In MATLAB, you can save variables to a file for later use using the `save` command, which stores the specified variables in a .mat file.
save('myVariables.mat', 'variableName');
Understanding the `save` Command
What is the `save` Command?
The `save` command in MATLAB is a crucial function that allows users to preserve their workspace variables. This command helps users retain data across sessions, ensuring that work is not lost and can be continued at a later date.
Why Saving Variables is Important
Saving variables serves several significant purposes in MATLAB, such as:
- Data Persistence: By saving your variables, you can easily resume your work at any point without needing to replicate calculations.
- Resource Management: Many computations can be resource-intensive. Instead of recalculating data, saving variables allows for quick access and reuse.
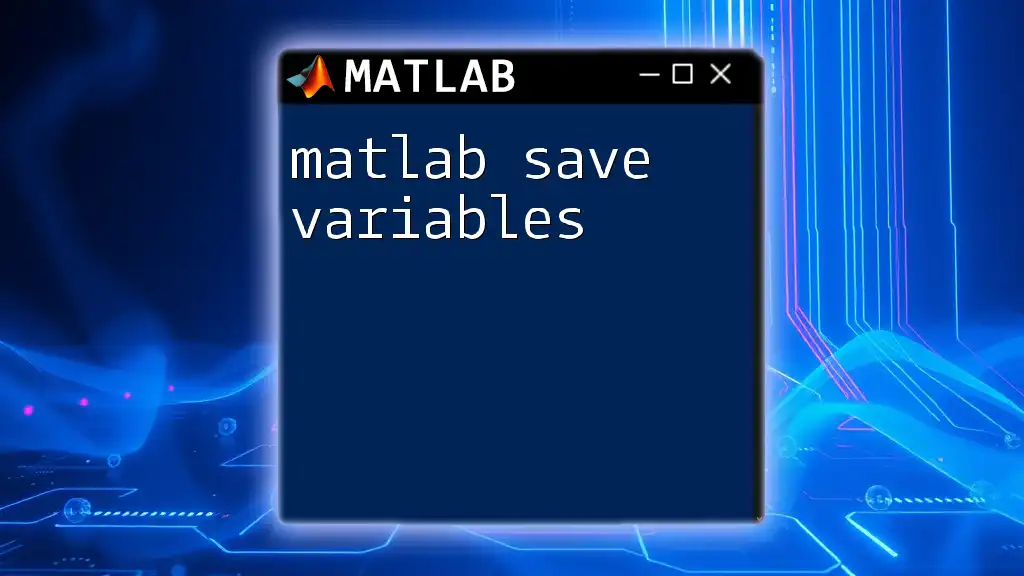
Saving Variables in MATLAB
Basic Syntax of the `save` Command
The basic syntax for the `save` command is straightforward:
save('filename.mat', 'var1', 'var2', ...)
In this command:
- File name: This specifies the name of the file in which the variables will be stored, and it is conventionally assigned an extension of `.mat`.
- Variables: List the specific variables you want to save. If you do not specify any variables, MATLAB will save all variables from the workspace by default.
Saving All Variables
You can easily save all your workspace variables without having to list them individually. To do this, simply call:
save('filename.mat')
This command is especially useful when you want to save your entire workspace state quickly. However, be cautious—using this command during a large session may result in substantial file sizes.
Saving Variables in Different Formats
Saving as `.mat` Files
The `.mat` file format is MATLAB's native format, enabling efficient storage of variables, including large matrices. This is often the preferred choice due to its compatibility and efficiency. Here’s how to save variables in a `.mat` file:
save('myData.mat', 'var1', 'var2', 'var3')
This saves `var1`, `var2`, and `var3` into `myData.mat`. The `.mat` format supports a wide range of data types, making it a great option for preserving your work.
Saving as Text or ASCII Files
In cases where compatibility with other software is essential, you might want to save variables as a text file. To do this, use the `-ascii` flag like this:
save('data.txt', 'var1', '-ascii')
This command will save the variable `var1` into a plain text file. While text files are more widely compatible, they may not work well for complex data types such as cell arrays or structures.
Specifying Data Types
When dealing with large datasets or specific data types, you might need to save your file in a particular format using options:
save('filename.mat', '-v7.3')
The `-v7.3` option allows for saving variables that exceed 2 GB in size, making it an essential feature for handling large data sets in MATLAB. Understanding and specifying the right version for your data is crucial for successful data management.
