In MATLAB, the "less than or equal to" operator (<=) is used to compare two values and returns true if the left value is less than or equal to the right value.
Here’s a simple code snippet demonstrating its use:
% Example of using the less than or equal to operator
a = 5;
b = 10;
result = a <= b; % This will return true (1) since 5 is less than 10
Understanding the Basics of Comparison Operators
What Are Comparison Operators?
Comparison operators are fundamental components of programming that allow you to evaluate the relationship between two values. In the context of MATLAB, these operators can be used to create conditions within your code that drive logical decisions or data filtering. The primary comparison operators available in MATLAB include:
- Less than (`<`)
- Greater than (`>`)
- Less than or equal to (`<=`)
- Greater than or equal to (`>=`)
- Equal to (`==`)
- Not equal to (`~=`)
The Less Than or Equal To Operator: `<=`
The less than or equal to operator, represented as `<=`, checks if the value on the left is either less than or equal to the value on the right. If this condition is true, it returns a logical value of `1` (true); otherwise, it returns `0` (false). This operator is crucial in various programming scenarios, particularly when making decisions based on numerical comparisons.
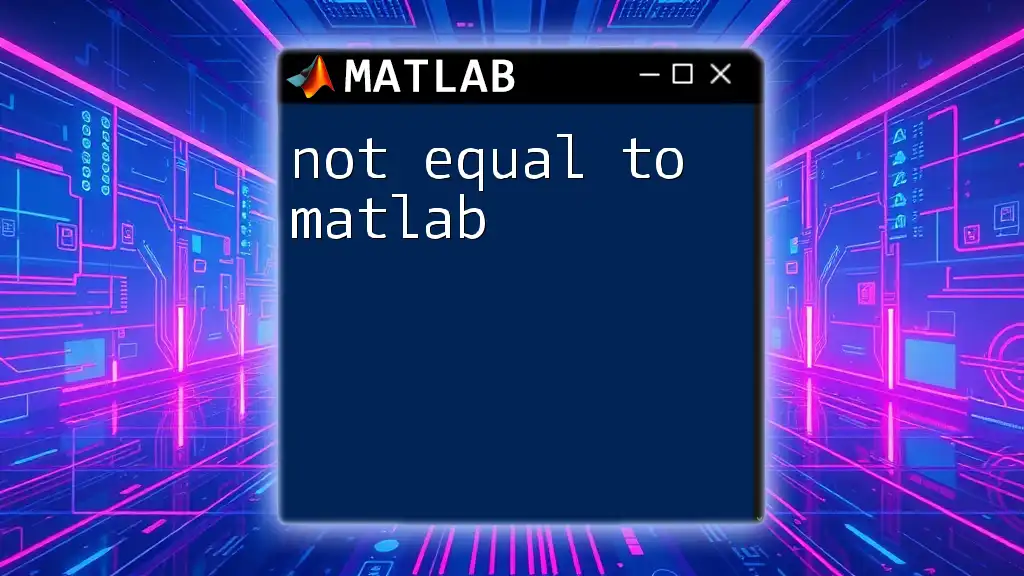
Syntax and Usage of `<=`
Basic Syntax Explained
The general syntax for the less than or equal to operator is simply:
a <= b
Here, `a` and `b` can be scalars, arrays, or symbolic variables. This relative simplicity enables a wide range of applications within scripts, functions, or any MATLAB code blocks.
Types of Operands
Scalars
To illustrate the basic use of the `<=` operator with scalars, consider the following example:
result = 5 <= 10; % result will be true (1)
In this instance, since 5 is indeed less than 10, MATLAB returns true.
Arrays
The capability of the `<=` operator to handle arrays allows for simultaneous comparisons. For instance:
A = [1, 2, 3];
B = [2, 2, 4];
result = A <= B; % result will be [1 1 1]
In this example, every element of array A is compared to the corresponding element of array B, yielding a logical array where each element reflects the result of the comparison.
Mixed Types
The `<=` operator can also compare numeric values with logical values. For example:
x = true; % logical 1
y = 1; % numeric 1
result = x <= y; % result will be true (1)
Here, MATLAB treats `true` as `1`, enabling a valid comparison.
