A scatter plot in MATLAB is a graphical representation used to display values for typically two variables for a set of data, where each point represents an individual data point.
Here’s a simple example of how to create a scatter plot in MATLAB:
x = rand(1, 100); % Generate random data for x
y = rand(1, 100); % Generate random data for y
scatter(x, y); % Create the scatter plot
xlabel('X-axis Label'); % Label for the X-axis
ylabel('Y-axis Label'); % Label for the Y-axis
title('Scatter Plot Example'); % Title for the plot
Understanding Scatterplots
A scatterplot is a type of data visualization that displays values for two variables as coordinates in a Cartesian plane. Each point on the plot corresponds to one observation from your dataset, facilitating a visual inspection of the relationship between the two variables. In other words, scatterplots allow you to quickly assess how one variable may influence or relate to another.
The key components of a scatterplot include:
- X and Y axes: These represent the two variables being compared. The X-axis typically represents the independent variable, while the Y-axis represents the dependent variable.
- Data points: Each point on the scatterplot represents an observation in the dataset, plotted according to its X and Y values.
- Labels and titles: Providing clear labels and a descriptive title is crucial to ensure viewers understand what the scatterplot represents.
Scatterplots are particularly useful in applications such as statistics, finance, biology, and engineering, where understanding the relationship between two quantitative variables is essential.
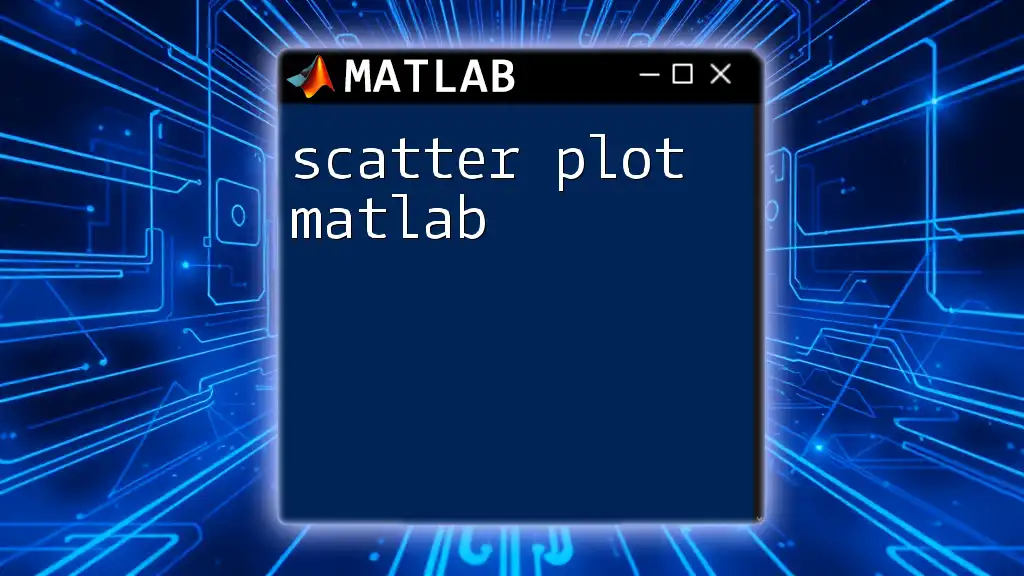
Getting Started with MATLAB
Before diving into creating scatterplots, familiarize yourself with the MATLAB environment. MATLAB features a workspace where variables are stored and a command window for executing commands directly. If you plan to utilize advanced plotting features, consider exploring MATLAB toolboxes such as the Statistics and Machine Learning Toolbox.
Key commands to know when creating a scatterplot in MATLAB include:
- `figure`: Creates a new figure window for your plot.
- `hold on`: Reserves the current plot so that additional graphical elements can be added without replacing the existing plot.
- `grid`: Adds a grid to the plot for better readability.
- `xlabel` and `ylabel`: Set the labels for the X and Y axes, respectively.
- `title`: Provides a title for the plot.
Now that you have a grasp of the basics, let's create a simple scatterplot.
