QR factorization is a mathematical technique that decomposes a matrix into a product of an orthogonal matrix \( Q \) and an upper triangular matrix \( R \), which is useful for solving linear systems and performing least squares fitting.
Here's a simple example of QR factorization in MATLAB:
A = [12 6; 4 3];
[Q, R] = qr(A);
Understanding QR Factorization
Fundamentals of QR Factorization
QR factorization is a mathematical method that decomposes a matrix A into two components: an orthogonal matrix Q and an upper triangular matrix R. Mathematically, this is presented as:
\[ A = QR \]
Orthogonal matrices, which have the property that their transpose is equal to their inverse (Q^T = Q^{-1}), are fundamental in ensuring that the lengths of vectors are preserved during transformations. They enable meaningful rotation and reflection operations in multidimensional spaces.
In contrast, an upper triangular matrix contains zeros below its diagonal, which simplifies solving systems of linear equations. The combination of these two matrices provides powerful tools for numerical computation.
Geometric Insight of QR Factorization
Geometrically, QR factorization can be viewed as the process of expressing a set of vectors as a combination of orthogonal components. When performing QR decomposition, we envision the space being transformed into a new basis created by the orthogonal vectors represented in matrix Q.
This technique is especially significant in least squares problems, where we seek the best-fit solution to an overdetermined system of equations. QR factorization paves the way to find the solution efficiently while minimizing numerical errors.
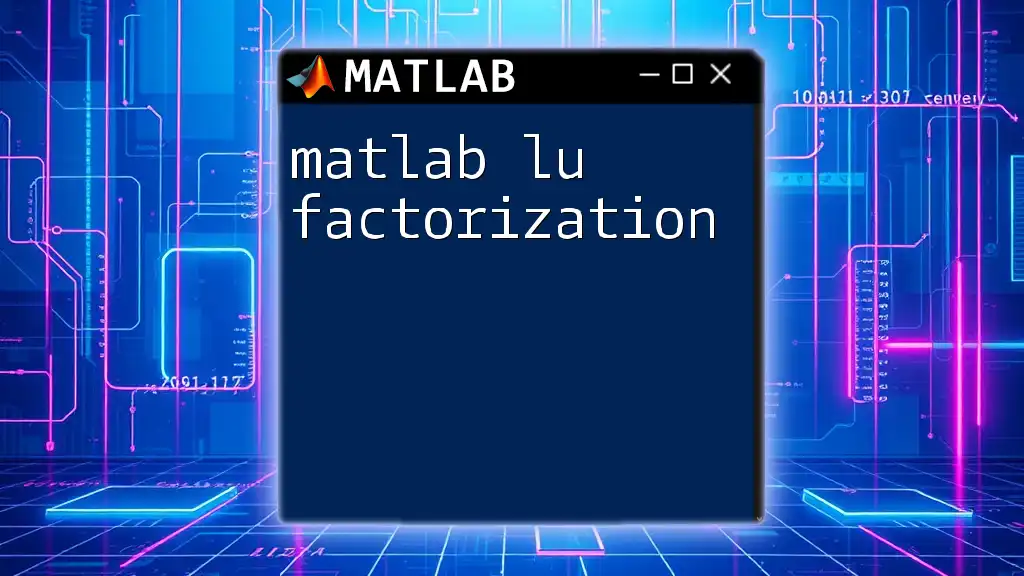
Implementing QR Factorization in MATLAB
MATLAB Functions for QR Factorization
MATLAB provides a straightforward built-in function for QR factorization. The `qr()` function performs both the decomposition and returns the matrices Q and R quickly and efficiently. The syntax of this function is as follows:
[Q, R] = qr(A);
This indicates that you input your matrix A and receive both Q and R as output.
Step-by-Step Code Example
To illustrate how QR factorization works in MATLAB, let's start with a simple matrix. Consider the following matrix:
A = [1, 2; 3, 4; 5, 6];
Using the QR function, we can decompose matrix A:
[Q, R] = qr(A);
After running this code, we will obtain matrices Q and R. For a deeper understanding, let’s take a moment to analyze these outputs.
Breakdown of the Output
The matrix Q will consist of orthogonal columns, meaning that when you take any two columns, their dot product will be zero (indicative of orthogonality). You can verify this property using:
orthogonality_check = Q' * Q;
This should yield an identity matrix if Q is orthogonal.
