To check if a file exists in MATLAB, you can use the `isfile` function, which returns true if the specified file exists and false otherwise.
fileExists = isfile('example.txt');
Understanding File Existence in MATLAB
What Does It Mean for a File to Exist?
In the realm of programming, particularly in MATLAB, understanding whether a file exists is pivotal for effective file handling. When we refer to a file's existence, we mean that the file is available in a specified location within the file system, and it can be accessed by operations such as reading or writing. Before executing any operation on a file, it's crucial to confirm its presence to avoid runtime errors.
Common Scenarios for File Checking
Checking if a file exists often arises in various situations. Some common scenarios where this is important include:
- Loading Data Files: When working with datasets, it’s crucial to ensure that data files (e.g., `.mat`, `.csv`) are available.
- Reading Configuration Files: Configuration files often dictate the behavior of scripts or applications; their absence might lead to unexpected behavior.
- Managing Output Files: During lengthy computations, ensuring that output files are written to appropriately is vital for data integrity.
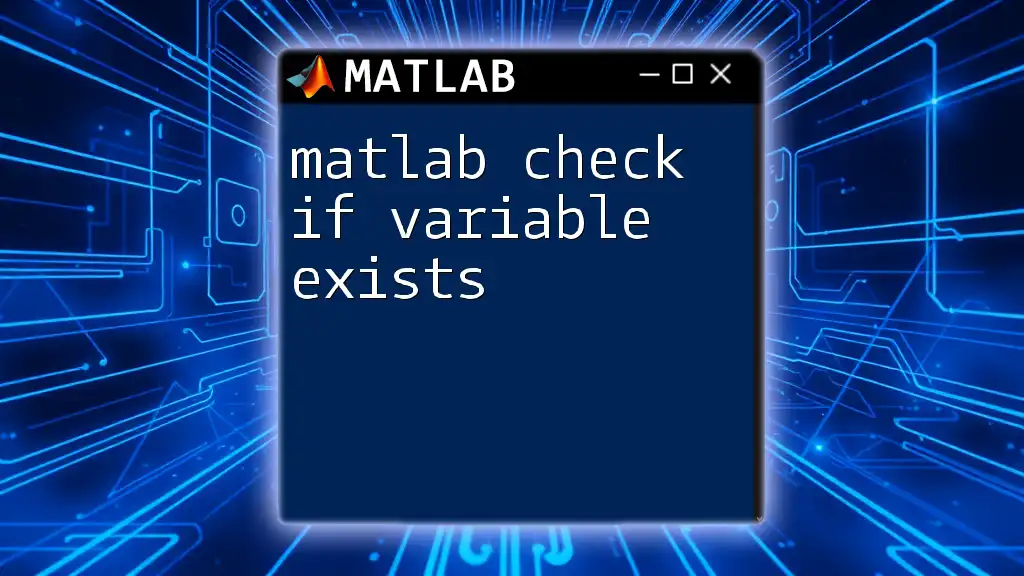
MATLAB Functions to Check File Existence
`exist` Function
The `exist` function is one of the fundamental ways to check for a file's existence in MATLAB. Its syntax is:
exist('filename', 'type')
This function checks for the existence of a file or variable in different contexts. The type can be set to check specifically for files or directories. Here are the possible types:
- `'file'`: Checks for a file.
- `'dir'`: Checks for a directory.
- `'builtin'`: Checks for built-in MATLAB functions.
A practical example using the `exist` function would look like this:
if exist('data.csv', 'file') == 2
disp('File exists.');
else
disp('File does not exist.');
end
In this example, if `data.csv` is present in the current directory, MATLAB displays "File exists." If not, it will return "File does not exist."
`isfile` Function
For users with MATLAB version R2016a and later, the `isfile` function offers a more straightforward alternative for checking file existence. The syntax is:
isfile('filename')
This function returns a logical value and is particularly handy for its simplicity. Here’s how it can be implemented:
if isfile('settings.txt')
disp('Settings file found.');
else
disp('Settings file not found.');
end
In this case, if `settings.txt` is located in the specified directory, the display will confirm its presence.
