The `strcat` function in MATLAB is used to concatenate strings or arrays of strings, combining them into a single string array.
result = strcat('Hello', ' ', 'World'); % Concatenates "Hello", a space, and "World"
Understanding String Concatenation
Definition of String Concatenation
String concatenation is the process of joining two or more strings together to form a single string. This fundamental operation is crucial when working with text data in programming. In various applications, you might need to combine names, create file paths, or construct messages dynamically.
Why Use `strcat`?
When incorporating strings in MATLAB, you may wonder why you should use `strcat` instead of other string functions like `sprintf` or `strjoin`. `strcat` is particularly designed for string concatenation, making it a more straightforward choice for this task. It handles input strings efficiently and enables easy manipulation. Using `strcat` ensures your code remains concise and readable, minimizing the possibility of errors that can arise from manual concatenation methods.
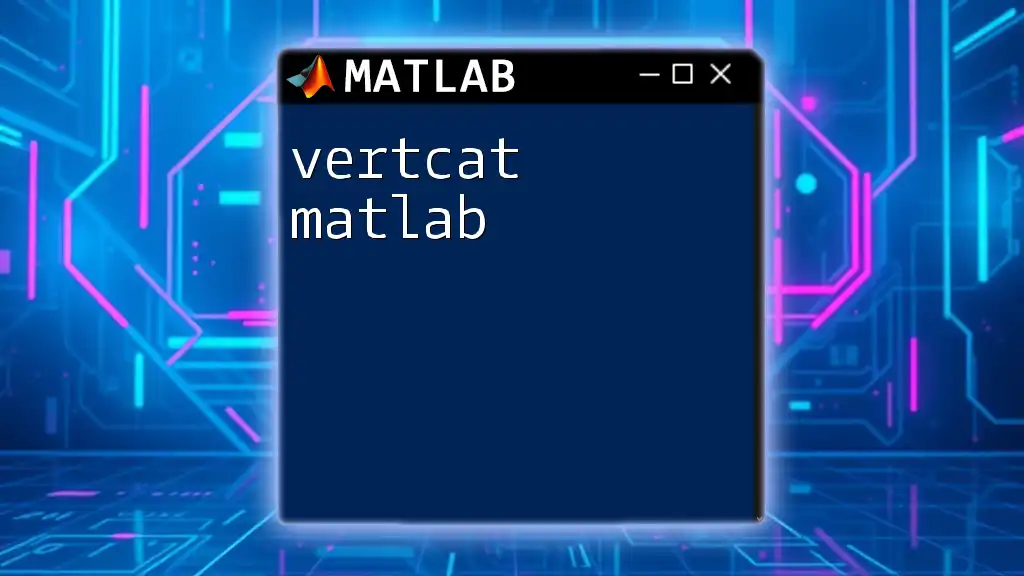
Syntax of `strcat`
The syntax for `strcat` is simple. The function accepts multiple string inputs and returns a single concatenated string.
result = strcat(string1, string2, ...)
This flexibility allows you to concatenate two or more strings seamlessly in one line of code.
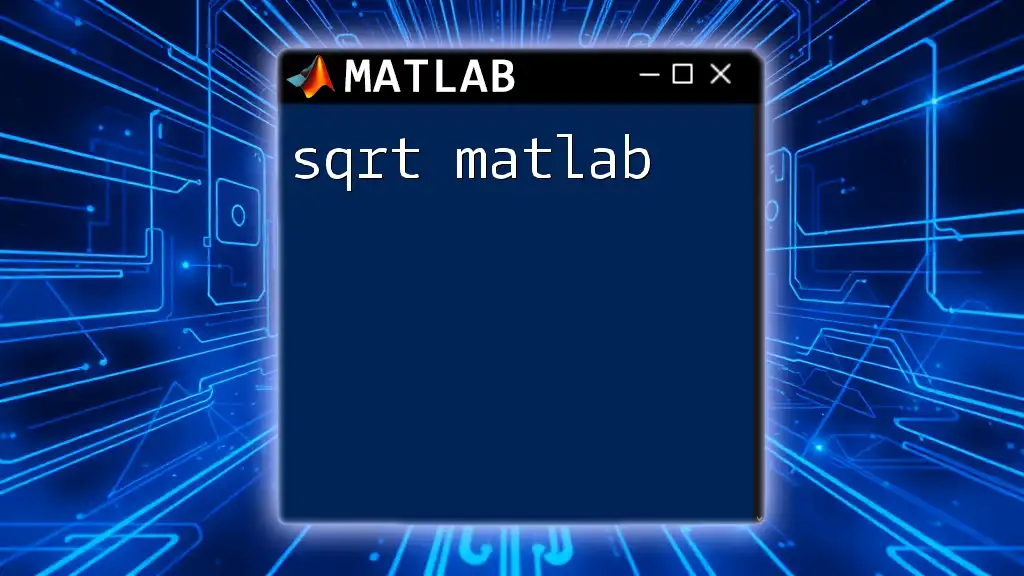
How `strcat` Works
Rules of String Concatenation
Understand how `strcat` behaves with various inputs. Notably, this function removes trailing whitespace from each input string, which can be useful in ensuring a clean output. If one (or more) of the input strings is empty, `strcat` will simply skip it while concatenating other non-empty strings.
