Matlab indexing allows you to access and manipulate specific elements or subsets of arrays using numeric or logical indices.
Here's a code snippet demonstrating basic indexing in Matlab:
% Create a 1D array
A = [10, 20, 30, 40, 50];
% Access the third element
element = A(3); % element will be 30
% Access the first three elements
subset = A(1:3); % subset will be [10, 20, 30]
Understanding MATLAB Data Structures
Common Data Structures
Arrays are the most fundamental data type in MATLAB. They can be one-dimensional (vectors) or multi-dimensional (matrices). Understanding how to manipulate arrays is crucial since most MATLAB operations revolve around these data structures.
Cell arrays are a unique feature in MATLAB, allowing you to store arrays of varying sizes and types in a single variable. Each element of a cell array can be different, enabling greater flexibility.
Tables and structures provide a way to organize data efficiently. Tables are particularly useful when working with datasets that have rows and columns, while structures allow the grouping of related data under one name.
Role of Indexing in Data Structures
Indexing is the mechanism through which you access and manipulate the elements of these data structures. Understanding how to use indexing effectively is key to performing data analysis and processing in MATLAB.
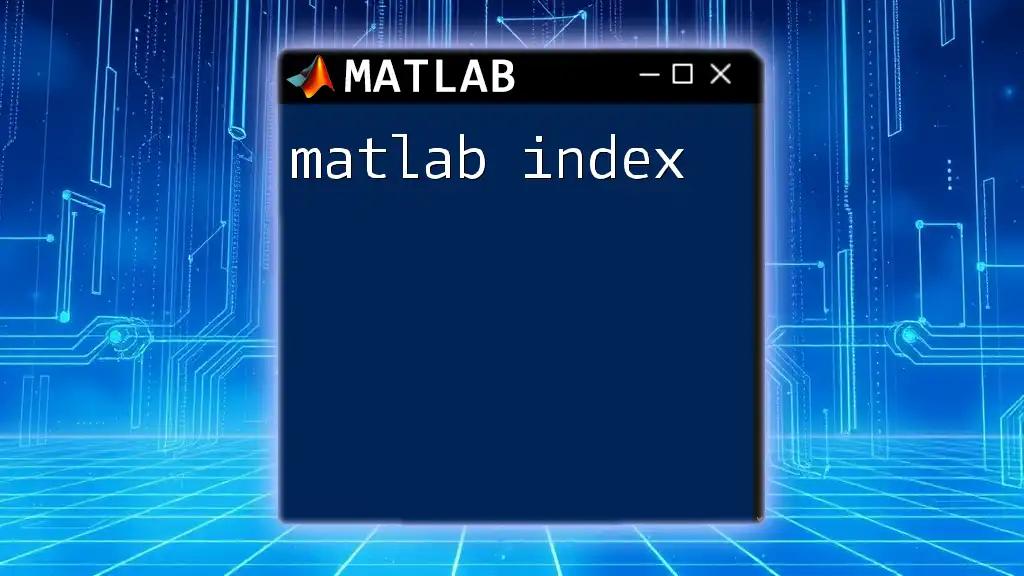
Basic Indexing in MATLAB
Linear Indexing
Linear indexing allows access to elements of an array as if it were a one-dimensional list. MATLAB stores array data in a column-major order, meaning that it fills columns before rows.
For example, consider the following code snippet:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
linear_index = A(5); % Accessing the 5th element
In this example, `A(5)` retrieves the element `5`, which is the fifth element in linear indexing. This concept is especially useful when working with larger multidimensional arrays, making data access efficient and concise.
Subscript Indexing
Subscript indexing relies on row and column subscripts to access specific elements. It provides intuitive access to two-dimensional arrays.
For instance, in the following example:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
elem = A(2,3); % Accessing the element at row 2, column 3
Here, `A(2,3)` accesses the element at the second row and third column, and the retrieved value is `6`. This method is straightforward and makes it easy to navigate through the data.
Logical Indexing
Logical indexing allows you to access elements of an array based on certain logical conditions, providing an efficient way to filter data.
Consider the following example:
A = [10, 20, 30, 40];
index = A > 20; % Logical indexing
result = A(index); % Accessing elements greater than 20
In this example, `index` becomes an array of logical values that indicate whether each element in `A` meets the condition (`> 20`). The `result` will yield `[30, 40]`, showcasing the power of logical indexing in quickly retrieving desired data.
