In MATLAB, you can concatenate strings using square brackets or the `strcat` function, which combines multiple strings into a single string.
Here's a code snippet demonstrating both methods:
% Concatenating strings using square brackets
str1 = 'Hello';
str2 = 'World';
result1 = [str1, ' ', str2]; % Result: 'Hello World'
% Concatenating strings using strcat function
result2 = strcat(str1, {' '}, str2); % Result: 'Hello World'
Understanding String Types in MATLAB
Character Arrays vs. String Arrays
In MATLAB, there are two primary types of string representations: character arrays and string arrays.
- Character Arrays are a sequence of characters that are enclosed within single quotes. They allow for traditional string manipulation but can be limited in functionality and often require element-wise operations for processing.
- String Arrays, introduced in more recent versions of MATLAB, are enclosed in double quotes and offer enhanced functionality, such as better integration with functions and more intuitive manipulation.
When to Use Each Type
Character arrays are generally used when you need to work with legacy code or perform operations that require compatibility with older MATLAB functions. On the other hand, string arrays should be your go-to when you require ease of use and modern features like built-in functions to handle string operations more efficiently.
Creating Strings in MATLAB
Creating strings in MATLAB is straightforward.
For character arrays, you can define them as follows:
charArray = 'Hello, MATLAB!';
For string arrays, you would create them like this:
strArray = "Hello, MATLAB!";
Understanding how to create and differentiate these types of strings is crucial for effectively using string concatenation.
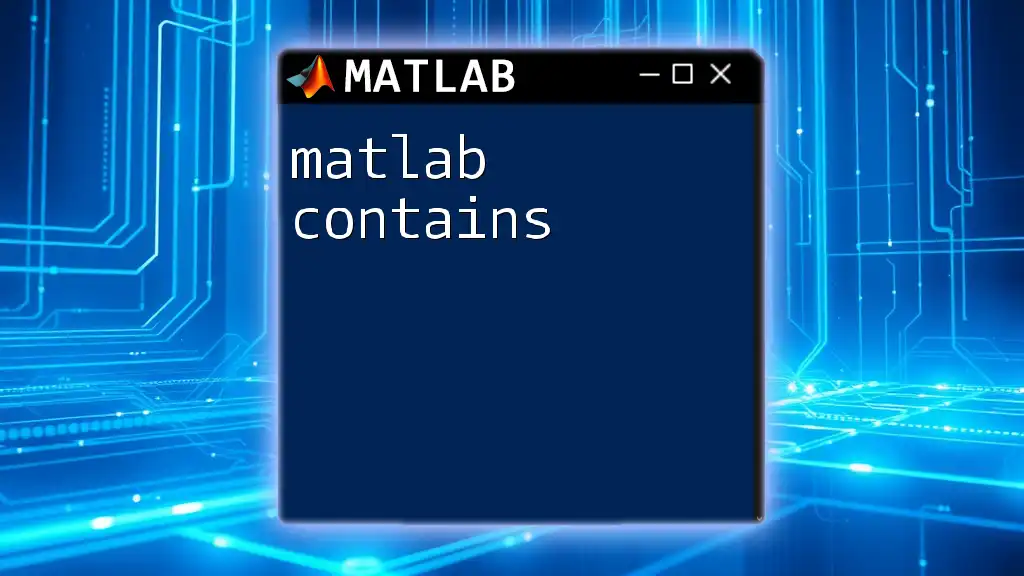
Methods for Concatenating Strings in MATLAB
Using the `strcat` Function
The `strcat` function is a common method for concatenating strings in MATLAB. This function removes any trailing whitespace from the input strings, making it ideal for cleaner outputs.
Code Example
str1 = 'Hello';
str2 = 'World';
result = strcat(str1, ' ', str2);
disp(result); % Output: Hello World
Here, the `strcat` function is used to combine two strings into a single output, separated by a space. This method is simple yet powerful for basic string manipulations.
Using the `horzcat` and `vertcat` Functions
When you need to concatenate strings horizontally or vertically, `horzcat` and `vertcat` come into play.
- `horzcat` combines arrays side-by-side.
- `vertcat` stacks arrays on top of each other.
Code Examples
charArray1 = ['Hello '; 'World '];
charArray2 = ['MATLAB'; 'Is Fun'];
resultHorz = horzcat(charArray1, charArray2);
disp(resultHorz);
resultVert = vertcat(charArray1, charArray2);
disp(resultVert);
In this example, `horzcat` combines `charArray1` and `charArray2` into a single array side by side, while `vertcat` stacks them vertically. The flexibility of `horzcat` and `vertcat` makes them valuable for formatting display outputs.
Using the `join` Function
The `join` function is another effective way to concatenate strings, especially when dealing with arrays of strings.
Code Example
strArray = ["This", "is", "a", "test"];
resultJoin = join(strArray, " ");
disp(resultJoin); % Output: This is a test
The `join` function takes a string array and concatenates its elements into a single string, separated by a specified delimiter (in this case, a space). This is particularly useful for assembling sentences or phrases from individual string elements.
Concatenating with the `+` Operator
Recent MATLAB versions allow for using the `+` operator for concatenating strings, providing a clean and intuitive syntax.
