In MATLAB, you can check if a variable exists in the workspace by using the `exist` function, which returns 1 if the variable exists and 0 otherwise.
if exist('variableName', 'var')
disp('Variable exists.');
else
disp('Variable does not exist.');
end
Understanding Variable Existence in MATLAB
What Does it Mean for a Variable to Exist?
In MATLAB, a variable is considered to exist if it has been defined and holds a value in the current workspace. When you attempt to reference a variable that does not exist, MATLAB will throw an error, which can lead to unexpected behavior in scripts or functions. Handling variable existence properly is crucial for robust programming and helps in debugging potential issues more efficiently.
Common Scenarios When You Need to Check for Variable Existence
There are several common situations where checking if a variable exists is essential:
- Conditional Logic: When using IF statements, you must ensure that the variable you're checking has been defined to prevent runtime errors.
- Dynamic Data Handling: In applications where user input is taken, a variable might not be defined under certain conditions. Checking existence can help manage these cases effectively.
- Avoiding Errors: Using variables that have not been initialized can cause your code to crash or behave unexpectedly, so confirming their existence beforehand is a wise practice.
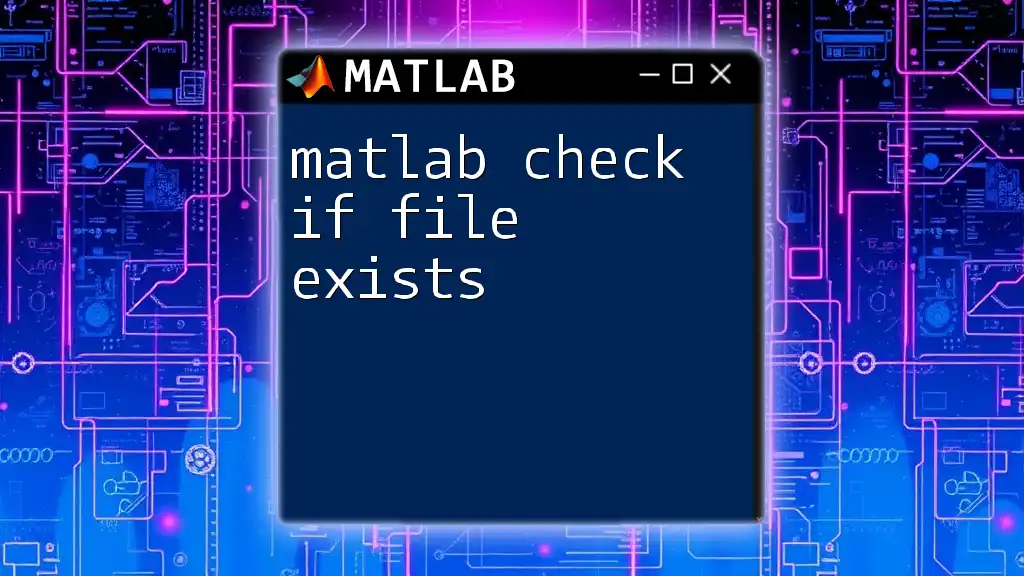
Methods to Check if a Variable Exists in MATLAB
Using the `exist` Function
One of the most straightforward methods to check if a variable exists in MATLAB is by using the `exist` function. Here’s how it works:
- Syntax: `exist('variable', 'var')`
- The first parameter is the name of the variable (as a string), and the second parameter `'var'` specifies that you are checking for a variable.
Example Code Snippet:
if exist('myVar', 'var')
disp('Variable exists');
else
disp('Variable does not exist');
end
This code checks whether the variable `myVar` exists in the current environment. The pros of this method include its simplicity and effectiveness, while the cons involve its limitation to checking only variables in the current workspace unless combined with other commands.
Utilizing the `evalin` Function
When you need to verify the existence of a variable in a different workspace, the `evalin` function is your go-to. This function allows for checking variables in either the base workspace or calling functions.
- Syntax: `evalin('workspace', 'exist(''variable'', ''var'')')`
- By changing 'workspace' to 'base', you can check for the existence of a variable globally.
Example Code Snippet:
if evalin('base', 'exist(''myVar'', ''var'')')
disp('Variable exists in the base workspace');
else
disp('Variable does not exist in the base workspace');
end
This method is particularly useful in GUI applications or when dealing with nested functions, where the variable might not be accessible directly.
Implementing `isfield` for Structs
If you're working with structures in MATLAB, checking for fields is also essential. In this case, you can use the `isfield` function.
- When to use: Use `isfield` when you want to verify if a specific field exists within a structure variable.
- Syntax: `isfield(structVariable, 'fieldName')`
Example Code Snippet:
myStruct = struct('field1', 10);
if isfield(myStruct, 'field1')
disp('Field exists in the structure');
else
disp('Field does not exist in the structure');
end
This method provides a clean and efficient way to verify the existence of specific fields within structures.
