An "if statement" in MATLAB is a conditional statement that executes specific code if a given condition evaluates to true.
Here’s an example of an if statement in MATLAB:
x = 10;
if x > 5
disp('x is greater than 5');
end
Syntax of If Statements in MATLAB
What is an If Statement? A MATLAB if statement serves as a fundamental building block in the world of programming. This structure allows programmers to implement conditional logic, meaning they can dictate certain actions based on whether specific conditions are met. In essence, if statements make decisions for your code, enabling it to behave differently under varied circumstances.
Basic Structure of an If Statement
The basic syntax for an if statement in MATLAB closely resembles other programming languages. It consists of the condition that is evaluated, followed by the actions to be taken if the condition is true. Here’s a quick representation:
if condition
% action
end
In this structure:
- Condition: This is the expression that MATLAB evaluates as either true or false.
- Action: This is the operation executed if the condition holds true.
- End Keyword: It signifies the conclusion of the if statement.
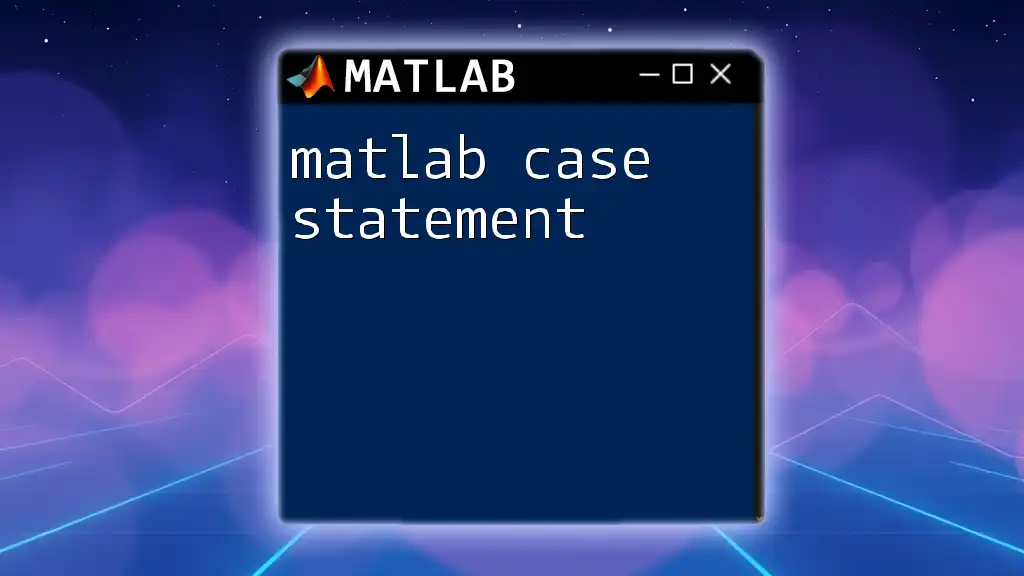
Types of If Statements
Simple If Statement
A simple if statement is the most straightforward form. It consists only of a condition and an action executed if that condition is true. For example:
x = 10;
if x > 5
disp('X is greater than 5');
end
In the snippet above, the action `disp('X is greater than 5');` executes only if `x` is indeed greater than 5. If not, there’s no output or action taken.
If-Else Statement
With the if-else structure, you can provide an alternative action if the original condition evaluates to false. This dual approach adds more versatility to your code. Here’s how it looks:
x = 3;
if x > 5
disp('X is greater than 5');
else
disp('X is not greater than 5');
end
In this code, if `x` is greater than 5, the program will display that it is true; otherwise, it will express that it is not.
If-ElseIf-Else Statement
The if-elseif-else statement allows for multiple conditions to be checked, creating a more complex decision-making tree. This structure is vital for scenarios where multiple outcomes are possible. Here is an example:
x = 0;
if x > 0
disp('X is positive');
elseif x < 0
disp('X is negative');
else
disp('X is zero');
end
This code checks if `x` is positive, negative, or zero, demonstrating the flexibility and power of conditional statements in your MATLAB scripts.
