Eigenvalues are scalar values that, when multiplied by an eigenvector, represent how much the eigenvector is stretched or compressed during a linear transformation in MATLAB. Here's how to compute eigenvalues in MATLAB:
A = [4, 2; 1, 3]; % Define a matrix A
eigenvalues = eig(A); % Compute the eigenvalues of the matrix A
What are Eigenvalues?
Eigenvalues are a fundamental concept in linear algebra that arise from the study of linear transformations. Essentially, an eigenvalue can be described as a scalar \( \lambda \) associated with a linear transformation represented by a matrix \( A \). They provide insights into the characteristics of the matrix, such as how vectors are scaled when transformed by that matrix.
The mathematical definition posits that for a square matrix \( A \) and a non-zero vector \( v \), if \( Av = \lambda v \), then \( \lambda \) is an eigenvalue of \( A \), and \( v \) is the corresponding eigenvector. This means that when we apply the linear transformation \( A \) to the vector \( v \), the result is a scalar multiple of that vector, which signifies that the direction of the vector remains unchanged while its magnitude can vary.
Importance of Eigenvalues in MATLAB
In the realm of numerical computing and data analysis, eigenvalues play an indispensable role. They are pivotal in fields such as physics, engineering, and statistics. In MATLAB, the ability to compute and analyze eigenvalues enables users to decipher complex systems, perform stability analysis, and conduct principal component analysis (PCA) for data reduction and classification tasks.
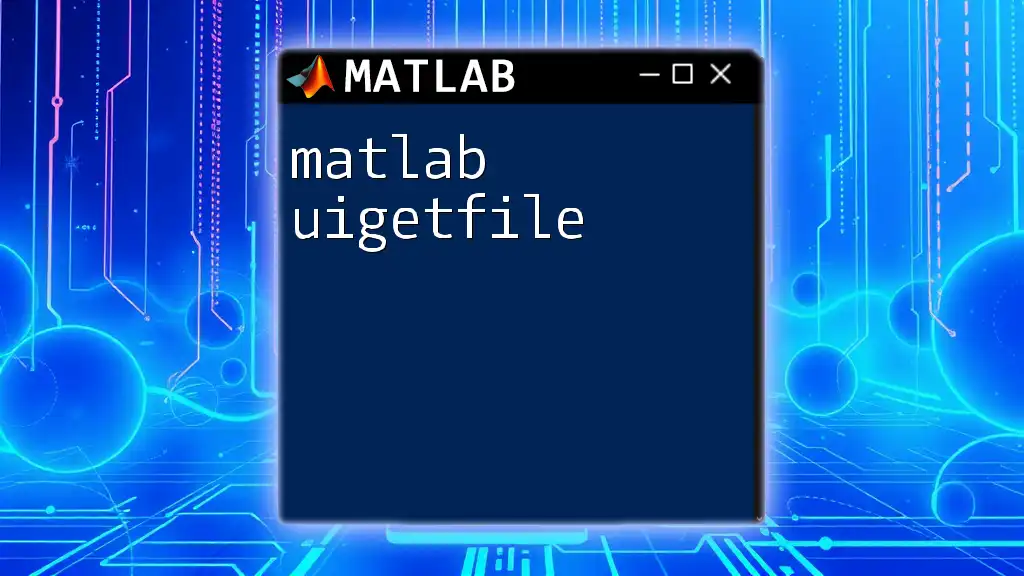
Understanding the Mathematical Background
Eigenvalue Equation
To obtain the eigenvalues of a matrix \( A \), one must solve the characteristic polynomial given by:
\[ \text{det}(A - \lambda I) = 0 \]
In this equation, \( \text{det} \) stands for the determinant of the matrix, \( I \) is the identity matrix of the same size as \( A \), and \( \lambda \) is the eigenvalue to solve for. Finding the roots of this polynomial yields the eigenvalues, which can be real or complex numbers depending on the properties of the matrix.
