The MATLAB interpreter allows users to incorporate LaTeX formatting in visuals and text annotations to enhance the presentation of mathematical expressions and symbols.
Here’s an example of how to use LaTeX in MATLAB to display a formatted equation:
% Example of LaTeX rendering in a text annotation
xlabel('Centimeters (cm)', 'Interpreter', 'latex');
ylabel('$\sin(x)$', 'Interpreter', 'latex');
title('Graph of $\sin(x)$', 'Interpreter', 'latex');
Understanding the MATLAB Interpreter
What is an Interpreter?
An interpreter is a vital component in the realm of programming languages. Unlike compilers, which translate the entire code into machine language before execution, interpreters process the code line-by-line. MATLAB serves as an interpreter, allowing users to execute commands interactively within its environment. This feature is particularly useful for rapid prototyping and experimentation, as it enables users to see results immediately as they write their code.
Key Features of the MATLAB Interpreter
Interactivity with Commands
The MATLAB command window is where all the magic happens. It allows you to perform calculations, test functions, and generate plots in real-time. You can execute simple commands to perform arithmetic operations, such as:
>> 2 + 2
ans = 4
This immediate feedback is invaluable for learners who are just starting with MATLAB, as it helps build confidence and understanding.
Variable Management
MATLAB makes it easy to manage variables dynamically. You can create a variable, assign it a value, and manipulate it in any way you desire. For instance, you can store results in variables as follows:
>> x = 5;
>> y = 10;
>> z = x + y;
In this example, the output will allow you to reference the value of `z` later, demonstrating MATLAB's strength in handling data.
Function Creation and Invocation
Creating custom functions in MATLAB streamlines your workflow and enhances code reusability. Functions can take inputs, perform calculations, and return outputs. Here’s a simple example of a function that adds two numbers:
function result = add(a, b)
result = a + b;
end
You can then call this function with specific values, allowing for modular and organized code development.
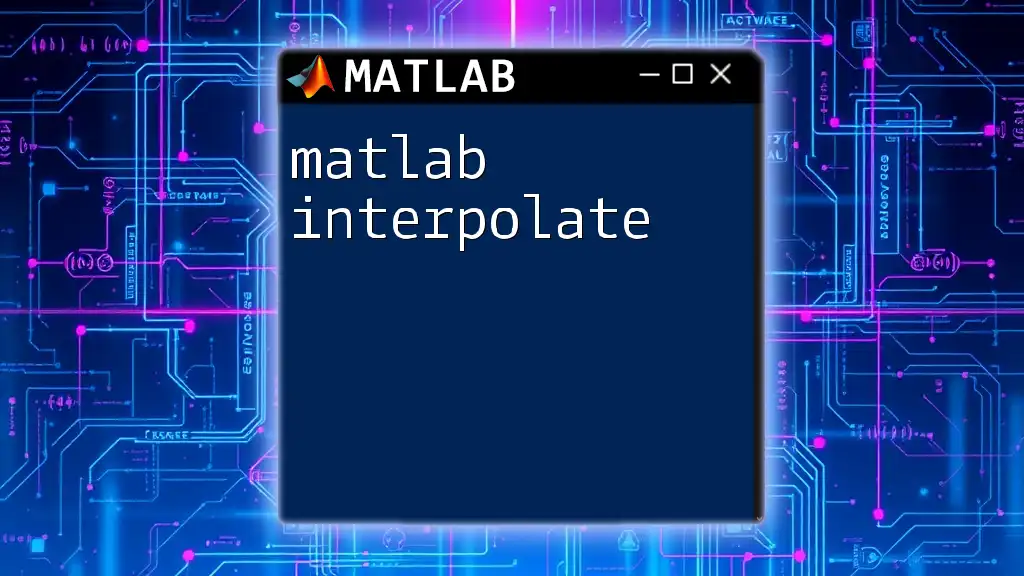
Integrating LaTeX with MATLAB
Why Integrate LaTeX with MATLAB?
The integration of LaTeX with MATLAB is a game-changer for presenting complex mathematical expressions and formal documentation. By leveraging LaTeX, you elevate the quality of your outputs, whether in figures, plots, or text displayed in the MATLAB environment. The professional touch that LaTeX provides is especially beneficial when preparing reports or academic papers that require precision in mathematical notations.
Using LaTeX to Format Text in MATLAB
Displaying LaTeX in Figures
MATLAB allows the incorporation of LaTeX commands directly into plot titles, axis labels, and annotations. This capability enhances the visual appeal of your graphs. For example, you can annotate a plot with a LaTeX-rendered title:
plot(x, y);
title('Plot of $y = x^2$', 'Interpreter', 'latex');
This code snippet demonstrates how to include a mathematical expression within the title, yielding a clear and professionally formatted presentation.
Creating Text Objects with LaTeX
You can also craft text for GUI elements and command windows with LaTeX syntax. For instance, you can label axes using LaTeX to ensure that the units are correctly formatted and visually coherent:
xlabel('Distance (m)', 'Interpreter', 'latex');
ylabel('Force (N)', 'Interpreter', 'latex');
This ensures that your text is not only descriptive but also adheres to typographical standards expected in scientific documentation.
