The `lsqcurvefit` function in MATLAB is used to perform nonlinear curve fitting by minimizing the sum of the squares of the differences between the observed data and the model predictions.
Here’s a simple example of using `lsqcurvefit`:
% Define the model function
model = @(b,x) b(1) * exp(b(2) * x);
% Sample data
xdata = [1, 2, 3, 4, 5];
ydata = [2.7, 7.4, 20.1, 54.6, 148.4];
% Initial guess for parameters
beta0 = [1; 0.5];
% Perform curve fitting
beta = lsqcurvefit(model, beta0, xdata, ydata);
This snippet defines a model, provides sample data, sets initial parameter guesses, and then uses `lsqcurvefit` to fit the model to the data.
Understanding the Syntax of `lsqcurvefit`
Overview of the Function
The `lsqcurvefit` function in MATLAB is employed to perform nonlinear least squares curve fitting. Its general syntax is as follows:
x = lsqcurvefit(fun,x0,xdata,ydata)
Here, `fun` is a handle to the model function that defines the relationship you presume or wish to investigate between your dependent and independent variables. The function works by utilizing an iterative optimization algorithm to minimize the sum of the squares of the differences between your predicted values and actual observations \( ydata \).
Detailed Breakdown of Parameters
fun: Model Function Handle
The `fun` parameter accepts a function handle representing your model. This can be a custom function you've defined or any anonymous function in MATLAB. The function should accept input parameters in the following manner: `fun(beta, xdata)`, where `beta` corresponds to the coefficients of your model you want to optimize.
For instance, consider the exponential decay model defined as:
modelFun = @(b,x) b(1) * exp(b(2) * x);
x0: Initial Guess for the Parameters
Providing an initial guess for the parameters is crucial for `lsqcurvefit`. The choice of `x0` can significantly affect the fitting results, especially in nonlinear models where multiple local minima may exist. A well-informed estimate based on prior knowledge of the data can enhance convergence.
xdata: Independent Variable Data
The `xdata` parameter should contain the independent variable or input data that corresponds to the model’s expected behavior. It's often a 1D array or column vector that reflects the variable that you manipulate or change in your experiments or dataset.
ydata: Dependent Variable Data
Similarly, `ydata` consists of the dependent variable or output data that you aim to model or fit. Both `xdata` and `ydata` should share the same dimensions, ensuring a valid correspondence between observed outputs and their respective inputs.
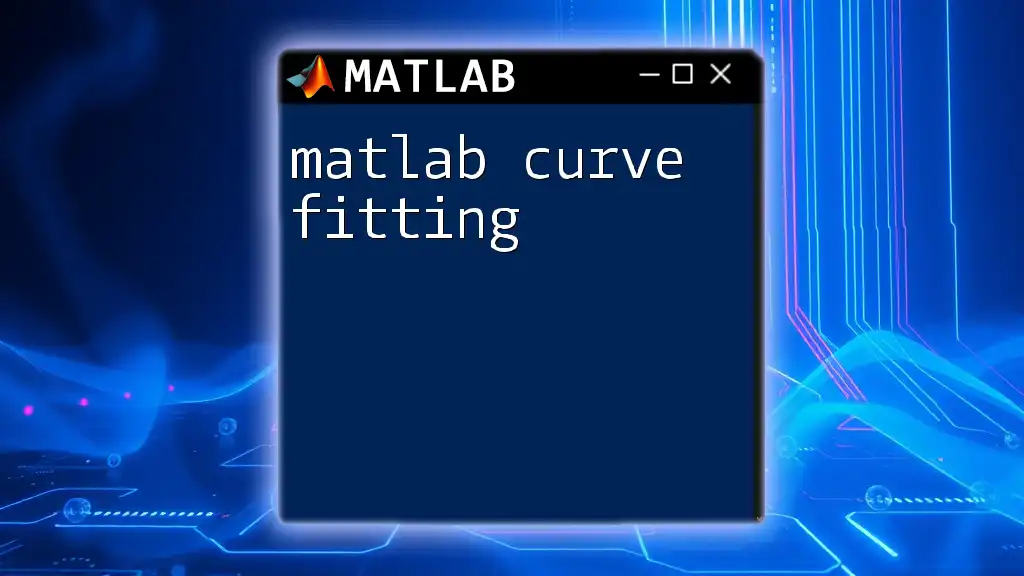
Setting Up Your Curve Fitting Problem
Choosing the Right Model
Selecting the appropriate model function is the cornerstone of effective curve fitting. Whether you're modeling physical processes, biological growth patterns, or any relationship in your data, the correct choice of model allows for better predictions. Common options include:
- Exponential models: For processes that grow or decay rapidly.