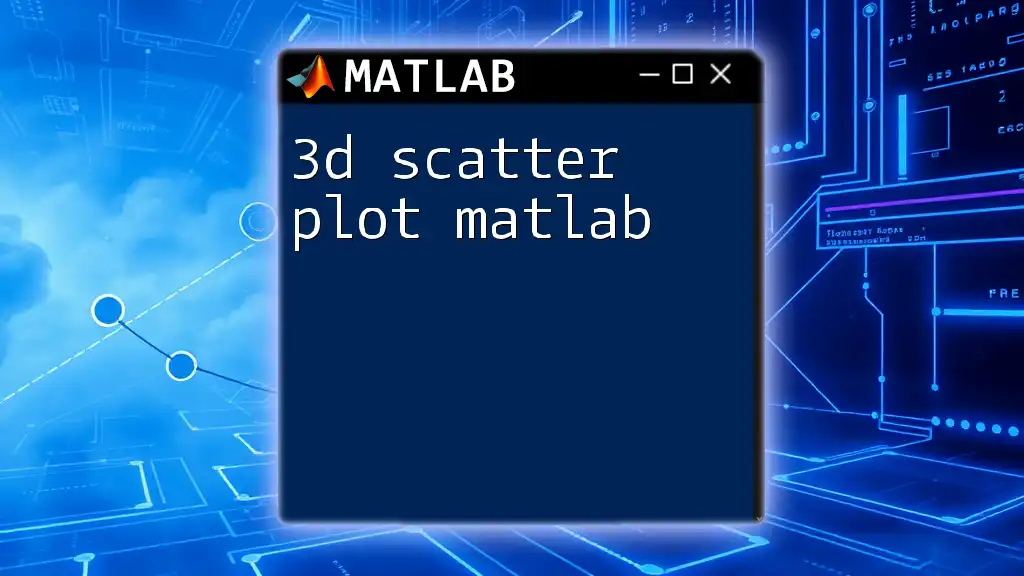
Creating a Basic Scatterplot
Crafting your first scatterplot in MATLAB is straightforward. Below is a simple code snippet that generates a scatterplot using random data:
% Sample data
x = rand(1, 20); % Random x values
y = rand(1, 20); % Random y values
% Creating scatterplot
scatter(x, y);
xlabel('X-axis Label');
ylabel('Y-axis Label');
title('Basic Scatterplot');
grid on;
In this code:
- `rand(1, 20)` generates 20 random values for both X and Y axes.
- The `scatter()` function creates the scatterplot using the data points.
- Using `xlabel()`, `ylabel()`, and `title()`, we provide context for what the axes represent and to give the plot a meaningful title.
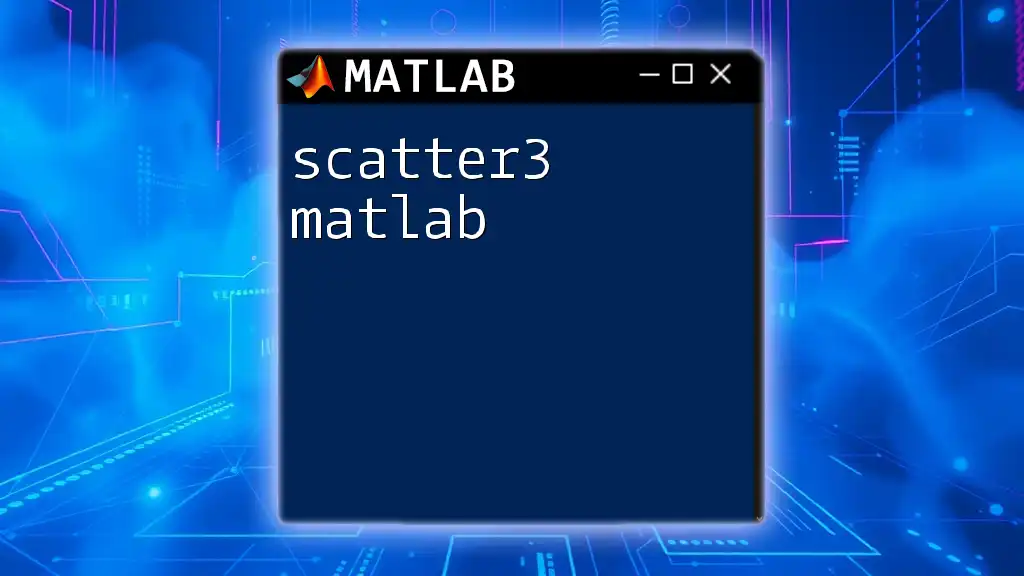
Customizing Your Scatterplot
Modifying Data Point Appearance
To enhance the visual appeal of your scatterplot, you can customize the data point appearance. This includes changing the marker style and size. For example:
scatter(x, y, 100, 'ro'); % Red circles of size 100
In this snippet:
- The `100` setting adjusts the size of the markers.