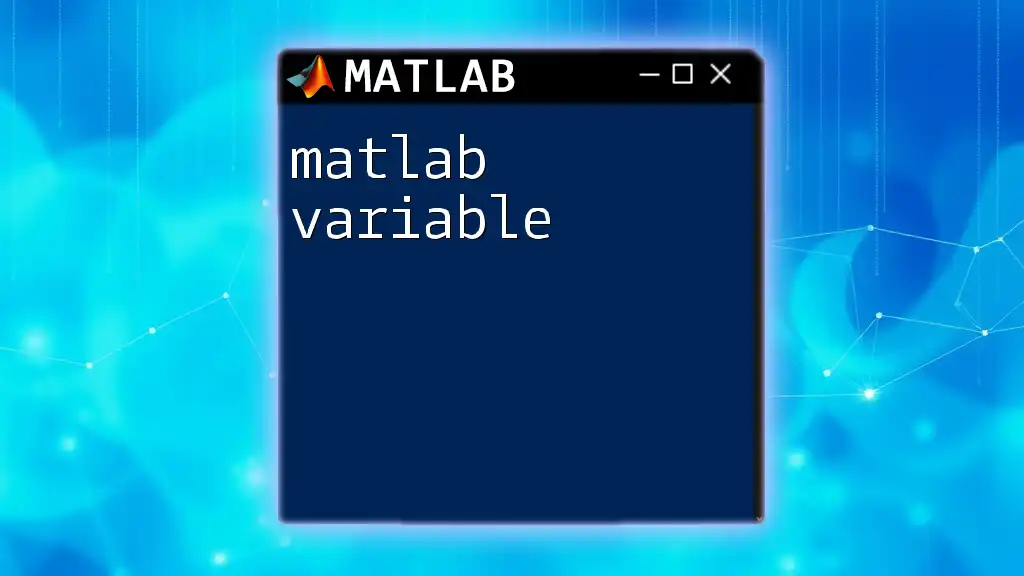
Advanced Uses of the `save` Command
Appending Data to Existing Files
Suppose you want to add new variables to an already existing `.mat` file without overwriting it. You can do this easily by using the `-append` flag:
save('myData.mat', 'newVar', '-append')
This command seamlessly incorporates `newVar` into `myData.mat`. This functionality is especially useful for iterative experiments where you collect data in stages.
Saving in a Specific Directory
To organize your files better, you may wish to save variables in a specific directory. Here’s how:
save(fullfile('path', 'to', 'directory', 'filename.mat'))
This code uses the `fullfile` function to concatenate directory paths, ensuring that files are saved in the correct folder, which helps maintain an orderly workspace.
Conditional Saving
You can also implement logic in your code to save variables conditionally. This is particularly useful to avoid errors or save only relevant variables:
if exist('var1', 'var')
save('filename.mat', 'var1')
end
In this example, `var1` is only saved if it exists in the workspace. This practice can prevent unnecessary errors, particularly when dealing with dynamic scripts.
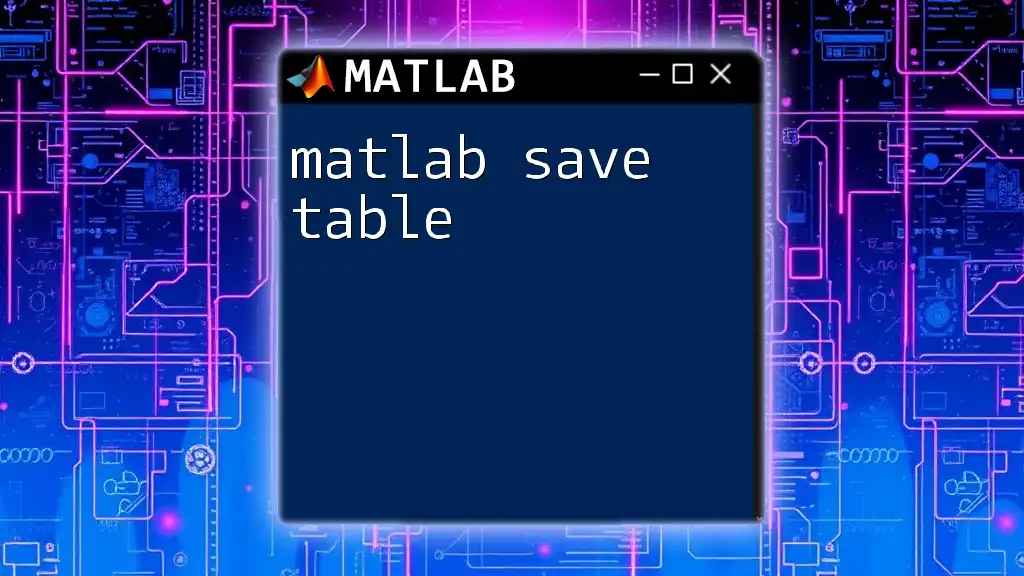
Loading Saved Variables
Using the `load` Command
Retrieving saved variables is just as straightforward as saving them. To load variables from a `.mat` file, you can use the `load` command:
load('filename.mat')
This command brings back all variables stored in `filename.mat`. Understanding how to load your saved data correctly is just as important as knowing how to save it.