Examples of Using `strcat`
Basic Examples
In the simplest case, concatenating two straightforward strings can be executed as follows:
result = strcat('Hello', ' World');
% Output: 'Hello World'
Multiple String Concatenation
You can also concatenate more than two strings effortlessly:
result = strcat('MATLAB', ' is', ' fun');
% Output: 'MATLAB is fun'
Using with String Arrays
`strcat` is capable of handling arrays of strings, making it a powerful tool for managing batch processing:
strArray = ["apple", "banana"];
result = strcat(strArray, "_fruit");
% Output: ["apple_fruit", "banana_fruit"]
This feature of `strcat` enhances its usability when dealing with larger sets of data.
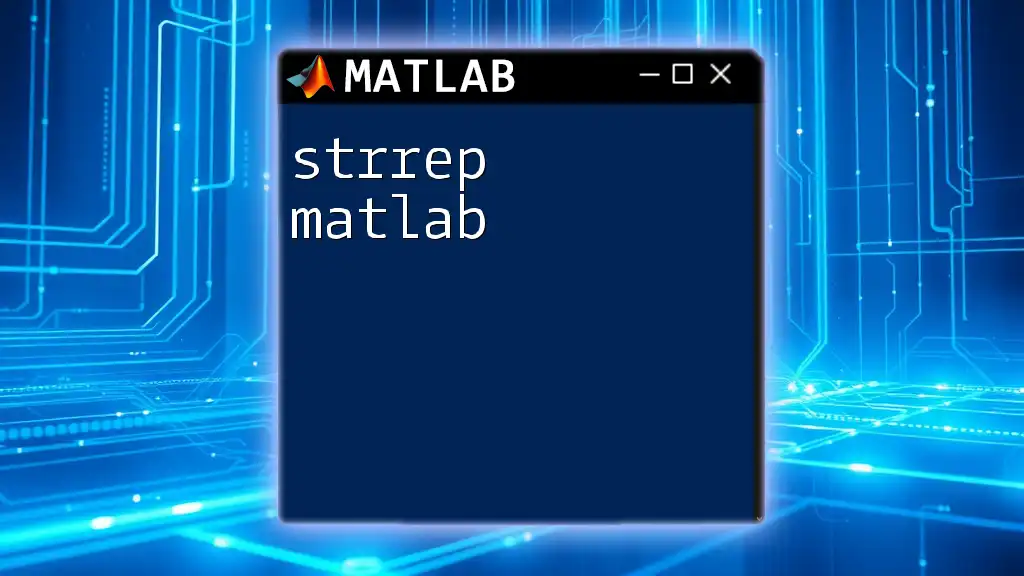
Common Use Cases for `strcat`
Creating File Paths
A practical application of `strcat` is in the creation of file paths. This capability is often required when dealing with dynamic file naming in scripts. Here’s how it can be done:
folder = 'C:\Users\';
fileName = 'data.txt';
filePath = strcat(folder, fileName);
% Output: 'C:\Users\data.txt'
Just as you can dynamically create paths, you can integrate variables and string manipulation to handle dynamic data.
Constructing Messages
`strcat` shines in instances where you need to construct user-friendly messages. This is crucial for user interfaces or console outputs. For instance:
name = 'Alice';
welcomeMessage = strcat('Welcome, ', name, '!');
% Output: 'Welcome, Alice!'
This function enhances the interaction between the program and its users, allowing for clear communication throughout the user experience.