`isfolder` Function
In addition to checking files, it’s often necessary to verify if a directory exists. The `isfolder` function is explicitly designed for this purpose, with the syntax:
isfolder('foldername')
For instance, the following code checks whether a directory named `data` exists:
if isfolder('data')
disp('Data folder exists.');
else
disp('Data folder does not exist.');
end
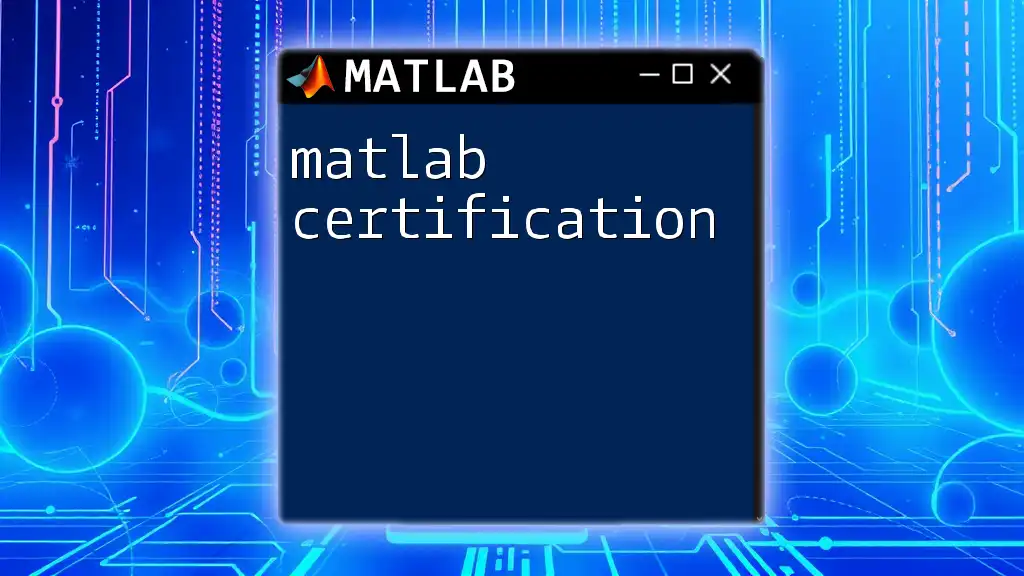
Combining Checks: File and Directory
Why Combine Checks?
In many scenarios, a file may exist only if a specified directory is present. This connection between files and directories necessitates combined checks to avoid errors that could arise from assuming a file's presence without confirming its directory's existence.
Example of Combined Checks
Here is a practical implementation that combines checks for both the directory and the file:
folderName = 'data';
fileName = 'data.csv';
if isfolder(folderName) && isfile(fullfile(folderName, fileName))
disp('File exists within the directory.');
else
disp('File or directory does not exist.');
end
In this example, the script checks first for the existence of the `data` folder and then checks for the `data.csv` file within that folder. This method enhances code robustness by ensuring that necessary preconditions are met before proceeding.
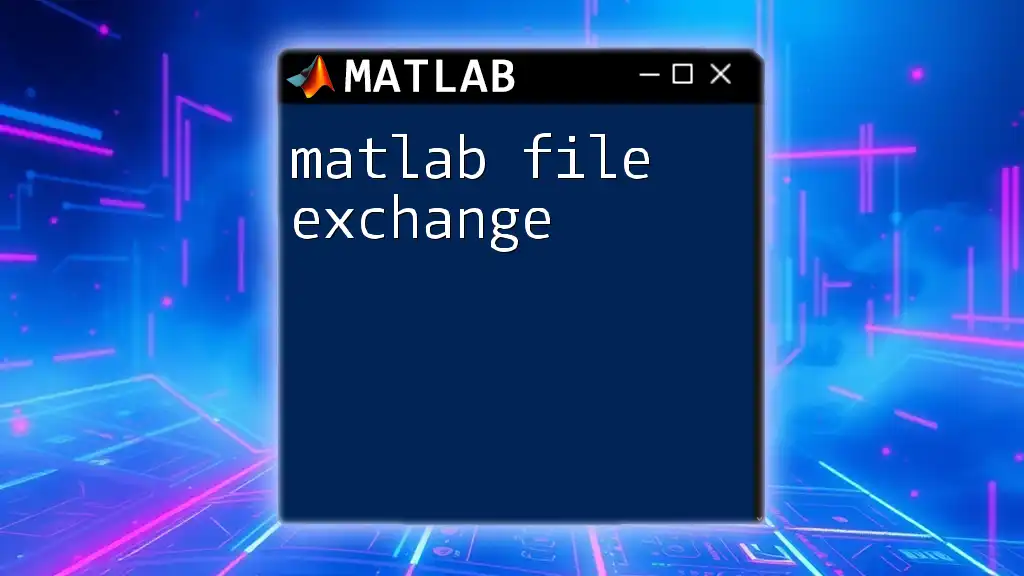
Handling Non-Existent Files Gracefully
Best Practices for Error Handling
Effective error handling is crucial for writing stable MATLAB scripts. Verifying file existence before performing actions on files can eliminate abrupt script terminations and provide a smoother user experience.
Using `try-catch` Blocks
The `try-catch` block offers a way to handle exceptions gracefully. If a file does not exist, this construct allows the program to catch the error and execute an alternative line of code. Here’s how you can implement it:
try
data = load('data.mat');
catch
disp('data.mat does not exist. Please check the file path.');
end
In this example, if the file `data.mat` isn't found, MATLAB will catch the error and display a user-friendly message instead of crashing.