- Polynomial models: Useful for representing relationships with varying behaviors.
- Logistic models: Ideal for growth processes that have saturation effects.
Creating Function Handles
To implement a model, you must define a function handle. This handle serves as a link between your data and the mathematical structure you are trying to fit. Here’s how you might create a basic model function for fitting:
modelFun = @(b,x) b(1) * exp(b(2) * x);
This definition allows you to parameterize the model, where `b(1)` could represent the maximum value and `b(2)` the decay rate in the exponential model.
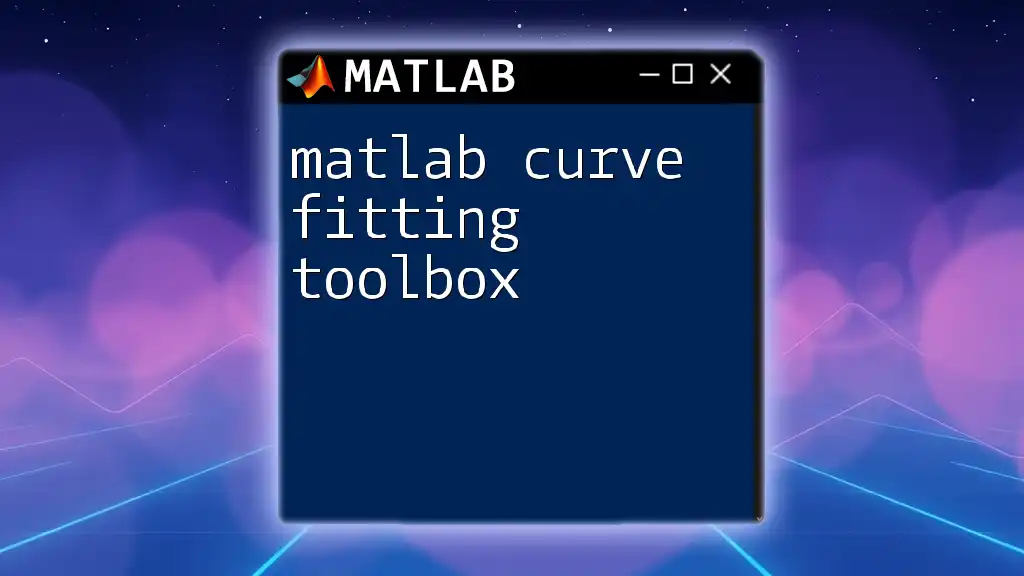
Executing `lsqcurvefit`
Step-by-Step Walkthrough
- Setting Up Your Data: Before fitting, prepare your dataset. For instance, generating synthetic data can be useful for practice:
xdata = linspace(0, 10, 100);
ydata = 5 * exp(-0.7 * xdata) + randn(size(xdata)); % synthetic noisy data
- Calling the `lsqcurvefit` Function: Use the `lsqcurvefit` function to fit your model to the data. Here’s how a complete execution looks:
% Initial guess for the parameters
beta0 = [1, -1];
% Execute lsqcurvefit
beta = lsqcurvefit(modelFun, beta0, xdata, ydata);
Analyzing the Output
The output from `lsqcurvefit`, represented by `beta`, holds the optimized parameters that best fit your data according to the least squares criteria. It is essential to review these values in the context of your model to ensure they are physically meaningful and accurately represent the underlying process.
