To solve a system of equations in MATLAB, you can use the backslash operator (\) for efficient computation, as demonstrated in the following code snippet:
A = [2 1; 5 7]; % Coefficient matrix
b = [11; 13]; % Right-hand side vector
x = A\b; % Solve for x
Understanding Systems of Equations
Definition of a System of Equations
A system of equations consists of multiple equations that share common variables. The objective is to find values for these variables that satisfy all equations simultaneously. Systems can be classified into two broad categories:
- Linear Systems: These consist of linear equations. The solution can be visualized as the intersection of straight lines in a graph.
- Nonlinear Systems: These involve at least one nonlinear equation. The solution may not always be straightforward, as the curves can intersect at multiple points or not at all.
Graphical Interpretation
Graphically, the solution to a system of equations is represented by the points where the curves or lines intersect. For example, if two lines intersect at a single point, that point represents the only solution to the system. Understanding this graphical representation is critical for comprehending the nature of the solutions, which can include:
- A single solution (consistent and independent).
- No solution (inconsistent).
- Infinitely many solutions (dependent).

Methods for Solving Systems of Equations in MATLAB
Analytical Methods
Symbolic Computation using the `syms` Function
In cases of linear equations, MATLAB allows for symbolic computations, which enable you to express and manipulate equations algebraically. The `syms` function initializes symbolic variables, allowing you to solve equations symbolically.
syms x y
eq1 = x + y == 10;
eq2 = 2*x - y == 3;
sol = solve([eq1, eq2], [x, y]);
disp(sol);
In this code snippet, we define two equations (`eq1` and `eq2`) and use the `solve` function to find the values of `x` and `y`. When you run this code, MATLAB will output the solutions for the variables, helping you understand how to manipulate symbolic data.
Numerical Methods
Using Matrix Algebra
Most systems can be expressed using matrices, making the solution process efficient. To represent a system using matrix algebra, we can rewrite it in the form Ax = B, where A is the coefficients matrix, x is the variable matrix, and B is the constants matrix.
A = [1 1; 2 -1]; % Coefficient matrix
B = [10; 3]; % Constants matrix
X = A\B; % Solving for X
disp(X);
In this example, the matrix A represents the coefficients of the equations, and B contains the constants. The backslash operator (`\`) in MATLAB serves to find the solution vector X efficiently. This approach can be highly efficient for larger systems.
Built-in Functions
- Using `linsolve` for Solving Linear Systems
The `linsolve` function in MATLAB is another powerful method for solving linear systems. It is particularly useful when you need to maintain numerical stability.
X = linsolve(A, B);
disp(X);
This code snippet performs a similar operation as before, solving the system using a built-in MATLAB function. This method is especially favorable for its robustness, and it can be faster than using the backslash operator under certain conditions.
Alternative Methods
Using `fsolve` for Nonlinear Equations
For solving nonlinear systems, MATLAB's `fsolve` function is the go-to method. It approaches the problem numerically, and a good initial guess can significantly affect its success.
fun = @(x) [x(1)^2 + x(2)^2 - 1; x(1) - x(2)];
x0 = [0, 0];
solution = fsolve(fun, x0);
disp(solution);
In this example, we define a nonlinear system involving a circle and a line. The function `fun` encapsulates these equations. The initial guess `x0` helps `fsolve` converge to a solution.
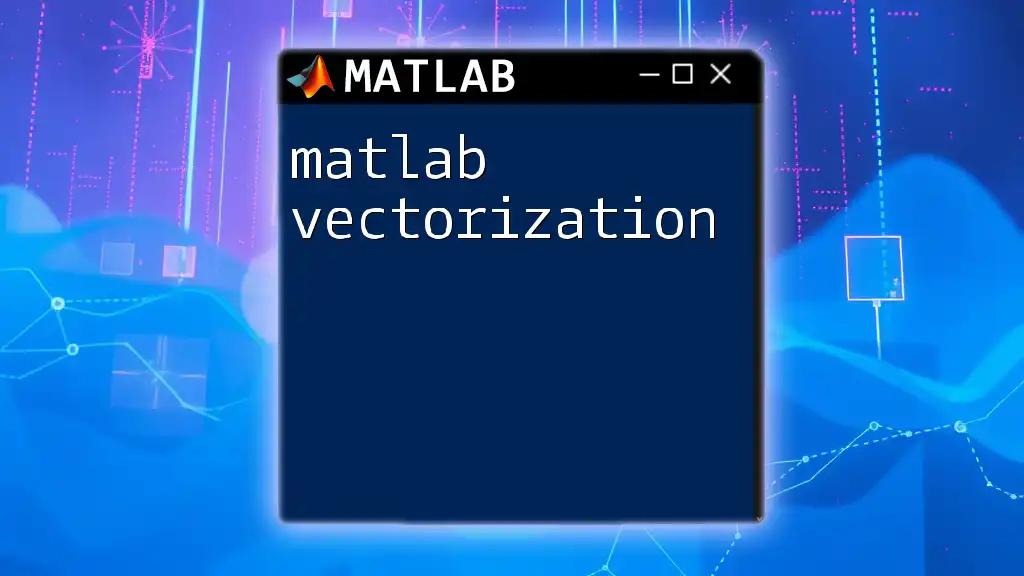
Practical Examples
Example: Solving a Linear System
Let's consider an example where you are tasked with solving a system of linear equations—a common situation in electrical circuit analysis.