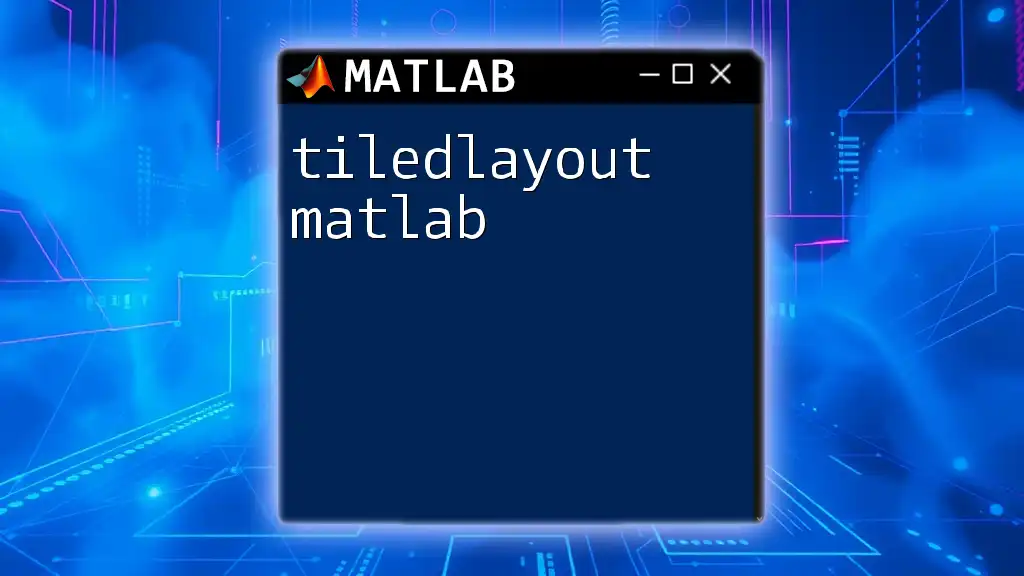
Practical Applications of the Less Than or Equal To Operator
Filtering Data
One of the most practical applications of the `<=` operator in MATLAB is data filtering. For example, if you have an array and wish to extract the elements that meet a specific criterion, you can do so efficiently:
data = [1, 3, 5, 7, 9];
filteredData = data(data <= 5); % result will be [1, 3, 5]
In this snippet, only the elements of `data` that are less than or equal to 5 are retained in `filteredData`.
Conditional Statements
Using `<=` in If Statements
The `<=` operator is commonly used in conditional statements, such as `if` clauses, to dictate the flow of your program based on conditions. For example:
value = 4;
if value <= 5
disp('Value is less than or equal to 5');
end
This will display a message if the condition is true.
Utilizing `<=` in Loops
Loops often leverage the `<=` operator to control iterations. For instance, you can employ it within a `for` loop to check values:
for i = 1:10
if i <= 5
disp(i);
end
end
This loop will output numbers from 1 to 5, as the condition filters out values greater than 5.
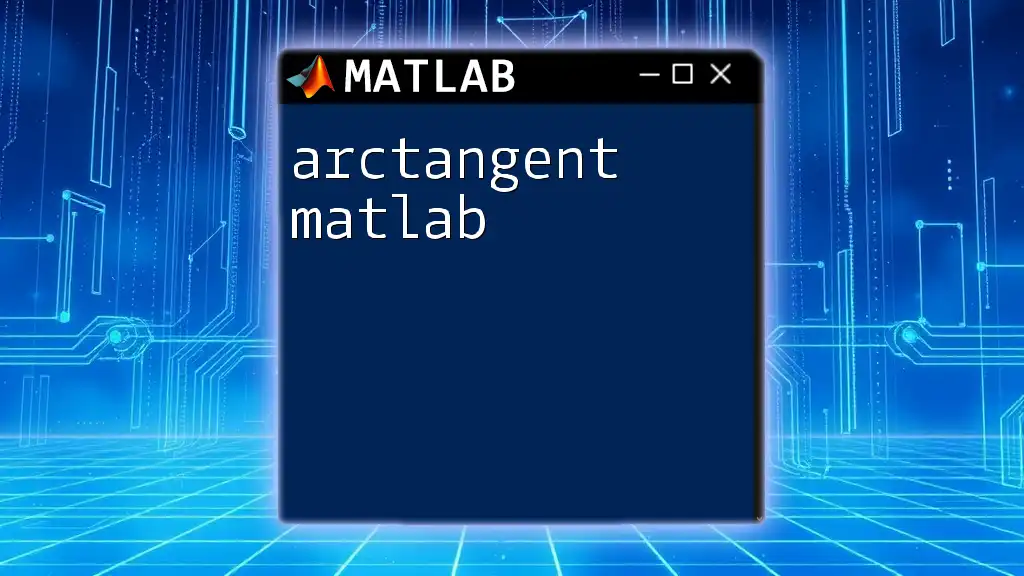
Advanced Applications of `<=`
Logical Indexing
Another excellent use of the `<=` operator is through logical indexing, which simplifies the process of selecting elements from arrays or matrices based on a condition. Consider the following example:
matrix = [0, 1, 2; 3, 4, 5];
index = (matrix <= 2);
selectedElements = matrix(index); % extracts elements less than or equal to 2
In this case, the condition generates a logical array, which is then used to filter the original `matrix`, yielding only the elements that satisfy the condition.
