The `lsqnonlin` function in MATLAB is used to solve nonlinear least squares problems, providing a way to minimize the sum of squares of nonlinear functions.
% Example of using lsqnonlin to fit a model to data
data_x = [1, 2, 3, 4, 5];
data_y = [1.2, 1.9, 3.3, 4.1, 5.5];
model = @(b, x) b(1) * x.^2 + b(2); % Quadratic model
% Initial guess for parameters
b0 = [1, 1];
% Objective function to minimize
residuals = @(b) model(b, data_x) - data_y;
% Using lsqnonlin to find optimal parameters
b_opt = lsqnonlin(residuals, b0);
What is `lsqnonlin`?
The `lsqnonlin` function in MATLAB is designed for solving nonlinear least squares problems. This is a common objective in parameter estimation, where we aim to minimize the sum of the squares of nonlinear functions, typically to fit a model to experimental or observed data. It's widely applicable in areas such as engineering, finance, and data analysis, where precise modeling of system behavior is crucial.
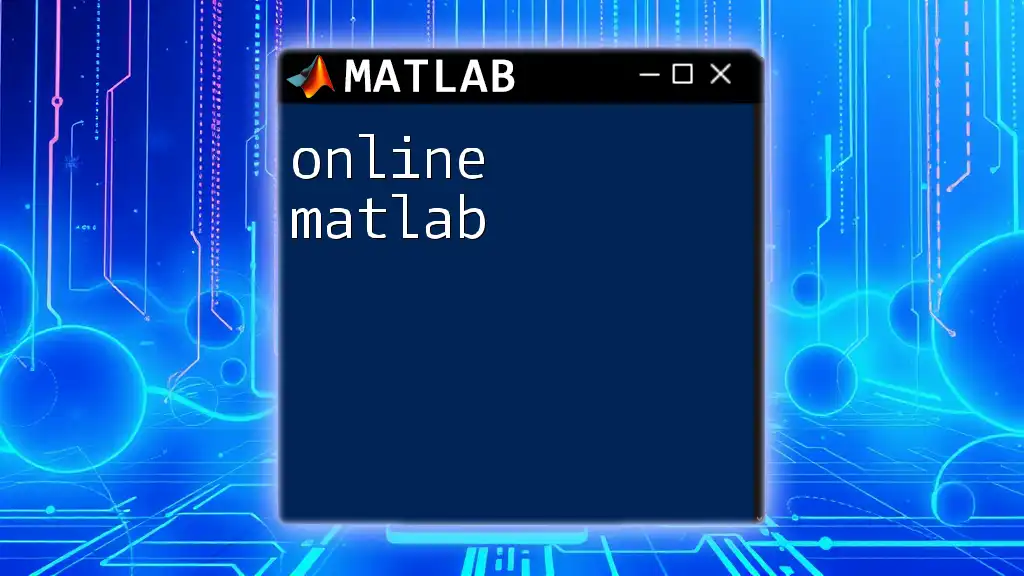
Why use `lsqnonlin`?
Using `lsqnonlin` provides several advantages over traditional linear least squares methods. Nonlinear relationships are common in real-world data, and `lsqnonlin` allows for the direct handling of such relationships without transforming the data into a linear form. This function is capable of handling:
- Complex Models: It can fit complex functions that cannot be expressed as linear equations.
- Automatic Differentiation: It can compute Jacobians automatically if you provide the option.
- Constraints: Bound and linear constraints can easily be incorporated.
