The `readtable` function in MATLAB is used to import data from a file into a table format, which can then be easily plotted for visualization; here’s a quick example:
data = readtable('datafile.csv'); % Read data from CSV file
plot(data.Var1, data.Var2); % Plot variable 1 against variable 2
xlabel('Variable 1'); % Label X-axis
ylabel('Variable 2'); % Label Y-axis
title('Plot of Variable 1 vs Variable 2'); % Add a title
Understanding `readtable`
What is `readtable`?
The `readtable` function in MATLAB is designed to facilitate the importing of data from a variety of file formats into MATLAB as a table. This allows for efficient manipulation and analysis of data, especially when dealing with tabular datasets like CSV or Excel files. By organizing the data into a table, columns can be referred to by their names, leading to an intuitive and readable code structure for later analysis.
Basic Syntax of `readtable`
The basic syntax for using `readtable` is quite straightforward:
T = readtable(filename);
In this example, `filename` is a string representing the path to your data file. The function returns `T`, a table consisting of the data read from the specified file. You can further customize the table import using additional parameters such as:
- `opts`: A structure for specifying import options.
- `Sheet`: If reading from Excel, specify the sheet name.
Examples of Using `readtable`
Example 1: Reading a CSV File
To read a simple CSV file, you can use the following command:
T = readtable('data.csv');
This command will automatically convert the rows of data into a table `T`, which MATLAB structure easily interprets. You will find the columns named according to the headers in your CSV file, making it intuitive to access individual data points.
Example 2: Reading an Excel File
If you're importing data from an Excel file, you can specify the sheet as follows:
T = readtable('data.xlsx', 'Sheet', 'Sheet1');
This imports data from "Sheet1" of the Excel file. Utilizing the sheet option allows for flexibility when dealing with multi-sheet Excel documents.
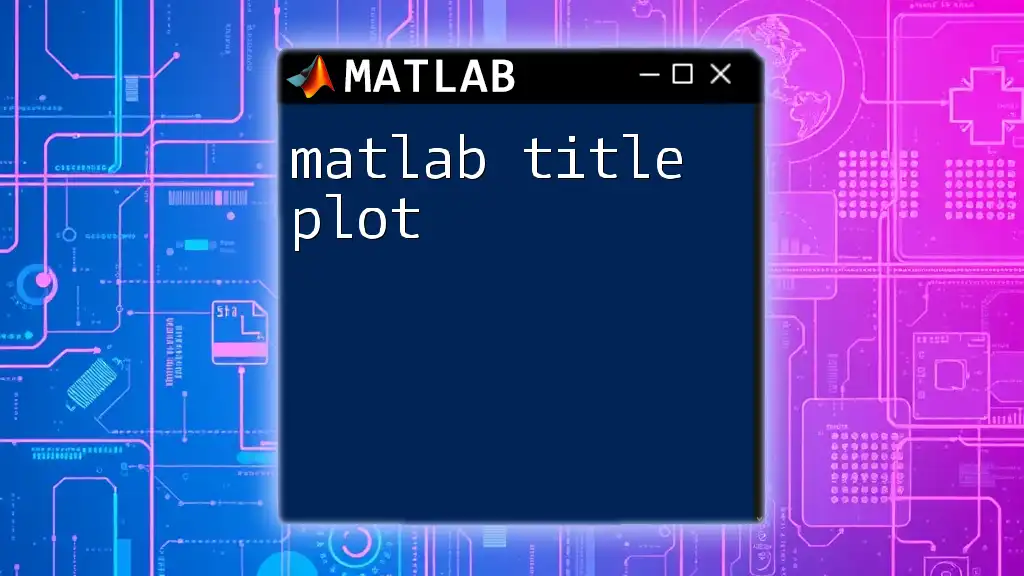
Data Preparation
Inspecting the Table
Once you have imported your data, it's crucial to inspect it before proceeding to plotting. MATLAB offers several functions for this purpose:
- `head(T)`: Displays the first few rows of the table, allowing you to quickly check the data structure.
- `tail(T)`: Shows the last few rows, helping to ensure there are no unexpected entries.
Familiarizing yourself with the data layout will enable you to make better decisions when creating your visualizations.
Cleaning the Data
Handling Missing Values
Data cleaning is a significant step, especially if your dataset contains missing values. The `rmmissing` function is beneficial in this case:
T_cleaned = rmmissing(T);
This command removes any rows that contain missing values in the table `T`, resulting in a cleaner dataset for plotting.
Data Type Conversion
At times, the data may not be in the intended format. You can check and convert variable types to ensure they match your expectations:
T.varName = str2double(T.varName);
This one-liner effectively converts `varName` from string to double, accommodating operations requiring numerical data.
