A global variable in MATLAB is a variable that is accessible from any function or workspace, allowing for shared data across different functions without needing to pass it as an argument.
Here's a simple example of how to declare and use a global variable in MATLAB:
% Declare the global variable
global myGlobalVar;
% Assign a value to the global variable
myGlobalVar = 10;
% Function to display the global variable
function displayGlobalVar()
global myGlobalVar; % Access the global variable
disp(myGlobalVar);
end
% Call the function
displayGlobalVar(); % This will output: 10
Understanding Variable Scope in MATLAB
Local vs. Global Variables
In MATLAB, local variables are those that are defined within a specific function or workspace. Their visibility is limited to that function or workspace, meaning they cannot be accessed from other functions unless explicitly passed as arguments. This localized use helps in maintaining the integrity of data but can be restrictive when you want to share information across multiple functions.
On the other hand, global variables are accessible from any function or workspace within the same MATLAB session. They are denoted by the `global` keyword, providing a mechanism to share data easily. While this can enhance efficiency in data sharing, global variables come with risks—such as unintentional modification which can lead to harder-to-trace bugs.
Scope of Variables
The concept of variable scope is crucial in programming. It refers to the context within which a variable can be accessed. In MATLAB, the scope can either be:
- Local: The variable is only accessible within the function where it is defined.
- Global: The variable is accessible from any function.
Understanding the implications of variable scope is essential when deciding whether to use a global variable. Effective use can streamline processes, whereas poor management can lead to confusion and unpredictable results.
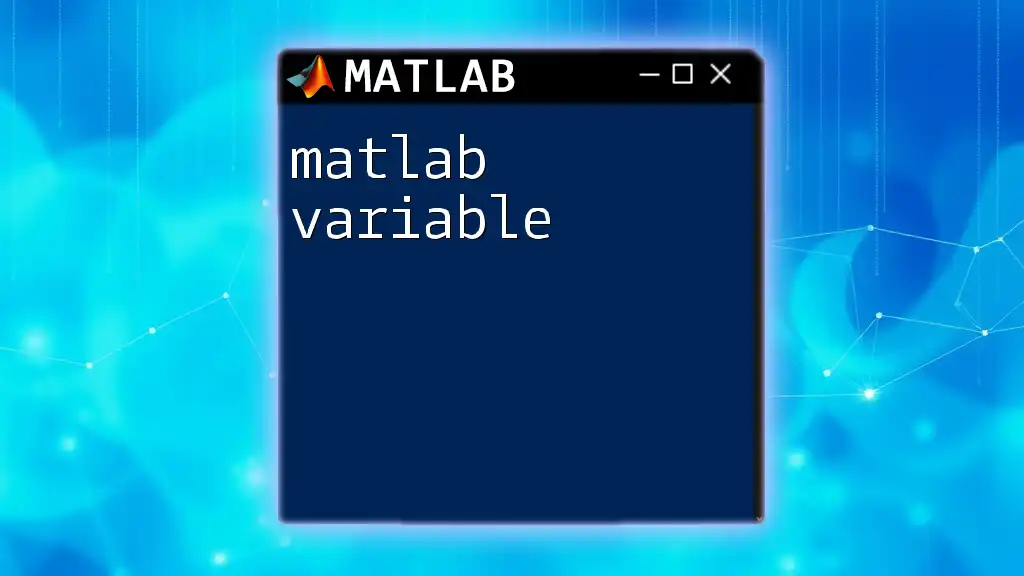
Implementing Global Variables in MATLAB
Declaring Global Variables
To declare a global variable in MATLAB, you simply use the `global` keyword. The declaration needs to occur in every function that intends to use the global variable. The syntax is straightforward:
global myVar
Accessing Global Variables
When using global variables, it's necessary to access them within the context of functions. Here's a practical example:
function myFunction()
global myVar
myVar = 10; % Assigning a value to the global variable
end
In this example, `myVar` is declared as global in `myFunction`, allowing it to store a value that can be accessed elsewhere. Consider this scenario where global variables are used across multiple functions:
global myVar
function setGlobalVar(value)
global myVar
myVar = value;
end
function displayGlobalVar()
global myVar
disp(myVar);
end
In this code snippet, `setGlobalVar` changes the value of `myVar`, while `displayGlobalVar` allows you to view it. If we call `setGlobalVar(20);` followed by `displayGlobalVar();`, it will display `20`.