Using Logical Conditions with Try-Catch
Another approach to checking for a variable’s existence is employing a `try-catch` block. This method attempts to access the variable and catches any errors if the variable does not exist.
- Example Code Snippet:
try
myVar;
disp('Variable exists');
catch
disp('Variable does not exist');
end
While this method can be effective, it is not always advisable to rely on error handling for flow control, as it could lead to less readable and less efficient code.
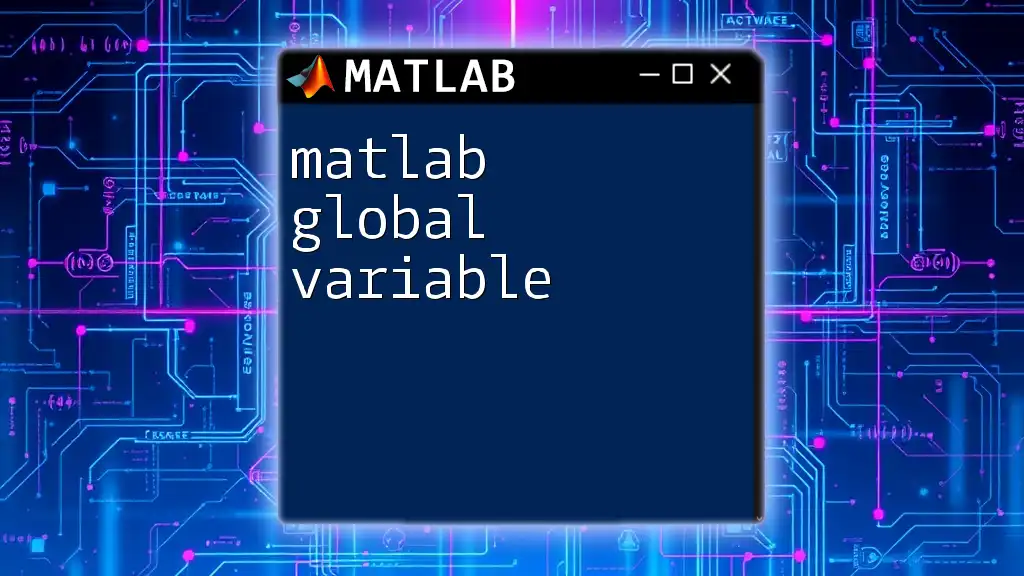
Best Practices for Checking Variable Existence
Always Initialize Your Variables
Ensuring that your variables are initialized before use greatly reduces the number of runtime errors related to variable existence. By assigning a default value upon declaration, you provide a safety net that confirms the variable is valid.
Use Meaningful Variable Names
Utilizing clear and descriptive names for your variables can significantly reduce confusion, which often leads to accidentally referencing non-existent variables. A well-named variable can provide context, making your code easier to read and understand.
Error Handling
Incorporating error handling in your code can help gracefully manage situations where a variable may not exist. This adds robustness to your code and minimizes disruptions in the program flow.
Regular Code Review
Engaging in routine code reviews is an excellent practice. It helps identify potential issues surrounding variable usage, ensures adherence to coding standards, and promotes better overall programming practices.
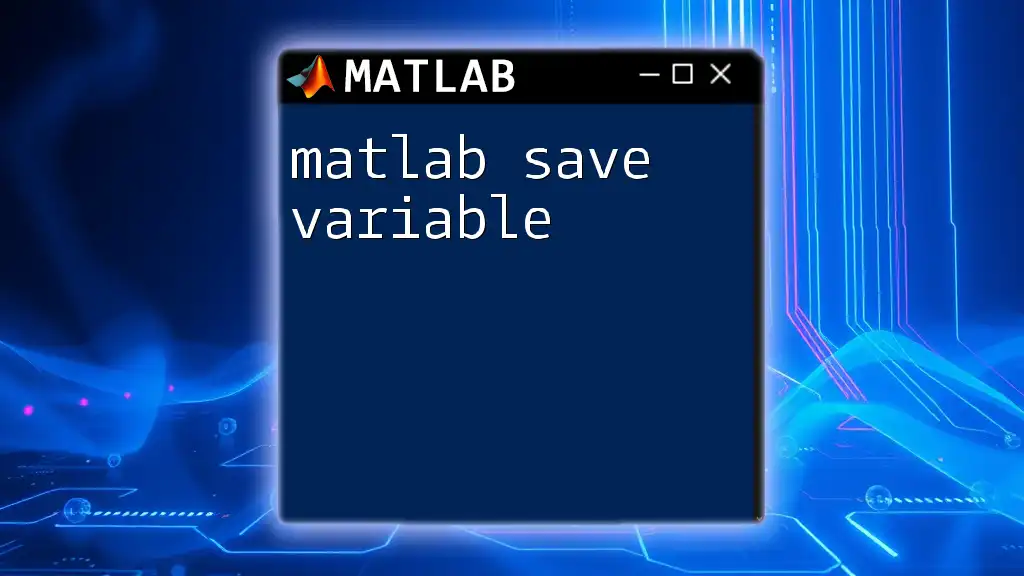
Conclusion
In summary, checking for variable existence in MATLAB is a fundamental skill that can save time and prevent errors in your code. With methods such as `exist`, `evalin`, `isfield`, and try-catch blocks, you have a range of tools at your disposal to manage this vital aspect of MATLAB programming efficiently. Pursuing best practices like initializing variables, using meaningful names, and regular code reviews will further enhance your coding effectiveness. Implement these techniques in your MATLAB routines to ensure smooth and error-free programming experiences.