A = [2 3; 4 -1];
B = [5; 11];
X = A\B;
disp(X);
In this scenario, we define the coefficient matrix A and the constants matrix B. The result stored in X represents the voltage across different components in the circuit.
Example: Using `fsolve` for a Nonlinear System
Suppose you need to find points of intersection between a circle and a linear function, which is applicable in many fields like computer graphics.
fun = @(x) [x(1)^2 + x(2)^2 - 1; x(1) - x(2)];
x0 = [0, 0];
solution = fsolve(fun, x0);
disp(solution);
This example highlights how to set up nonlinear equations and find their solution using numerical methods.
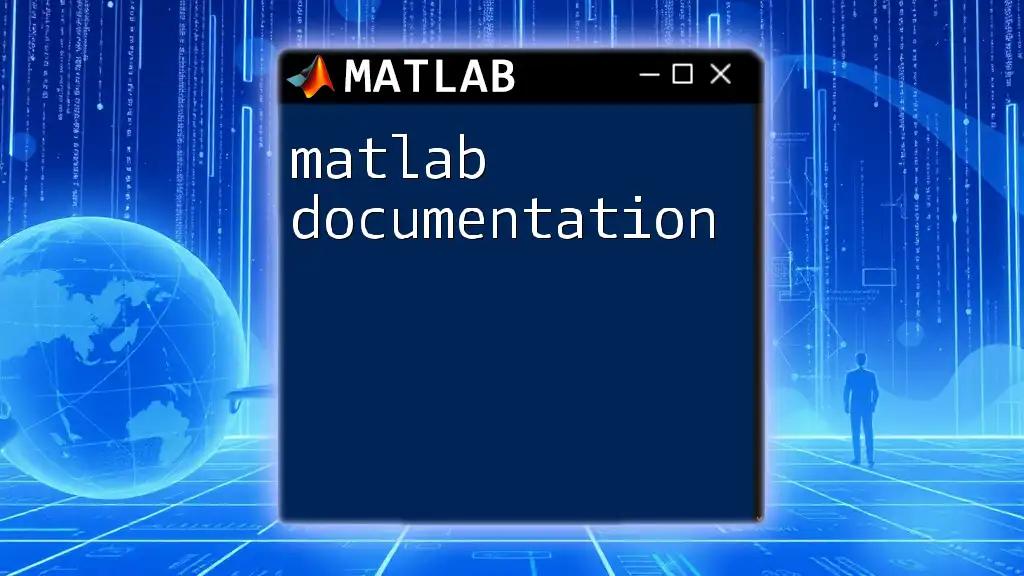
Best Practices and Tips for Solving Systems of Equations
Ensuring Accurate Solutions
After obtaining a solution, it's prudent to check its validity by substituting the values back into the original equations. This step ensures that the calculated solution satisfies all equations, preserving accuracy.
Handling Inconsistent Systems
Should you encounter systems that yield no solutions (inconsistent), employing different methods such as examining the rank of the coefficient matrix can help. MATLAB’s `rank()` function assists in identifying issues related to inconsistencies.
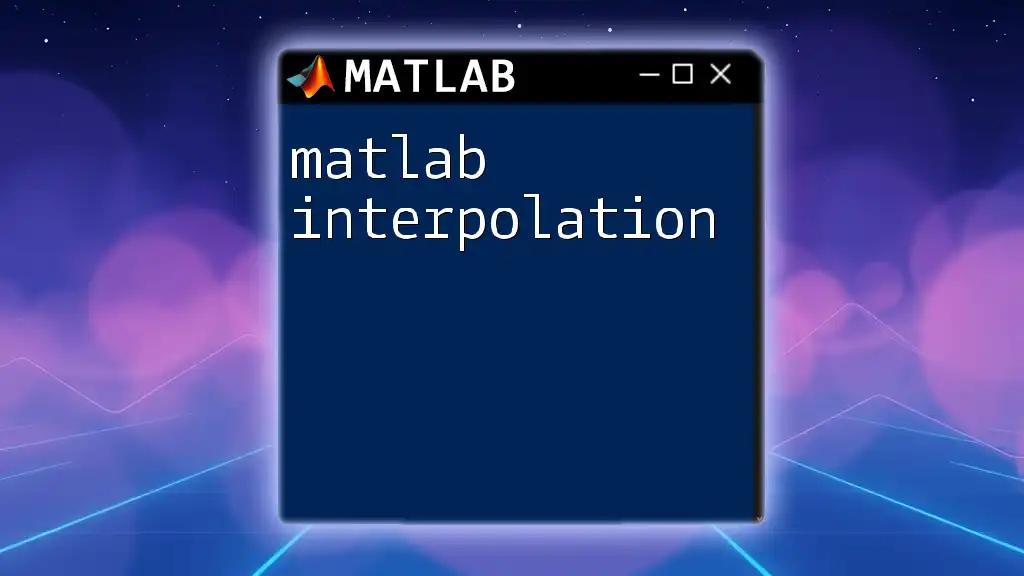
Conclusion
In summary, MATLAB provides a comprehensive suite of tools for solving systems of equations, whether linear or nonlinear. By mastering functions like `solve`, matrix operations, `linsolve`, and `fsolve`, you empower yourself to tackle complex mathematical challenges effectively. The best way to enhance your understanding is to practice these concepts.