Eigenvectors and Their Relation to Eigenvalues
For each eigenvalue \( \lambda \), there exists a corresponding eigenvector \( v \) such that:
\[ Av = \lambda v \]
This relation showcases that eigenvectors point in directions that remain unchanged under the transformation represented by \( A \), while the eigenvalues indicate the factor by which these vectors are stretched or shrunk. Understanding this relation helps in visualizing the influence of the matrix on various vector spaces.
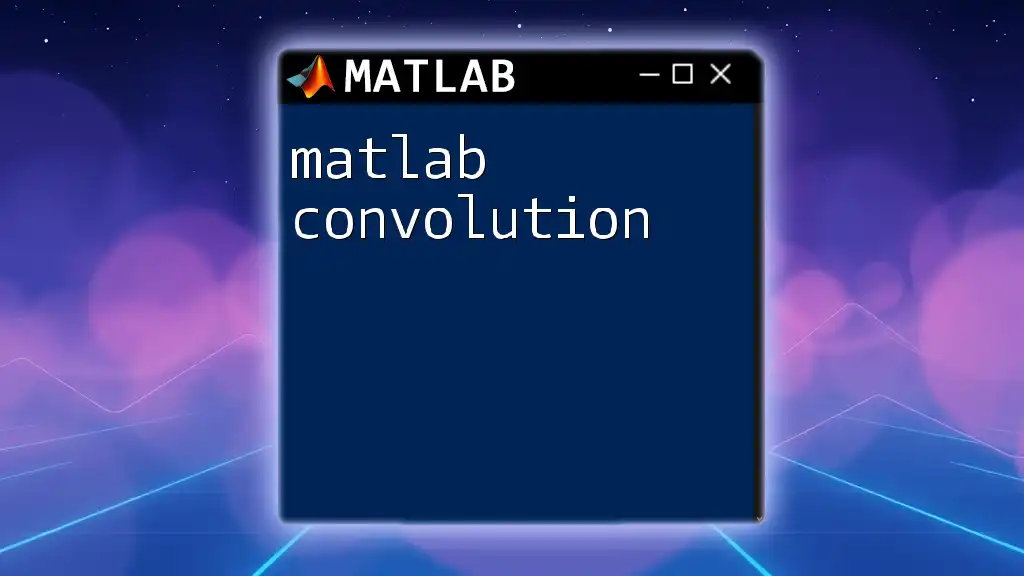
Getting Started with MATLAB for Eigenvalues
Installing MATLAB
To begin using MATLAB, you will need to install it on your computer. You can download a trial version from MathWorks' official website or purchase a license if you intend to use it long-term. Follow the installation instructions provided with the software, ensuring all components are properly set up for numerical computing.
Basic MATLAB Commands
Before diving into eigenvalues, it's essential to understand MATLAB's basic syntax. The fundamental concept is that matrices are constructed using square brackets. For example, a 2x2 matrix can be created as follows:
A = [a, b; c, d]; % Where a, b, c, and d are numerical values
This format will be utilized as we start computing eigenvalues.