On the other hand, the matrix R will consist of values that layout in upper triangular form, retaining the core structure of the original matrix A in a simplified manner. Such a structure is advantageous for solving systems of equations, as it allows for back substitution methods.
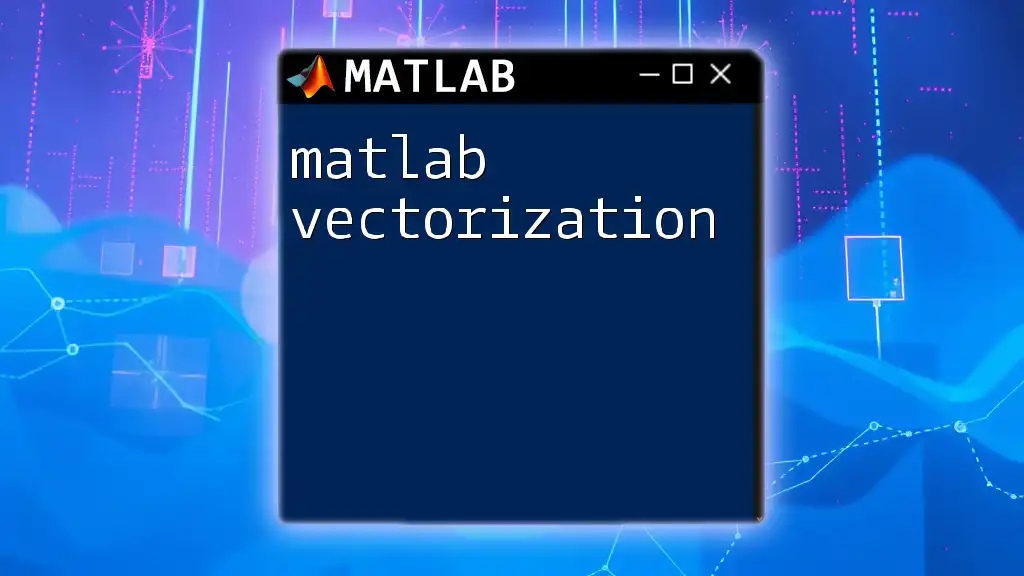
Practical Applications of QR Factorization
Solving Least Squares Problems
QR factorization is particularly useful in solving least squares problems, where the goal is to minimize the error in approximating a set of equations. Given an overdetermined linear system \(Ax = b\), we can use QR to reframe the problem in a manner that is both computationally efficient and numerically stable.
Consider an example matrix b:
b = [1; 2; 3];
To find the least squares solution \(x\), we can express it as:
x = R \ (Q' * b);
Here, \(Q'\) signifies the transpose of matrix Q, and we are solving a triangular system. This example exhibits QR factorization's strength in transforming complex problems into simpler, manageable components.
Eigenvalue Problems
In addition to least squares problems, QR factorization plays a critical role in eigenvalue calculations. The iterative QR algorithm utilizes this decomposition to compute the eigenvalues of a matrix.
The method involves calculating the QR factorization iteratively and updating the original matrix. This process converges to an upper triangular matrix, where the diagonal elements represent the eigenvalues. The ability to efficiently find eigenvalues has broad implications across various scientific and engineering domains.
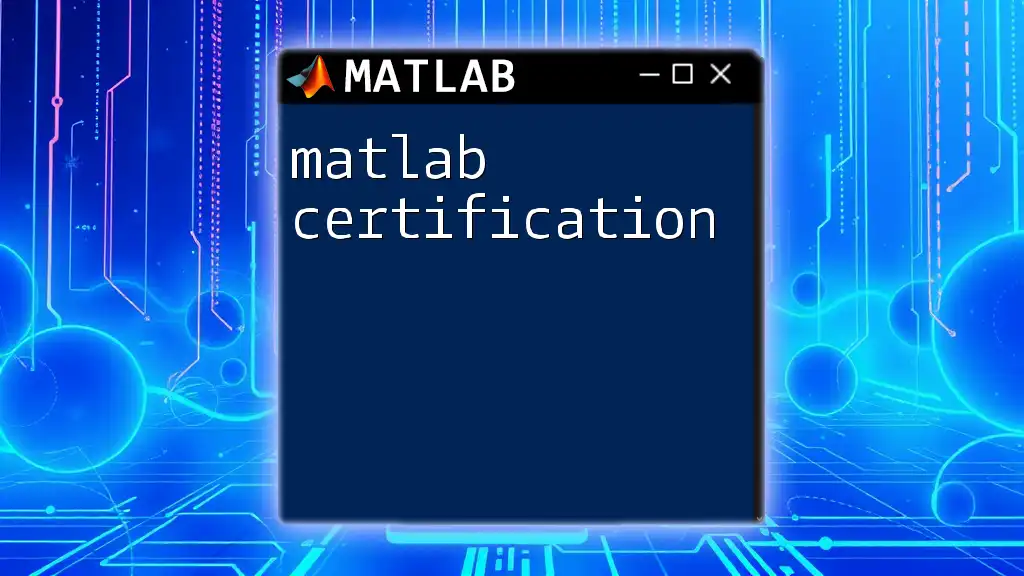
Enhancing QR Factorization Techniques
Variants of QR Factorization
QR factorization offers several variations that further enhance its efficiency and applicability:
-
Modified Gram-Schmidt Process: This method refines the standard Gram-Schmidt approach, focusing on improved numerical stability. You can implement this in MATLAB, though the built-in `qr()` function is usually sufficient due to its robustness.
