MATLAB curve fitting is a technique used to create a continuous curve that best represents a set of data points, allowing for predictions and analysis of trends in the data.
Here’s a simple code snippet that demonstrates how to perform curve fitting using the `fit` function in MATLAB:
% Sample data
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Fit a linear polynomial (line) to the data
ft = fit(x', y', 'poly1');
% Plot the data and the fit
plot(ft, x, y);
title('Curve Fitting Example');
xlabel('X-axis');
ylabel('Y-axis');
Understanding Basic Concepts
What is a Fit?
In the context of data analysis, a fit refers to a mathematical function that describes the trend of a dataset. The goal of curve fitting is to find a curve that best represents the relationship between variables. There are two primary types of fits:
- Linear Fits: These assume a straight-line relationship between variables (e.g., \(y = mx + b\)).
- Non-linear Fits: These accommodate more complex relationships and may take forms such as polynomial, exponential, or logarithmic equations.
An essential concept in curve fitting is the analysis of residuals, which are the differences between observed values and the values predicted by the fitted model. Evaluating residuals helps determine how well the model fits the data, leading to the concept of Goodness of Fit.
Common Uses of Curve Fitting
Curve fitting is ubiquitous across various fields. In engineering, it is used for process modeling and simulation. In scientific research, researchers employ it to analyze experimental data and extract meaningful insights. Furthermore, businesses use curve fitting for economic forecasting, predicting trends based on historical data.
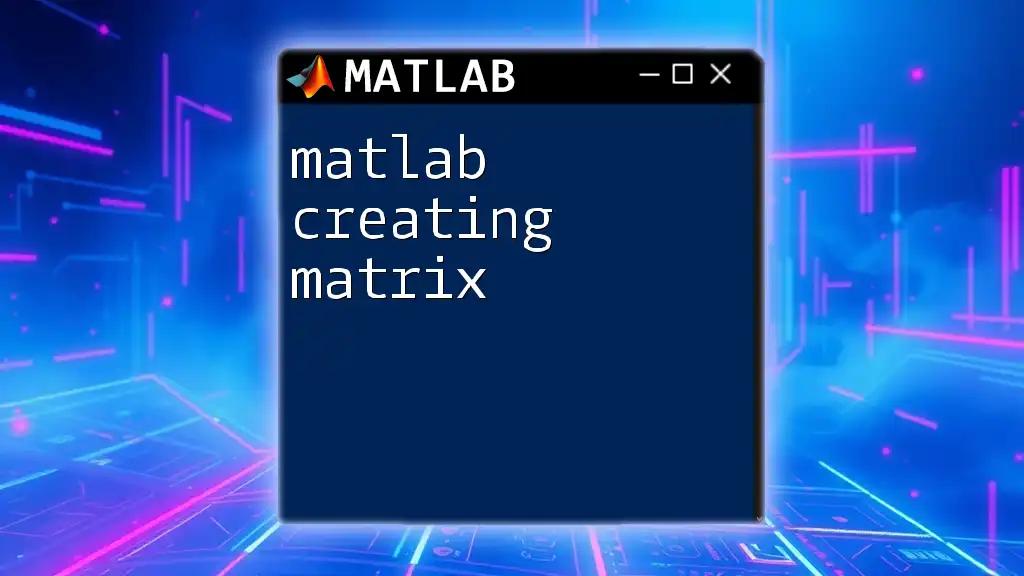
Getting Started with MATLAB
Setting Up MATLAB for Curve Fitting
Before diving into curve fitting, ensure you have the required software. MATLAB is a powerful tool equipped with a Curve Fitting Toolbox that enhances its functionality. If you haven’t already, check the installation guidelines on MathWorks and ensure your toolbox is ready for use.
Importing Data into MATLAB
Data must be prepared for curve fitting. MATLAB supports various data formats, including CSV, Excel, and TXT. Here’s a simple example of loading data from a CSV file into MATLAB:
data = readtable('data.csv');
x = data.x;
y = data.y;
In this snippet:
- `data` is the table variable storing the imported data.
