MATLAB's `interp1` function allows for one-dimensional interpolation of data points, enabling you to estimate values at intermediate points based on observed data.
% Example of MATLAB interpolation
x = [1 2 3 4 5]; % Given data points
y = [2 3 5 7 11]; % Corresponding values
xi = 1.5; % Point to interpolate
yi = interp1(x, y, xi); % Interpolating to find the value at x = 1.5
disp(yi); % Display the interpolated value
What is Interpolation?
Interpolation is a technique used to estimate unknown values that fall between known data points. It plays a crucial role in various applications such as data analysis, image processing, engineering, and scientific research. By using interpolation, one can generate a smoother dataset from discrete points without losing significant detail.
Overview of MATLAB's Interpolation Functions
MATLAB provides several built-in functions to facilitate interpolation, making it easier for users to perform data analysis efficiently. The primary function for one-dimensional interpolation is `interp1`, while multi-dimensional interpolation is handled by `interp2`, `interp3`, and so on. These functions allow users to choose different interpolation methods that best fit their dataset.
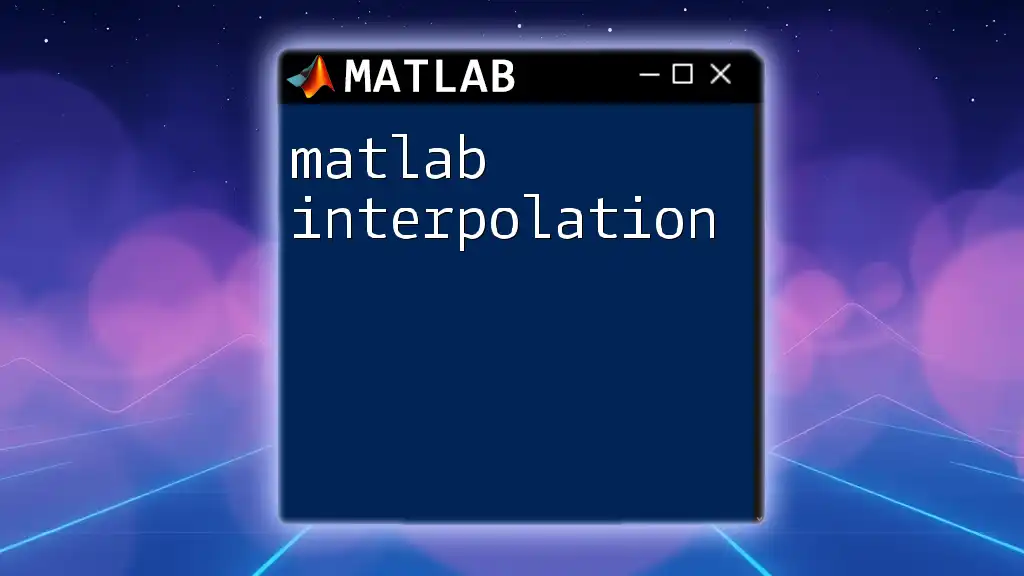
Types of Interpolation Methods in MATLAB
Linear Interpolation
Linear interpolation is the simplest method, which connects two adjacent data points with a straight line. This method is effective for datasets where the values change uniformly.
To perform linear interpolation in MATLAB, use the following command:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
xi = 2.5;
yi = interp1(x, y, xi, 'linear');
disp(yi);
In this example, MATLAB calculates the value of `yi` at `xi` using linear interpolation, providing a quick estimation based on the surrounding points.
Spline Interpolation
Spline interpolation involves using piecewise polynomials to accurately fit the dataset. This method offers a smoother curve compared to linear interpolation, which can be beneficial in applications requiring higher precision.
To implement spline interpolation, use the following command:
xi = linspace(1, 5, 100);
yi = interp1(x, y, xi, 'spline');
plot(x, y, 'o', xi, yi, '-');
legend('Data Points', 'Spline Interpolation');
This code snippet demonstrates how to visualize the original data points alongside the spline-fit curve, showcasing the advantage of smoother interpolation features.
