The MATLAB `intersect` function returns the common elements of two arrays, with options to specify sorting and to return indices in the original arrays.
A = [1, 2, 3, 4, 5];
B = [4, 5, 6, 7, 8];
C = intersect(A, B); % C will be [4, 5]
Understanding the `intersect` Function
What is the `intersect` Function?
The `intersect` command in MATLAB is one of the essential functions used to identify common elements between two arrays. It is particularly useful in data analysis and mathematical computations, making it easier to manage and understand overlapping data sets. Whether you're dealing with numerical data, strings, or even multi-dimensional arrays, the `intersect` function provides a robust solution to find shared elements seamlessly.
Syntax of the `intersect` Function
The basic syntax of the `intersect` function is as follows:
[C, ia, ib] = intersect(A, B)
- A, B: These are the input arrays from which you want to identify common elements.
- C: This output variable returns the common elements found in both arrays.
- ia, ib: These outputs provide the indices of the common elements in arrays A and B respectively, making it easier to trace back to the original data if needed.
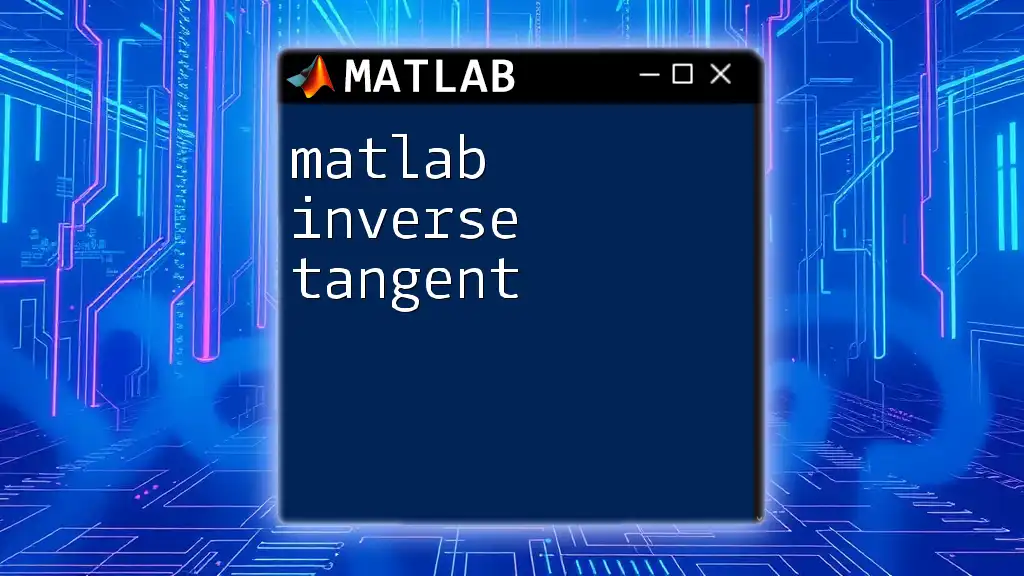
Practical Usage of the `intersect` Function
Finding Common Elements in Two Vectors
A common scenario is identifying shared data points between two datasets. Consider the following example:
A = [1, 2, 3, 4, 5];
B = [3, 4, 5, 6, 7];
[C, ia, ib] = intersect(A, B);
Here, the variable C will output the common elements, which in this case are `[3, 4, 5]`. The ia array provides the indices of these common elements in A, and ib gives the indices in B. This is especially useful when you need to track where the common elements originated from, facilitating further analysis.
Working with Different Data Types
Numerical Arrays
`intersect` can handle various data types, including numerical arrays. For example:
A = [10, 20, 30, 40];
B = [20, 30, 40, 50];
[C, ia, ib] = intersect(A, B);
The output C would yield `[20, 30, 40]`, demonstrating how `intersect` can identify overlapping values effectively.
Character Arrays
The function is equally versatile with character arrays. This is beneficial when working with datasets that include textual information. For example:
A = ['apple', 'banana', 'cherry'];
B = ['banana', 'dragonfruit', 'apple'];
[C, ia, ib] = intersect(A, B);
In this case, the output C will be an array of characters containing `['apple', 'banana']`, which represents the common entries found in both character arrays.
Using `intersect` with Multi-Dimensional Arrays
`intersect` isn't limited to one-dimensional arrays; it also works with multi-dimensional datasets. You can utilize the function on matrices as follows:
M1 = [1, 2; 3, 4; 5, 6];
M2 = [4, 5; 6, 7; 1, 2];
[C, ia, ib] = intersect(M1, M2, 'rows');
In this example, `intersect` checks for entire rows that match across the two matrices. The results may include specific rows that are duplicated but will simplify data identification in larger datasets.