Code Example
greeting = "Hello";
place = "World";
resultPlus = greeting + " " + place;
disp(resultPlus); % Output: Hello World
Using the `+` operator simplifies string concatenation, making the code easier to read and understand. It’s an attractive option for those looking for clarity in their operations.
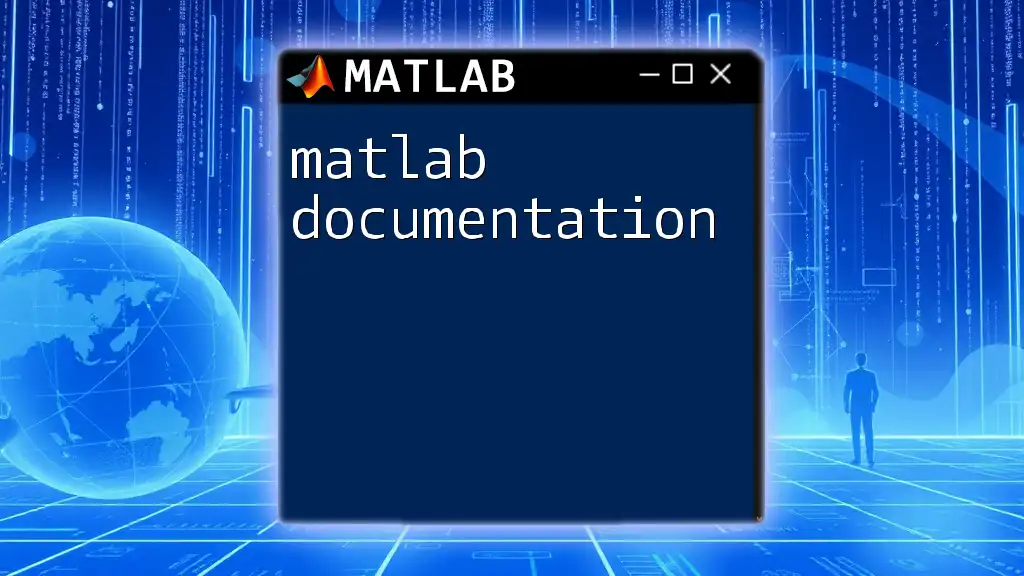
Advanced String Concatenation Techniques
Handling Cell Arrays of Strings
Cell arrays are versatile data structures in MATLAB that can hold a collection of strings of varying lengths. To concatenate strings from cell arrays, you can utilize functions like `strjoin`.
Code Example
cellArray = {'Hello', 'World', 'from', 'MATLAB'};
concatenatedCell = strjoin(cellArray, ' ');
disp(concatenatedCell); % Output: Hello World from MATLAB
The `strjoin` function allows you to concatenate strings stored in a cell array, which can be particularly beneficial for dynamic or user-generated content.
Conditional String Concatenation
You can also concatenate strings based on conditions, enabling dynamic formatting of output.
Code Example
status = true;
str1 = "Hello";
str2 = "World";
result = status * str1 + ~status * str2;
disp(result); % Output: Hello
In this example, depending on the value of the `status` variable, either `str1` or `str2` is selected for the final output. This ability to control string concatenation through conditions enhances adaptability in your code.
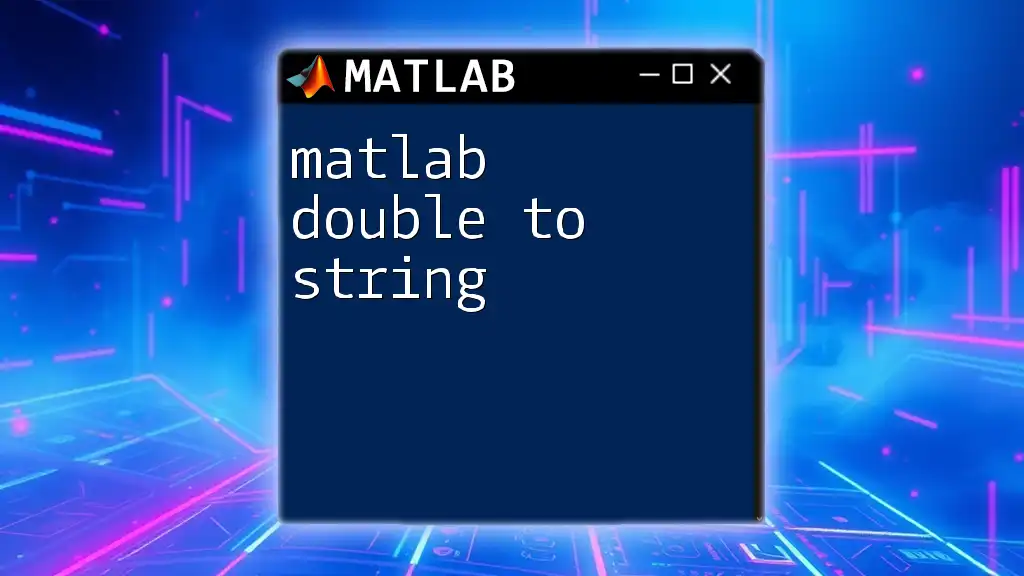
Common Errors and Troubleshooting
When working with string concatenation, it's essential to be aware of common pitfalls. A frequent error involves type mismatches, such as attempting to concatenate character arrays with string arrays without converting types.