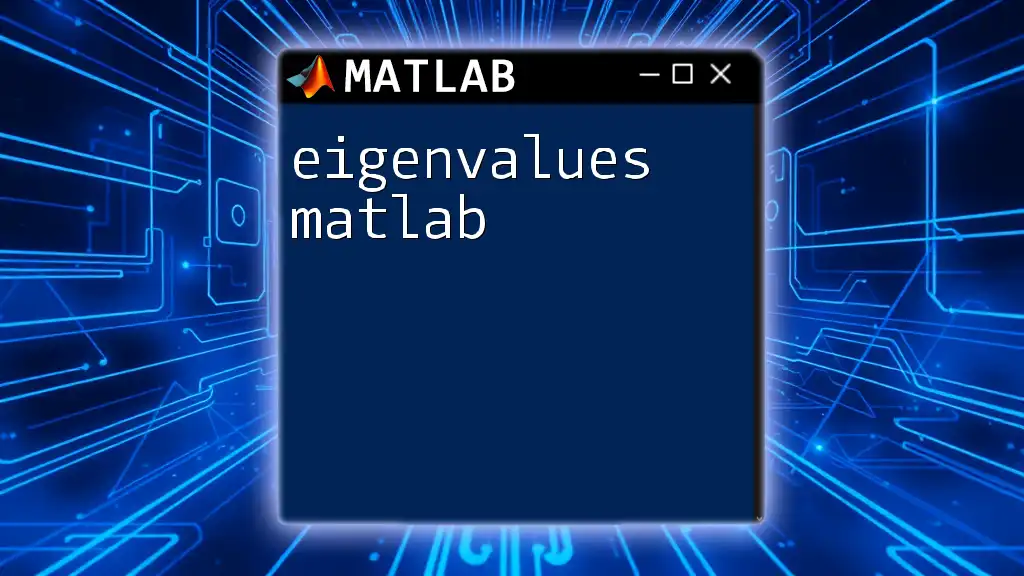
Computing Eigenvalues in MATLAB
The `eig` Function
MATLAB provides the `eig` function for computing the eigenvalues of matrices efficiently. The basic syntax is:
V = eig(A);
Here, \( A \) is the input matrix, and \( V \) will contain the eigenvalues. To compute both eigenvalues and eigenvectors simultaneously, the command is:
[V, D] = eig(A);
Where \( D \) will be a diagonal matrix consisting of the eigenvalues.
For example, let’s calculate the eigenvalues of a simple 2x2 matrix:
A = [4, 2; 1, 3];
eigenvalues = eig(A);
disp(['The eigenvalues are: ', num2str(eigenvalues')]);
In this case, running this code will provide the eigenvalues indicating how the transformation affects any eigenvector.
Eigenvalues of Square Matrices
It's crucial to remember that only square matrices (where the number of rows equals the number of columns) can have eigenvalues. When you attempt to calculate eigenvalues for non-square matrices, MATLAB will return an error.
Let's explore the computation of eigenvalues for a 3x3 matrix:
B = [1, 2, 3; 0, 4, 5; 0, 0, 6];
eigenvalues_B = eig(B);
disp(eigenvalues_B);
This example highlights the ease with which eigenvalues can be derived from different types of square matrices in MATLAB.
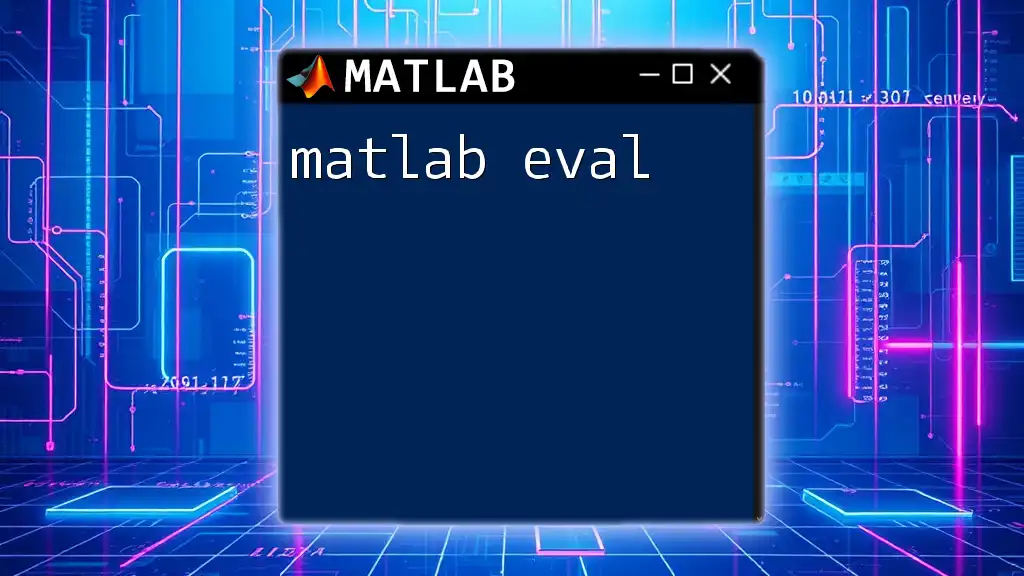
Visualizing Eigenvalues
Understanding Eigenvalue Plots
Visual representation plays a significant role in understanding the implications of eigenvalues. By plotting eigenvalues, particularly in the complex plane, one can gather insights about the stability and behavior of systems described by their corresponding matrices.