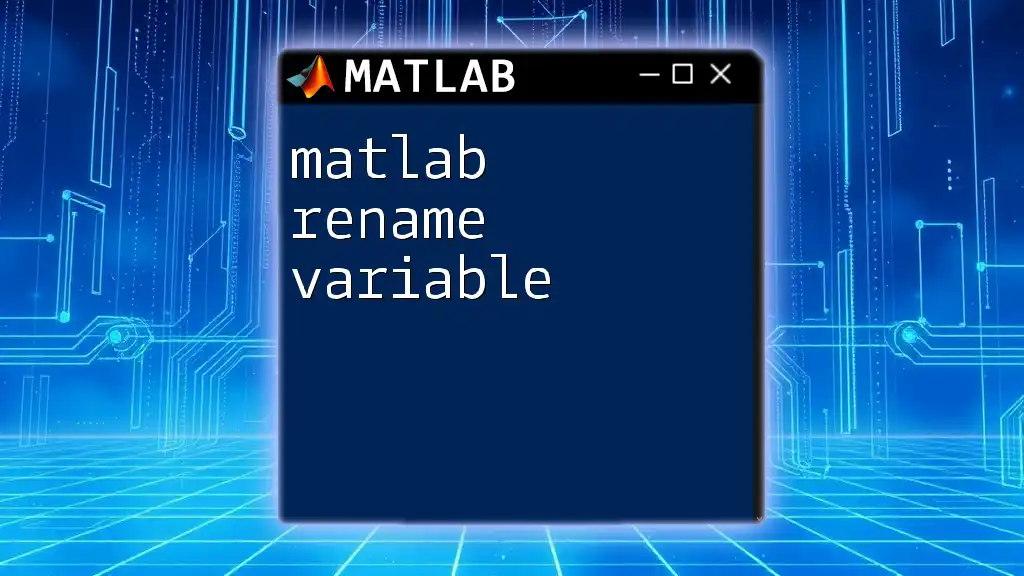
Troubleshooting Common Issues
Issues Related to File Paths
One common issue is related to the file path when using the `save` command. If MATLAB cannot find the specified directory, it will throw an error. Always check that your specified file path is correct and that you have write permissions in the target directory.
Version Compatibility Problems
When sharing `.mat` files, it's essential to consider compatibility between different versions of MATLAB. For instance, files saved in newer versions of MATLAB may not open in older versions. Always ensure that your colleagues are using compatible software versions or utilize options that enhance compatibility.
Handling Variable Overwrites
Accidentally overwriting a file can lead to lost work, which is why ensuring the uniqueness of your file names is critical. Consider using timestamps or descriptive prefixes to differentiate your files:
currentTime = datestr(now, 'yyyy-mm-dd_HH-MM-SS');
filename = sprintf('data_%s.mat', currentTime);
save(filename, 'var1');
This approach prevents overwriting by generating unique filenames based on the current time and date.