- `x` and `y` are the vectors that will be used for fitting.
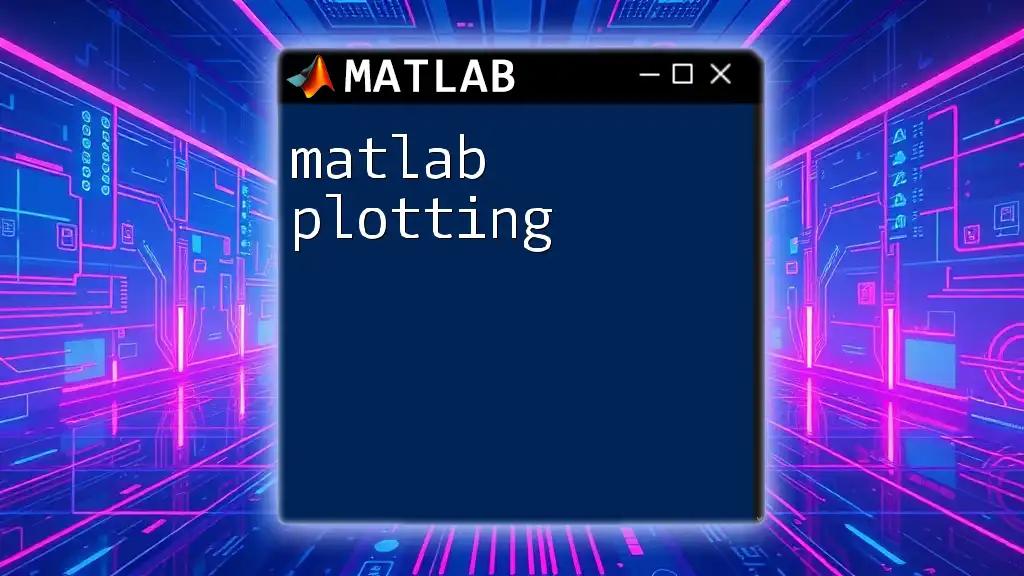
Types of Curve Fitting
Linear Curve Fitting
Linear models serve as the fundamental building blocks in data analysis. To execute linear curve fitting in MATLAB, you can use the `polyfit` function, which computes the coefficients for a polynomial of a specified degree. The following example demonstrates a linear fit:
p = polyfit(x, y, 1);
Here, `1` signifies that you're fitting a linear polynomial.
To visualize the results of your linear fit, you can plot the data along with the fitted line:
yfit = polyval(p, x);
plot(x, y, 'o', x, yfit, '-');
title('Linear Fit');
xlabel('X-axis');
ylabel('Y-axis');
This code plots the original data points (in circles) and the fitted line (in a solid line), enabling you to see how well the linear model fits the data.
Polynomial Curve Fitting
Polynomial curve fitting extends the concept of linear fits to allow for curvatures. You can achieve polynomial fitting similarly with `polyfit`. For example, a second-degree polynomial fit can be performed as follows:
p2 = polyfit(x, y, 2);
The coefficients found can be used to evaluate the fit and its accuracy. For instance, you can examine the residuals:
residuals = y - polyval(p2, x);
Analyzing the residuals will help you gauge how well your model describes the data.