Nearest Neighbor Interpolation
Nearest neighbor interpolation is a straightforward method that assigns the value of the nearest data point to the new point. It is particularly useful in discrete data scenarios where maintaining original values is critical.
To compute nearest neighbor interpolation, use:
yi = interp1(x, y, xi, 'nearest');
disp(yi);
This simple command provides a quick and efficient way to estimate new values without introducing any intermediate values.
Pchip Interpolation
The Piecewise Cubic Hermite Interpolating Polynomial (Pchip) method is another useful interpolation technique. It provides a smoother curve while maintaining the monotonicity of the dataset, making it suitable for datasets with various trends.
You can perform Pchip interpolation with the following MATLAB command:
yi = interp1(x, y, xi, 'pchip');
This method gives a good balance between flexibility and maintaining the original properties of the data, producing fewer oscillations than spline interpolation.
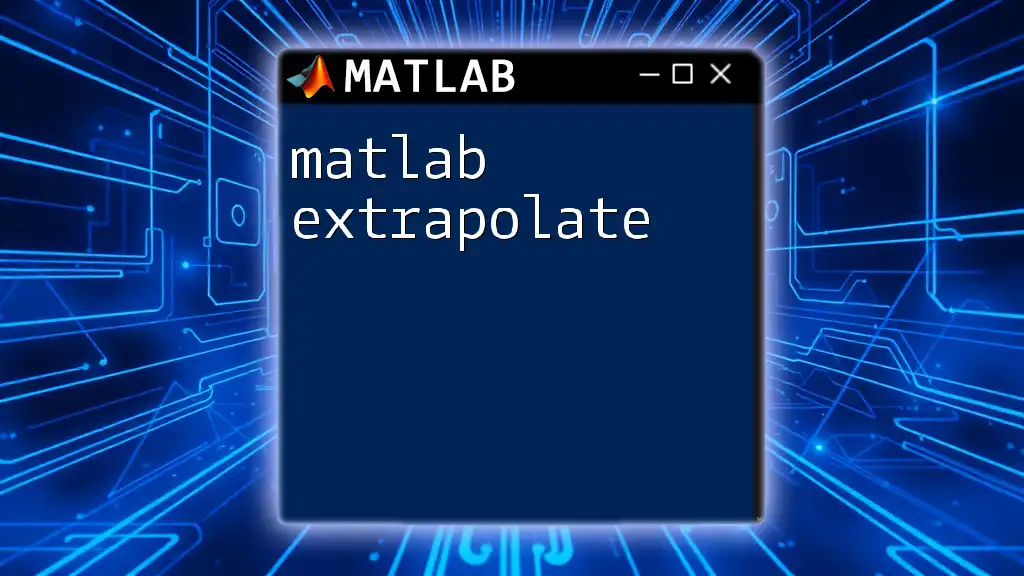
Practical Examples of MATLAB Interpolation
Example 1: Interpolating Missing Data Points
Interpolation is often employed to fill gaps in datasets. Let’s consider a dataset with missing values represented as NaNs:
x = [1, 2, 3, NaN, 5];
y = [2, 3, NaN, 7, 11];
x( isnan(x) ) = [];
y( isnan(y) ) = [];
xi = linspace(1, 5, 10);
yi = interp1(x, y, xi, 'linear');
plot(x, y, 'o', xi, yi, '-');
Here, we remove NaNs for interpolation and generate a continuous estimation of the missing values. Visualizing this can help assess the accuracy and fit of the interpolation method chosen.
Example 2: Data Visualization with Interpolation
Effective visualization of data highlights the importance of interpolation. The following example shows how to compare original data with interpolated values:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
xi = linspace(1, 5, 100);
yi_linear = interp1(x, y, xi, 'linear');
yi_spline = interp1(x, y, xi, 'spline');
plot(x, y, 'o', xi, yi_linear, '-', xi, yi_spline, '--');
legend('Original Data', 'Linear Interpolation', 'Spline Interpolation');
title('Interpolation Comparison');
By visualizing the results, it's clear how different interpolation methods impact the representation of the data, allowing users to choose the best method for their specific cases.