Modifying Global Variables
Modifying a global variable isn't complicated once it's declared. You can change its value in any function where it’s declared global. Here's an example:
setGlobalVar(20);
displayGlobalVar(); % Outputs: 20
As you can see, the global variable `myVar` retains its value across functions, thus demonstrating the convenience of using global variables.
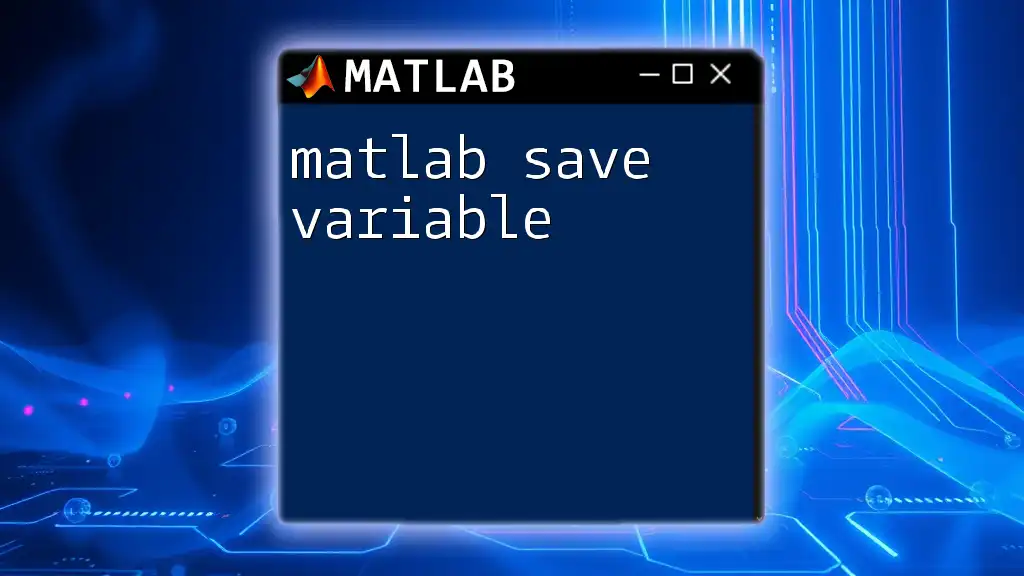
Practical Applications of Global Variables
Use Cases for Global Variables
Global variables can be beneficial in scenarios where multiple functions need to access and modify the same data without passing it explicitly as a parameter. For example, they can be used for tracking app configurations or user settings throughout the program's lifetime.
However, you should exercise caution when using global variables as they can lead to unexpected behavior and bugs, especially in larger programs where many functions might read or modify the same variable. To avoid conflicts, always strive for clarity regarding which functions will use which global variables.
Best Practices
When utilizing global variables in MATLAB, adhering to certain best practices can help maintain clean and manageable code. Here are some guidelines:
- Naming Conventions: Use clear and descriptive names for global variables. For instance, instead of `global x`, use `global totalScore` to convey the variable's purpose.
- Documentation: Comment on the purpose of each global variable within the code to assist any future developers (or yourself) in understanding its role.
- Minimize Use: Limit reliance on global variables. If you find that many functions need to access the same data, consider using alternative structures, such as structs, to group the data instead.
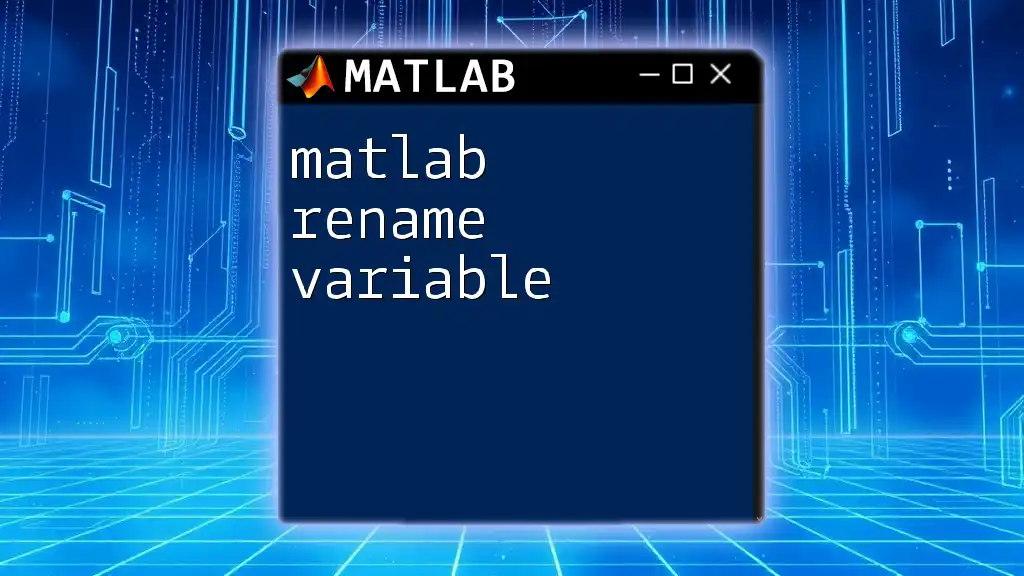
Example Project Using Global Variables
Project Overview
Let’s consider a basic project where we utilize a global variable to manage a simple counter. The goal is to demonstrate incrementing and resetting the counter through different functions.
