The "matlab square" command typically refers to squaring numbers or matrices in MATLAB, and can be accomplished using the power operator `.^` or the `^` operator for matrices.
Here’s an example in MATLAB:
% Squaring a number
number = 5;
squaredNumber = number^2;
% Squaring a matrix
matrix = [1, 2; 3, 4];
squaredMatrix = matrix^2;
Understanding Squaring Numbers in MATLAB
What is Squaring?
Squaring a number refers to multiplying that number by itself. For example, squaring the number 4 means calculating \(4 \times 4\), which equals 16. This operation is fundamental in various mathematical computations, including physics and statistics. Squared values often appear in formulas like those used for variance calculation, quadratic equations, and geometric properties such as area.
Basic Command for Squaring
In MATLAB, you can perform squaring using the exponentiation operator `^`. The syntax allows you to raise any number to a certain power.
Here’s how you can easily square a number:
% Squaring a number
num = 5;
squaredNum = num^2;
disp(squaredNum);
In this example, when you execute the code, the expected output will be `25`. This represents \(5^2\), showcasing how straightforward it is to work with squaring in MATLAB.
Alternative Methods to Square a Number
Using the `power` Function
An alternative approach to square a number in MATLAB is by using the built-in `power` function. This function can be useful when you want clarity in your code or when squaring larger matrices.
Here’s how you can use `power`:
% Using the power function
squaredNum = power(5, 2);
disp(squaredNum);
This code will also output `25`. The beauty of using `power` lies in its ability to extend to more complex calculations, making it easier to convey intention in your code.
Using Element-wise Squaring
When working with arrays, squaring can be performed element-wise. In MATLAB, you can easily square each element of an array using the `.^` operator. This is particularly useful in mathematical or engineering contexts where you manipulate datasets.
Here’s an example:
% Element-wise squaring for an array
A = [1, 2, 3, 4];
squaredArray = A.^2;
disp(squaredArray);
For the above code, the output will be `[1, 4, 9, 16]`. Each element of the original array has been squared individually, showcasing MATLAB's efficient array operations.

Visualizing Squares in MATLAB
Plotting Squares
Visualizing mathematical equations can greatly enhance understanding, and one of the simplest forms is plotting the function \(y = x^2\). Here’s how you can create a plot in MATLAB:
- Define a range of `x` values.
- Calculate `y` using the squaring operation.
- Use the `plot` function to create the graph.
% Plotting y = x^2
x = -10:0.1:10; % Define x range
y = x.^2; % Calculate squared values
plot(x, y); % Create the plot
title('Plot of y = x^2');
xlabel('x');
ylabel('y');
grid on; % Add grid for better visualization
When you run this code, you’ll see a parabola opening upwards, representing the squaring function. The title and axis labels help in understanding the graph at a glance.
Customizing Plots
MATLAB also allows for extensive customization of your plots. You can modify colors, line styles, and add markers to make your plots more informative and visually appealing. For example, let’s customize the above plot:
% Customizing the plot
plot(x, y, 'r--', 'LineWidth', 2); % Red dashed line
title('Customized Plot of y = x^2');
xlabel('x');
ylabel('y');
legend('y = x^2');
grid on; % Add grid for better visualization
This code changes the line to a red dashed style with increased thickness. Adding a legend makes it clear what the graph represents.