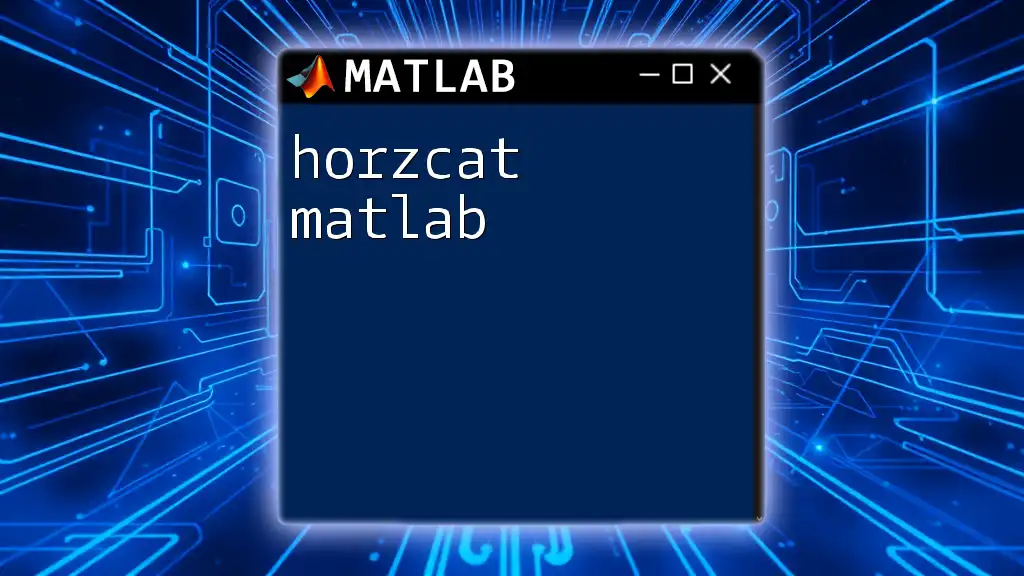
Handling Empty Strings and Special Cases
Working with Empty Inputs
When encountering empty input strings, `strcat` behaves intelligently. It skips any empty strings, maintaining the integrity of your concatenated result:
result = strcat('Hello', '');
% Output: 'Hello'
The Impact of Leading and Trailing Spaces
One important feature of `strcat` is its handling of spaces within input strings. It can automatically manage leading and trailing spaces, ensuring a spaced output that is consistent and formatted correctly:
result = strcat('Hello ', 'World');
% Output: 'Hello World'
Understanding this behavior enables you to control the appearance of your output effectively.
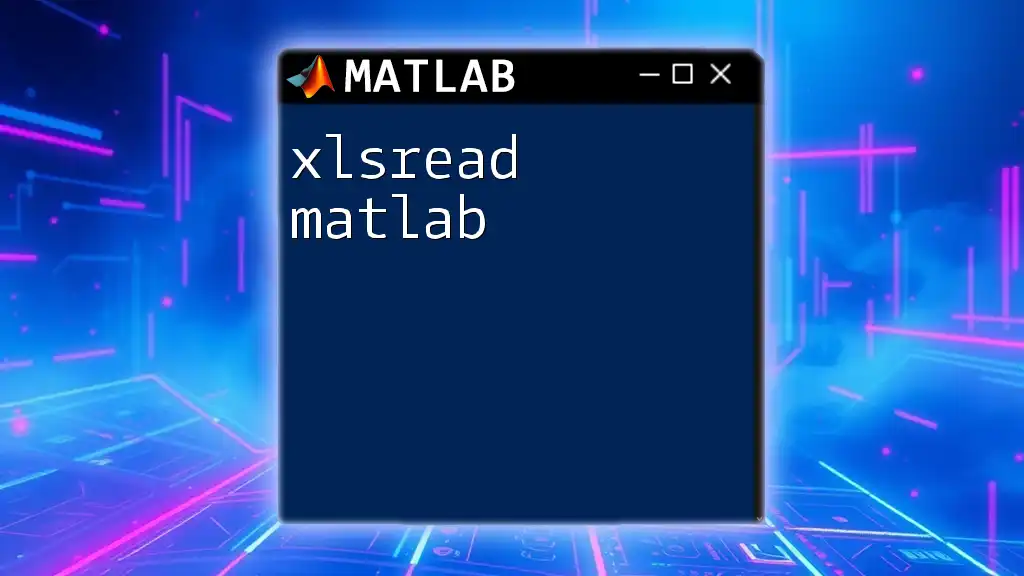
Tips and Best Practices
When to Use `strcat`
Employ `strcat` when you need to concatenate strings quickly and efficiently. This function is optimal when working with user-generated input or dynamically generated strings. In cases where strings come from arrays or are combined in large datasets, `strcat` saves time and enhances performance.
Common Pitfalls to Avoid
Despite its simplicity, there are common pitfalls to be aware of when using `strcat`. Ensure all inputs are either character arrays or string arrays. Attaching non-string data types may lead to errors. Additionally, while `strcat` ignores trailing whitespace during concatenation, developers should still be mindful of their input format for consistency.