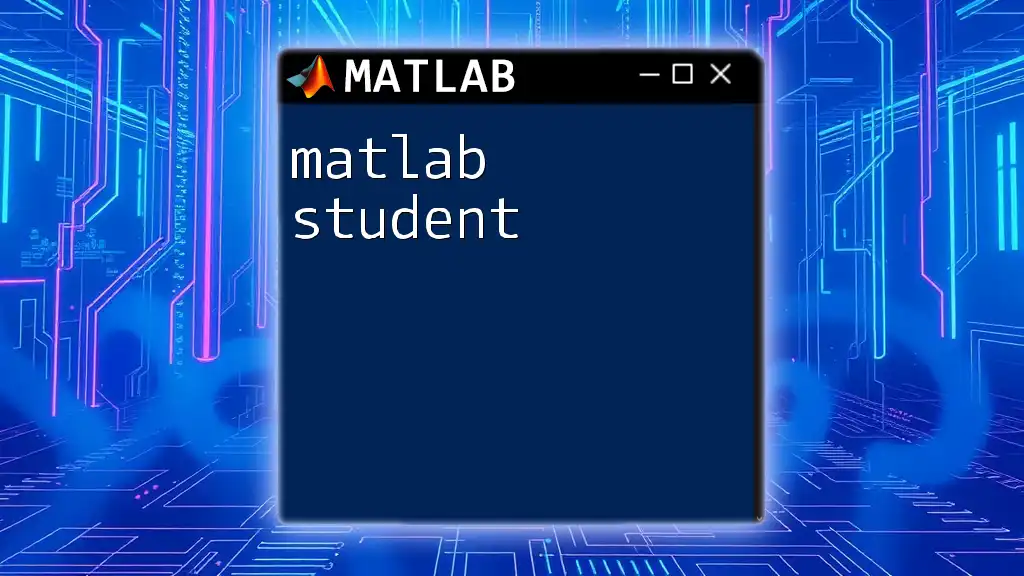
Nested If Statements
What are Nested If Statements?
Nested if statements occur when an if statement resides within another if statement. This functionality is useful for testing multiple layers of conditions or criteria. They can make your code more organized when dealing with complex logic flows.
Example of Nested If Statements
Here’s how nested if statements look:
x = 7;
if x > 0
disp('X is positive');
if mod(x, 2) == 0
disp('X is even');
else
disp('X is odd');
end
else
disp('X is not positive');
end
In this example, the code first checks if `x` is greater than zero. If this condition is true, it further checks if `x` is even or odd, showcasing a clear hierarchical logic flow through nested conditions.
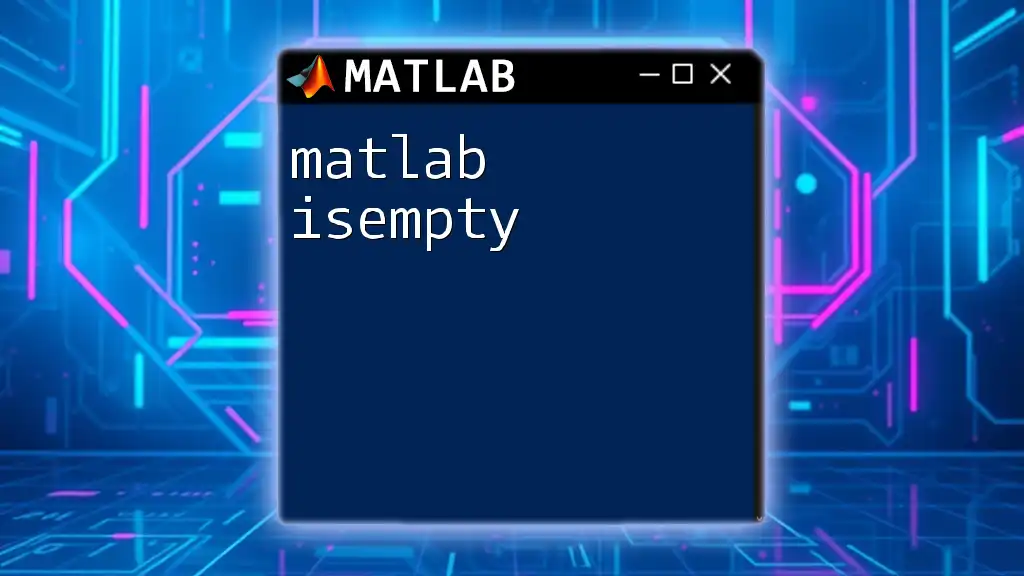
Logical Operators in If Statements
Using Logical Operators
MATLAB supports several logical operators that enhance the functionality of if statements. Understanding these operators can immensely improve condition checking:
- AND (`&&`): Evaluates to true if both conditions are true.