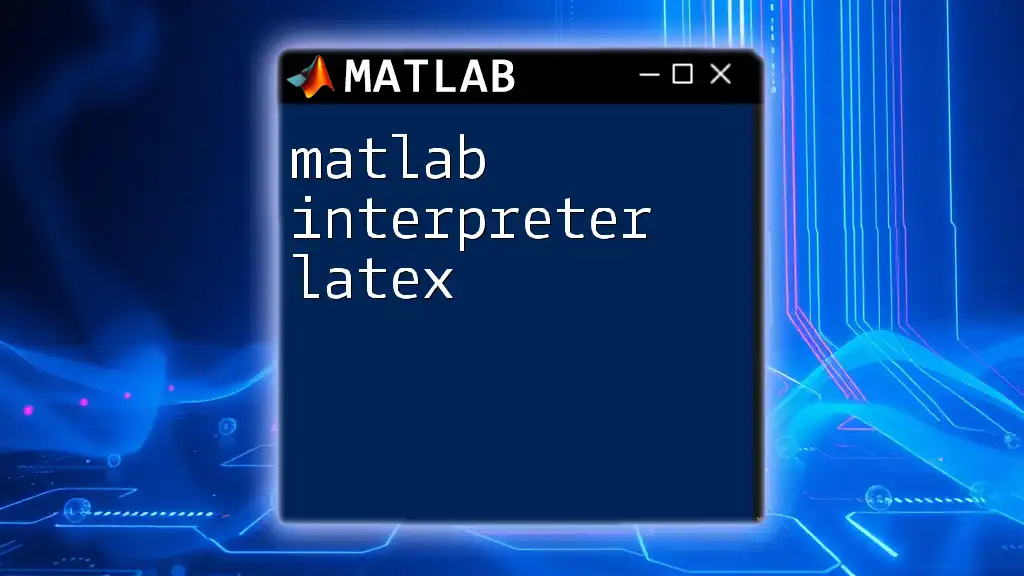
Advanced Interpolation Techniques
Multi-dimensional Interpolation
For datasets that span multiple dimensions, MATLAB provides functions like `interp2` for 2D data. This allows users to compute interpolations in two dimensions by specifying a grid of known data points.
Custom Interpolation Functions
Sometimes, built-in functions may not fully meet the unique requirements of the dataset. Creating a custom interpolation function can be a valuable approach. By defining your interpolation algorithms, you can tailor the method specifically to your needs, ensuring the accuracy and performance of the interpolation tasks.
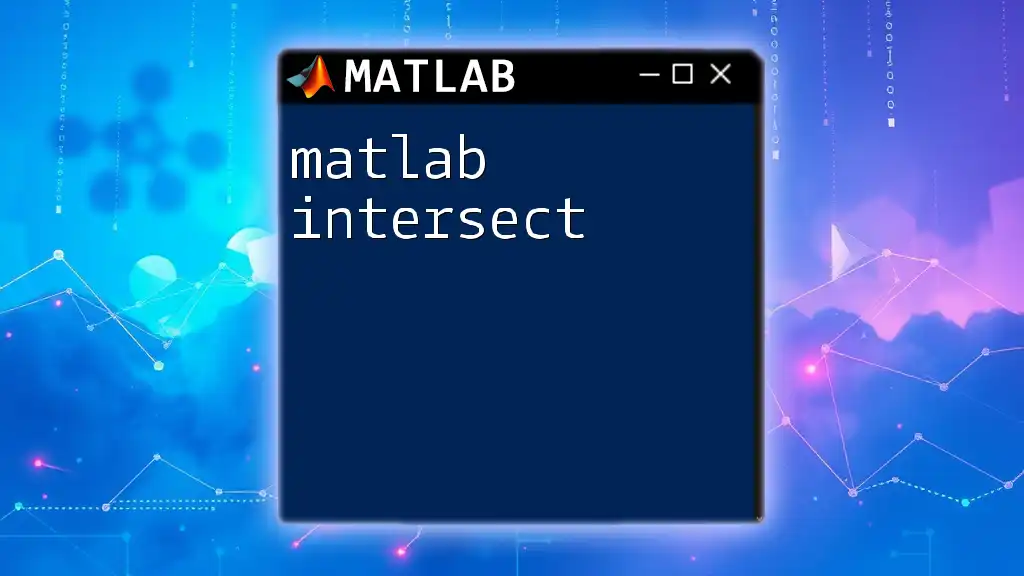
Error Handling and Best Practices
Common Errors and Their Solutions
When using MATLAB's interpolation functions, users might encounter several common errors, such as attempting to interpolate outside the range of provided data. To avoid this, always ensure new points lie within the boundaries of the original dataset.
