LU factorization is a matrix decomposition technique that expresses a given square matrix as the product of a lower triangular matrix (L) and an upper triangular matrix (U).
Here's a simple example of LU factorization in MATLAB:
A = [4, 3; 6, 3];
[L, U] = lu(A);
What is LU Factorization?
LU Factorization is a fundamental technique in numerical analysis that decomposes a given matrix into two simpler components: a lower triangular matrix \( L \) and an upper triangular matrix \( U \). This decomposition can be expressed as:
\[ A = LU \]
where \( A \) is the original matrix, \( L \) contains all the elements below and on the diagonal, and \( U \) contains all elements above and on the diagonal.
Importance in Numerical Methods
LU Factorization plays a critical role in various numerical methods. It simplifies the process of solving linear systems of equations, making it easier and more efficient to find solutions, especially when multiple systems share the same coefficient matrix. LU Factorization also underpins many algorithms in engineering and scientific computations, ensuring that these calculations can be conducted with increased accuracy and speed.
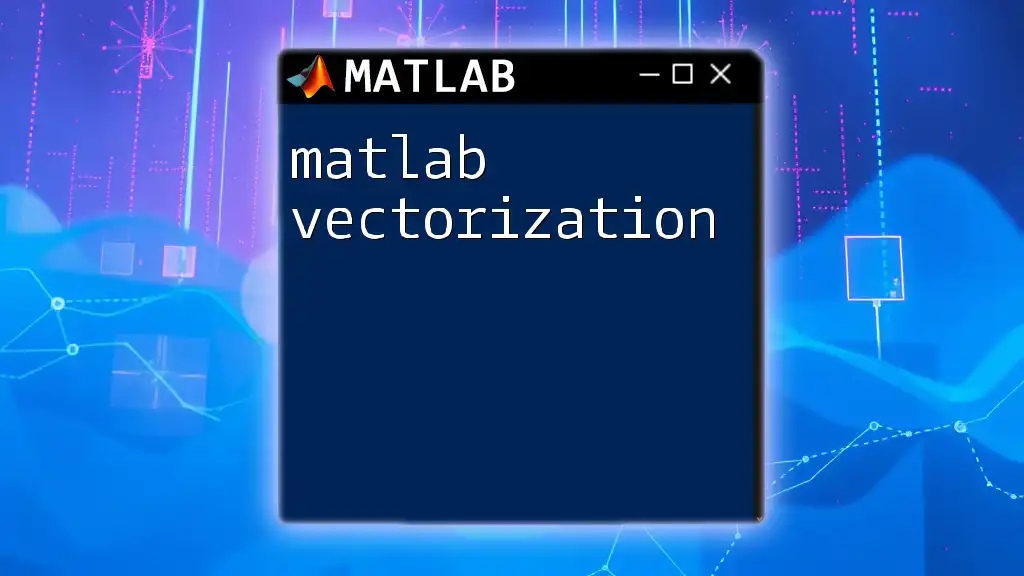
Mathematical Background
Matrix Types
LU Factorization is primarily applied to square matrices, meaning matrices that have the same number of rows and columns. Although it can also be extended to some rectangular matrices, the standard application focuses on square matrices. For matrices that are sparse, special techniques may be needed to enhance computational efficiency and reduce memory usage.
Properties of L and U
The characteristics of \( L \) and \( U \) matrices have significant mathematical implications. A lower triangular matrix \( L \) has ones on its diagonal and zeros above it, while an upper triangular matrix \( U \) has zeros below its diagonal. These properties make \( L \) and \( U \) particularly useful because they simplify the processes of matrix operations, such as calculating the determinant and the inverse.