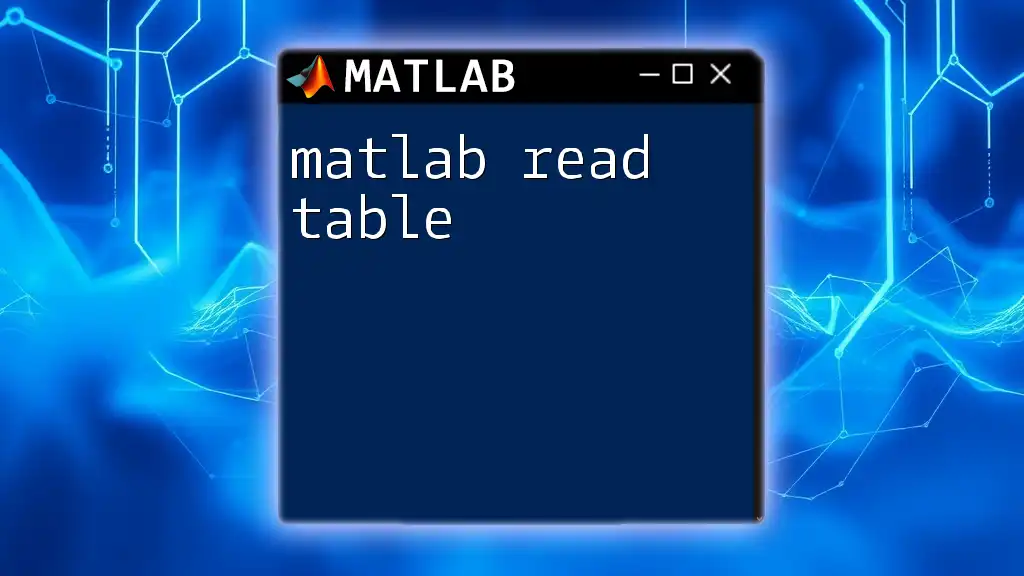
Creating Plots with MATLAB
Basic Plotting Functions
Using the `plot` Function
Creating a basic plot in MATLAB is straightforward. To visualize time series data, for instance, you could use:
plot(T.Time, T.Measurement);
In this code, `Time` is represented on the x-axis and `Measurement` on the y-axis, allowing a clear representation of how measurements change over time.
Customizing the Plot
MATLAB provides an array of functions for enhancing the clarity of your plots. Consider adding titles, axis labels, and legends:
title('My Plot Title');
xlabel('Time');
ylabel('Measurement');
legend('Data Series');
These additions transform a simple plot into a comprehensive visual, making it easier to understand and interpret.
Advanced Plotting Techniques
Subplots
When working with related data series, using subplots can offer comparative insights. For instance:
subplot(2,1,1);
plot(T.Time, T.Var1);
subplot(2,1,2);
plot(T.Time, T.Var2);
In this example, two plots are displayed vertically in one figure, enabling a direct comparison between `Var1` and `Var2`.
Customizing Plot Aesthetics
MATLAB allows for extensive customization of plots to enhance visual appeal. You can adjust the style, colors, and markers:
plot(T.Time, T.Measurement, 'r--o');
This command plots `Measurement` with red dashed lines and circle markers, significantly improving the plot's legibility and aesthetics.
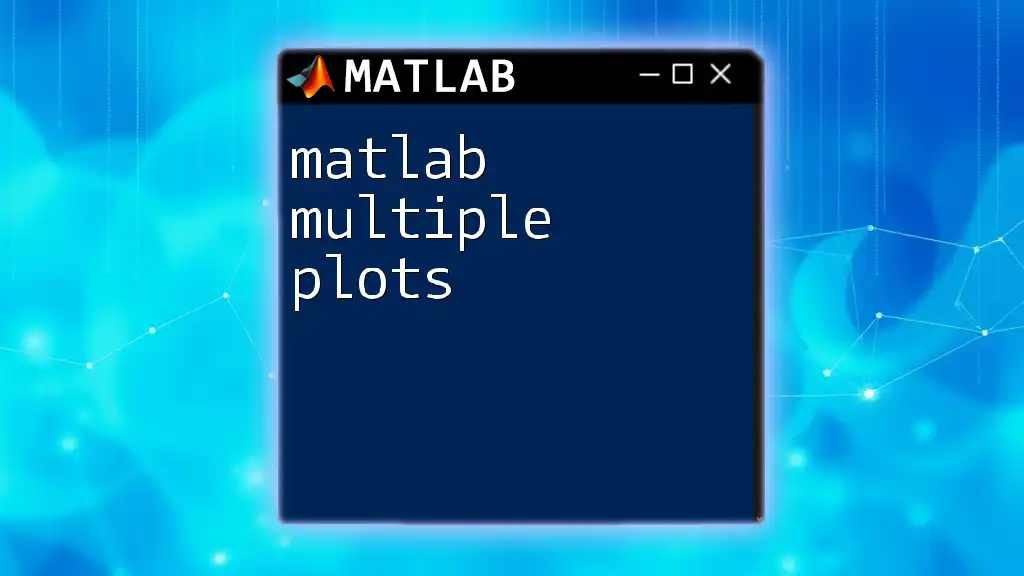
Analyzing the Plots
Interpreting the Results
After creating visualizations, interpreting the results is pivotal. Look for trends, fluctuations, or anomalies within the data. Understanding the visual output can yield valuable insights into underlying patterns and behaviors within your dataset.
