In MATLAB, the "not equal to" operator is represented by `~=` and is used to compare two values, returning true if they are not equal.
% Example usage of not equal to operator
a = 5;
b = 10;
result = (a ~= b); % This will return true (1) since 5 is not equal to 10
Understanding the "Not Equal To" Operator in MATLAB
What is the "Not Equal To" Operator?
In MATLAB, the `not equal to` operator is represented by the symbol `~=`. It’s a crucial logical operator that is used to compare two values or expressions to determine if they are not equal. This operator is instrumental in various programming scenarios, enabling developers to implement conditional logic and perform data manipulations effectively.
Syntax of the "Not Equal To" Operator
The basic syntax for using the not equal to operator is:
A ~= B
Here, `A` and `B` can be various data types such as scalars, vectors, or matrices. This operator will evaluate to true (1) if `A` is not equal to `B` and false (0) otherwise. This makes it a powerful tool for conditional checks in your code.
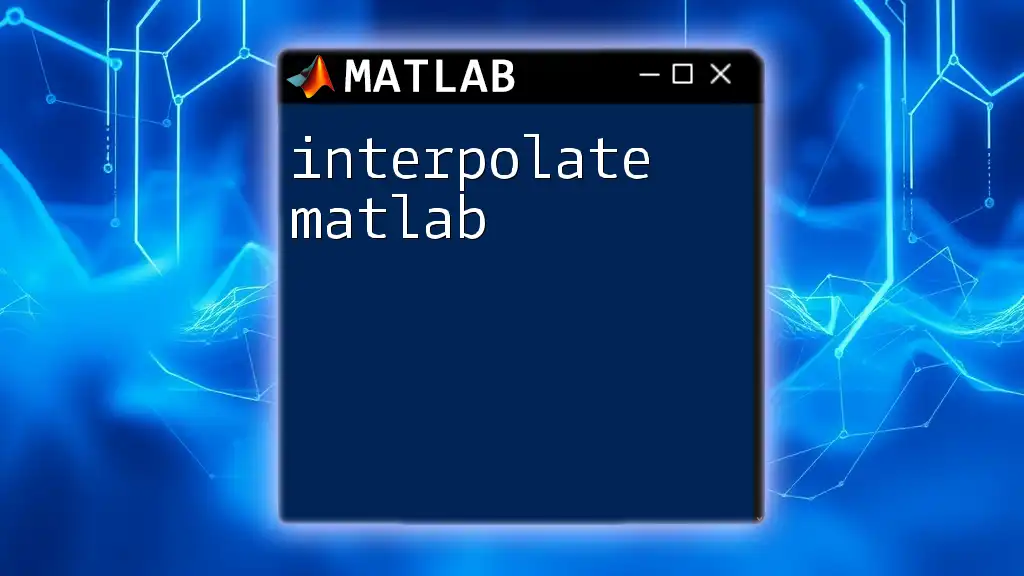
Practical Applications of "Not Equal To"
Conditional Statements
One of the primary uses of the not equal operator is within conditional statements like `if`. By leveraging this operator, developers can control the flow of their programs based on value comparisons.
For example, consider the following code snippet:
A = 10;
B = 5;
if A ~= B
disp('A is not equal to B');
end
In this example, the condition evaluates to true since 10 is indeed not equal to 5. As a result, the output will be: A is not equal to B.
Filtering Data
The not equal to operator is also invaluable for filtering datasets. By using this operator, one can easily sift through arrays or matrices to exclude unwanted values.
Working with Arrays and Matrices
Here’s how you can filter elements from an array based on a specific value:
data = [1, 2, 3, 4, 5, 6];
filteredData = data(data ~= 3);
In this case, the filtered data will exclude the number `3`, resulting in the output: filteredData → [1, 2, 4, 5, 6]. This technique can be particularly useful when you want to clean datasets and remove specific unwanted elements.
Use in Loops
The not equal to operator can be effectively used within loops to execute commands based on specific conditions.
For instance, you might want to skip a number while iterating over a loop:
for i = 1:5
if i ~= 3
disp(['Number: ', num2str(i)]);
end
end
In this example, the loop will print the numbers from 1 to 5, excluding the number 3. The output will be: Number: 1, Number: 2, Number: 4, Number: 5.
