A MATLAB function is a group of statements that perform a specific task, which can be reused throughout your code, and is defined using the `function` keyword followed by the output and input parameters.
Here’s a simple example of a MATLAB function that adds two numbers:
function sum = addNumbers(a, b)
sum = a + b;
end
Understanding MATLAB Functions
What is a MATLAB Function?
A MATLAB function is a group of statements that together perform a specific task. Unlike scripts, which run in the base workspace, functions have their own workspace and can accept input and returns outputs. This encapsulation makes functions particularly useful for code reusability and organization.
Structure of a MATLAB Function
The syntax of a MATLAB function can be broken down into several components:
- Function Keyword: Every function begins with the `function` keyword.
- Input Arguments: These are variables passed to the function for processing.
- Output Variables: The results returned after the function execution.
- Function Body: This consists of the code that defines what the function does.
The basic structure looks like this:
function [output1, output2] = functionName(input1, input2)
% Function body
end
Types of Functions in MATLAB
Built-in Functions
MATLAB comes equipped with a myriad of built-in functions that simplify tasks such as mathematical computations, data analysis, and visualization. Some commonly used built-in functions include:
- `sum()`: Computes the sum of array elements.
- `mean()`: Returns the average of array elements.
- `plot()`: Creates a 2D line plot of data.
Example: Using a built-in function to calculate the mean of an array.
data = [1, 2, 3, 4, 5];
average = mean(data);
disp(average); % Output: 3
User-Defined Functions
User-defined functions are essential in MATLAB, allowing users to tailor functionalities that are specific to their needs. To create a user-defined function, follow these steps:
- Define the function in a `.m` file (the filename should match the function name).
- Use the `function` keyword, followed by the output, function name, and inputs.
Example: A simple user-defined function to calculate the area of a rectangle.
function area = rectangleArea(length, width)
area = length * width;
end
When called with specific values, this function returns the area.
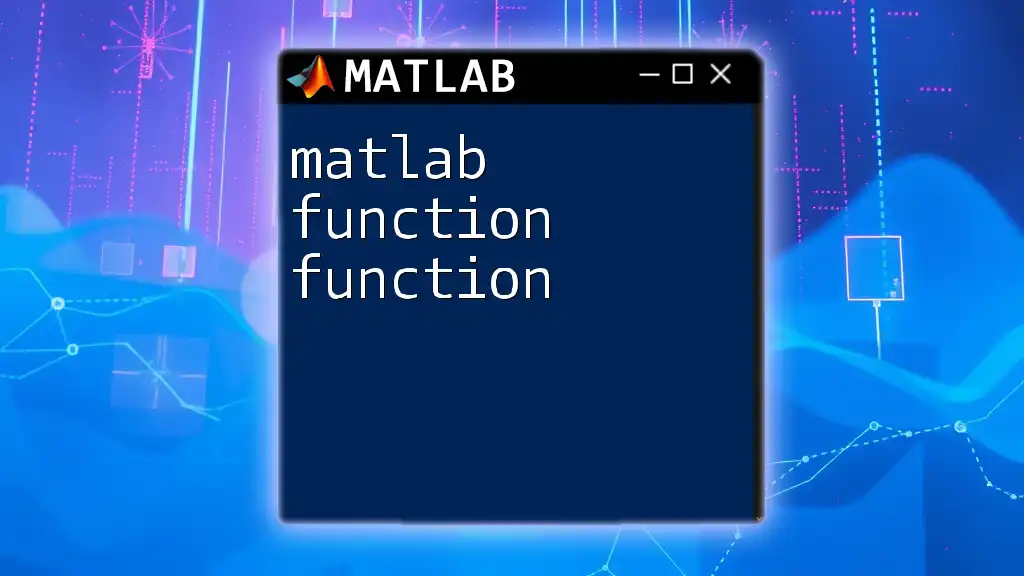
Creating MATLAB Functions
Writing Your First MATLAB Function
Writing a function involves creating a separate file with the aforementioned structure. Let’s walk through a more detailed example where we create a function to calculate the area of a rectangle:
function area = rectangleArea(length, width)
% This function calculates the area of a rectangle
area = length * width;
end
To call the function:
areaRect = rectangleArea(5, 10); % Returns 50
disp(areaRect);
This example illustrates how straightforward it is to build reusable code in MATLAB.