Example: Plotting Eigenvalues on the Complex Plane
To illustrate how eigenvalues can be plotted:
C = [0, -1; 1, 0];
eigenvalues_C = eig(C);
plot(real(eigenvalues_C), imag(eigenvalues_C), 'o');
title('Eigenvalues of Matrix C in Complex Plane');
xlabel('Real Part');
ylabel('Imaginary Part');
axis equal;
grid on;
In this code snippet, we visualize the eigenvalues obtained from a matrix \( C \) on the complex plane. Such plots allow for a more intuitive understanding of the locations and properties of eigenvalues, especially for complex numbers.
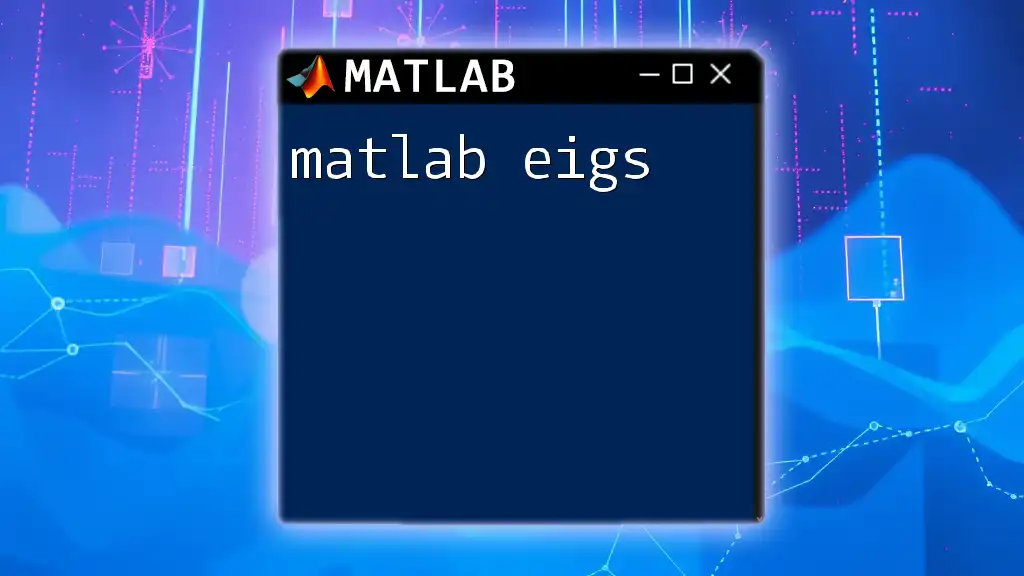
Advanced Applications of Eigenvalues
Eigenvalues in Principal Component Analysis (PCA)
Eigenvalues are crucial in PCA, a method commonly used for data reduction while preserving variance. PCA identifies the axes (principal components) that maximize the variance in the data, and this is done by calculating the covariance matrix and its eigenvalues and eigenvectors.