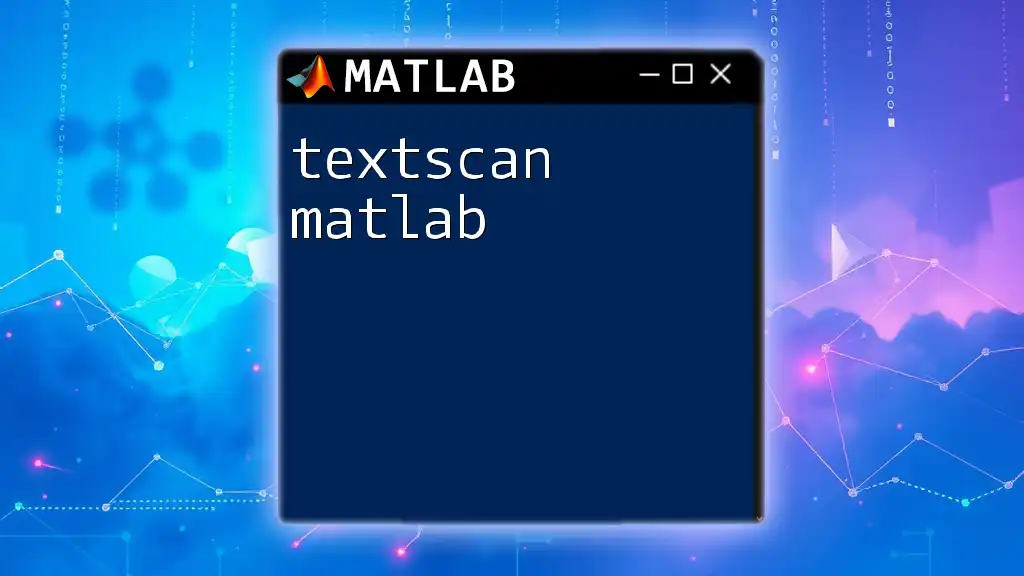
Conclusion
In summary, `strcat` is an invaluable function in MATLAB for string concatenation. Its versatility and efficiency empower developers to manipulate strings effectively, making it an essential tool for both beginners and seasoned programmers. By mastering `strcat`, you can enhance your coding capabilities in MATLAB and elevate the quality of your text-based outputs.
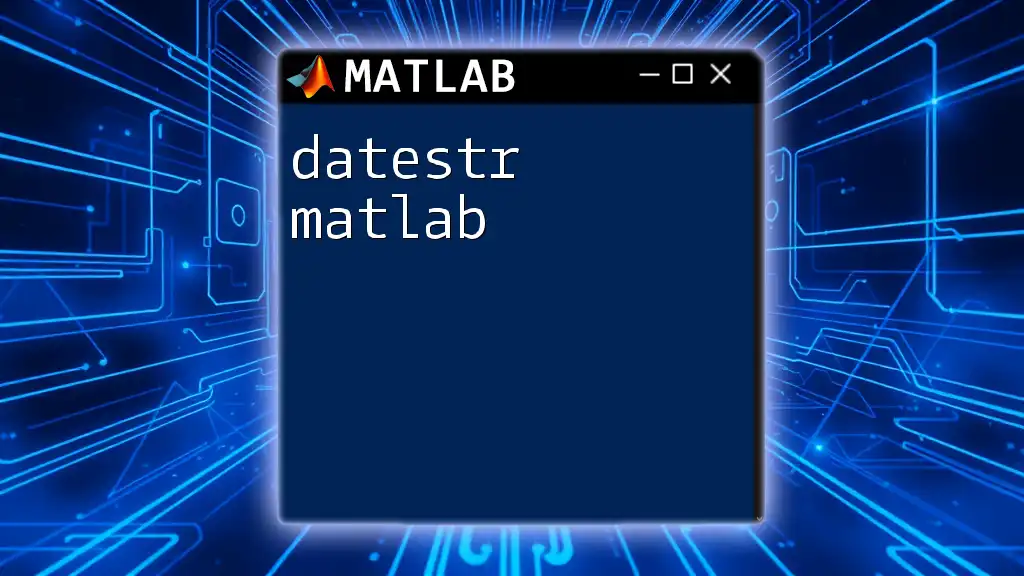
Additional Resources
Recommended Readings
For further exploration of string manipulation in MATLAB, consult the official MATLAB documentation, which offers in-depth insights and tutorials.
Practice Problems
To solidify your understanding, try creating your own examples using `strcat` by constructing messages, file paths, or combining string arrays. Hands-on practice will deepen your grasp of how to utilize this function effectively in your programming endeavors.