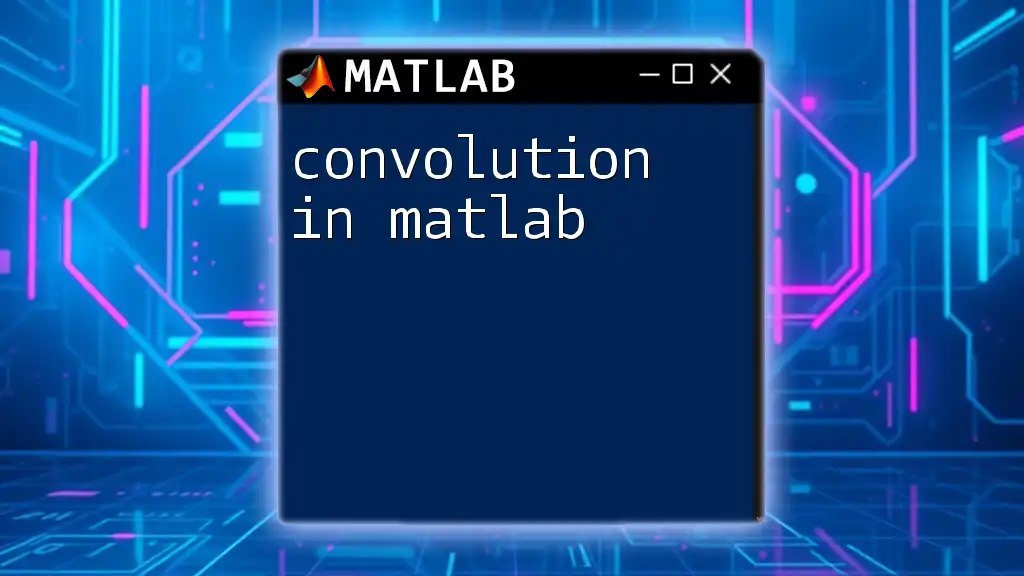
Understanding the Syntax of `lsqnonlin`
The basic syntax of `lsqnonlin` is as follows:
x = lsqnonlin(fun, x0)
Detailed Parameters Explanation
-
`fun`: A function handle for the residuals function that returns the errors to minimize. The function should accept a vector of parameters as input and return a vector of residuals.
-
`x0`: The initial guess for the solution, which can significantly impact convergence.
-
`lb` and `ub`: Optional parameters that define lower and upper bounds on the solution. Use these to limit the search space.
-
`options`: A structure that allows you to customize settings such as the display of output, tolerances, and maximum iterations.
-
`A`, `b`, `Aeq`, `beq`: Optional matrices that specify linear inequality (`Ax <= b`) and equality (`Aeqx = beq`) constraints.
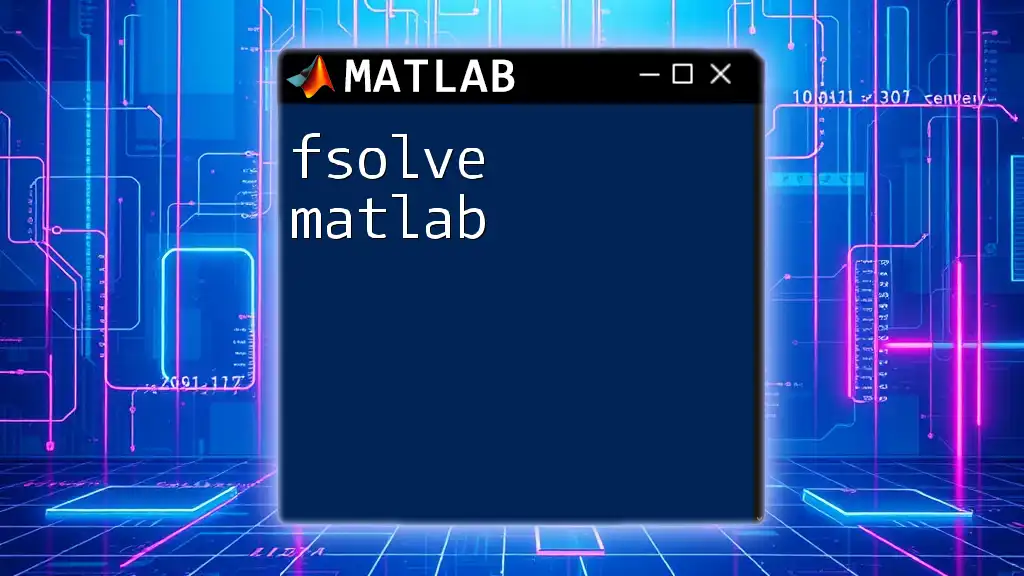
Setting Up Your Problem
Defining the Objective Function
To use `lsqnonlin` effectively, you also need to define the function that calculates the residuals. Here’s an example structure for a residuals function:
function F = myResiduals(x)
F = [x(1)^2 + x(2) - 10; x(1) + x(2)^2 - 20];
end
In this function, `F` is a vector of differences between a model prediction and the actual data.