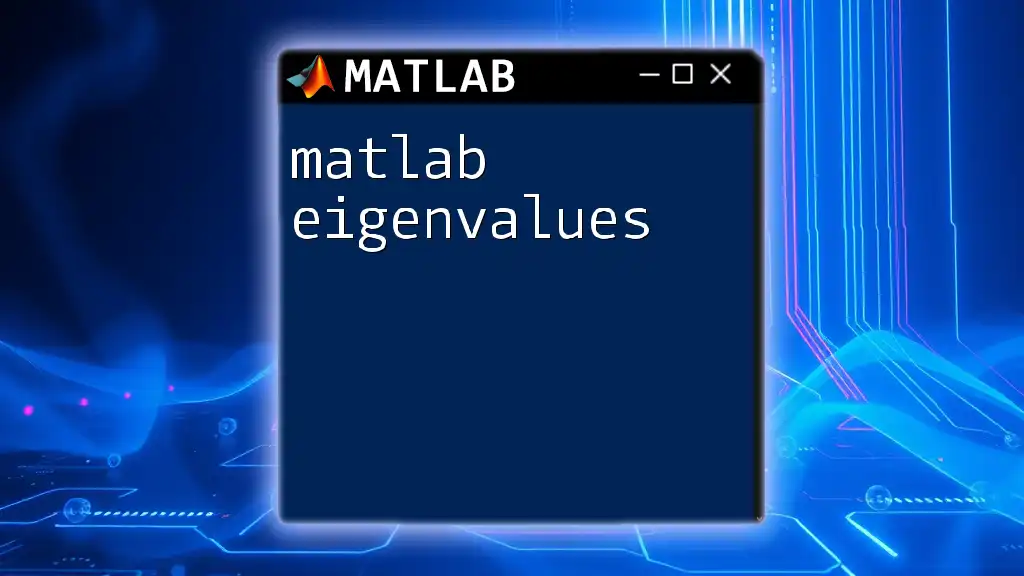
Additional Resources
For further information, consider diving into the official MATLAB documentation and exploring additional learning materials that provide insight into effective MATLAB usage. This foundational knowledge will equip you with the necessary skills to navigate variable handling and much more in MATLAB programming.
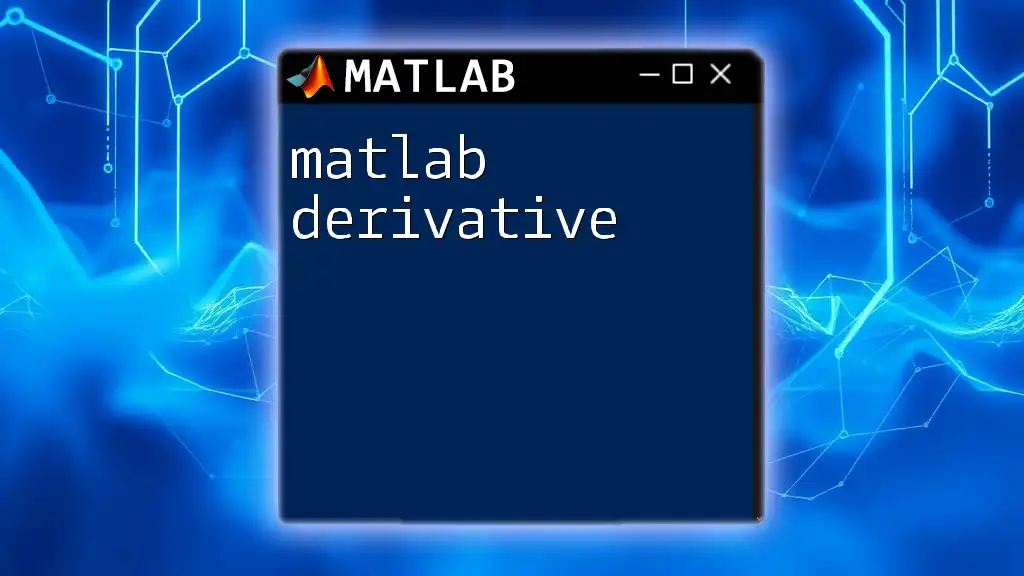
Call to Action
Stay tuned for more articles on mastering MATLAB commands. Share your experiences, insights, and any questions you may have about variable management in MATLAB!