- OR (`||`): Evaluates to true if at least one condition is true.
- NOT (`~`): Reverses the truth value of a condition.
Examples with Logical Operators
Using logical operators can refine the simplicity of your conditions. For instance:
x = 7;
if x > 0 && mod(x, 2) == 0
disp('X is positive and even');
else
disp('X does not meet both conditions');
end
In the above example, the action only takes place if `x` is both positive and even. This scenario efficiently combines multiple conditions into a single, compound decision.
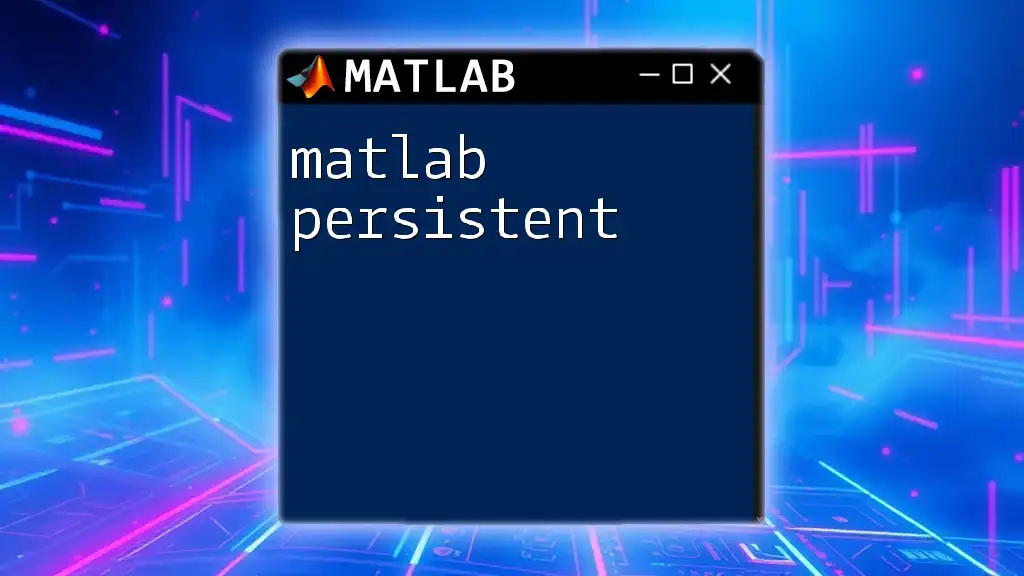
Best Practices for Using If Statements
Keep it Simple
Simplicity is the key to effective programming. The clearer your conditions, the easier it is for others (or future you) to understand your code. Avoid convoluted conditions that might confuse the reader.
Readability and Maintainability
Always aim for clear, readable code. Utilize proper indentation and spacing so that the structure of your if statements is visually appealing and easy to follow. Being mindful of how others will read your code can significantly enhance maintainability.
Using Comments
Integrate comments generously, especially in complex logical structures. A well-placed comment can clarify the purpose and logic of your code, making it more accessible for collaborators or yourself when revisiting it in the future.