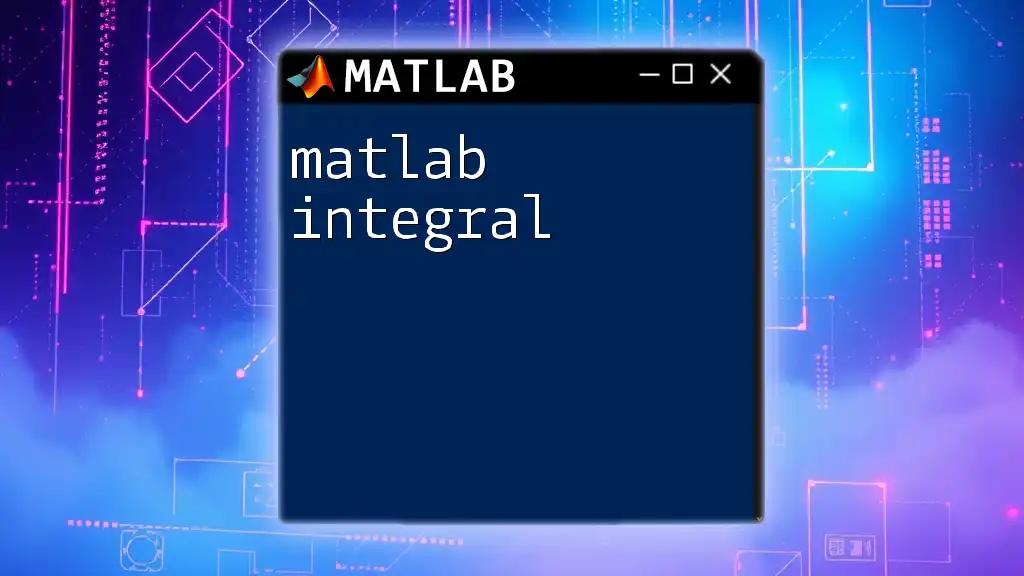
Advanced Indexing Techniques
Indexing with Colon Operator
The colon operator, represented by `:`, is a powerful tool for slicing arrays. It allows access to a continuous range of elements.
Below is a code snippet demonstrating this:
A = 1:10; % Creating an array from 1 to 10
sub_array = A(3:7); % Accessing elements from index 3 to 7
In this case, `sub_array` will yield `[3, 4, 5, 6, 7]`. This feature is exceptionally useful when needing to work with subarrays.
Using `end` Keyword
The `end` keyword is a handy feature for referencing the last element of an array or dimension. It streamlines code writing and improves clarity.
For example:
A = [10, 20, 30, 40];
lastElem = A(end); % Accessing the last element
In this example, `lastElem` retrieves the value `40`. Its use simplifies accessing elements without needing to count indices.
Multi-Dimensional Indexing
MATLAB allows indexing in multi-dimensional arrays, enabling targeted access to elements beyond just two dimensions.
Consider the following code:
A = rand(4, 3, 2); % Creating a 4x3x2 random array
elem = A(2, 3, :); % Accessing a specific slice
Here, `A(2, 3, :)` retrieves all values in the 2nd row, 3rd column across all "slices" in the third dimension, enhancing flexibility in data handling.
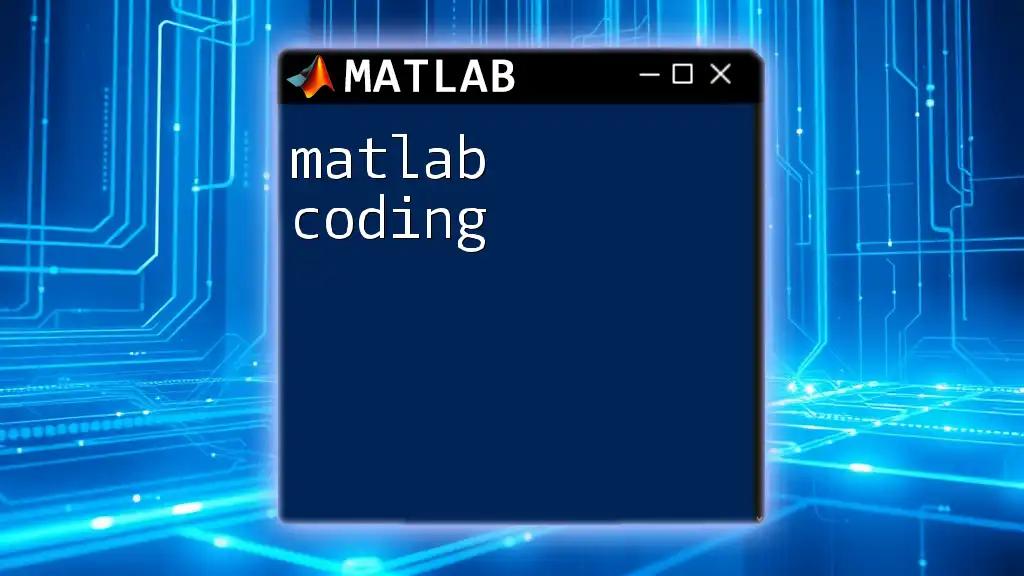
Modifying Data with Indexing
Replacing Elements
Indexing not only allows access but also facilitates modification. Elements can be easily replaced by assigning new values to specific indices.
For instance:
A = [1, 2, 3; 4, 5, 6];
A(1,2) = 10; % Changing the element in the first row, second column to 10
In this case, the original matrix `A` will have its second element of the first row changed to `10`, resulting in `A` becoming `[1, 10, 3; 4, 5, 6]`.
Adding and Deleting Elements
Indexing can also assist in dynamically resizing arrays by adding or deleting elements.
For example:
A = [1, 2; 3, 4];
A(2,3) = 5; % Adding a new element
A(1,:) = []; % Deleting the first row
Here, the code adds a new element `5` to the second row and third column and then deletes the first row entirely, showing how indexing can manage array sizes effectively.