Non-linear Curve Fitting
Non-linear models offer even greater flexibility when fitting complex datasets. MATLAB provides the `fit` function to tackle non-linear fits. This is demonstrated below with an exponential model:
ft = fittype('a*exp(b*x)', 'independent', 'x');
fitresult = fit(x, y, ft);
In this example, `fitresult` contains your fitted model parameters (a and b). You can evaluate fit quality using R-squared values or by visualizing the fit:
plot(fitresult, 'Style', 'plot');
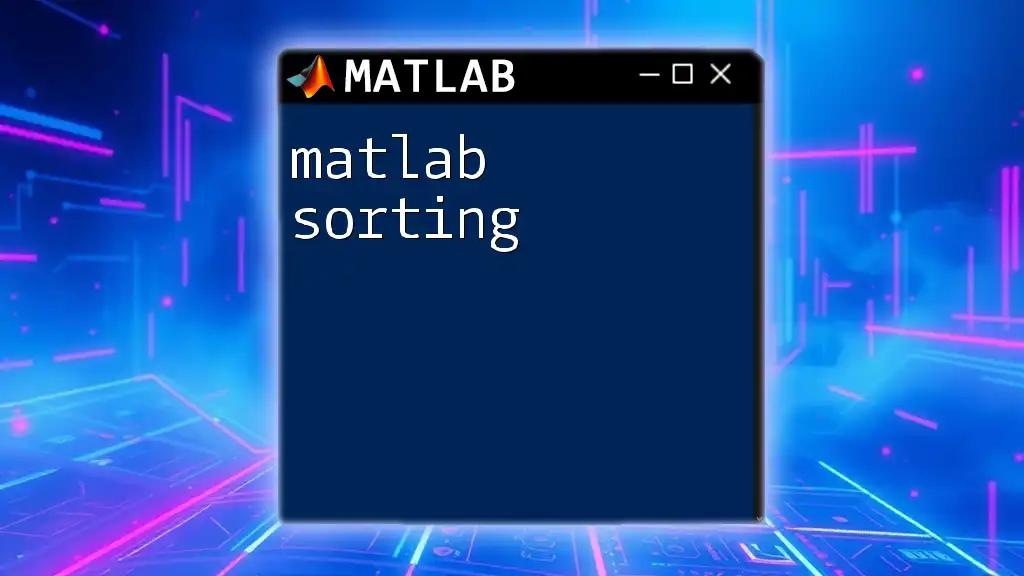
Advanced Curve Fitting Techniques
Multiple Curve Fitting
When working with multiple datasets or trends, it may be necessary to fit multiple curves effectively. This can be accomplished by implementing loops in MATLAB. For instance, if you have multiple datasets stored in a cell array, you can iterate over each one, applying the fitting functions consistently.
Model Validation Techniques
It is critical to validate your model to ensure its reliability. Employing cross-validation methods can help assess how the model performs on unseen data. Diagnostic plots, such as residual plots and QQ plots (quantile-quantile plots), are useful for visualizing any deviations from the expected pattern.
Customizing Fit Models
Sometimes, fitted models do not conform to standard equations. MATLAB allows creating bespoke models, accommodating unique relationships found in specific datasets. For instance, to define a custom equation:
ft_custom = fittype('a*x^b + c', 'independent', 'x', 'dependent', 'y');
In this case, parameters \(a\), \(b\), and \(c\) can be estimated based on your data, giving you precise control over the model.

Visualization and Interpretation of Fit Results
Plotting Fitted Curves
Visualizing fitted curves against your original data strengthens your analysis. Use MATLAB’s plotting functions to overlay fitted curves convincingly:
plot(fitresult, 'Style', 'plot');
Doing this helps confirm whether the model indeed captures the underlying trends in the data effectively.
Analyzing Fit Output
When your model fitting is complete, interpreting model coefficients becomes paramount. Understanding the significance of each parameter can give you deeper insight into the data relationship. Additionally, evaluate fit statistics like the residual standard error and \(R^2\), which elucidate the model’s explanatory power.