Using `<=` with Functions
In Built-in Functions
The `<=` operator can also be integrated into built-in MATLAB functions for enhanced functionality. For example, using the `sum` function to count how many elements meet the specified condition can be done as follows:
values = [1, 2, 3, 4];
sumResult = sum(values <= 2); % returns count of elements <= 2
This effectively counts how many elements in the `values` array are less than or equal to 2, demonstrating the versatility of `<=` in MATLAB functions.
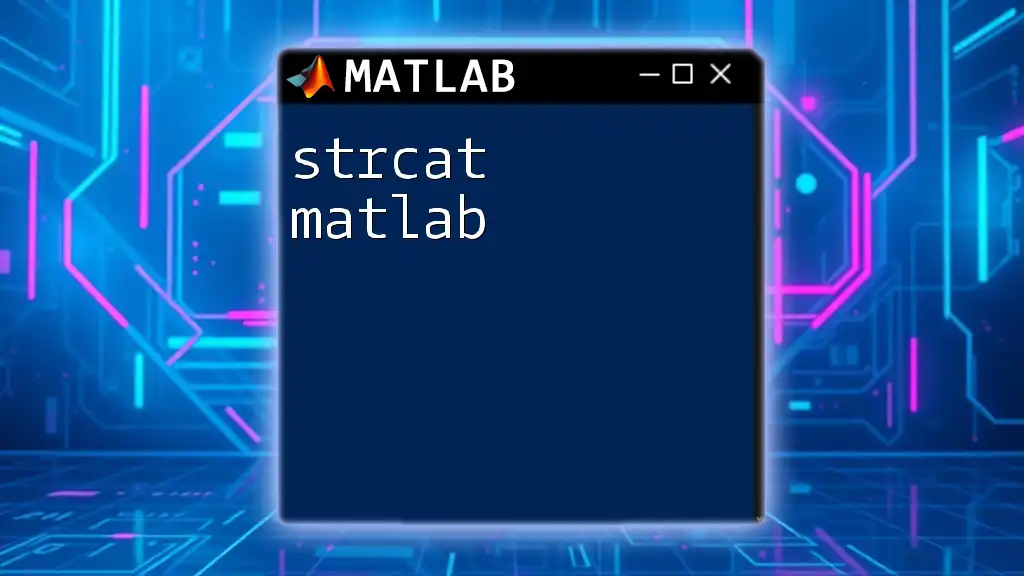
Common Pitfalls and Troubleshooting
Understanding Element-wise vs. Matrix Comparison
While using the `<=` operator, it's essential to understand that it performs element-wise comparisons. An error may occur if the operands do not adhere to the size requirements. For example:
A = [1, 2, 3];
B = [1; 2]; % B is a column vector
result = A <= B; % This will cause an error
It's crucial that both operands have compatible dimensions for comparison.
Debugging Comparisons
When troubleshooting comparison results, MATLAB offers powerful functions such as `disp` and `fprintf` to display outputs clearly. Consider the following debugging example:
a = [1, 2, 3];
b = [1, 2, 5];
disp(a <= b); % debugging output for comparison
By examining the output, you can ensure that your comparisons are functioning as intended.