Code Snippet for Example Project
global counter
function incrementCounter()
global counter
counter = counter + 1;
end
function resetCounter()
global counter
counter = 0;
end
In this project, we declare `counter` as a global variable. The `incrementCounter` function increases the counter's value, while `resetCounter` sets it back to zero.
Running the Project
You can run this example in MATLAB by first initializing the global variable in your command window:
global counter
counter = 0; % Initialize the counter
Now, after you call `incrementCounter`, you can check the value of `counter`:
incrementCounter(); % Increment counter by 1
disp(counter); % Outputs: 1
incrementCounter(); % Increment counter again
disp(counter); % Outputs: 2
resetCounter(); % Reset counter to 0
disp(counter); % Outputs: 0
This illustrates how global variables sustain their values across function calls and how they can facilitate quick access to shared data.
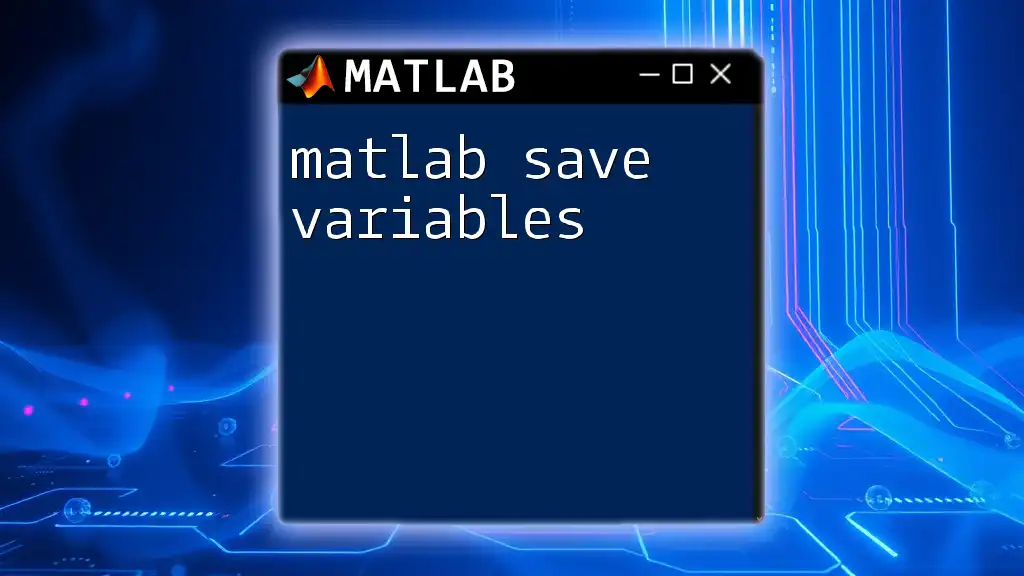
Conclusion
In summary, MATLAB global variables offer a robust means to manage data shared across functions. Understanding their scope, usage, and the implications of their accessibility is crucial for effective programming. While they provide undeniable convenience, it is essential to approach global variables judiciously to avoid potential pitfalls and maintain code clarity.