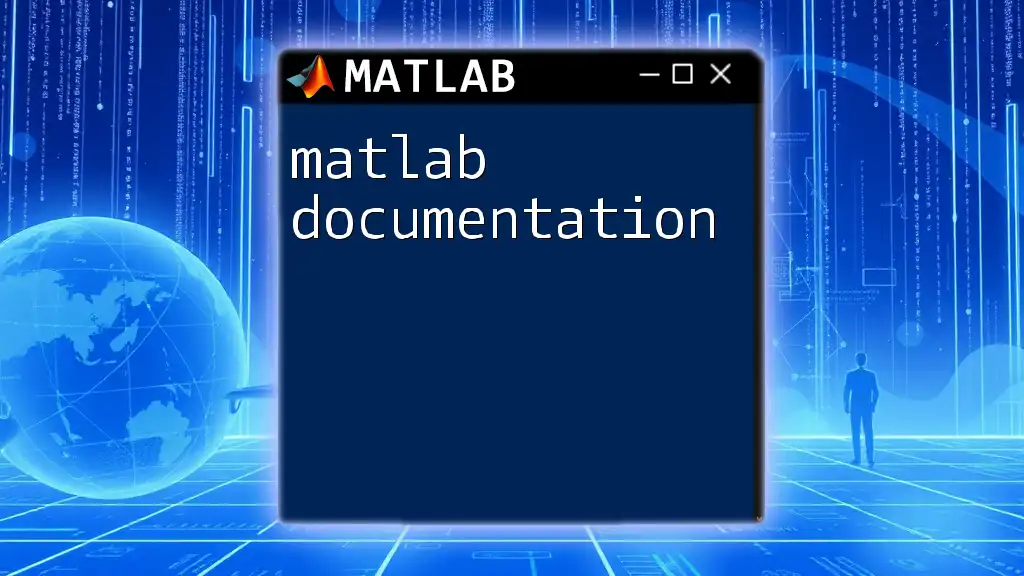
Advanced Features of `lsqcurvefit`
Control Options and Optimization
MATLAB's `optimoptions` functionality allows you to control the behavior of the optimization algorithm used in `lsqcurvefit`. You can adjust settings such as tolerances and maximum iterations, which may be beneficial for specific datasets or models that require fine-tuning for convergence:
options = optimoptions('lsqcurvefit', 'Display', 'iter', 'TolFun', 1e-6);
beta = lsqcurvefit(modelFun, beta0, xdata, ydata, [], [], options);
Weighting the Fit
In some instances, specific observations may carry more significance than others. Applying weights through `lsqcurvefit` allows you to account for this variability. For example:
weights = 1./(ydata.^2); % Example weights indicating the reliability of each observation
beta_weighted = lsqcurvefit(modelFun, beta0, xdata, ydata, [], [], weights);
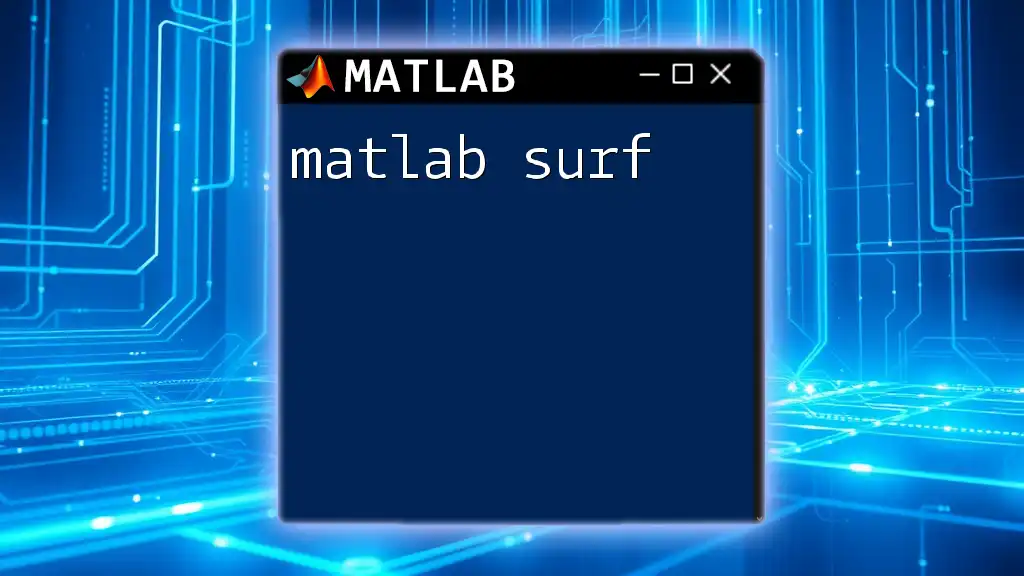
Visualizing the Results