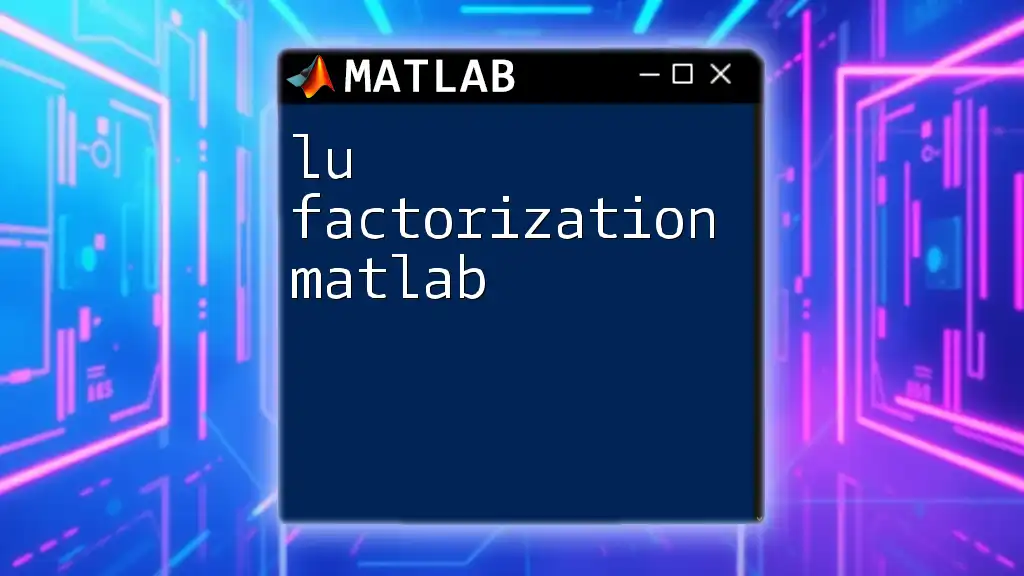
How to Perform LU Factorization in MATLAB
Using `lu()` Function
MATLAB provides a built-in function called `lu()` that makes LU Factorization straightforward to implement.
Syntax
The syntax for the `lu()` function is as follows:
[L, U] = lu(A)
where \( A \) is the matrix you wish to factor.
Example
Here’s a simple way to use the `lu()` function:
A = [4, 3; 6, 3];
[L, U] = lu(A);
disp('Lower triangular matrix L: '), disp(L)
disp('Upper triangular matrix U: '), disp(U)
In this example, the original matrix \( A \) is decomposed into matrices \( L \) and \( U \), which can then be used in further calculations.
Step-by-Step Manual Factorization
For educational purposes, understanding how to perform LU Factorization manually can solidify your comprehension of the process. Let’s consider a simple \( 2 \times 2 \) matrix \( A \):
\[ A = \begin{bmatrix} a & b \\ c & d \end{bmatrix} \]
To perform manual LU Factorization, follow these steps:
- Set \( L \) as a lower triangular matrix and \( U \) as an upper triangular matrix. Initially, they can be expressed as:
\[ L = \begin{bmatrix} 1 & 0 \\ l_{21} & 1 \end{bmatrix}, \quad U = \begin{bmatrix} u_{11} & u_{12} \\ 0 & u_{22} \end{bmatrix} \]
- To find \( l_{21} \), calculate:
\[ l_{21} = \frac{c}{u_{11}} \text{, where } u_{11} = a \]
- Subsequently, use this to find the values for \( u_{12} \) and \( u_{22} \):
\[ u_{12} = b \quad \text{and } u_{22} = d - l_{21} \cdot u_{12} \]
Following these steps will denote how \( A \) can be successfully expressed as the product \( LU \).