-
Householder Reflections: This approach utilizes reflections to progressively zero out sub-diagonal elements, achieving the same QR decomposition but often with greater accuracy for ill-conditioned matrices. In MATLAB, Householder reflections can be implemented manually, providing flexibility for advanced use cases.
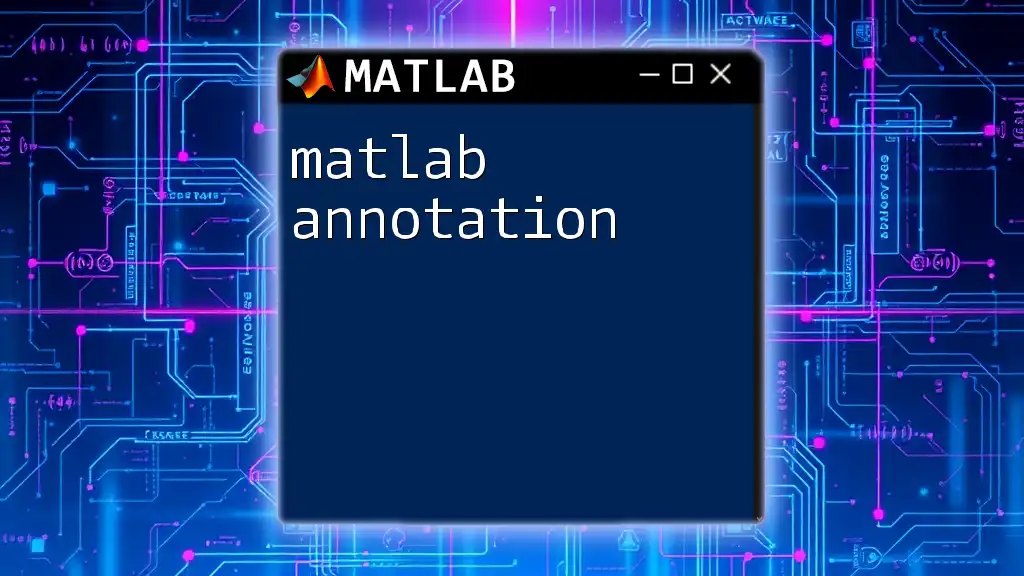
Common Errors and Troubleshooting
Errors to Watch Out For
While QR factorization in MATLAB is generally robust, users may encounter common errors, especially when working with ill-conditioned matrices or singular systems. It's essential to be wary of:
-
Inaccurate results due to floating-point precision: Understanding the limits of numerical representation in computing can help frame your expectations.
-
Non-unique solutions for underdetermined systems: Recognizing the unique challenges posed by such systems can guide considerations for method selection.
Tips for Debugging
When debugging QR factorization implementations, consider using MATLAB's built-in diagnostic tools. Check conditions using:
cond_A = cond(A);
A high condition number indicates potential numerical instability, guiding users to reconsider their methods or input data. Additionally, visualizing output matrices can uncover hidden patterns or anomalies, allowing for a deeper understanding of the decomposition.