Debugging Common Plotting Issues
When plotting, you may encounter common errors such as mismatched dimensions or non-existent variable names. To debug, check the table structure using `T.Properties.VariableNames` to ensure you've referenced column names correctly. Adjust your plot commands accordingly to correct any issues.
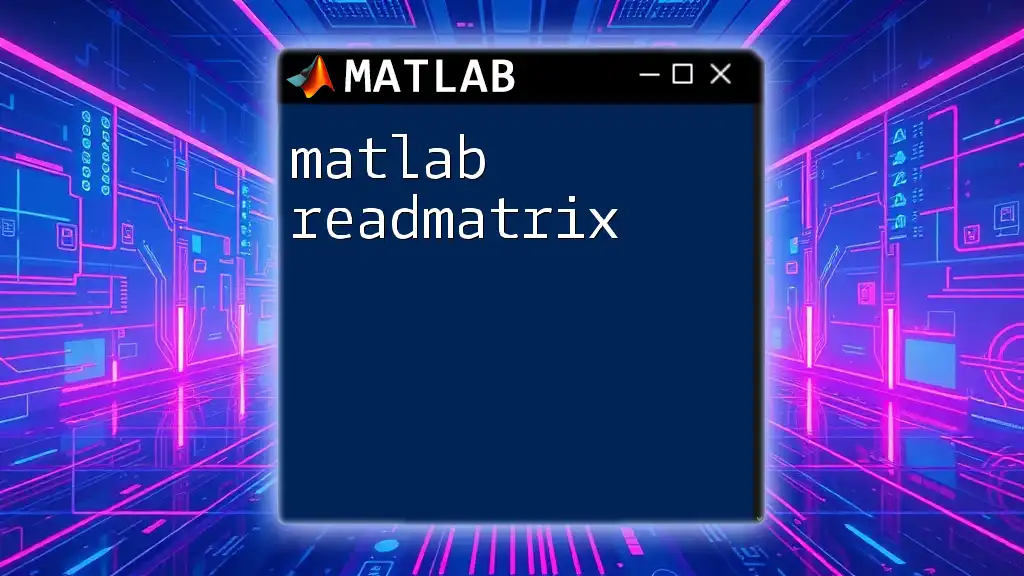
Conclusion
The `readtable` function is a powerful tool within MATLAB, transforming raw data into a structured format suitable for analysis and visualization. By effectively using the plotting capabilities of MATLAB, you can create meaningful visualizations that enable effective data interpretation. Experimenting with the examples provided will empower you to harness the full potential of MATLAB for your data analysis needs.
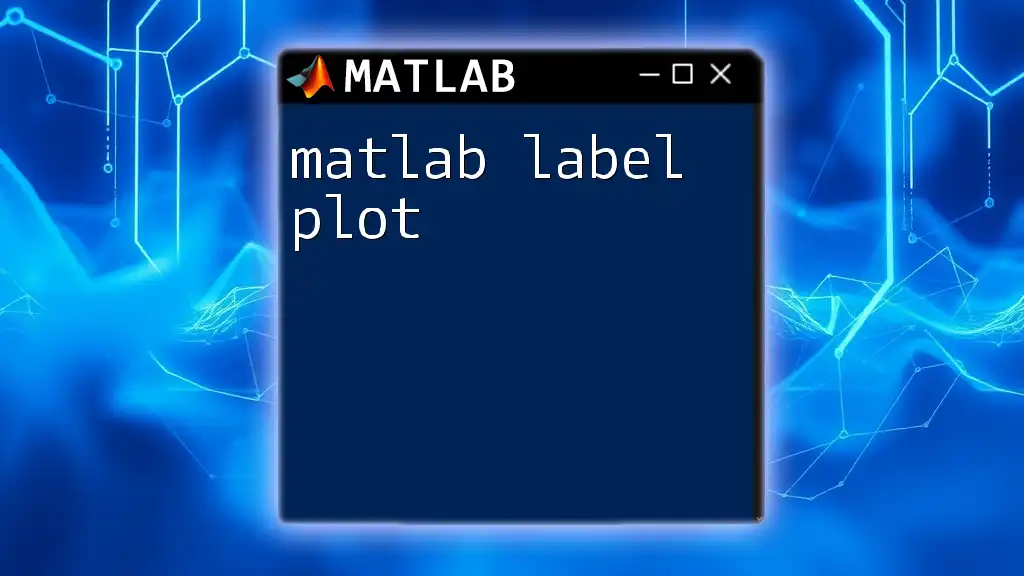
Additional Resources
Helpful MATLAB Functions
Beyond `readtable`, several other functions enhance data handling and visualization, such as `scatter`, `histogram`, and `boxplot`. Exploring these options expands your analytical toolkit.
Further Reading
For more in-depth knowledge, consider visiting the official MATLAB documentation and engaging with community forums to share insights and learn from others in the field.