Choosing the Right Interpolation Method
Choosing the most suitable interpolation method depends on several factors, including:
- Data density: Sparse datasets may benefit from simpler methods like linear or nearest neighbor.
- Smoothness: If a smooth curve is essential, spline or Pchip might be the better options.
- Computational cost: Consider the efficiency required for large datasets, as some methods may be computationally intensive.
Performance Optimization
Optimizing interpolation speed often involves minimizing the size of datasets pre-interpolation or leveraging MATLAB’s vectorization capabilities. This can significantly improve the performance of time-critical applications.
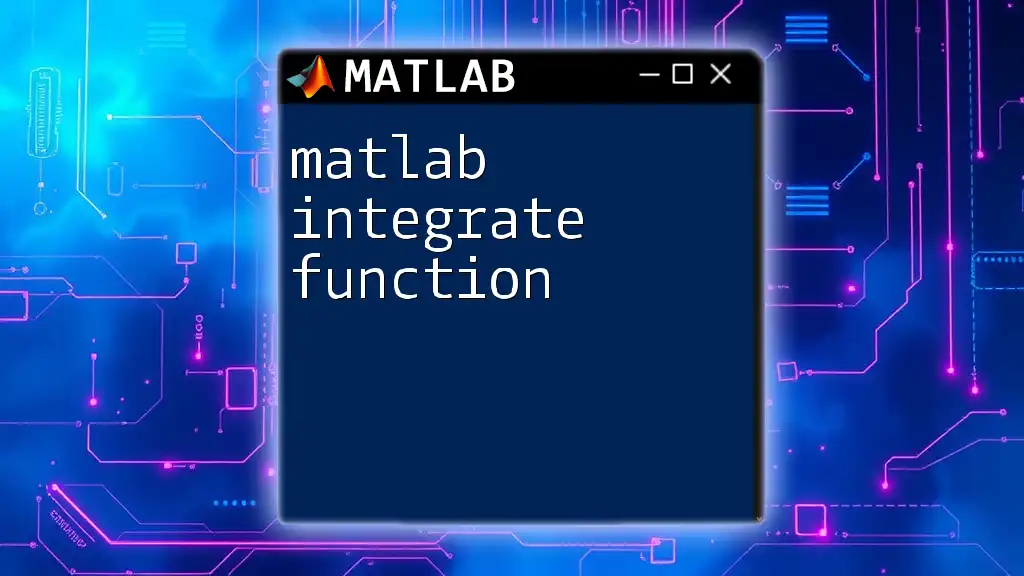
Conclusion
In summary, MATLAB interpolation is a highly versatile technique applicable across various domains. Whether filling in missing data, creating smooth curves, or handling multi-dimensional datasets, mastering these methods equips users with powerful tools for data analysis. Understanding the different techniques available, alongside their respective advantages and limitations, is crucial for achieving optimal results.