Tips for Handling Errors
- Check Types: Use the `class` function to verify the datatype of your strings before concatenation.
- Convert Types: Use the `string` function to convert character arrays to string arrays or vice versa as needed.
- Read Error Messages: MATLAB provides informative error messages. Pay close attention to these to understand the underlying issues in your code.
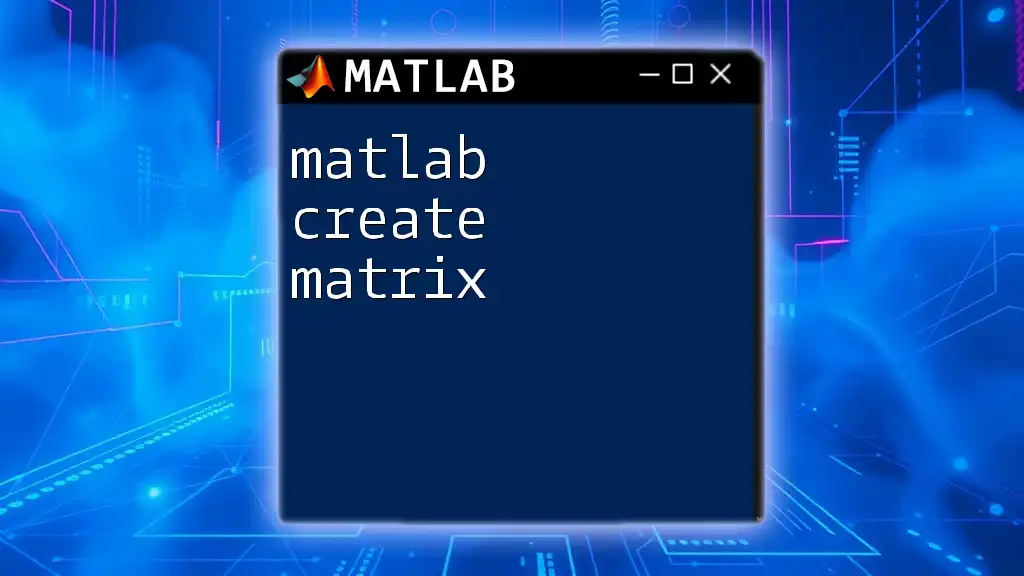
Conclusion
String concatenation in MATLAB is a versatile tool that enhances how you manage and manipulate textual data. By leveraging functions like `strcat`, `horzcat`, `join`, and the `+` operator, you can concatenate strings in various styles to suit your programming needs.
The ability to handle different types of strings, including cell arrays, offers further control and flexibility. As you experiment with these techniques, you will find creative ways to utilize string concatenation effectively in your MATLAB projects.
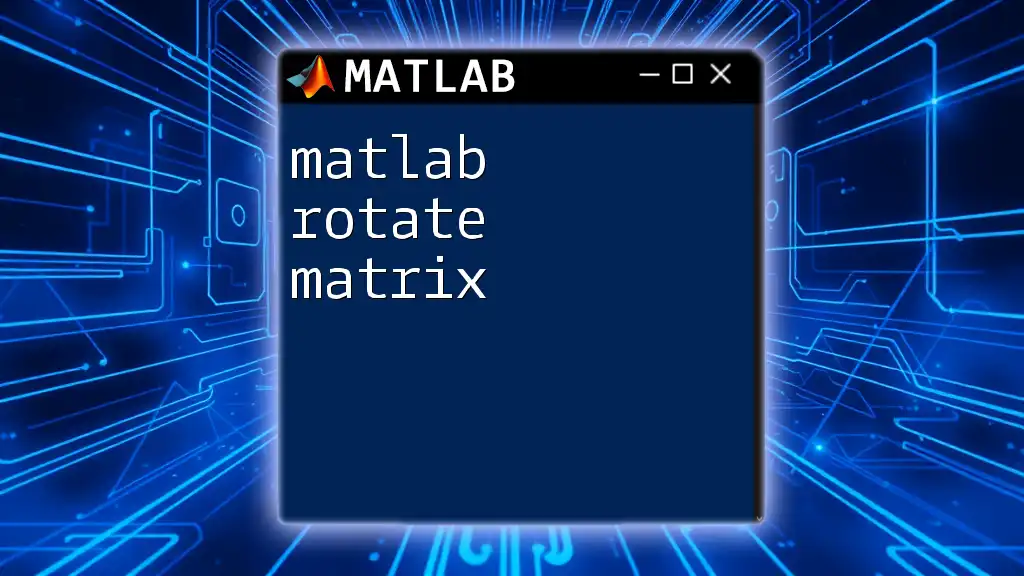
Call to Action
We encourage you to practice these concepts in your own projects! If you have questions or tips to share about string manipulation in MATLAB, please comment below. Join our MATLAB learning community for more insights, tips, and techniques on mastering MATLAB commands.