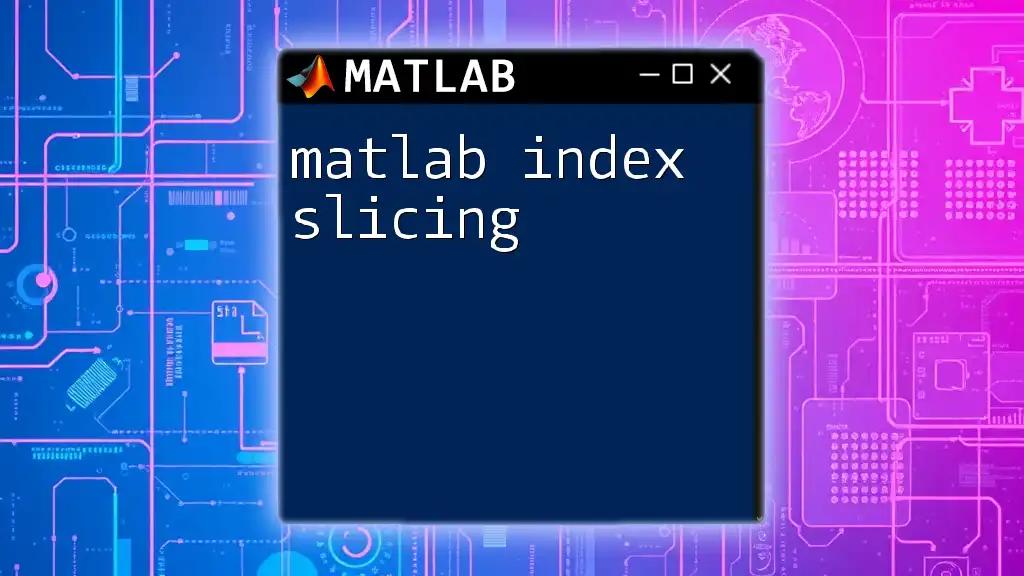
Practical Applications of Indexing
Data Extraction from Large Datasets
Efficient indexing is critical when dealing with large datasets, allowing you to extract relevant information quickly.
Consider the following example using a table:
T = readtable('data.csv');
filteredData = T(T.Var1 > 50, :); % Extracting rows where Var1 > 50
This code filters the table `T`, keeping only those rows where the variable `Var1` is greater than `50`. Such operations illustrate the effectiveness of indexing for data manipulation tasks.
Indexing in Functions and Scripts
In MATLAB, functions can utilize indexing to create reusable and versatile code snippets. Incorporating indexing allows you to write functions that can handle varying input sizes and types efficiently.
By mastering indexing, you can streamline your workflows and enhance the ease of data manipulation.
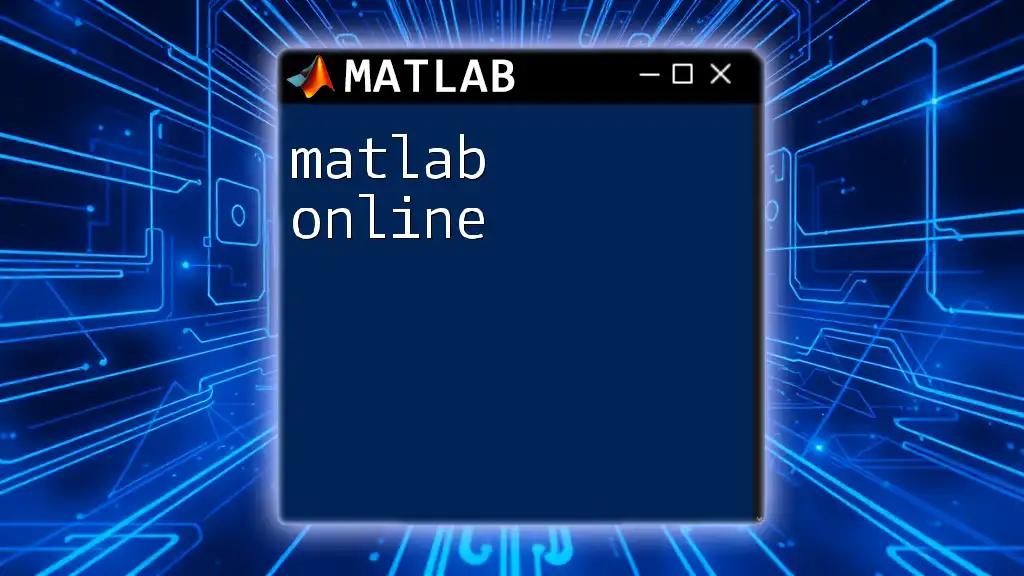
Conclusion
MATLAB indexing is a foundational concept that enhances your ability to handle and manipulate data structures effectively. By understanding various indexing techniques—linear, subscript, logical, and advanced methods—you can streamline data access, modification, and extraction.
As you integrate these techniques into your MATLAB toolkit, you'll find that indexing not only simplifies your coding experience but also significantly improves performance, especially when working with larger datasets.