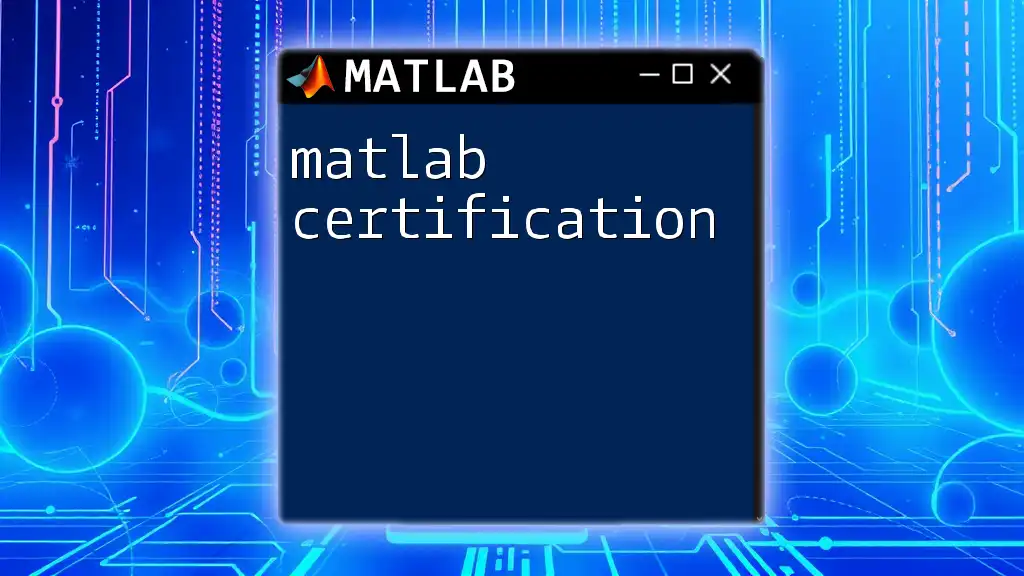
Applications of LU Factorization
Solving Linear Systems
One of the primary uses of LU Factorization is solving linear systems of equations in the form \( Ax = b \). Here’s how the process works:
- Decompose \( A \) into \( L \) and \( U \) using the `lu()` function.
- Solve for \( y \) in the equation \( Ly = b \).
- Finally, solve for \( x \) in the equation \( Ux = y \).
Here's how you can perform this in MATLAB:
b = [11; 15];
y = L\b; % Solving Ly = b
x = U\y; % Solving Ux = y
disp('Solution x: '), disp(x)
Matrix Inversion
LU Factorization can also be utilized to compute the inverse of a matrix. The relationship is derived from the property that if \( A = LU \), then:
\[ A^{-1} = (LU)^{-1} = U^{-1} L^{-1} \]
This means once you have calculated \( L \) and \( U \), you can find the inverse using:
invA = U \ (L \ eye(size(A)));
Determinants
Another application of LU Factorization is to calculate the determinant of a matrix. The relationship is given by:
\[ \text{det}(A) = \text{det}(L) \cdot \text{det}(U) \]
Since \( L \) is unit lower triangular (i.e., has ones on its diagonal), its determinant is simply 1. Therefore, the determinant of \( A \) can be computed as the product of the diagonal elements of \( U \):
detA = prod(diag(U)); % Since L is unit lower triangular
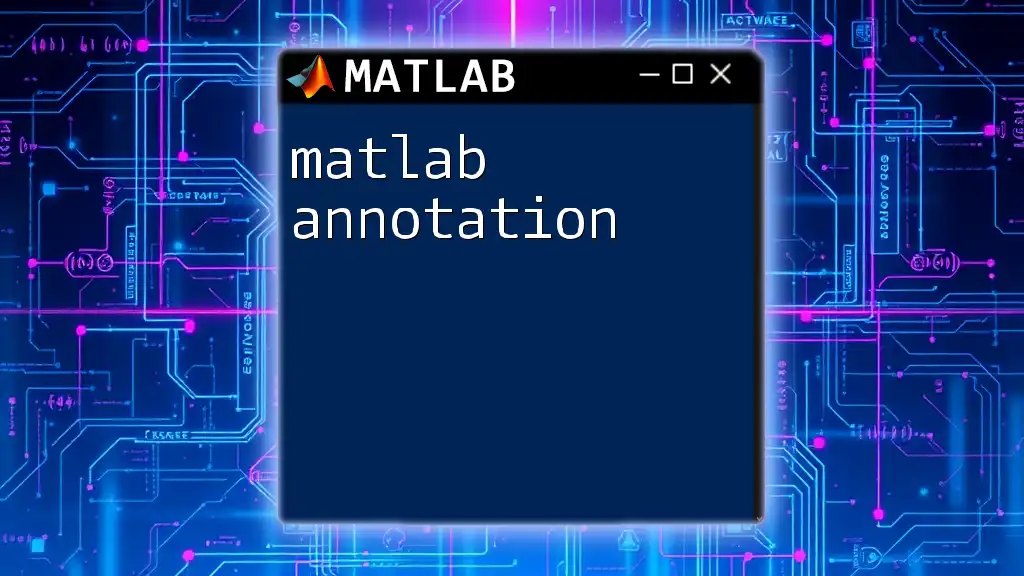
Advantages and Limitations of LU Factorization
Advantages
Computational Efficiency: LU Factorization is computationally less expensive compared to other methods used for solving linear systems, particularly in larger matrices.