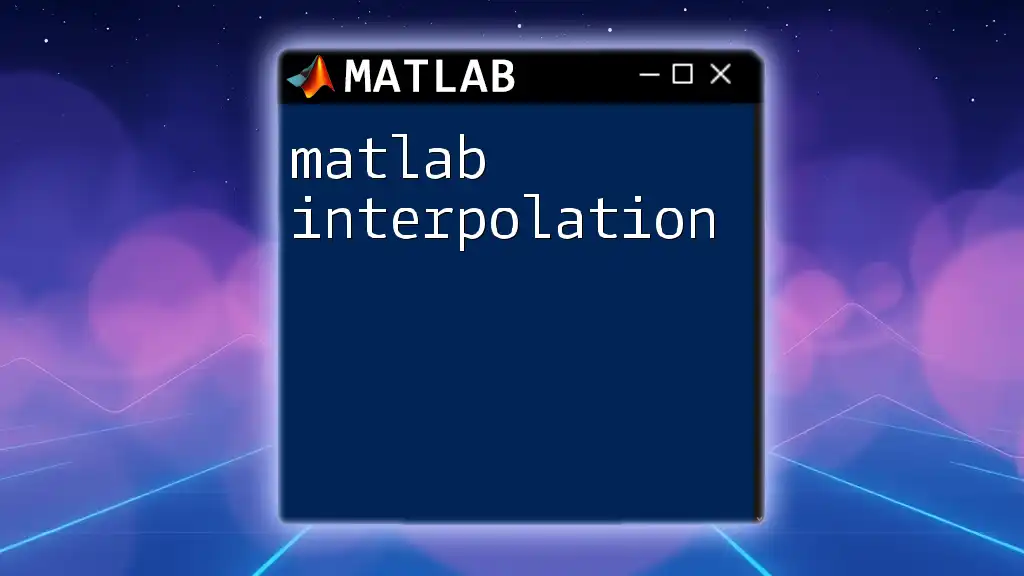
Advanced LaTeX Features in MATLAB
Using Mathematical Symbols
MATLAB supports numerous LaTeX symbols that allow for the visualization of mathematical concepts effortlessly. From Greek letters to integral signs, these symbols can enhance your output's clarity. Here's how you can incorporate summations in your plots:
text(0,0,'$\sum_{i=1}^{n} x_i$', 'Interpreter', 'latex');
This code will display the summation symbol in the designated position on your plot, mirroring the standard mathematical notation.
Creating Complex Equations
For more sophisticated presentations, LaTeX allows you to align and display multi-line equations. You can craft an equation like this:
eq = '$\begin{aligned}
y &= mx + b \\
&= \frac{n}{(1+x^2)}
\end{aligned}$';
text(0.5, 0.5, eq, 'Interpreter', 'latex');
This snippet not only illustrates an equation across multiple lines but also aligns it neatly, enhancing readability—a crucial factor when dealing with complex formulas.
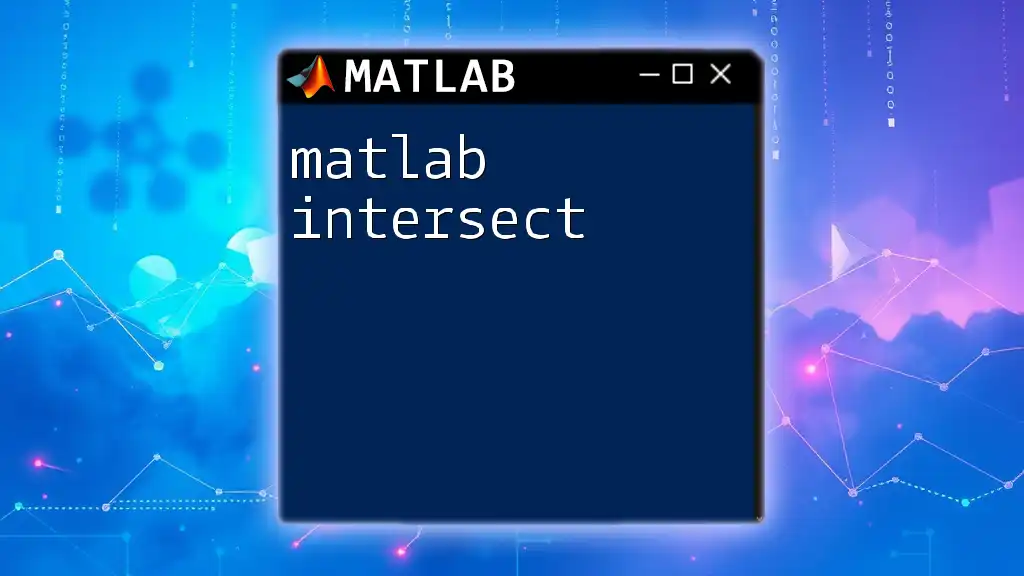
Tips for Effective Use of LaTeX in MATLAB
Common Pitfalls to Avoid
While integrating LaTeX in MATLAB is powerful, there are common pitfalls to be aware of. For example, forgetting to set the 'Interpreter' property to 'latex' will result in LaTeX code being displayed as plain text rather than rendered beautifully. Always double-check your configurations to avoid such errors.
