precise results.
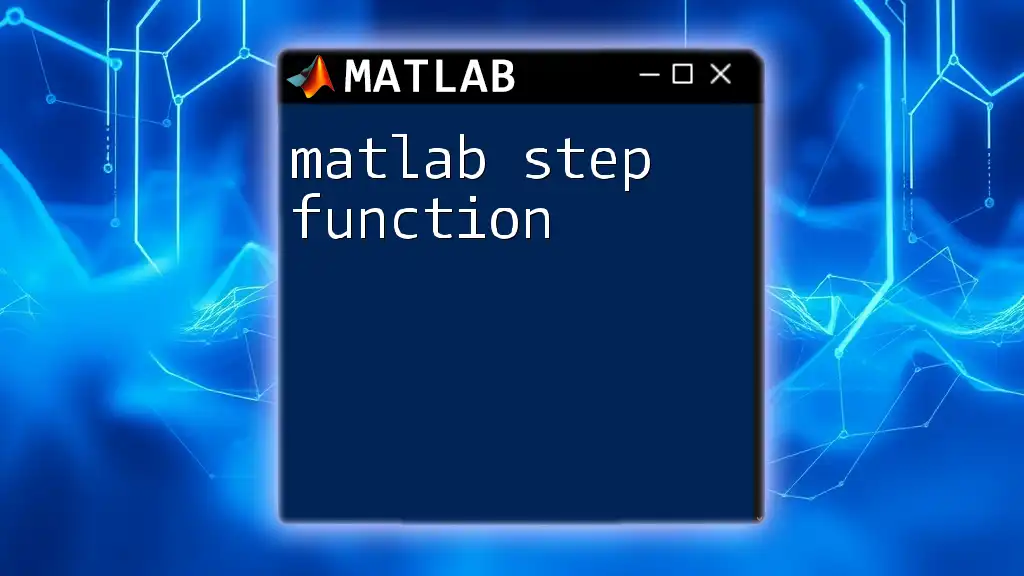
Conclusion
In conclusion, the matlab integrate function is an invaluable asset for those engaged in mathematical, engineering, and scientific computations. Its ability to perform both symbolic and numerical integration makes it essential for a wide variety of applications. Practicing with the examples provided will solidify your understanding and enhance your MATLAB skills. As you explore further, you'll uncover even more capabilities of MATLAB that will revolutionize how you approach calculus and integration challenges.
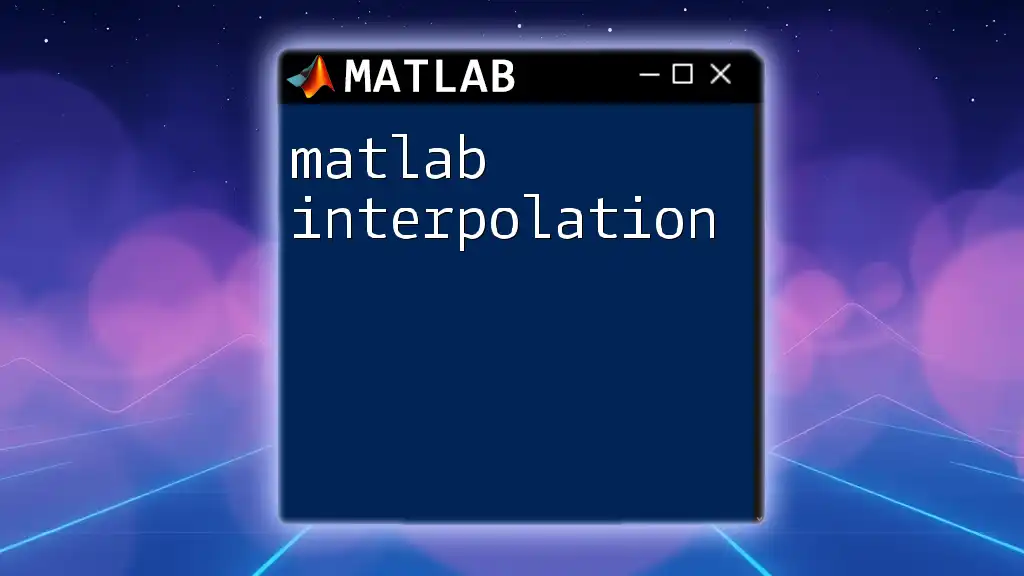
Additional Resources
For deeper exploration, refer to the official MATLAB documentation on integration. Additionally, consider recommended books and online courses that offer more context and exercises related to the matlab integrate function.