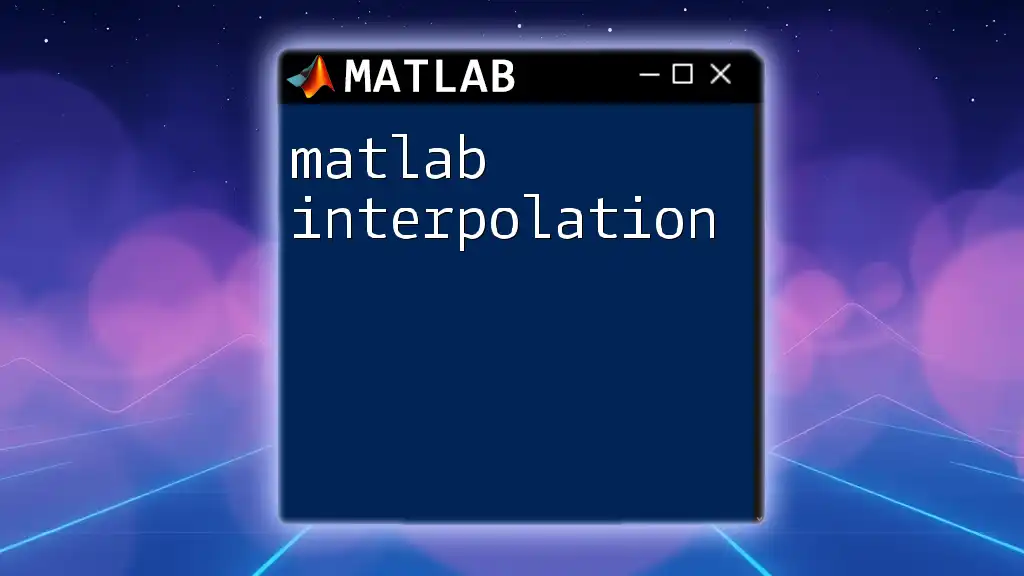
Advanced Features of the `intersect` Function
Options for Sorting the Output
The `intersect` command allows for flexibility regarding the order in which results are presented. By using the `'stable'` option, the function can maintain the original order of elements. For example:
[C, ia, ib] = intersect(A, B, 'stable');
This will ensure that the order of the output C persists as it was in the original array, which may be crucial for preserving the context of your data.
Handling Duplicate Entries
When analyzing datasets, you may encounter duplicates. The `intersect` function effectively manages these cases, only returning unique elements in its output. For instance:
A = [1, 1, 2, 3, 3, 4];
B = [1, 2, 2, 3, 4];
[C, ia, ib] = intersect(A, B);
Despite entries appearing multiple times, C will yield `[1, 2, 3, 4]`, reflecting distinct common values.
Customizing the Match
The `intersect` function also offers customization options. For instance, when working with character arrays, you can specify the comparison for case sensitivity. This is illustrated in the following example:
A = ['MATLAB', 'Python'];
B = ['matlab', 'JAVASCRIPT'];
[C, ia, ib] = intersect(A, B, 'rows');
In this case, C will yield an empty output since case-sensitive comparisons differentiate 'MATLAB' from 'matlab'.
Performance Considerations
When working with large datasets, performance can become a concern. To optimize, consider pre-sorting your arrays before applying the `intersect` function. This reduces the processing time since it utilizes efficient searching algorithms.
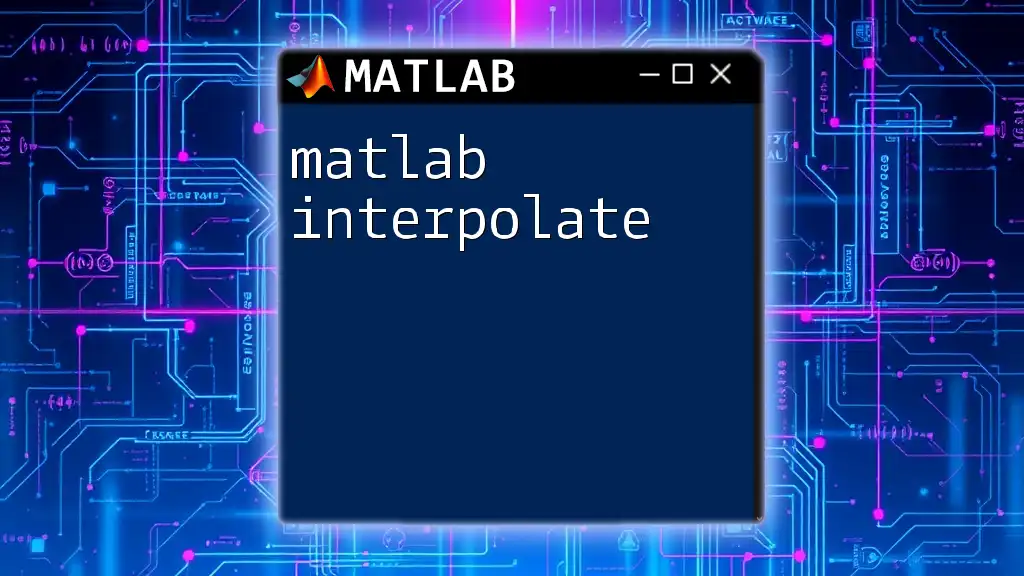
Common Pitfalls to Avoid
Index Misalignment
While using `intersect`, be mindful of index misalignment, as erroneous interpretations of indices can lead to data inaccuracies. Always double-check that the indices returned correspond to the correct elements in their respective arrays.