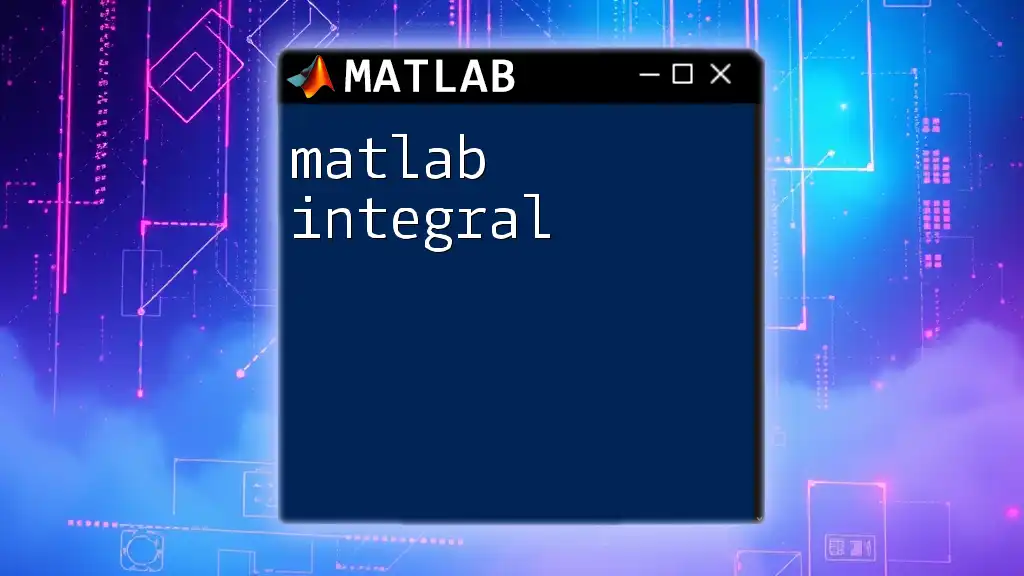
Further Reading and Resources
To deepen your understanding of MATLAB interpolation, consider exploring the official MATLAB documentation or supplementing your learning with books focusing on mathematical computing and data science practices. These resources provide valuable insights and advanced techniques to enhance your skills.
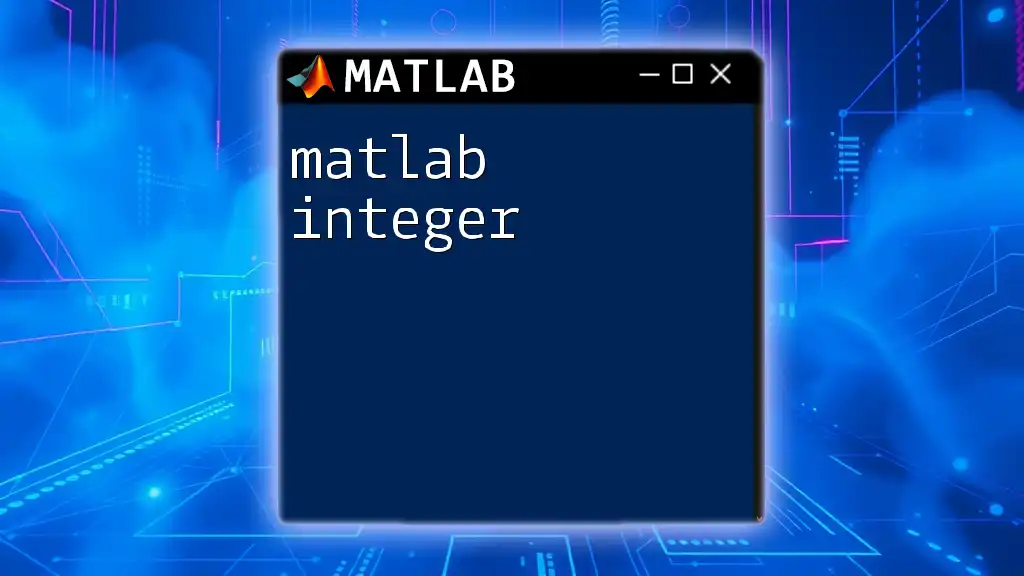
FAQs about MATLAB Interpolation
What if my data points are not sorted?
You must sort your data points before applying interpolation. MATLAB requires that the x-values be arranged in ascending order for proper calculations.
How do I handle large datasets in MATLAB interpolation?
For large datasets, consider using MATLAB's built-in functions that utilize efficient algorithms, or reduce the dataset size by sampling or applying downsampling techniques where appropriate.
Can I interpolate with more than two dimensions?
Yes! MATLAB provides functions like `interp3` for three-dimensional data, allowing you to perform interpolation in higher dimensions effectively.