To evaluate how good your model fit is, visualizing your results becomes critical. You can plot your original data alongside your fitted curve for a clear graphical representation:
% Plotting the results
figure;
scatter(xdata, ydata, 'r', 'filled'); hold on; % Original data
y_fit = modelFun(beta, xdata);
plot(xdata, y_fit, 'b-'); % Fitted curve
title('Data and Fitted Curve');
xlabel('X Data');
ylabel('Y Data');
legend('Data', 'Fitted Curve');
This simple visualization technique helps you analyze how well the model corresponds to the data points.
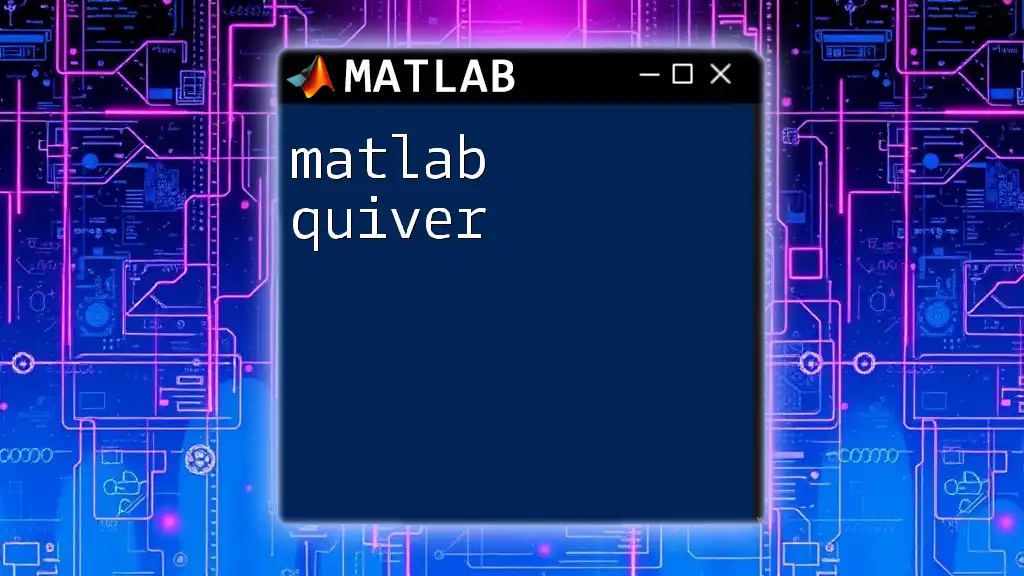
Common Errors and Troubleshooting Tips
Identifying Common Issues
When using `lsqcurvefit`, you might encounter convergence problems or dimensionality errors. For convergence issues, it's often beneficial to review the model, the initial guesses, and the nature of your data.
Strategies to Improve Fitting Performance
Improving the performance of the fitting can involve several methods such as:
- Refining Initial Guesses: Test with various initial values to ensure more reliable convergence.