- The `'ro'` argument specifies that the markers are red circles. You can choose from various marker options, such as square (`'s'`), diamond (`'d'`), or others.
Adding Legends and Annotations
Legends and annotations improve clarity in your scatterplot. To include a legend:
legend('Sample Data');
This command labels your data points, helping viewers interpret the plot's components. Additionally, you can add annotations to highlight specific data points:
text(x(1), y(1), 'Point A', 'VerticalAlignment', 'bottom', 'HorizontalAlignment', 'right');
This line places the text ‘Point A’ next to the first point on your scatterplot, with customization on vertical and horizontal alignment for better placement.
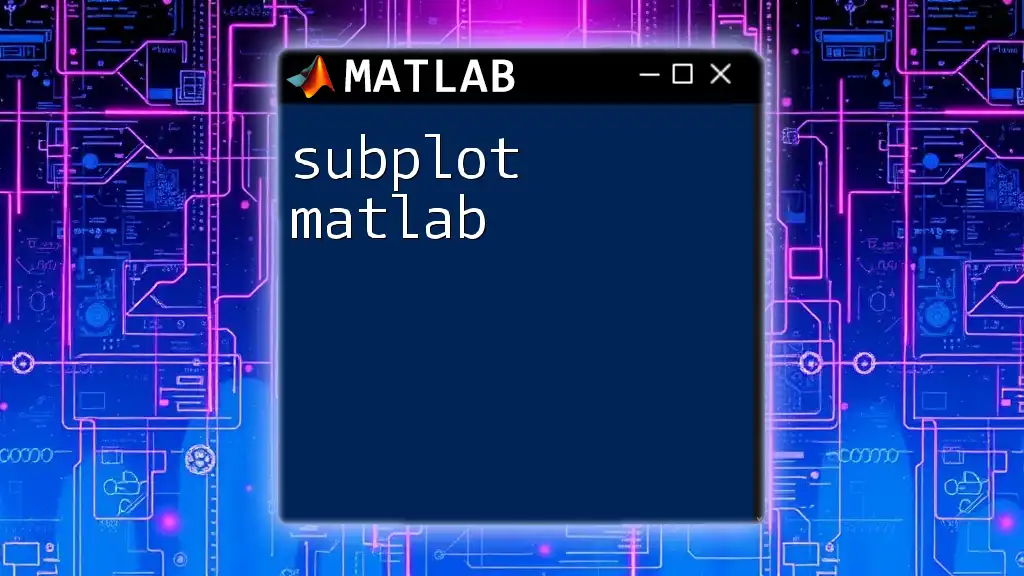
Advanced Scatterplot Techniques
Scatterplot with Multiple Datasets
Scatterplots become increasingly informative when comparing multiple datasets on the same graph. You can achieve this by layering multiple calls to the `scatter()` function. Here’s how to plot two datasets:
scatter(x1, y1, 'r'); % First dataset in red
hold on; % Keep the current plot
scatter(x2, y2, 'b'); % Second dataset in blue
This allows easy differentiation between datasets by assigning different colors, which aids in analysis and comparison.