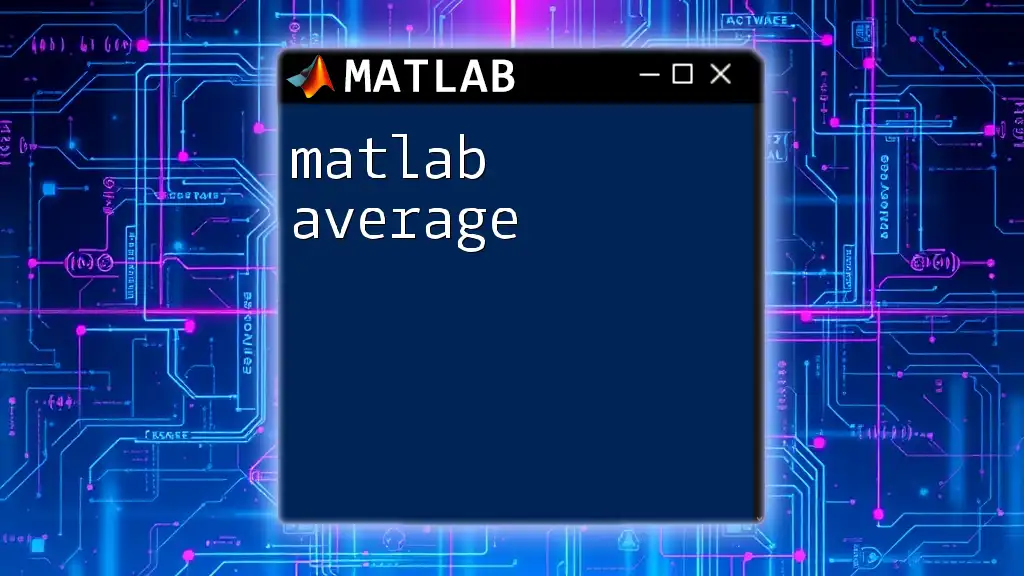
Additional Resources
For further exploration and a deeper understanding of global variables in MATLAB, consider referring to the official [MATLAB documentation](https://www.mathworks.com/help/matlab/matlab_globals.html) on global variables. Learning through tutorials and video lectures can also enhance your proficiency in MATLAB programming.
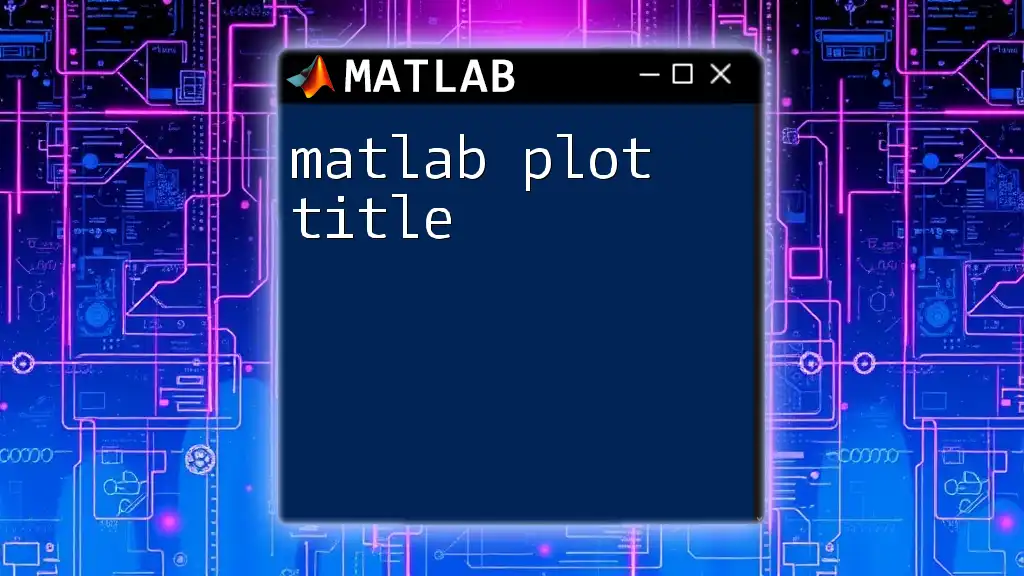
FAQs About MATLAB Global Variables
Common Questions
What happens if I forget to declare a variable as global?
If you don't declare a variable as global inside a function, you cannot manipulate the variable from that function, leading to an undefined variable error.
Are there alternatives to using global variables in MATLAB?
Yes, alternatives include using function parameters, persistent variables, or structs to encapsulate and manage related data, which can provide greater control and reduce the risk of unintentional modifications.