Choosing Initial Guess
The initial guess (`x0`) can profoundly affect the optimization process. Ideally, it should be close to the expected solution when possible. For example, if you're modeling a simple linear relationship, you might start with coefficients near the mean of your data.
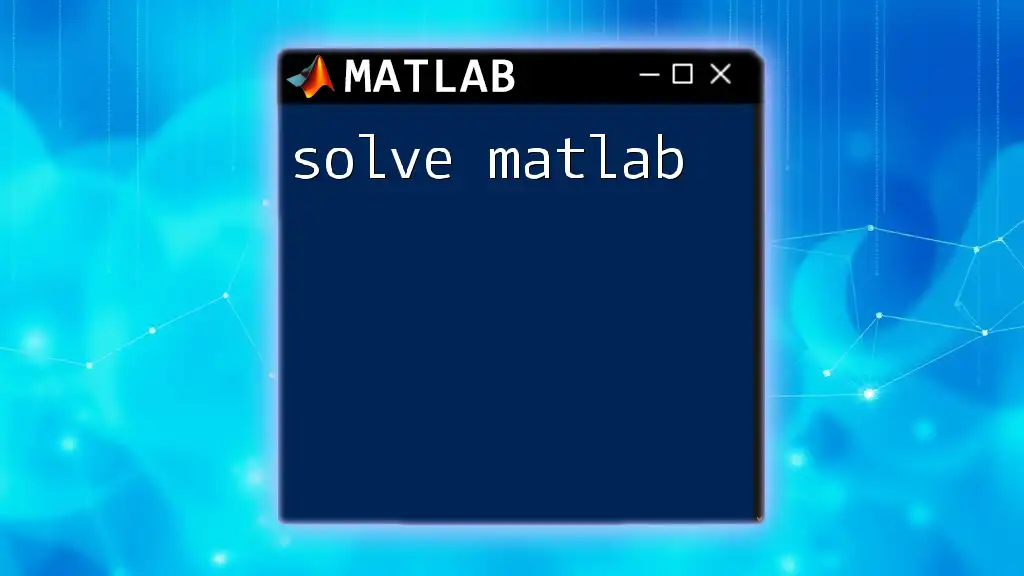
Example Problem: Fitting Data with `lsqnonlin`
Data Generation
To illustrate how to use `lsqnonlin`, let’s first create some synthetic data:
xData = linspace(0, 10, 100);
yData = 3 * exp(0.5 * xData) + randn(1, 100); % Adding noise to the data
Creating the Residual Function for Data Fitting
Now, let’s write a residual function to fit an exponential model to this data:
function F = fitExp(params)
a = params(1);
b = params(2);
F = yData - a * exp(b * xData); % Exponential fit
end
Running `lsqnonlin` to Fit the Model
With the data and residual function prepared, we can use `lsqnonlin` to find the best-fitting parameters:
initialGuess = [1, 0.1]; % Initial guesses for parameters
options = optimoptions('lsqnonlin', 'Display', 'iter'); % Options to display output
fittedParams = lsqnonlin(@fitExp, initialGuess, [], [], options);
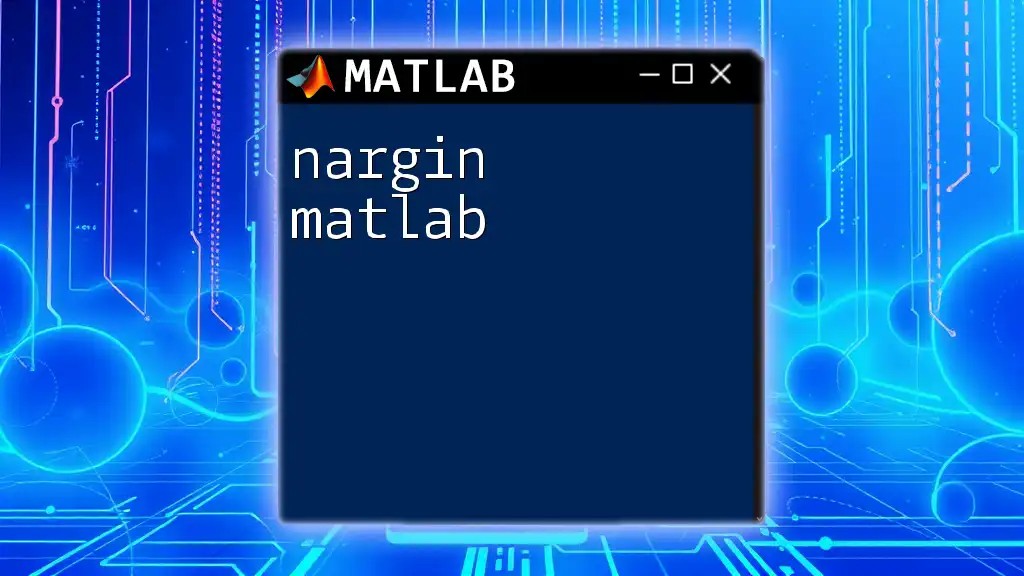
Analyzing Results
Interpreting the Output