Using Colors to Represent Data
You can also use color to represent an additional variable, enhancing the depth of your visualization. This technique allows viewers to observe more complex relationships within the data. Here’s how to implement it:
z = rand(1, 20); % Third variable
scatter(x, y, 100, z, 'filled'); % Color based on z
In this example, the markers are colored based on the values of the third variable `z`. The `filled` option allows for filled markers, adding to the visual aesthetics.
Adding Trend Lines
Adding trend lines to your scatterplot is vital for identifying the overall direction and relationship in your data. To fit a linear trend line:
p = polyfit(x, y, 1); % Linear fit
y_fit = polyval(p, x); % Evaluate fit
hold on;
plot(x, y_fit, 'k--'); % Plot the trend line
Here, the `polyfit()` function calculates a polynomial fit (in this case, a linear fit with degree 1), and `polyval()` evaluates it at the specified x values. The `plot()` function then draws the trend line as a dashed line in black (`'k--'`).
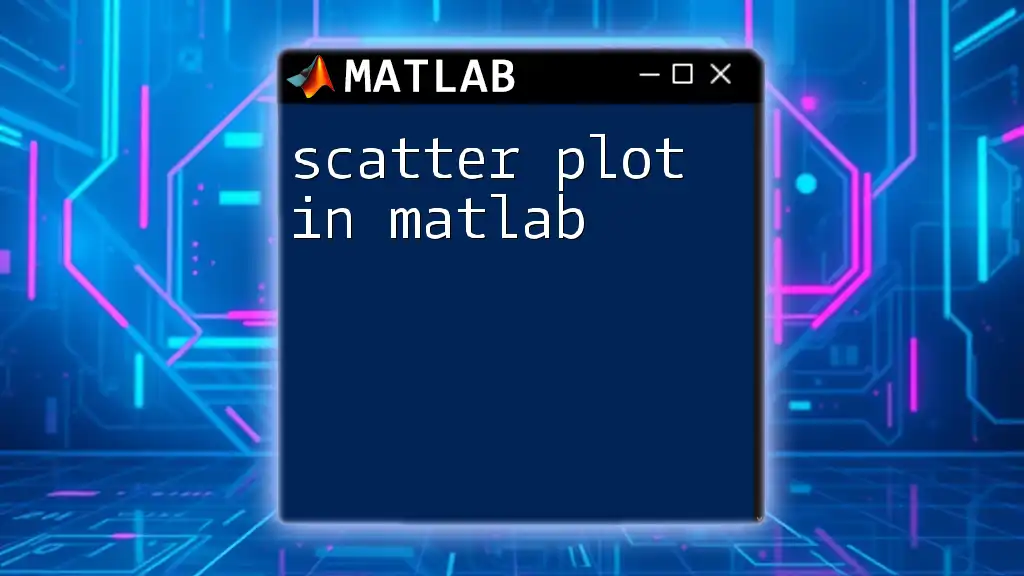
Exporting and Saving Your Scatterplot
After crafting your scatterplot, you may want to save it in various formats for presentation or sharing. You can easily save your plot with the following command:
print('scatterplot.png', '-dpng'); % Save as PNG
MATLAB offers several formats, including PNG, JPEG, and PDF, ensuring you have flexibility in how you export your plots.