Applications of Squaring in MATLAB
Statistical Analysis
In the realm of statistics, squaring plays a pivotal role, especially when calculating variance. The variance measures how far a set of numbers is spread out from their average value. Squaring the differences from the mean is crucial in these calculations.
For instance:
% Calculating variance using squaring
data = [2, 4, 6, 8, 10]; % Sample data
meanValue = mean(data); % Calculate the mean
variance = mean((data - meanValue).^2); % Calculate variance
disp(variance);
This code snippet first computes the mean of the dataset, then calculates the variance by squaring the differences from the mean. This demonstrates how squaring is integrated into statistical functions to derive meaningful insights.
Solving Equations
Squaring numbers is essential when solving quadratic equations of the form \(ax^2 + bx + c = 0\). MATLAB’s built-in functions make solving these equations straightforward. You can utilize `roots` to find the roots of a polynomial.
For example:
% Solving quadratic equation ax^2 + bx + c = 0
coefficients = [1, -3, 2]; % Represents x^2 - 3x + 2 = 0
solutions = roots(coefficients); % Calculate roots
disp(solutions);
Upon executing this code, MATLAB will provide the roots of the equation, helping us know where the parabola intersects the x-axis. This is impactful in various engineering applications where modeling behaviors is crucial.
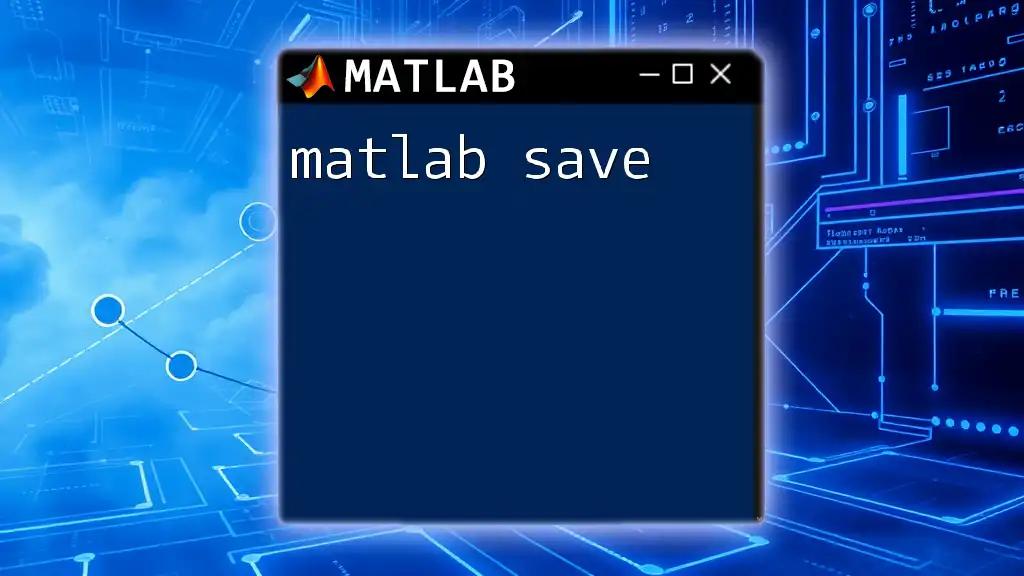
MATLAB Tips and Best Practices
Efficient Coding Practices
When working with MATLAB, leveraging vectorization greatly enhances performance. Instead of using loops to square each element, use vectorized operations provided by MATLAB to execute operations more efficiently. This approach not only speeds up your code but also makes it more readable.
Common Errors and Debugging Tips
Some common errors when working with squaring, particularly with matrices, arise from using the wrong operator. Always double-check to ensure you are using `.^` for element-wise squaring instead of `^`, which applies to matrix powers.
To help debug, utilize MATLAB’s debugging tools such as breakpoints and the `disp` function to track variable values at various stages in your code.

Conclusion
Understanding how to effectively use the matlab square operation enables you to improve your mathematical modeling skills and enhances your coding efficiency. By mastering various methods of squaring, visualizing these operations, and applying them to real-world problems, you position yourself well in tackling MATLAB challenges.
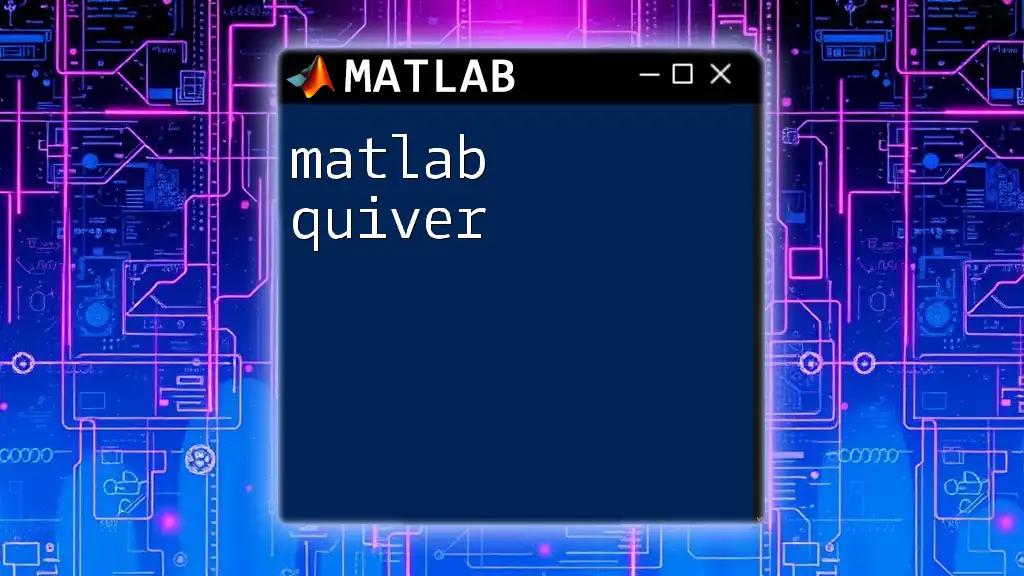
Resources for Further Learning
For those interested in deepening their understanding of MATLAB, consider exploring textbooks that focus on numerical methods, enrolling in online courses that specialize in MATLAB programming, or diving into official MATLAB documentation to uncover advanced functionalities related to squaring and visualizations. Engaging with practical projects will further cement your knowledge, making you proficient in this versatile programming environment.