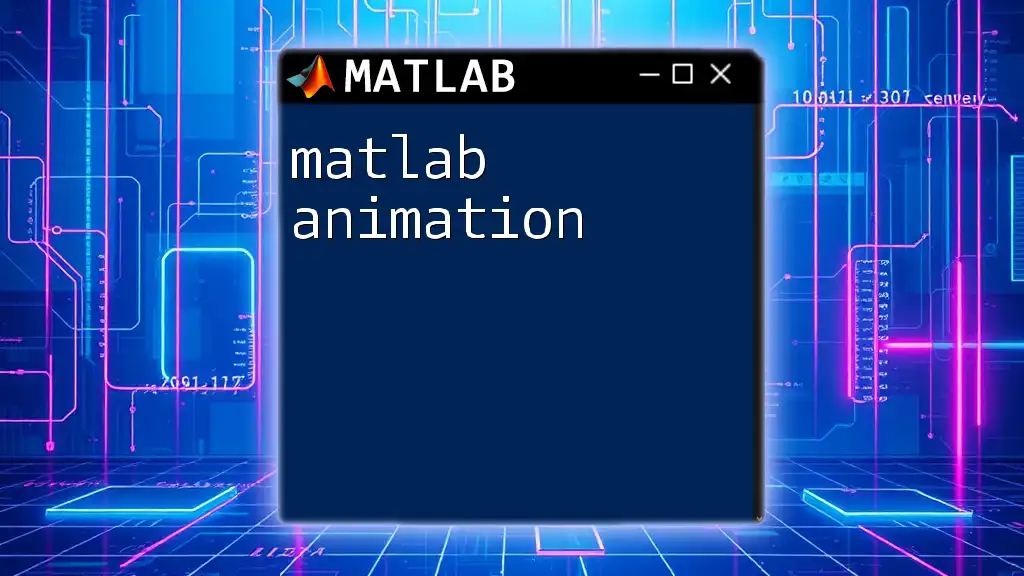
Conclusion
QR factorization is an essential tool in a data scientist's toolkit, facilitating numerous applications in numerical analysis, from solving complex equations to improving computational efficiency in eigenvalue problems. Mastering MATLAB QR factorization not only enhances your programming skills but also opens up avenues for more sophisticated analyses in your projects. Embrace the power of QR factorization, experiment with different datasets, and experience the impact it can have on your data-driven solutions.
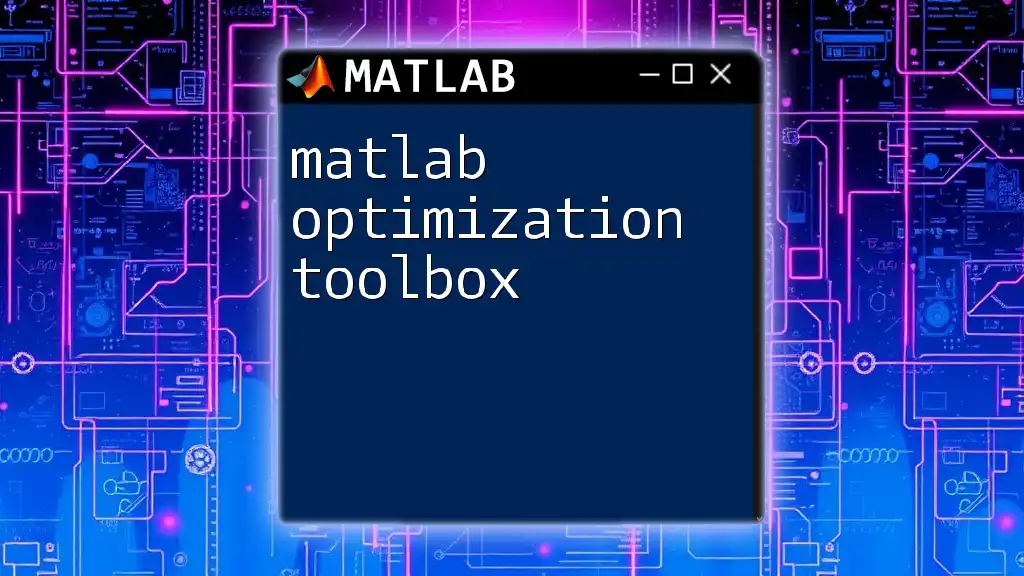
Additional Resources
For those eager to dive deeper into QR factorization, consider consulting the official MATLAB documentation for in-depth explanations and additional functions. Supplement your learning with recommended textbooks and online courses that delve into numerical methods, linear algebra, and MATLAB programming, laying a solid foundation for your exploration of these techniques.