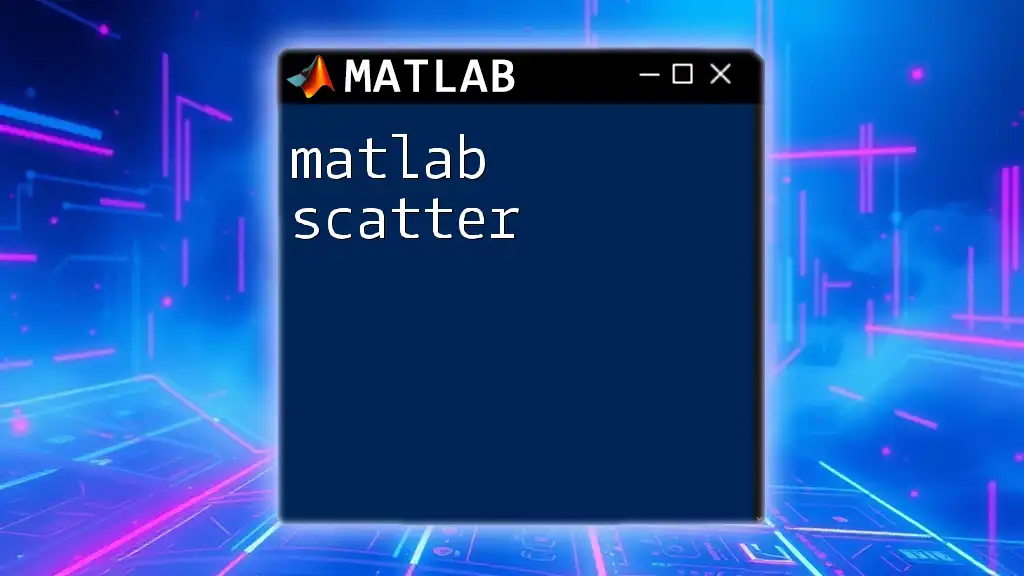
Common Mistakes to Avoid
Not Using the End Statement
One frequent error is neglecting to use the `end` statement to close out the if block. Failing to include it will result in syntax errors.
Confusing Logical Operators
Another common pitfall occurs when developers confuse the AND and OR operators, leading to erroneous outcomes. Pay close attention to operator usage to avoid confusing logic mishaps.
Overcomplicating Conditions
While it might be tempting to create intricate conditions, strive for simplicity and clarity. Overly complex conditions lead to bugs and make the code harder to debug or maintain.
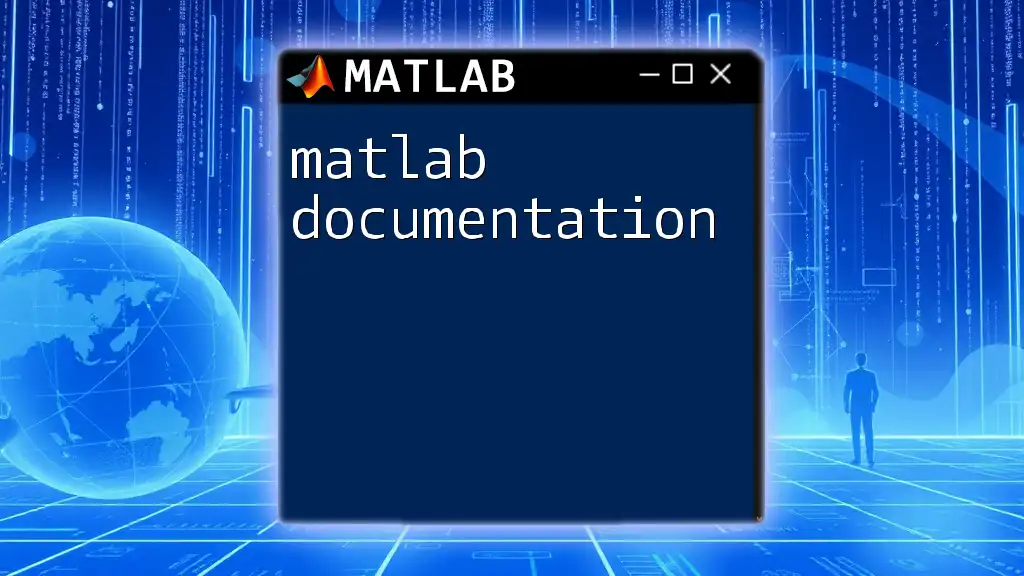
Practical Applications of If Statements
Real-World Scenarios
If statements are widely applicable in various programming tasks, such as input validation, workflow automation, and decision-making processes in data processing, simulation control, or algorithm development.
Data Processing and Analysis
In data analysis, if statements can help filter data or trigger specific actions based on dataset conditions. For instance, they can determine how to handle missing values or adjust processing techniques based on data characteristics.