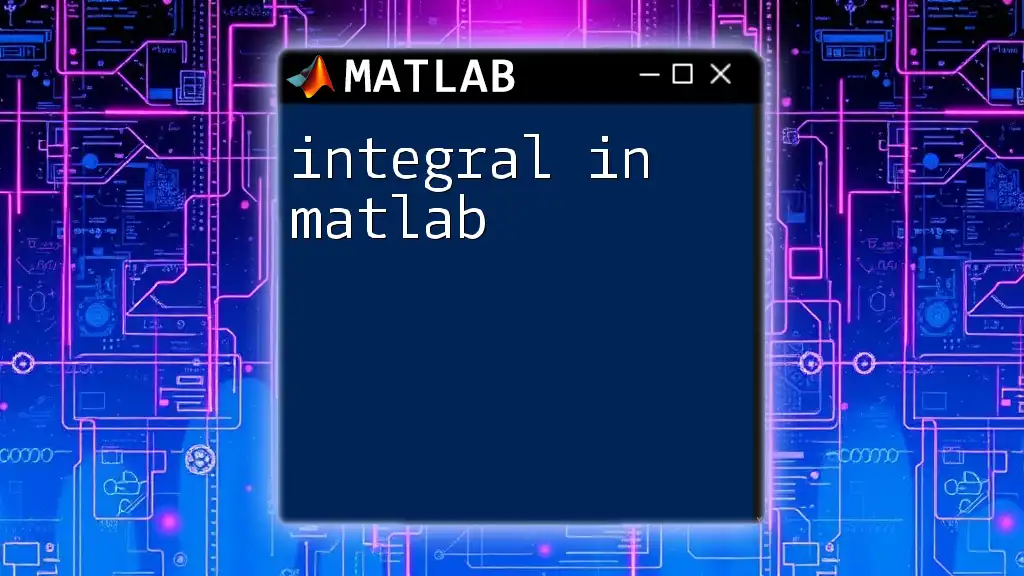
Advanced Usage
Comparing Arrays
The not equal operator can also facilitate element-wise comparisons between arrays, allowing developers to identify which elements differ.
For example:
A = [1, 2, 3];
B = [3, 2, 1];
result = A ~= B;
Here, the `result` will produce an array indicating which elements are not equal, resulting in: result → [1, 0, 1]. The first and third elements of `A` are not equal to those of `B`, while the second elements are equal.
Logical Indexing
Another powerful application of the not equal operator is logical indexing, which refers to indexing data based on specific logical conditions.
Consider the following matrix example:
matrix = [1, 2; 3, 4; 5, 6];
result = matrix(matrix(:,1) ~= 3, :);
In this snippet, the script extracts all rows from the matrix where the first column values are not equal to `3`, producing a submatrix without the second row. The output would be: result → [1, 2; 5, 6].
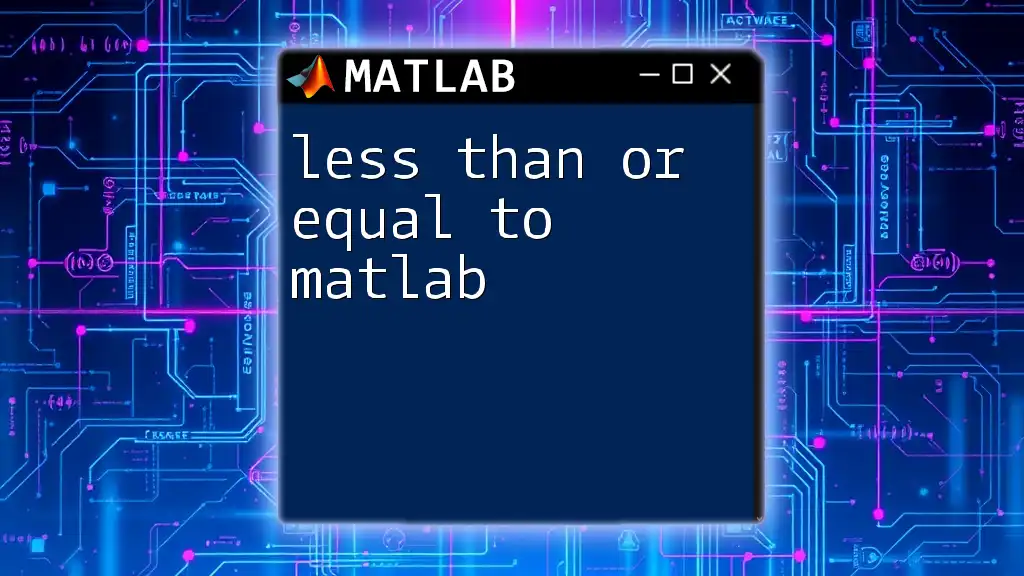
Common Errors and Troubleshooting
Mismatched Data Types
While using the not equal operator, one common pitfall is comparing variables of different data types. Such comparisons can lead to unexpected results or errors. Always ensure that the operands are of compatible types. For example, comparing a string with a numeric value can lead to confusion.
Using Parentheses
To avoid confusion in complex logical conditions, it is essential to use parentheses correctly. This ensures that MATLAB evaluates expressions in the intended hierarchy.
For instance:
if (A ~= B) && (B > 4)
disp('Conditions met');
end
This ensures that both conditions are evaluated before combining them with the logical operator `&&`.