- Adapting the Model Function: Sometimes, a more complex model may be required if the current function does not capture the data trend.
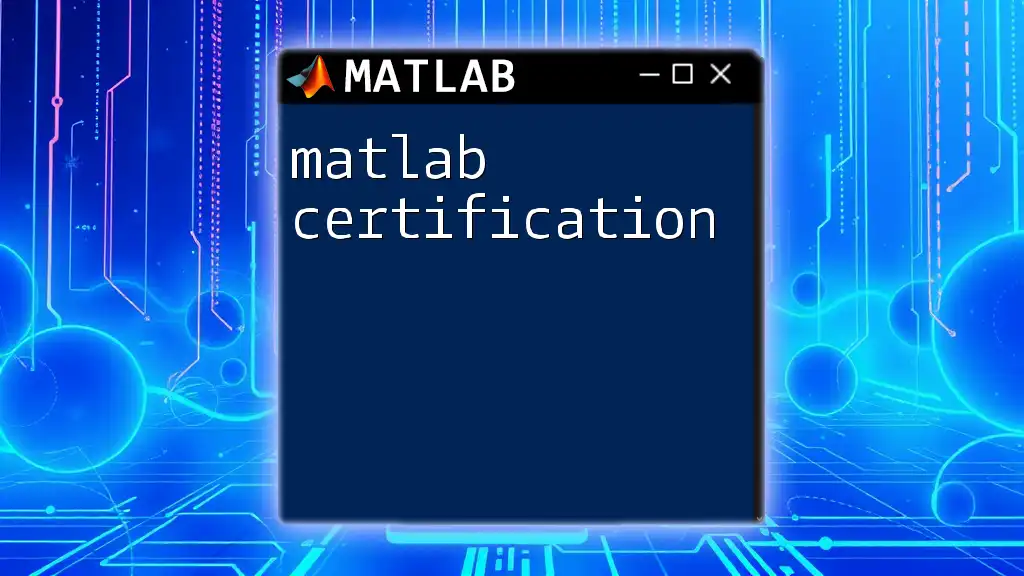
Case Studies
Example 1: Fitting an Exponential Decay Model
Let’s consider applying `lsqcurvefit` to a practical scenario like fitting an exponential decay model to real-world data, which could involve data collected from experiments that track the decay of a radioactive sample.
The synthetic data might look something like this:
xdata = linspace(0, 5, 50);
ydata = 10 * exp(-1 * xdata) + randn(size(xdata)); % Decay data with noise
You’d define your exponential decay function, set your initial guesses, and utilize `lsqcurvefit` to arrive at the optimized parameters.
Example 2: Fitting a Logistic Growth Model
Consider fitting a logistic growth model to data representing population growth, which often saturates as resources become limited. The logistic growth model is structured as follows:
modelFun = @(b,x) b(1) ./ (1 + exp(-b(2) * (x - b(3))));
Here, `b(1)` is the carrying capacity of the environment, `b(2)` relates to the growth rate, and `b(3)` indicates the time at which the population is half of the carrying capacity.
Using appropriate data and the `lsqcurvefit` method, you can extract meaningful parameters from such a model.