The output from `lsqnonlin` gives you the optimized parameters, which you can interpret in the context of your problem. For example, if your fitting yields `a = 3.1` and `b = 0.5`, you may conclude that your model `y = 3.1 * exp(0.5 * x)` adequately describes your data.
Plotting the Results
Visualization is vital for understanding model performance. You can plot both the observed and fitted values:
fittedData = fittedParams(1) * exp(fittedParams(2) * xData);
plot(xData, yData, 'o', xData, fittedData, '-r'); % Plot observed data and fitted curve
legend('Data', 'Fitted Curve'); % Add a legend for clarity
Error Metrics
Consider calculating metrics such as the coefficient of determination (R²) or Root Mean Square Error (RMSE) to quantitatively evaluate your model fit.
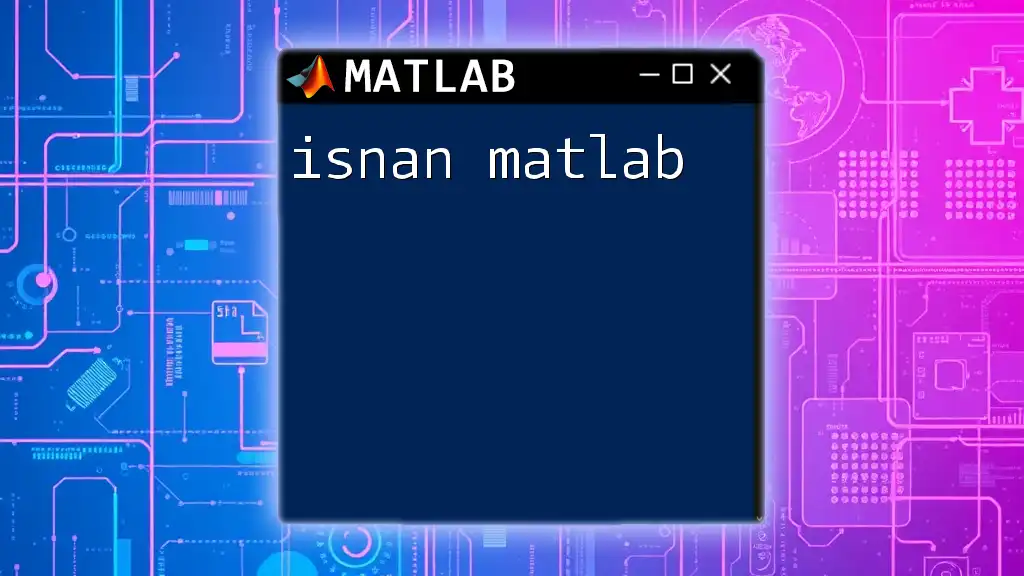
Advanced Features of `lsqnonlin`
Options and Customization
MATLAB provides extensive options within `lsqnonlin`. To customize your optimization process, you can set parameters like:
options = optimoptions('lsqnonlin', 'MaxIter', 1000, 'TolFun', 1e-6);
Handling Bounds and Constraints
Handling bounds efficiently can significantly enhance model fitting. Define them as follows:
lb = [0, -Inf]; % Lower bounds for the parameters
ub = [Inf, Inf]; % Upper bounds
Using Additional Inputs
Sometimes, you might have additional parameters to pass into your residual function. Use anonymous functions to incorporate these variables appropriately without changing the structure of your main fitting code.