Function File Naming Conventions
When creating function files in MATLAB, it’s vital to adhere to naming conventions for better organization and clarity. Function names must:
- Start with a letter
- Contain letters, digits, or underscores
- Not exceed 63 characters
Using Local and Global Variables
Local Variables
Local variables exist only within the function where they are created. This feature protects them from unintended interference from other parts of your code. For example:
function result = addNumbers(a, b)
sum = a + b; % 'sum' is a local variable
result = sum;
end
Global Variables
Global variables can be accessed from anywhere within a MATLAB session, including all functions and scripts. However, they should generally be avoided unless specifically required due to their potential to lead to complications and unexpected side effects.
function setGlobalValue(val)
global myGlobal; % Declare a global variable
myGlobal = val;
end
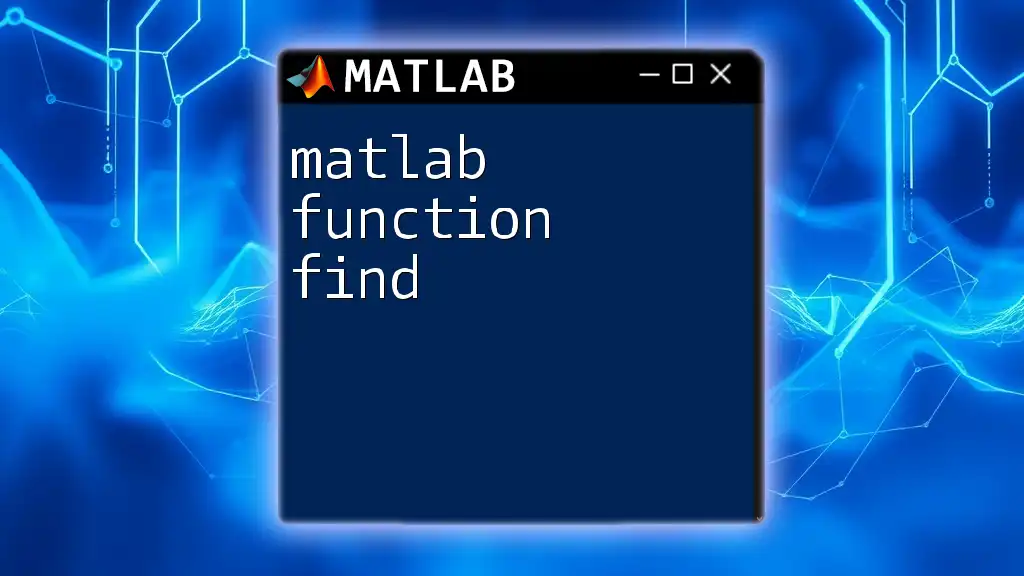
Function Inputs and Outputs
Input Arguments
Pass arguments to functions by placing them within the parentheses of the function definition. Inside the function body, you can use these input variables as regular variables. The special function `nargin` can be used to check how many arguments were passed.
function varArgFunc(varargin)
disp(['Number of input arguments: ', num2str(nargin)]);
end
When calling `varArgFunc(1, 2, 3)`, this would output "Number of input arguments: 3".
Output Variables
MATLAB allows functions to return multiple outputs, which can be particularly useful when a function produces several results. To do this, simply specify multiple output variables in square brackets.
Example: Creating a function that returns both the sum and product of two numbers:
function [sumResult, productResult] = myMath(a, b)
sumResult = a + b;
productResult = a * b;
end
When you call it like this:
[sumValue, productValue] = myMath(3, 5);
You get `sumValue = 8` and `productValue = 15`.