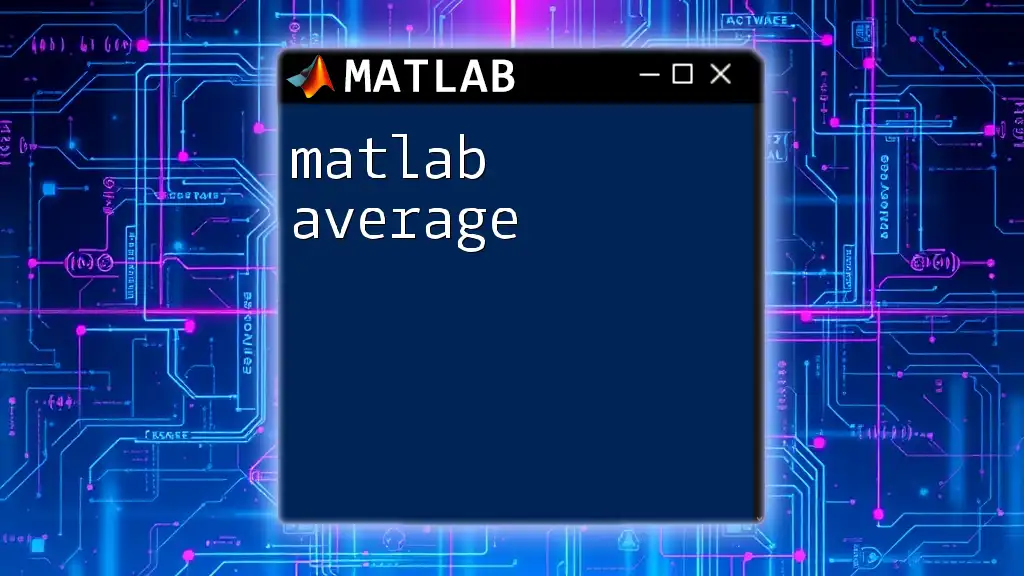
Conclusion
The `save` command in MATLAB is an essential tool for any user looking to efficiently manage and preserve their variables. By understanding its various functionalities—including saving formats, appending data, and conditional saving—you can create a more organized workflow. Practice incorporating these techniques into your own work to make your programming experience with MATLAB altogether smoother and more effective.
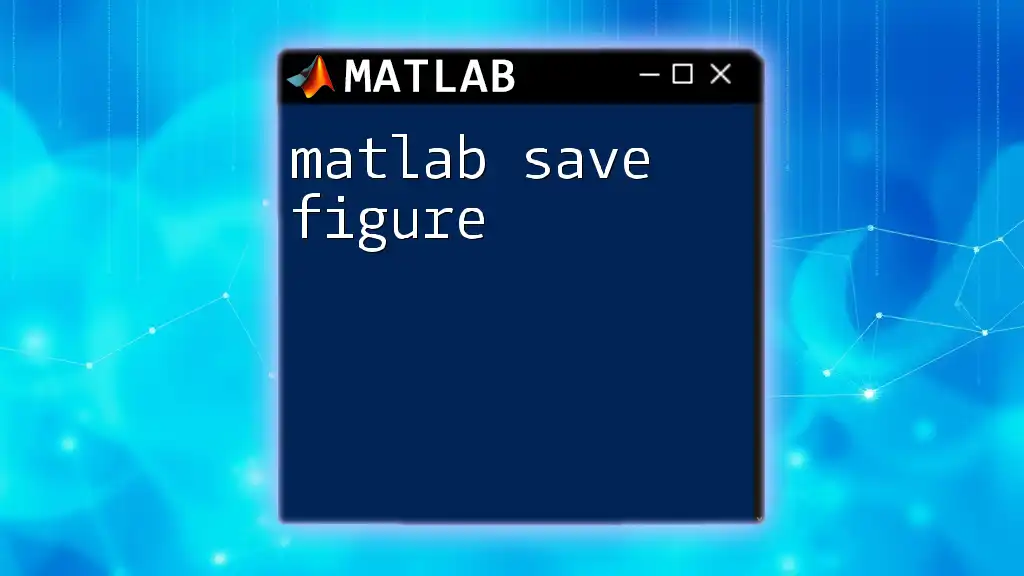
Additional Resources
For deeper insights into the `save` command and more advanced MATLAB usage, consider checking the [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/save.html) and engaging with [MATLAB forums](https://www.mathworks.com/matlabcentral/). These resources provide extensive information and community support that can help you enhance your understanding and skills.