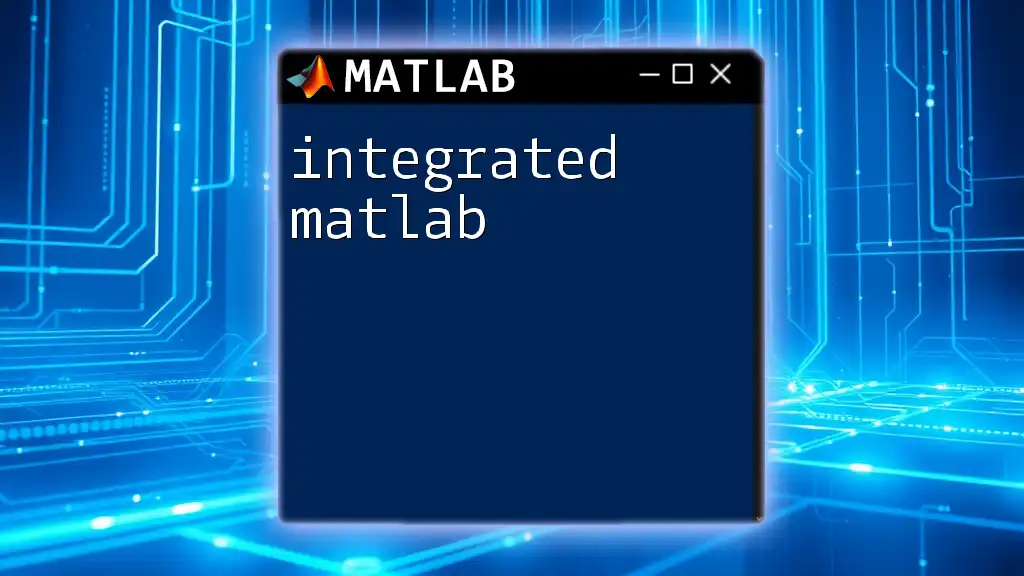
Conclusion
Understanding the "not equal to" operator in MATLAB is fundamental for anyone looking to enhance their programming skills. Its versatility in conditional statements, filtering data, and performing logical comparisons makes it an indispensable tool in your MATLAB toolkit. Practice utilizing these examples and scenarios for a deeper grasp of this operator and its wide-ranging applications in data analysis and programming.
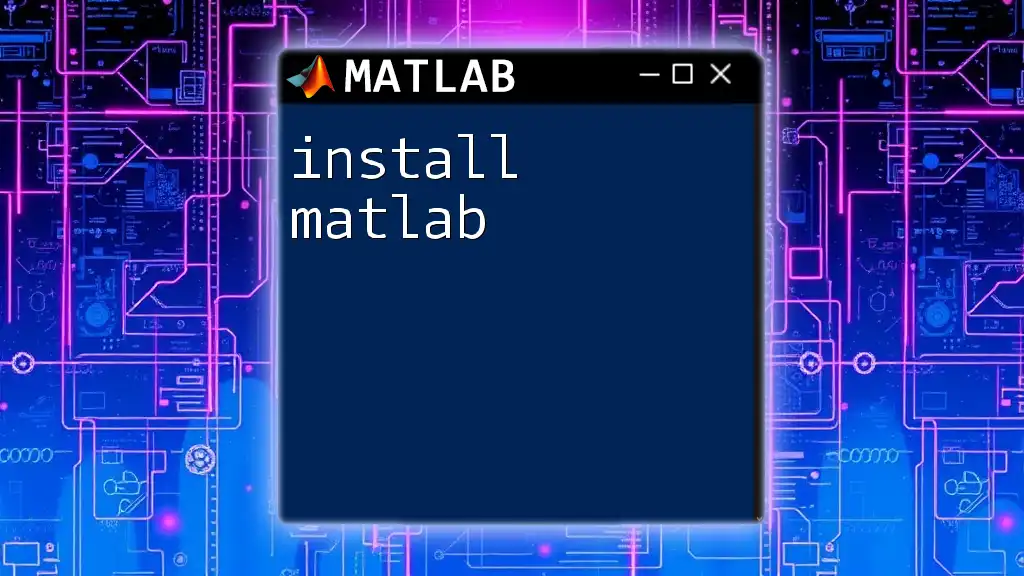
Additional Resources
Useful Links
Explore the official [MATLAB documentation](https://www.mathworks.com/help/matlab/) for further insights on logical operators and functionalities.
Learning Tools
Consider leveraging MATLAB toolboxes and community forums for support, such as MATLAB Central, where you can find additional resources and connect with other users.
By mastering concepts like the not equal operator in MATLAB, you will significantly enhance your capability to perform intricate data analyses and programming tasks efficiently.