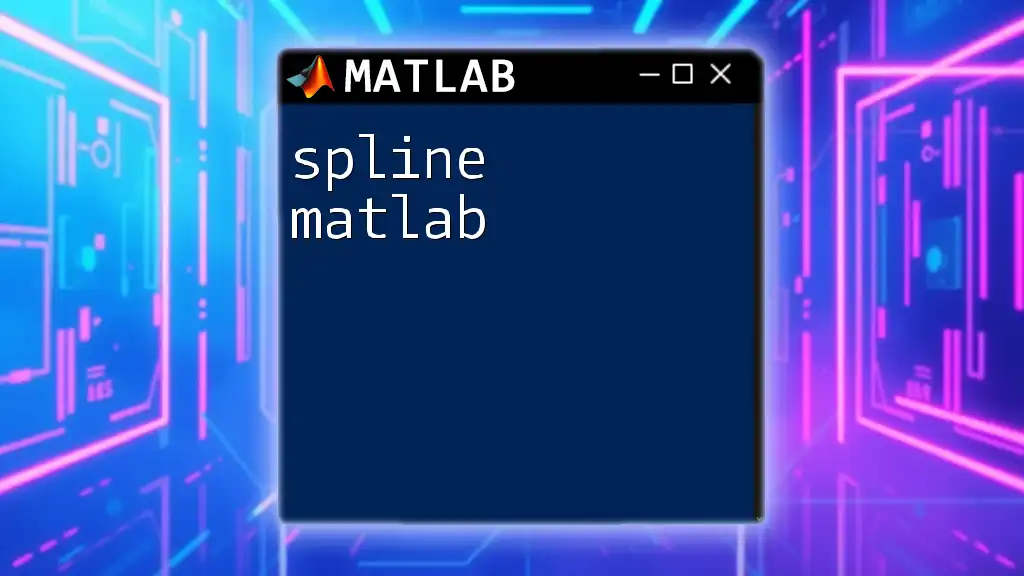
Common Pitfalls and Troubleshooting
Convergence Issues
If `lsqnonlin` fails to converge, consider the following common issues:
- Poor Initial Guesses: The initial guess may not be close enough to the solution.
- Scaling Problems: Functions with very large or very small values can lead to numerical issues. Normalize input data as needed.
Selection of Starting Points
Using domain knowledge to pick smart initial values can significantly improve convergence speed and success.
Understanding Warnings and Errors
Familiarize yourself with common warnings such as "Maximum number of function evaluations exceeded." This indicates you might need to adjust your options.