While performing PCA in MATLAB, you can compute eigenvalues from the covariance matrix derived from your dataset:
data = rand(100, 5); % Random dataset with 100 samples and 5 variables
cov_matrix = cov(data);
[eig_vectors, eig_values] = eig(cov_matrix);
Stability Analysis in Control Systems
In control systems, eigenvalues are a reflection of the system's stability. Specifically, the locations of eigenvalues relative to the imaginary axis determine whether a system will exhibit stable or unstable behavior.
For instance, by computing eigenvalues of a system's state matrix, you can ascertain system dynamics:
A = [0, 1; -1, -1]; % A sample state matrix for a control system
eigenvalues_A = eig(A);
disp(eigenvalues_A);
In this context, evaluation of eigenvalues informs engineers about stability, enabling adjustments to the system design as necessary.
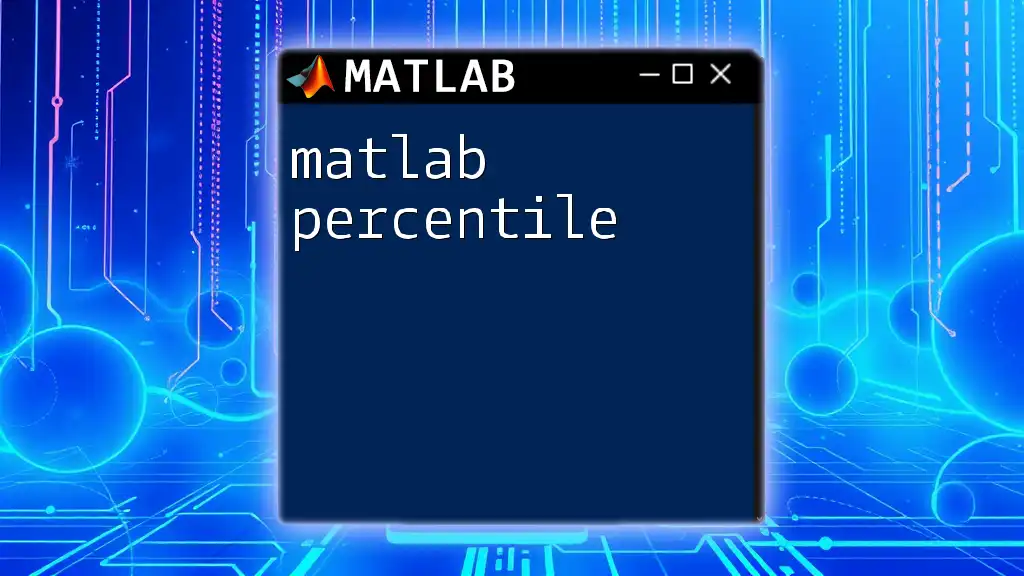
Common Errors and Troubleshooting
Possible Issues When Calculating Eigenvalues
In MATLAB, various issues may arise while computing eigenvalues, one notable mistake is attempting to compute eigenvalues of a non-square matrix, which will prompt an error.
To overcome this challenge, ensure your matrix is properly defined using MATLAB's size-checking functions like `size(A)`.
How to Interpret Errors
MATLAB provides clear error messages that can guide you through debugging. For example, an error indicating that the matrix must be square is a direct hint that your matrix dimensions need to be adjusted.