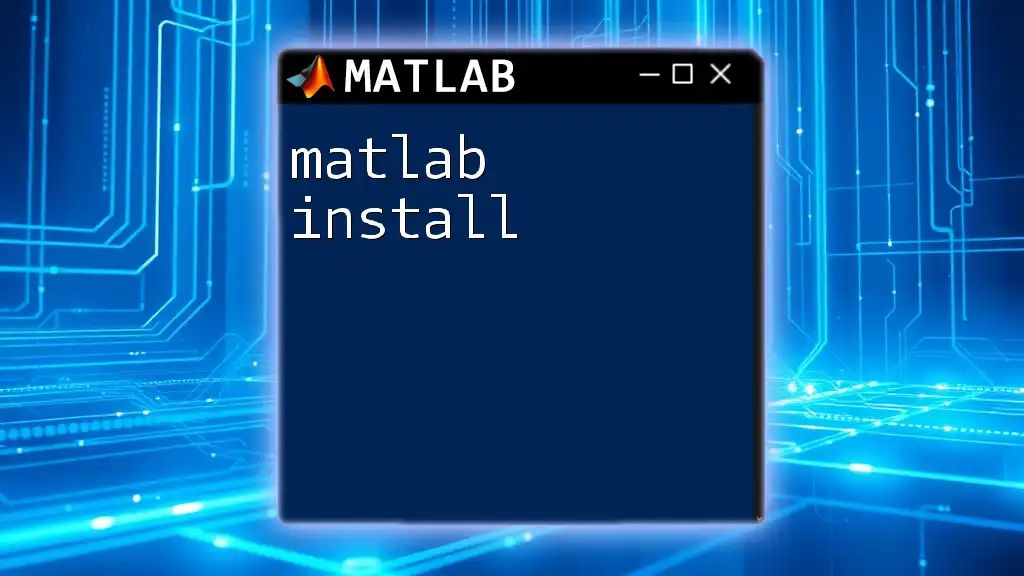
Conclusion
Understanding and effectively using the MATLAB if statement is pivotal in enhancing the functionality of your scripts. With practice, you’ll quickly grasp how to implement these conditional checks in your coding toolkit. Keep experimenting with different structures and conditions to reinforce your understanding and capabilities in using if statements creatively and efficiently.
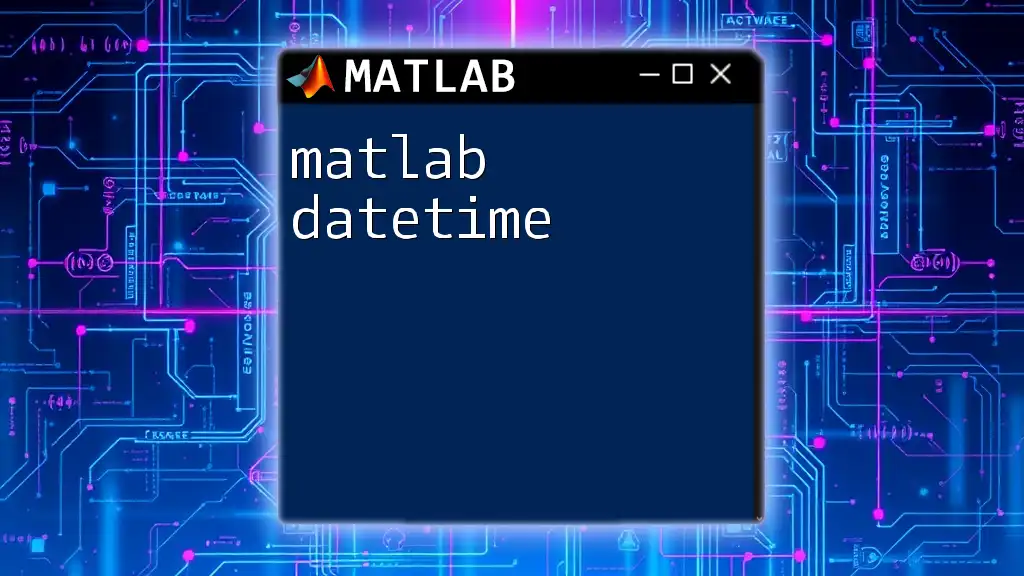
Additional Resources
Further Reading and References
For more detailed insights, consult the official MATLAB documentation, which offers a wealth of information on conditional statements.
Video Tutorials and Community Forums
Explore additional video resources or engage in community forums for collaborative learning and troubleshooting advice. Learning from a community can significantly enhance your programming journey, especially with complex topics like conditional statements.