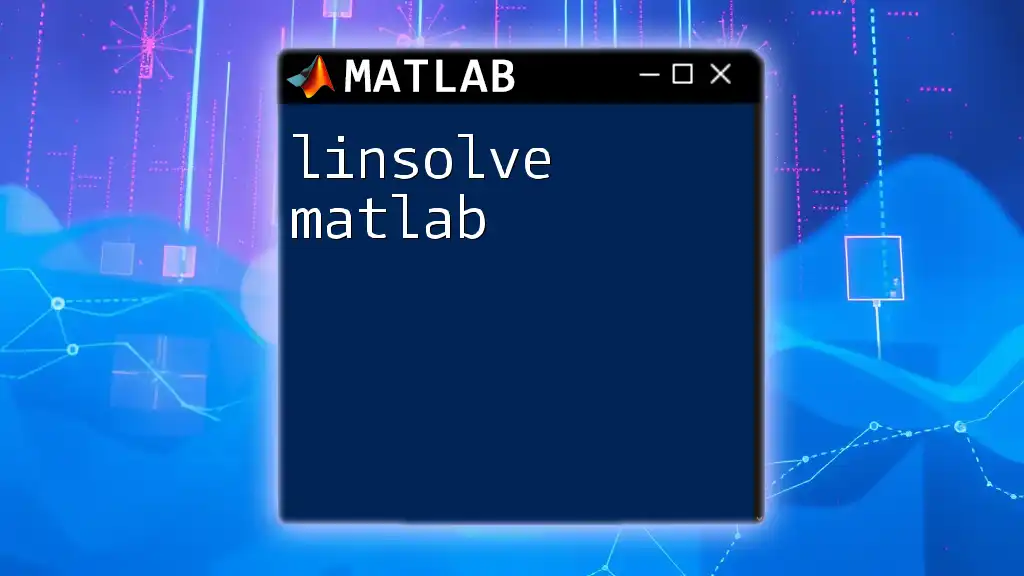
Conclusion
Understanding how to use `lsqnonlin` in MATLAB is essential for solving non-linear least squares problems efficiently. By following the steps outlined in this guide, from defining your residuals function to interpreting the results, you’ll be well-equipped to tackle a variety of real-world modeling scenarios.
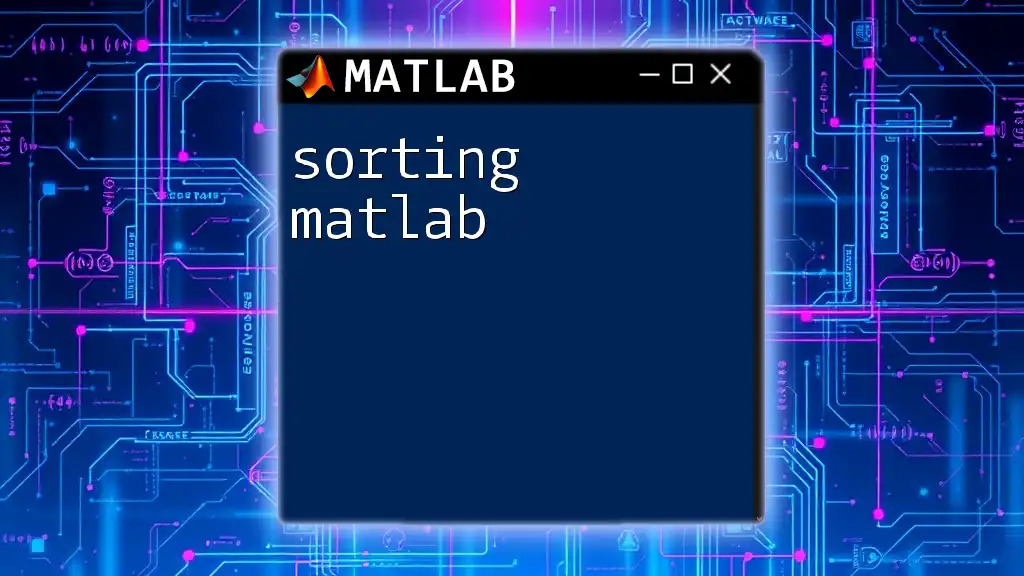
Additional Resources
For further exploration, refer to the official MATLAB documentation for `lsqnonlin`, which provides deeper insights into advanced functionality. Additionally, consider engaging with books and online tutorials focusing on optimization techniques in MATLAB to enhance your skills effectively.