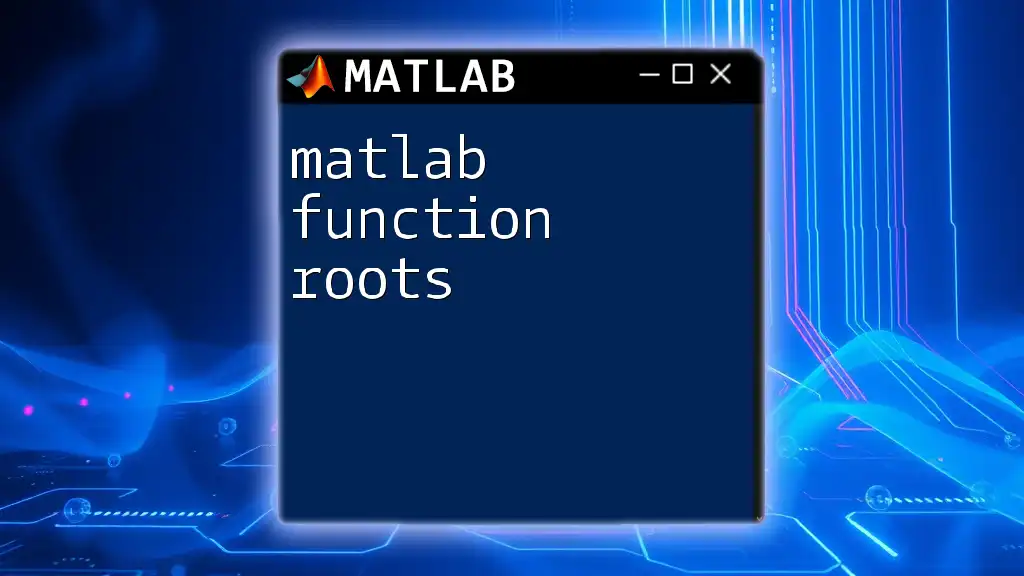
Common MATLAB Functions
Arithmetic Functions
MATLAB provides several built-in arithmetic functions. For instance:
- Sum
values = [1, 2, 3];
total = sum(values); % Returns 6
- Mean
average = mean(values); % Returns 2
String Functions
String functions allow manipulation of text data easily. Examples include `strcat` for joining strings and `upper` for converting strings to uppercase:
str1 = 'Hello';
str2 = 'World';
combinedStr = strcat(str1, ' ', str2); % Returns 'Hello World'
uppercaseStr = upper(combinedStr); % Returns 'HELLO WORLD'
Array Functions
Handling and manipulating arrays is a central feature in MATLAB. Functions like `reshape` allow you to change the dimensions of an array:
matrix = 1:6; % A row vector
matrix2D = reshape(matrix, 2, 3); % Reshapes into a 2x3 matrix
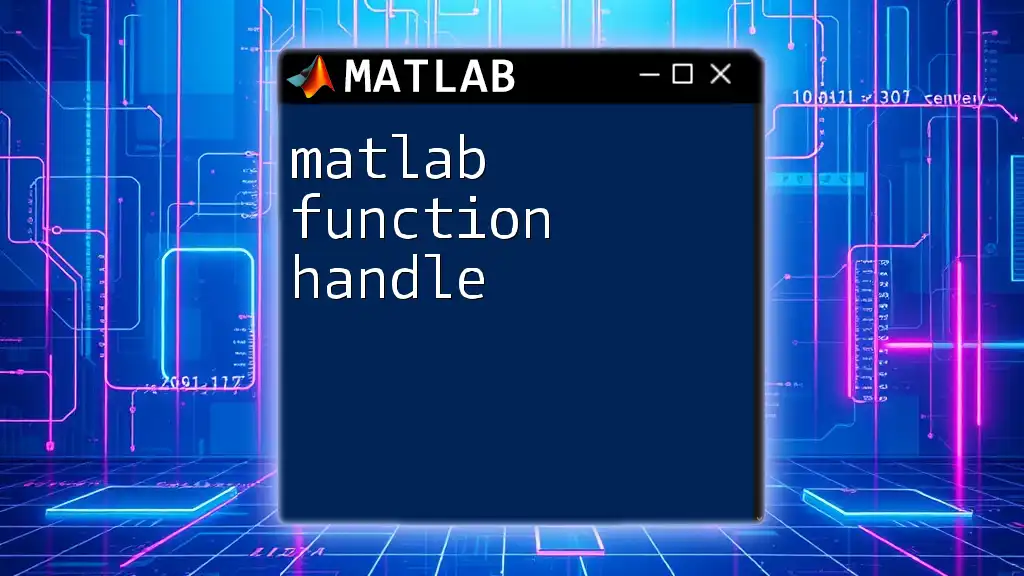
Best Practices for Function Design
Writing Clean and Readable Code
To ensure your functions are easy to read and maintain, consider the following tips:
- Use meaningful names for your functions and variables.
- Comment your code generously to explain what each part does, particularly complex calculations.
- Maintain consistent indentation and spacing for better readability.
Debugging MATLAB Functions
Debugging is an essential skill when writing MATLAB functions. Common techniques include:
- Using the `dbstop` command to set breakpoints,
- Executing functions step by step using `dbcont`.
Optimizing Function Performance
To enhance the efficiency of your functions, focus on avoiding unnecessary calculations and utilizing vectorization. For example, rather than using loops, you can often achieve better performance by applying operations directly to arrays.