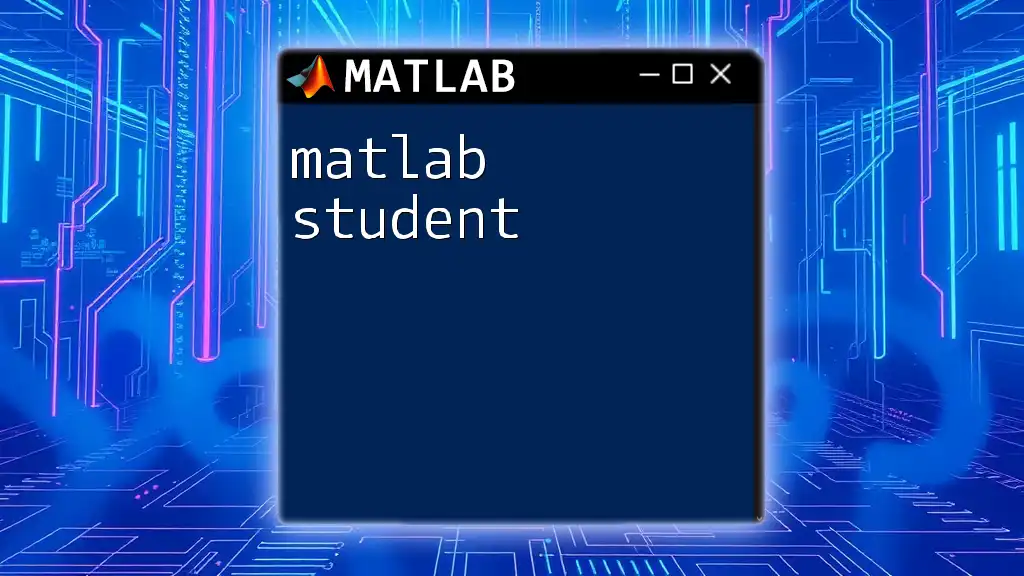
Conclusion
In summary, `lsqcurvefit` in MATLAB is a powerful tool for nonlinear curve fitting, enabling users to derive optimized parameters that best explain their data. By choosing the right model, leveraging initial guesses wisely, and interpreting the output correctly, you can efficiently address various data fitting challenges. Beyond basic implementations, adjusting control parameters and applying weights can further refine your results, guiding more informed decision-making in data analysis.
As you experiment with different models and datasets, remember that the key to mastering curve fitting lies in practice and exploration. With the tools at your disposal in MATLAB, the possibilities are extensive and engaging.
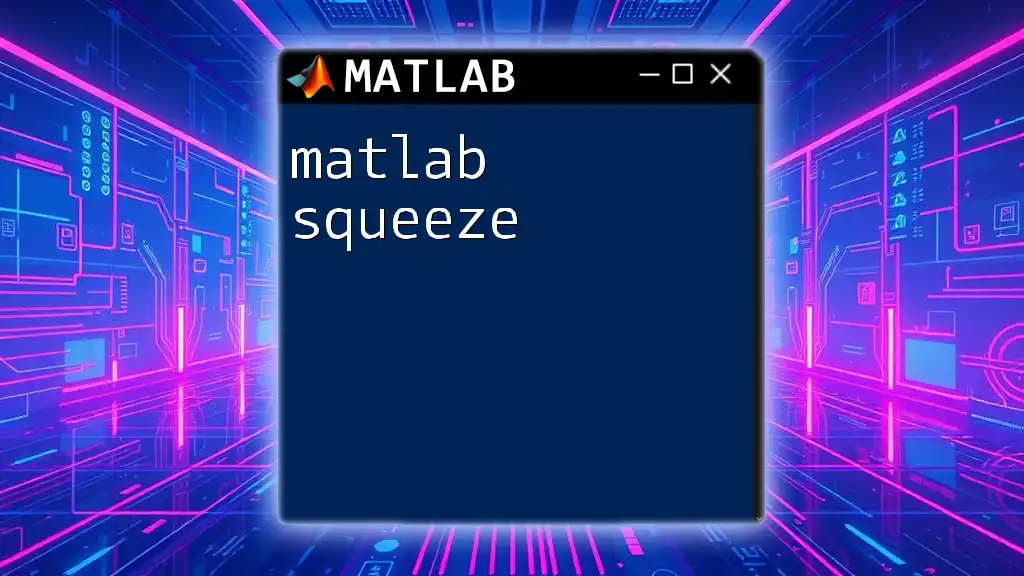
Additional Resources
For further study, consider exploring additional literature on curve fitting techniques, as well as the official MATLAB documentation that provides in-depth insights. Such resources can offer supplementary knowledge that will bolster your understanding of the intricate world of data fitting using MATLAB's `lsqcurvefit`.