Data Type Mismatches
A prevalent error occurs when there's a mismatch between data types. Ensure both arrays being compared are of similar data types to avoid unexpected results, such as empty outputs or errors.
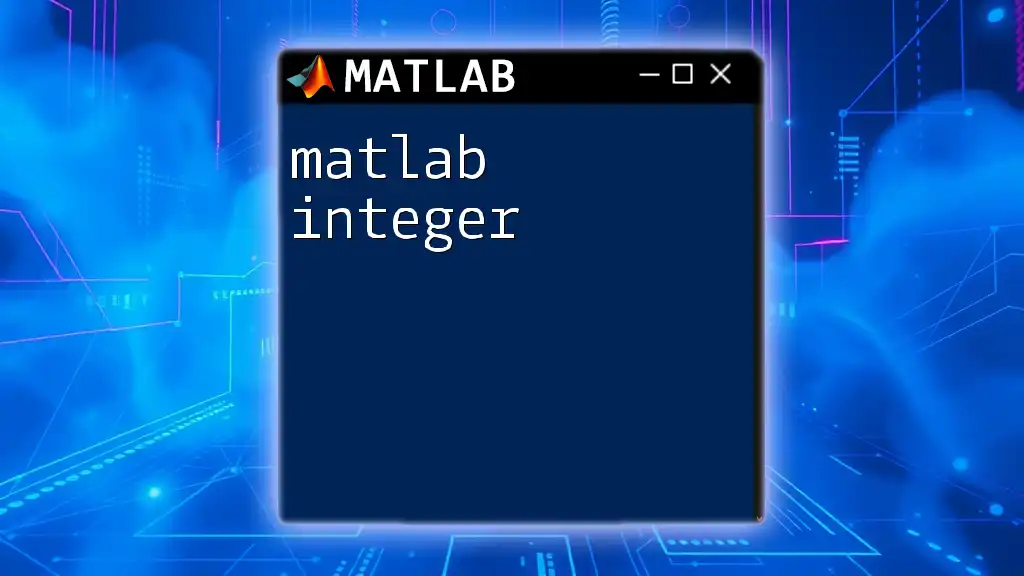
Real-World Applications
The `intersect` function plays a significant role in various real-world applications, particularly in data analysis within research and business settings. It helps identify overlaps between customer datasets, shared research data among scientists, or duplicates in inventory lists—making it a highly versatile tool for any MATLAB user.
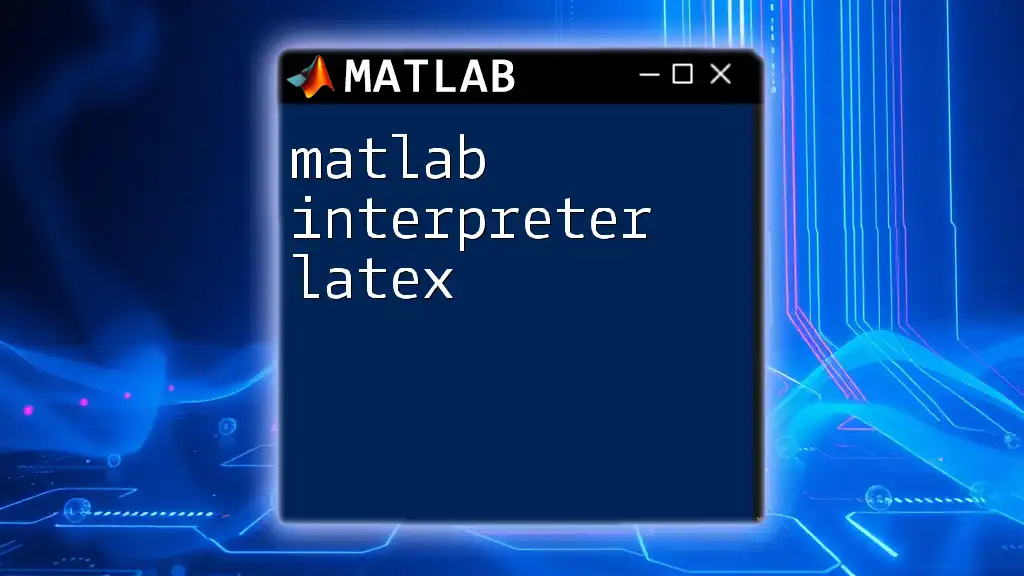
Conclusion
Mastering the `intersect` function is essential for anyone looking to elevate their data analysis skills within MATLAB. By understanding its syntax, applications, and advanced features, you can effectively manage and analyze data. As you grow more comfortable with this powerful command, practice with various datasets to enhance your proficiency and confidence in using MATLAB for your analytical needs.