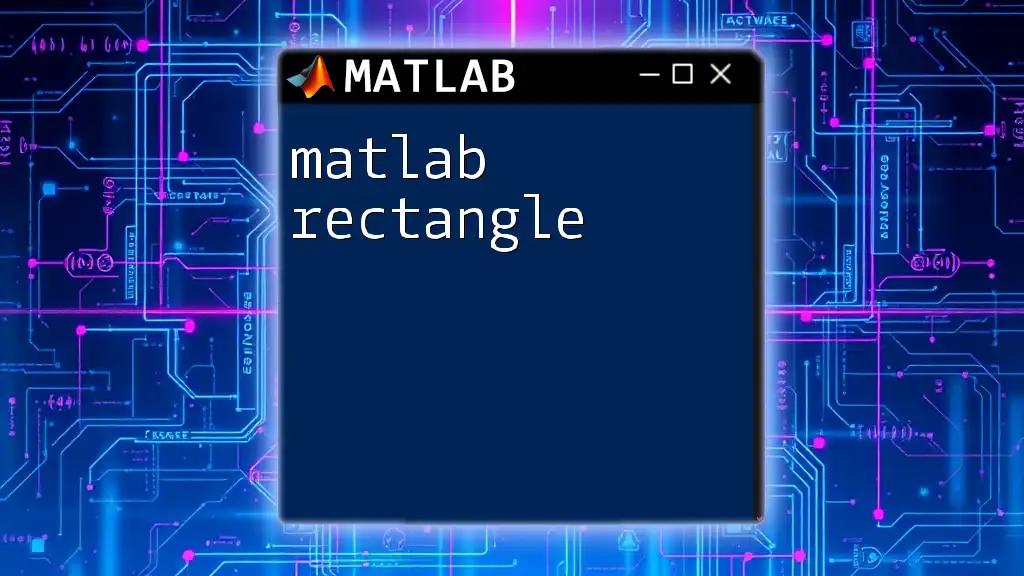
Troubleshooting Common Issues
Poor Fit Quality
Encountering poor fit quality is not uncommon. Signs include high residuals and coefficients that lack biological or physical interpretation. To improve model fit, you may need to try alternative fitting methods, re-evaluate the chosen model type, or consider transforming your data.
Handling Outliers
Outliers can skew your results dramatically. Techniques for detecting outliers include visual inspections of residual plots. After identifying outliers, you can remove them and re-fit the model to obtain a more accurate representation of the data.
Best Practices for Curve Fitting
To ensure quality in your curve fitting efforts, keep the following tips in mind:
- Choose the right model based on data characteristics.
- Always visualize your data and fitted curves.
- Regularly assess and validate model fits against new or synthetic datasets.
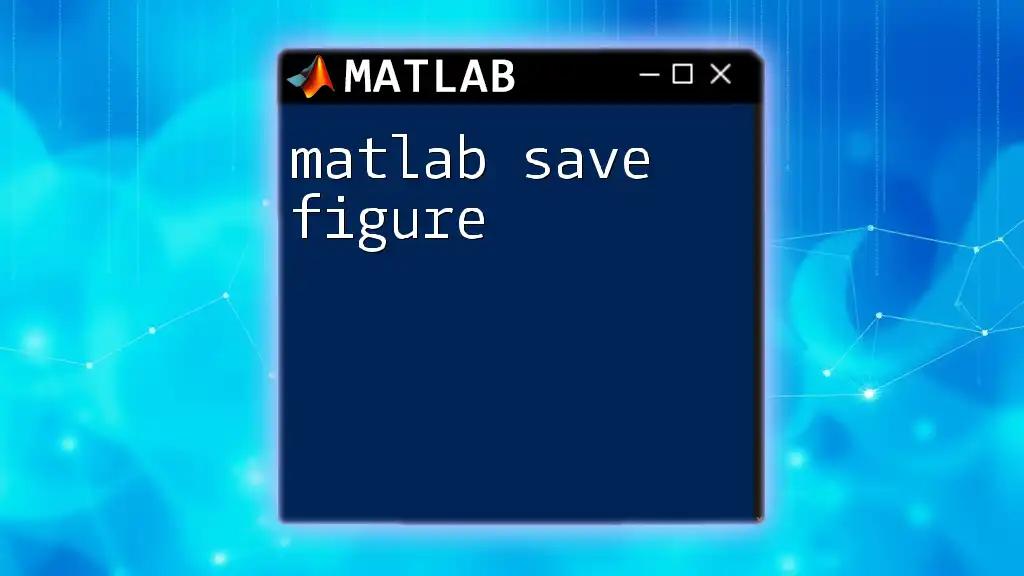
Conclusion
In summary, mastering MATLAB curve fitting equips you with powerful tools for extracting insights from your data. By understanding the concepts discussed and practicing the code snippets provided, you can confidently apply curve fitting techniques across various applications. Don't hesitate to explore further resources to expand your knowledge and capabilities in MATLAB!