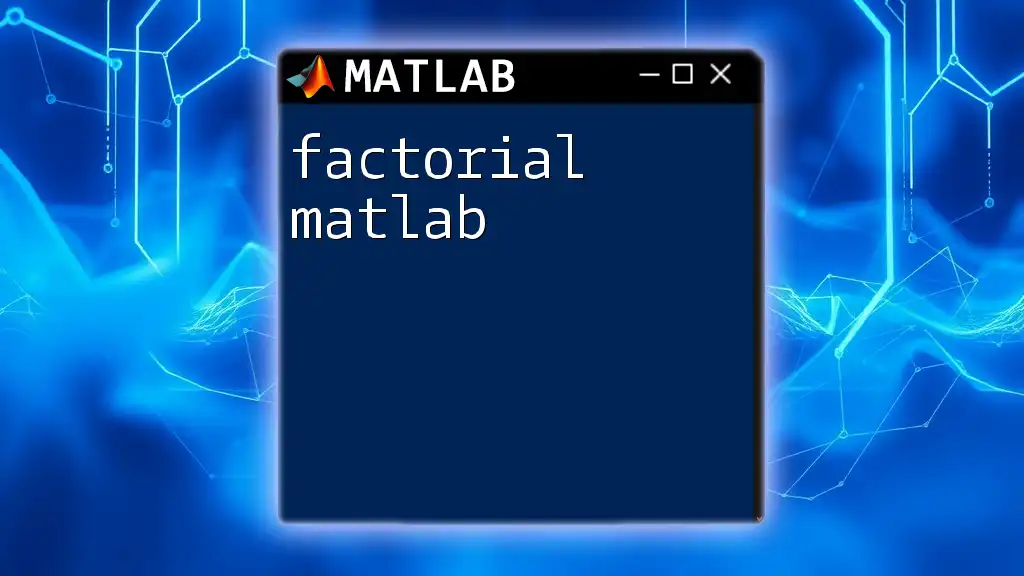
Conclusion
Understanding how to use the less than or equal to operator in MATLAB is essential for logical operations, data analysis, and programming constructs. By mastering this simple yet powerful operator, you can enhance your coding efficiency and accuracy. Practice applying `<=` in various scenarios to become proficient in leveraging it within your programming endeavors.
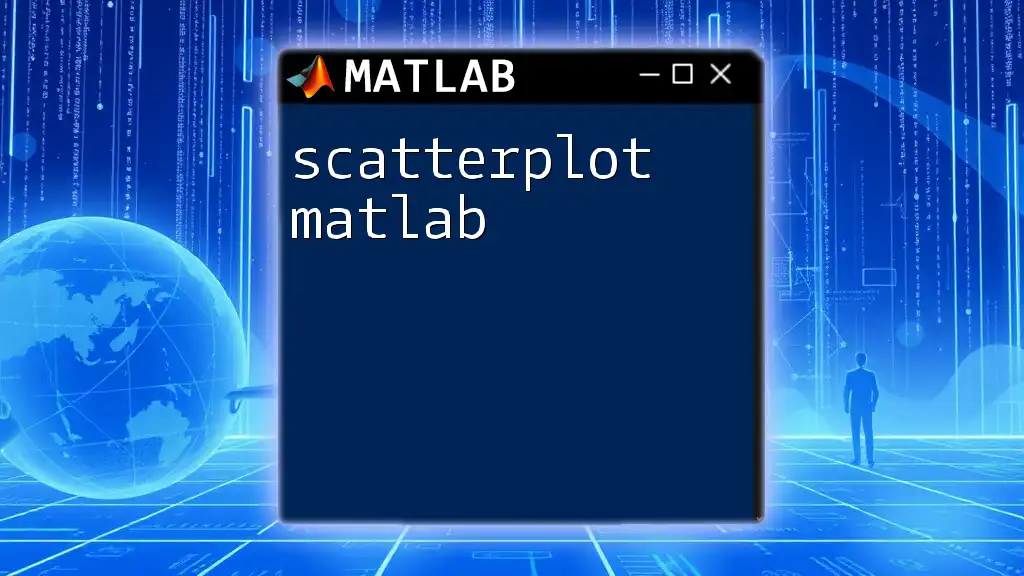
Additional Resources
For more in-depth information, consider consulting the MATLAB documentation on comparison operators. Engaging with exercises or practice problems can significantly reinforce your understanding and ability to use the less than or equal to operator effectively. Join our community to access concise and user-friendly MATLAB learning resources tailored to your needs!