Best Practices for Code Readability
Maintaining readability is critical in coding, especially when mixing MATLAB and LaTeX. Use clear indentation, consistent formatting, and comments to guide anyone who may read your code. This practice not only helps you keep track of your logic but also aids collaborators or future reviewers.
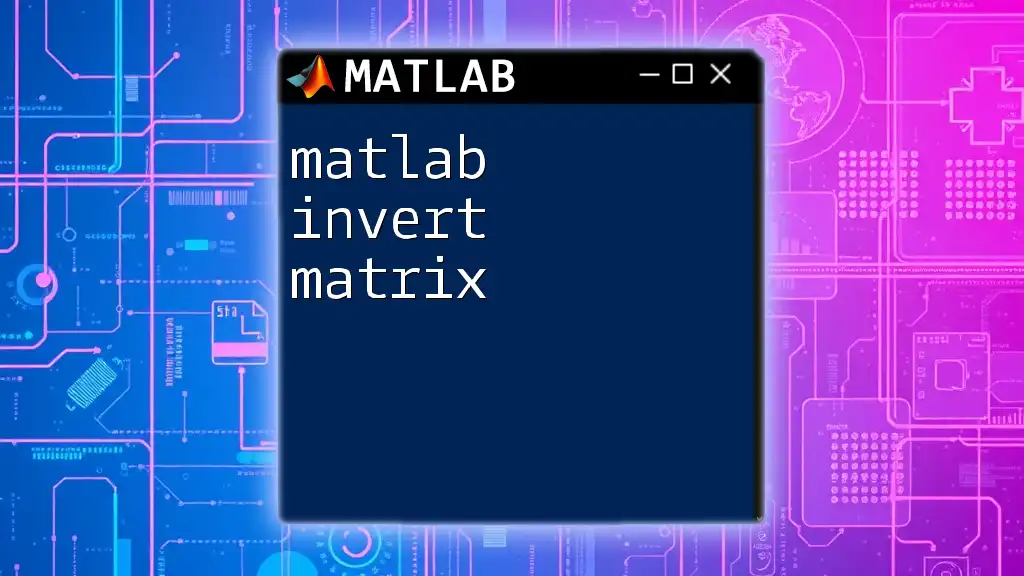
Conclusion
The integration of matlab interpreter latex not only enriches the functionality of MATLAB but also significantly enhances the visual quality of outputs. By mastering the usage of LaTeX within MATLAB, you are equipped to present complex mathematical concepts clearly and professionally. Practice these techniques to achieve fluency, and don’t hesitate to experiment with various LaTeX features to maximize the impact of your presentations.
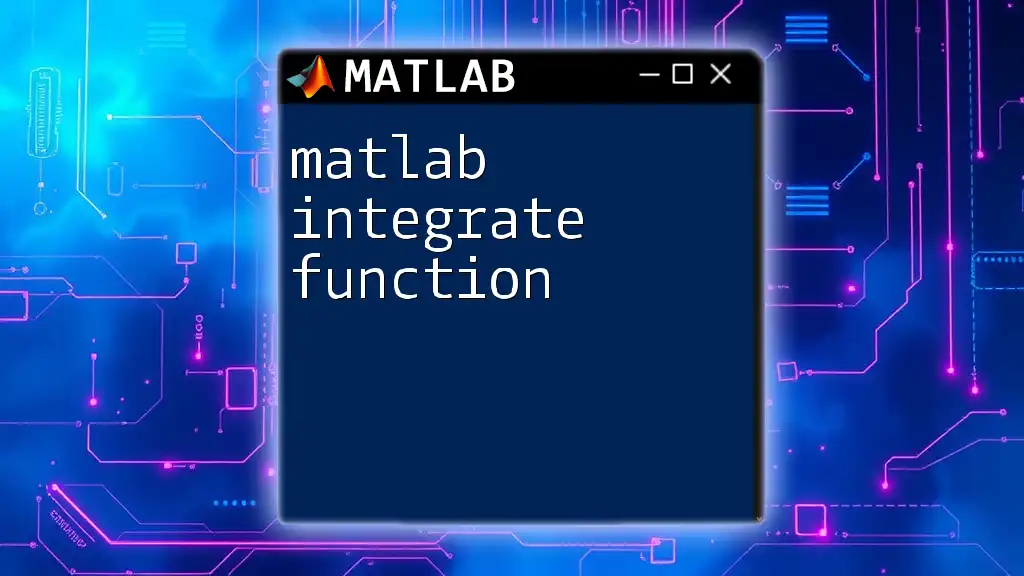
Additional Resources
For extended learning, the official MATLAB documentation is an invaluable resource, offering in-depth guidance on both MATLAB functionalities and LaTeX formatting. Engaging with community forums and online classes can also provide support and additional insights into the best practices in using MATLAB and LaTeX together effectively.