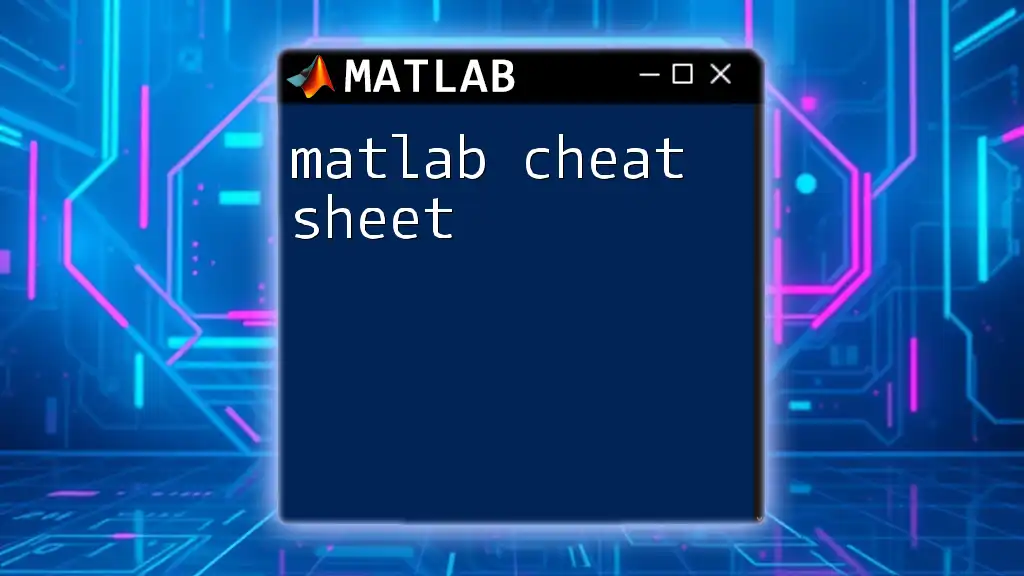
Conclusion
When conducting file operations in MATLAB, a robust approach that includes checking if a file exists is crucial for preventing runtime errors and ensuring smooth execution. By utilizing functions like `exist`, `isfile`, and `isfolder`, MATLAB users can handle files with confidence. Integrating checks into your scripts, coupled with proper error handling strategies, not only prepares you for unforeseen file issues but also enhances the overall reliability of your coding practices.
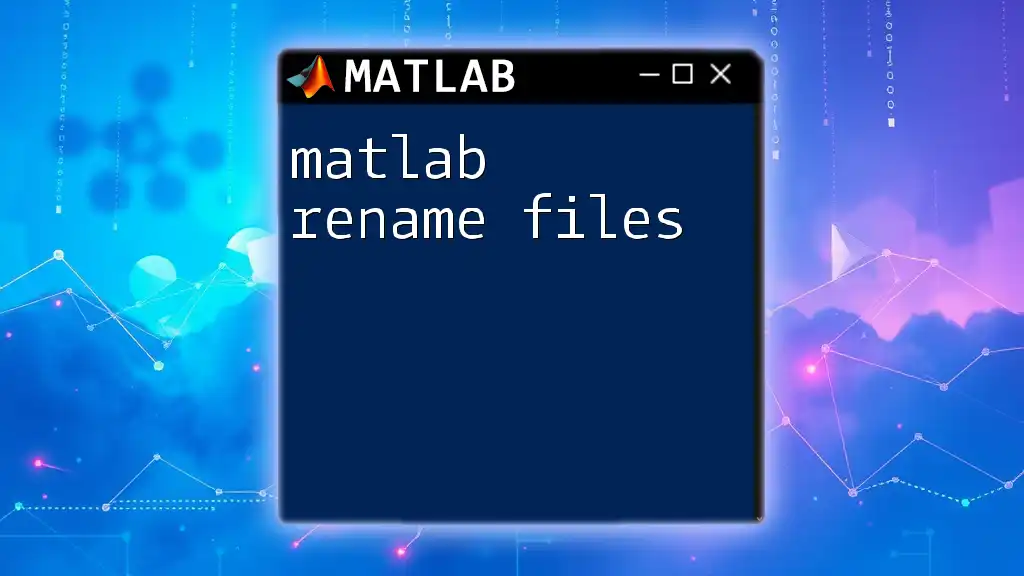
Additional Resources
Suggested Reading
For more in-depth understanding, refer to the official MATLAB documentation on file I/O, where you will find comprehensive discussions and examples related to file handling.
Further Learning
Keep an eye out for upcoming workshops focused on file handling and other essential MATLAB skills, designed to help you deepen your knowledge and coding proficiency.