Stability in numerical methods: It enhances the accuracy of computations by reducing round-off errors.
Reusability: When you need to solve multiple equations with the same coefficient matrix, LU Factorization allows for the reuse of \( L \) and \( U \), significantly speeding up computations.
Limitations
Not all matrices can be factored using LU. Singular matrices or matrices that are not square cannot be effectively decomposed. Additionally, pivoting is often required to improve numerical stability, which can complicate the factorization process.
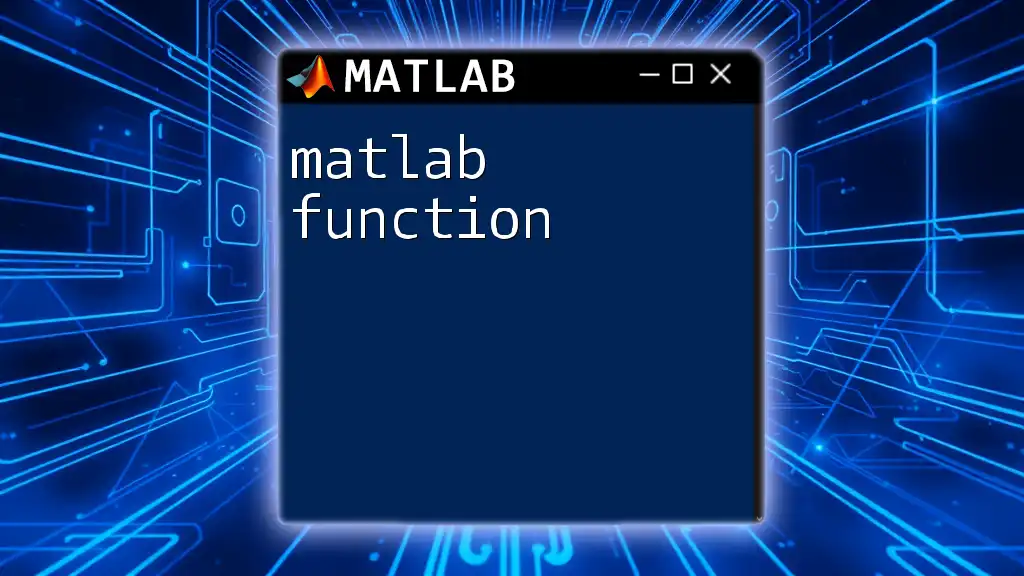
Conclusion
Understanding MATLAB LU Factorization is crucial for anyone involved in computational mathematics, engineering, or the sciences. By grasping both the theoretical aspects and practical applications, you can tackle a wide range of problems more efficiently. Mastering LU Factorization will not only aid in solving linear equations but also provide insights into matrix operations and numerical stability.
As you continue to practice and explore, you will uncover further applications and methodologies, expanding your MATLAB skill set and knowledge in numerical analysis.