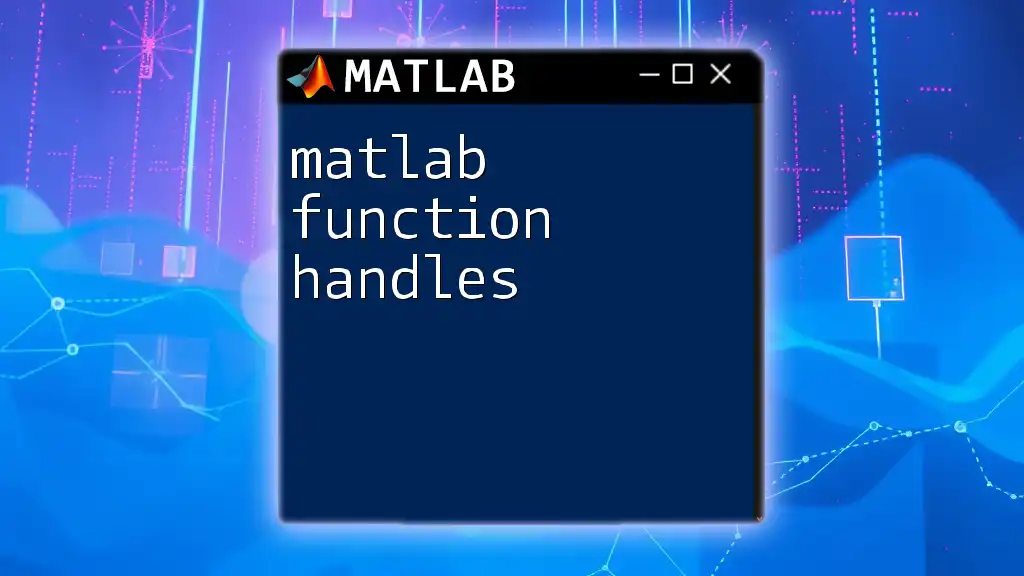
Conclusion
Becoming proficient with MATLAB functions is a transformative step in leveraging the power of MATLAB for computational tasks. Understanding how to create, utilize, and design functions effectively will not only improve the usability of your code but also enhance the overall productivity of your projects. The key to mastering MATLAB lies in consistent practice and exploration of its functionalities.
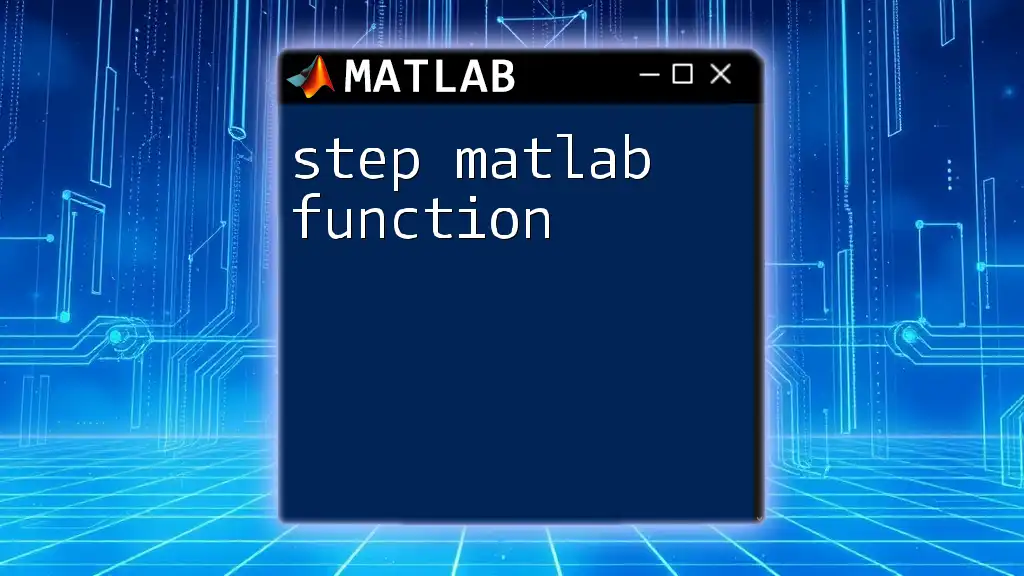
Additional Resources
For further learning, consider exploring books, online tutorials, and the MATLAB documentation that delve deeper into advanced functions and programming techniques. Engaging with community forums can also provide valuable insights and solutions to specific problems.