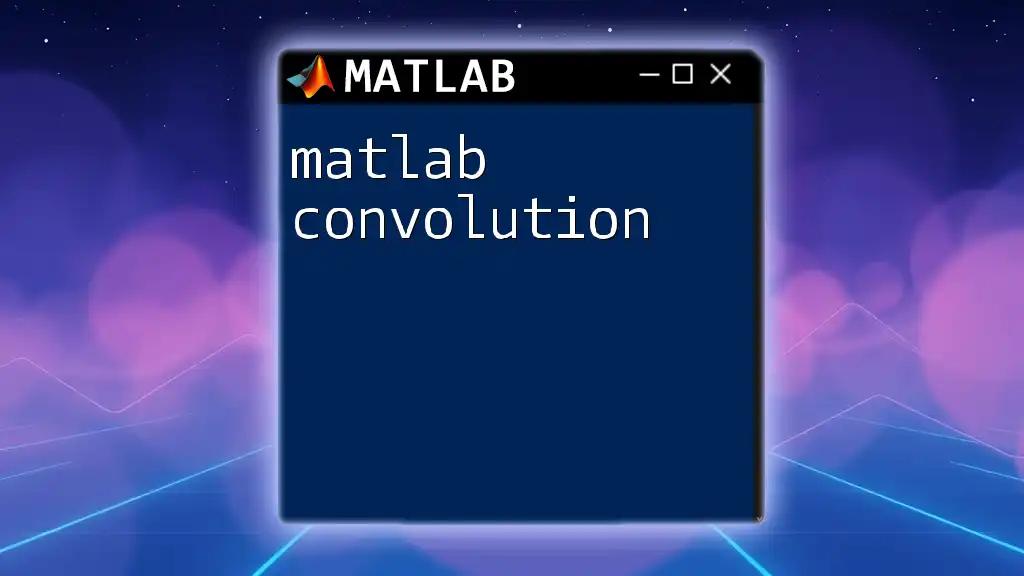
Resources
To further your knowledge, explore the official MATLAB documentation and community forums. Engaging in exercises and tackling real-world problems will reinforce your proficiency in using MATLAB to solve systems of equations.
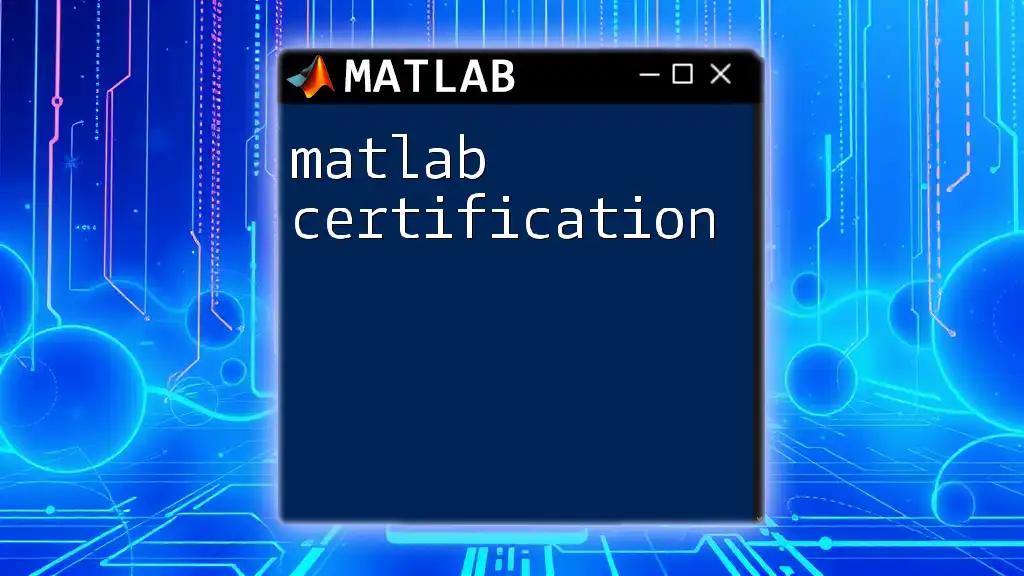
Call to Action
If you have experiences or questions regarding solving systems of equations in MATLAB, feel free to share them in the comments below. Your insights could benefit others on their MATLAB journey!