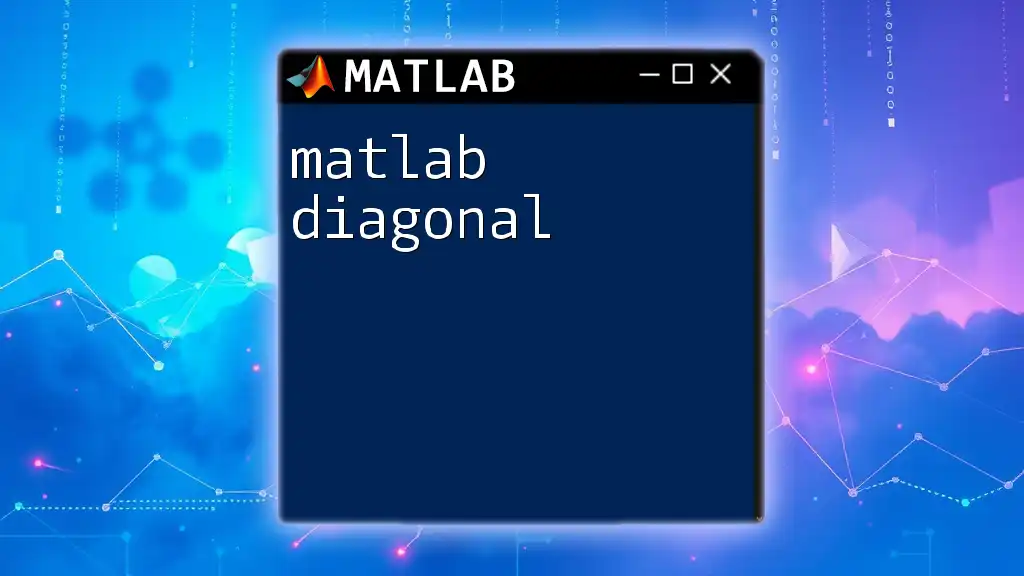
Conclusion
In summary, understanding MATLAB eigenvalues empowers users to tackle complex mathematical problems and applications across various disciplines. From computing eigenvalues and eigenvectors to employing these concepts in practical scenarios such as PCA and stability analysis, mastering these tools enhances analytical capabilities.
Further Learning Resources
To further expand your knowledge, consider exploring books, online courses, and official MATLAB documentation focused on linear algebra applications, eigenvalue problems, and data analysis techniques within MATLAB.
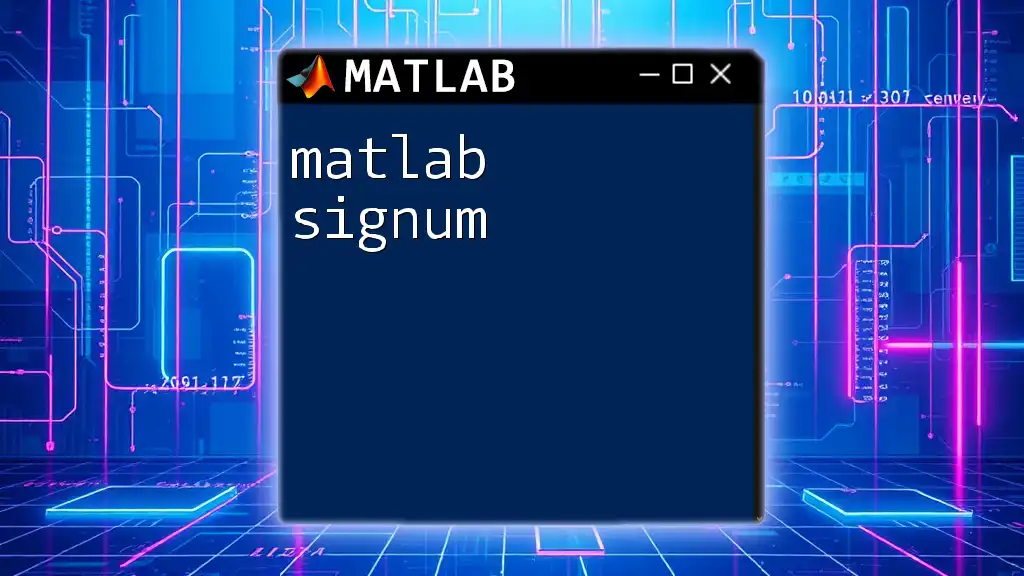
Call to Action
Now that you are familiar with the concept of MATLAB eigenvalues, it is time to put this knowledge into practice. Try out the provided examples and experiment with different matrices to experience firsthand the power of eigenvalues in MATLAB. Join our community to access additional resources and ongoing support as you sharpen your MATLAB skills!