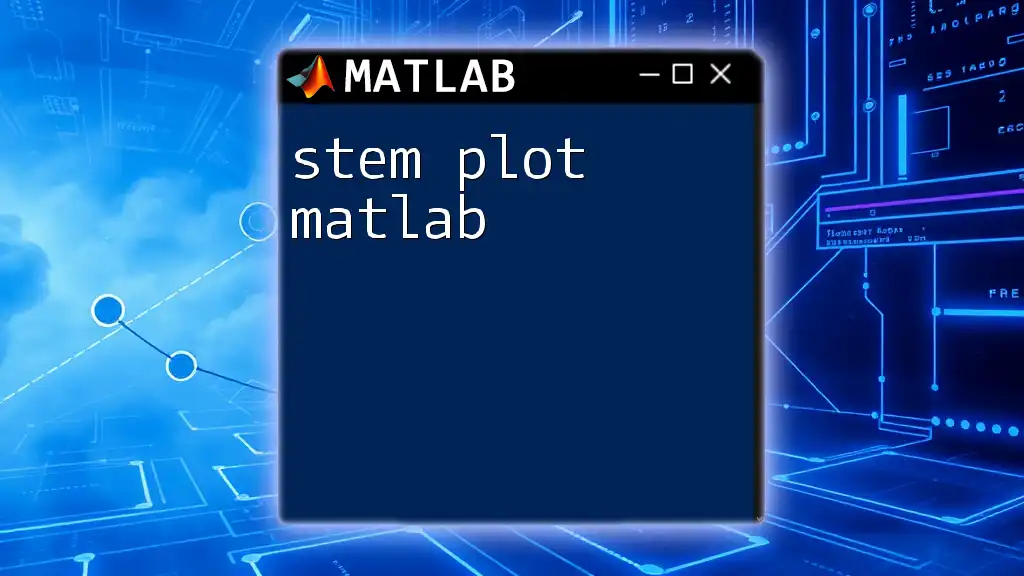
Troubleshooting Common Issues
When creating scatterplots, you may encounter common issues. One frequent problem is having data points overlap, making it difficult to interpret results. Use the `gscatter()` function to create grouped scatterplots and distinguish overlapping points easily.
Another common pitfall is mismatched data dimensions. Ensure the X and Y variables are of the same length to avoid errors. You can also use transparency with the `Alpha` property to help with overlapping points, making the scatterplot easier to read.
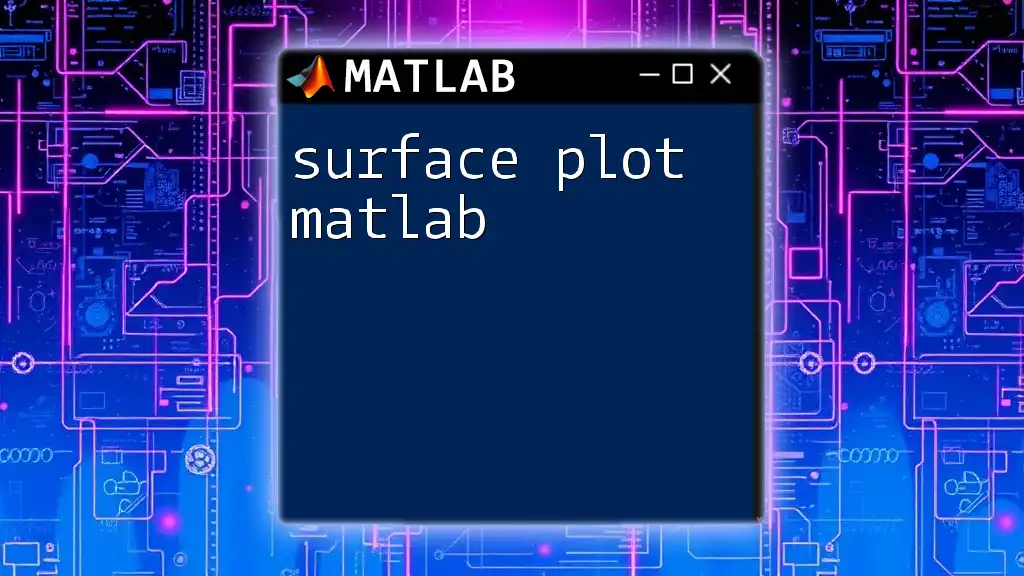
Conclusion
Scatterplots are powerful tools for visualizing the relationship between two variables, and MATLAB provides you with the capabilities to create and customize these plots effectively. From basic plots to advanced techniques that incorporate multiple datasets and trend lines, understanding how to leverage this functionality will significantly enhance your data analysis skills.
Explore the resources available, engage with MATLAB's extensive documentation, and experiment with your data to unlock the full potential of scatterplots within MATLAB. Happy plotting!